Operators in C++ are special symbols that perform operations on variables and values, such as arithmetic, comparison, and logical operations.
Here’s a code snippet demonstrating some basic operators:
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 5;
// Arithmetic Operators
int sum = a + b; // Addition
int product = a * b; // Multiplication
// Comparison Operators
bool isEqual = (a == b); // Equal to
// Logical Operators
bool result = (a > b) && (b > 0); // Logical AND
cout << "Sum: " << sum << endl;
cout << "Product: " << product << endl;
cout << "Is a equal to b? " << (isEqual ? "Yes" : "No") << endl;
cout << "Result of logical operation: " << (result ? "True" : "False") << endl;
return 0;
}
What Are Operators in C++?
Operators in C++ are special symbols or keywords that perform operations on variables and values. They can be thought of as the building blocks of any C++ program, enabling us to manipulate data and perform calculations. Understanding operators is crucial not only for beginners but also for experienced programmers who want to write efficient and effective code.
C++ operators fall into several categories, each serving different purposes. These categories include:
- Unary Operators: Operate on a single operand.
- Binary Operators: Operate on two operands.
- Ternary Operators: Operate on three operands.
You will find that different programming languages have similar operators with sometimes varying syntax or behavior, which is key to understanding and applying them effectively in C++.

Types of Operators in C++
Arithmetic Operators
Arithmetic operators are used to perform mathematical operations. They include the following:
- Addition: `+`
- Subtraction: `-`
- Multiplication: `*`
- Division: `/`
- Modulus: `%` (returns the remainder of a division)
For example, consider the following code snippet demonstrating these operators:
int a = 10, b = 20;
int sum = a + b; // Sum is 30
int difference = b - a; // Difference is 10
int product = a * b; // Product is 200
int quotient = b / a; // Quotient is 2
int remainder = b % a; // Remainder is 0
Understanding operator precedence is essential, as it determines the order in which operations are performed. For instance, multiplication and division have higher precedence compared to addition and subtraction.
Relational Operators
Relational operators are used to compare two values and return a Boolean result (`true` or `false`). The main relational operators are:
- Equal to: `==`
- Not equal to: `!=`
- Greater than: `>`
- Less than: `<`
- Greater than or equal to: `>=`
- Less than or equal to: `<=`
Here’s an example of relational operators in action:
if (a == b) {
// This block executes if a is equal to b
} else if (a != b) {
// This block executes if a is not equal to b
}
In practice, relational operators are frequently utilized in conditional statements to control program flow based on comparisons.
Logical Operators
Logical operators are used to combine multiple Boolean expressions. The three main logical operators are:
- Logical AND: `&&`
- Logical OR: `||`
- Logical NOT: `!`
Here is a code example illustrating the use of logical operators:
if (a > 0 && b > 0) {
// This block executes if both a and b are positive
}
if (a < 0 || b < 0) {
// This block executes if either a or b is negative
}
if (!condition) {
// This block executes if the condition is false
}
One important aspect of logical operators is short-circuit evaluation, where the second operand is only evaluated if necessary. For instance, if the first condition of an AND operator is false, the overall expression cannot be true, and the second condition won’t be evaluated.
Bitwise Operators
Bitwise operators operate on the binary representations of integers. They are particularly useful for low-level programming tasks and performance optimizations. The main bitwise operators include:
- Bitwise AND: `&`
- Bitwise OR: `|`
- Bitwise XOR: `^`
- Bitwise NOT: `~`
- Left Shift: `<<`
- Right Shift: `>>`
Here is an example that showcases various bitwise operations:
int x = 5; // Binary: 0101
int y = 3; // Binary: 0011
int result_and = x & y; // Result: 0001 (1 in decimal)
int result_or = x | y; // Result: 0111 (7 in decimal)
int result_xor = x ^ y; // Result: 0110 (6 in decimal)
Understanding bitwise operations is crucial when performance is a priority, especially in systems programming, networking, and graphics programming.
Assignment Operators
Assignment operators are used to assign values to variables. They can also perform operations simultaneously. The primary assignment operator is `=`, but there are also several compound assignment operators:
- Addition Assignment: `+=`
- Subtraction Assignment: `-=`
- Multiplication Assignment: `*=`
- Division Assignment: `/=`
- Modulus Assignment: `%=`
Here’s how you might use assignment operators in C++:
int c = 10;
c += 5; // c now equals 15
c -= 2; // c now equals 13
These compound operators simplify code by reducing the need for repetitious expressions, enhancing readability.
Increment and Decrement Operators
Increment and decrement operators are shorthand ways to increase or decrease integer values by one. They include:
- Increment: `++`
- Decrement: `--`
These operators can be used in two forms: pre-increment and post-increment. The same applies to decrement operators.
Example:
int d = 5;
d++; // Post-increment (d becomes 6)
++d; // Pre-increment (d becomes 7)
These operators are commonly used in loops where values need to be modified frequently, such as counters.
Conditional (Ternary) Operator
The conditional operator, also known as the ternary operator, provides a shorthand way to perform if-else operations in a single line. Its syntax is:
condition ? expression1 : expression2
Here's a practical example:
int max = (a > b) ? a : b; // max now holds the larger of a or b
The ternary operator can make your code more concise, but use it cautiously, as overusing may lead to reduced readability.
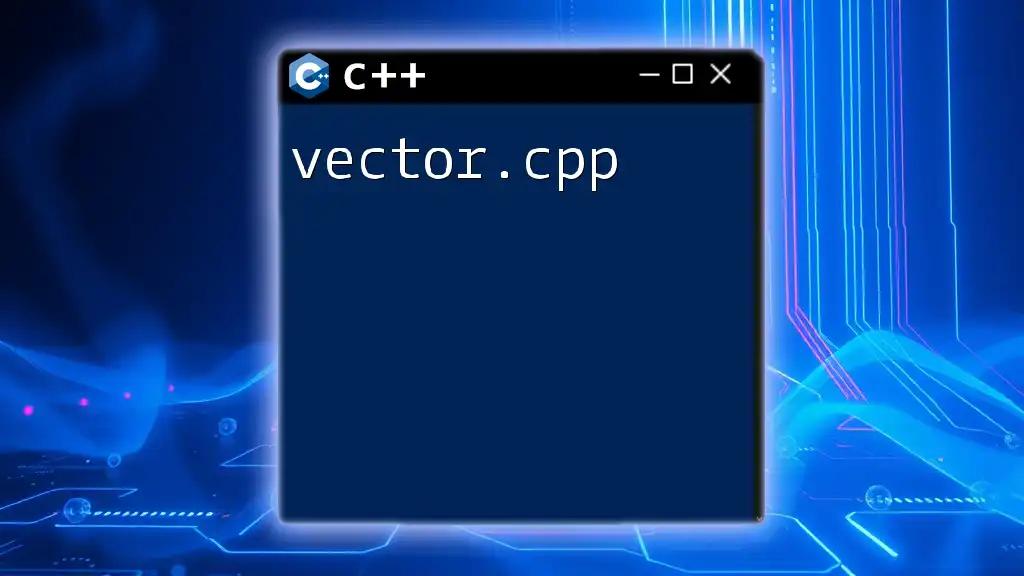
Understanding Operands in C++
Operands are the data items on which operators work. For instance, in the expression `a + b`, both `a` and `b` are operands. Understanding the difference between operators and operands is critical in recognizing how expressions are evaluated.
When writing expressions, ensure that you understand the types of the operands being used, as this will affect the operation's outcome. For example, adding an integer to a float results in a float, leading to potential precision issues.
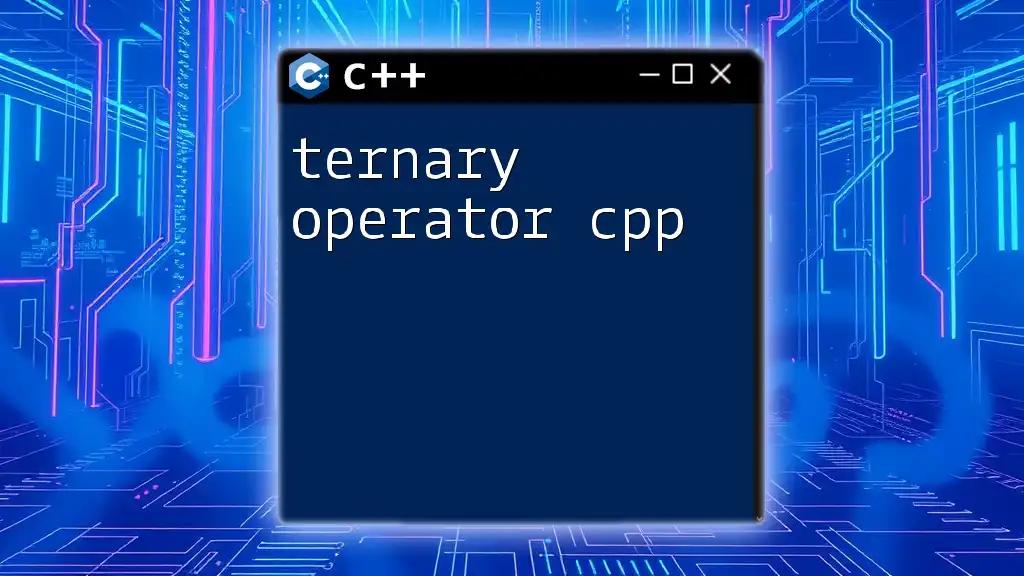
Best Practices for Using Operators in C++
To ensure clarity and good coding practices when using operators, keep the following points in mind:
- Write clear expressions: Break down complex expressions into simpler ones to enhance readability.
- Use parentheses: When necessary, use parentheses to clarify the order of operations, especially when mixing different types of operators.
- Don’t overcomplicate: Avoid using too many operators in one expression, as it might confuse the reader or yourself in the future.
- Comment your code: When using complex logic with multiple operators, add comments to explain what the code is doing to aid in understanding later.

Common Mistakes with Operators
Understanding operators can come with its own set of challenges. Here are some common mistakes to watch out for:
- Overusing operators: Writing very long expressions with too many operators can be hard to follow. Simplifying your code can help.
- Misunderstanding precedence and associativity: When you don’t fully grasp how operators relate to each other in terms of execution order, you may get unexpected results. Always refer to operator precedence to clarify.
- Incorrectly using increment/decrement operators: Confusing pre-increment with post-increment can lead to off-by-one errors, especially in loops and complex expressions.

Conclusion
Mastering operators in C++ is foundational for creating efficient and clear code. By understanding and applying the various types of operators correctly, you can enhance both the functionality and readability of your programs. Regular practice and engagement with coding challenges will deepen your familiarity and confidence with operators, making your programming journey more successful.

Additional Resources
For further reading on C++ operators, explore the official C++ documentation and various online programming tutorials that offer hands-on practice with coding challenges. Familiarizing yourself with practical applications of operators in real-world coding scenarios will reinforce your skills and broaden your understanding of this essential topic.