The keyword operator in C++ allows you to define or overload operators for custom types, enabling you to specify how operators like +, -, and [] behave when applied to user-defined objects.
Here is an example of overloading the + operator for a simple Vector class:
#include <iostream>
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
// Overload the + operator
Vector operator+(const Vector& other) {
return Vector(this->x + other.x, this->y + other.y);
}
};
int main() {
Vector v1(1, 2);
Vector v2(3, 4);
Vector v3 = v1 + v2; // Uses the overloaded + operator
std::cout << "Result: (" << v3.x << ", " << v3.y << ")" << std::endl; // Output: Result: (4, 6)
return 0;
}
Understanding C++ Keywords
Keywords are the reserved words in C++ that have special meaning to the compiler. These words are integral to the language's syntax and structure, serving as the foundation upon which all C++ programs are built. Understanding keywords is crucial for writing effective C++ code, as they dictate how the program behaves and understands data.
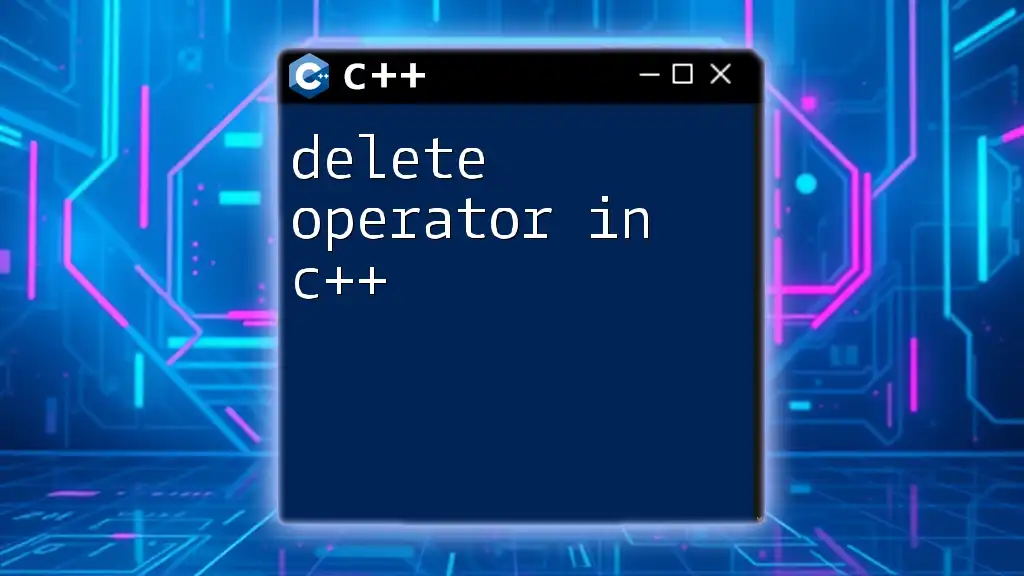
What Are Operator Keywords?
Operator keywords are a subset of keywords that pertain specifically to operations on data. They allow developers to manipulate data types and create dynamic, functional applications. It’s important to differentiate between general keywords and operator keywords; while all operator keywords are keywords, not all keywords are operators.
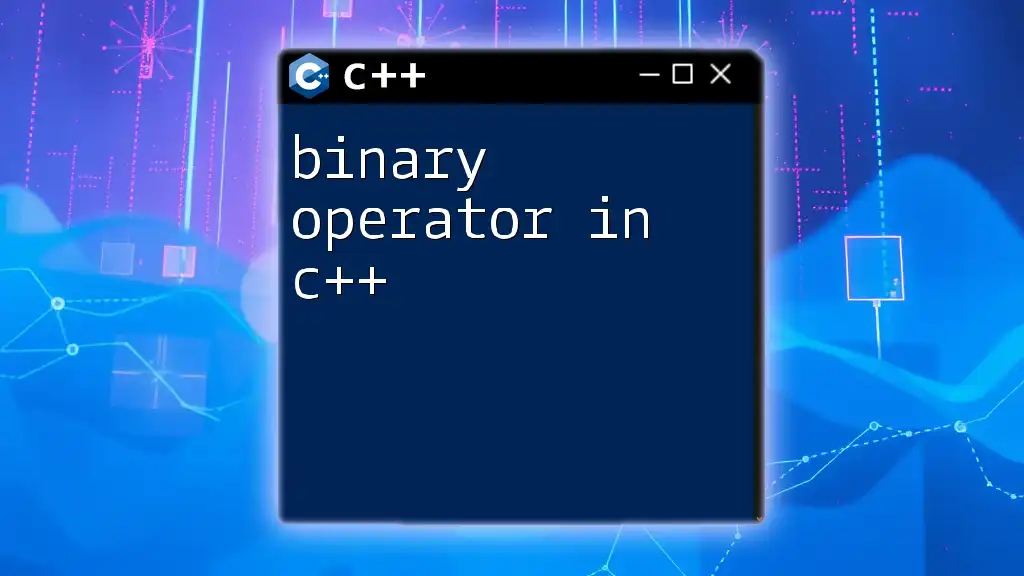
Keyword Operator in C++
Definition of the Keyword Operator
The keyword operator in C++ enables programmers to perform operations on variables and values, using operators that the compiler recognizes. These operators can be unary, binary, or ternary and can perform a wide variety of tasks, from simple mathematical calculations to complex logical comparisons.
Types of Keyword Operators
Overview of Keyword Operators
Keyword operators in C++ can be classified into several categories, including:
Unary Operators
Unary operators operate on a single operand. Common unary operators include:
- Increment (`++`): Increases the value of a variable by one.
- Decrement (`--`): Decreases the value of a variable by one.
- Negation (`-`): Returns the opposite of the operand's value.
Example Code: Unary Operators
int a = 5;
int b = ++a; // b is 6, a is 6
int c = --b; // c is 5, b is 5
Binary Operators
Binary operators operate on two operands. Examples include:
- Addition (`+`): Sums two numbers.
- Subtraction (`-`): Finds the difference.
- Multiplication (`*`): Computes the product.
- Division (`/`): Divides one number by another.
Example Code: Binary Operators
int x = 10;
int y = 20;
int sum = x + y; // sum is 30
int product = x * y; // product is 200
Ternary Operators
The ternary operator takes three operands and is often used as a shortcut for an if-else statement. The syntax is: `condition ? true_case : false_case`.
Example Code: Ternary Operator
int a = 5;
int b = 10;
int max = (a > b) ? a : b; // max is 10
How the Keyword Operator Works in C++
Syntax of Keyword Operators
Understanding the syntax of keyword operators is key to using them properly. Each operator comes with a unique syntax structure that the compiler interprets to perform actions on the involved variables.
Examples of Common Operators:
- Assignment Operator (`=`):
int value = 100;
- Comparison Operator (`==`):
if (value == 100) {
// Condition is true
}
Real-life Scenarios Using Keyword Operators
Keyword operators are used extensively in programming to solve real-world problems. For example, in a banking application, you might use:
- Arithmetic operators to calculate transactions.
- Logical operators to validate user inputs.
Example Code: Using Operators in a Banking Context
float balance = 1000.0;
float transaction = 250.0;
if (balance >= transaction) {
balance -= transaction; // Deducting transaction from balance
// Proceed with the transaction
}
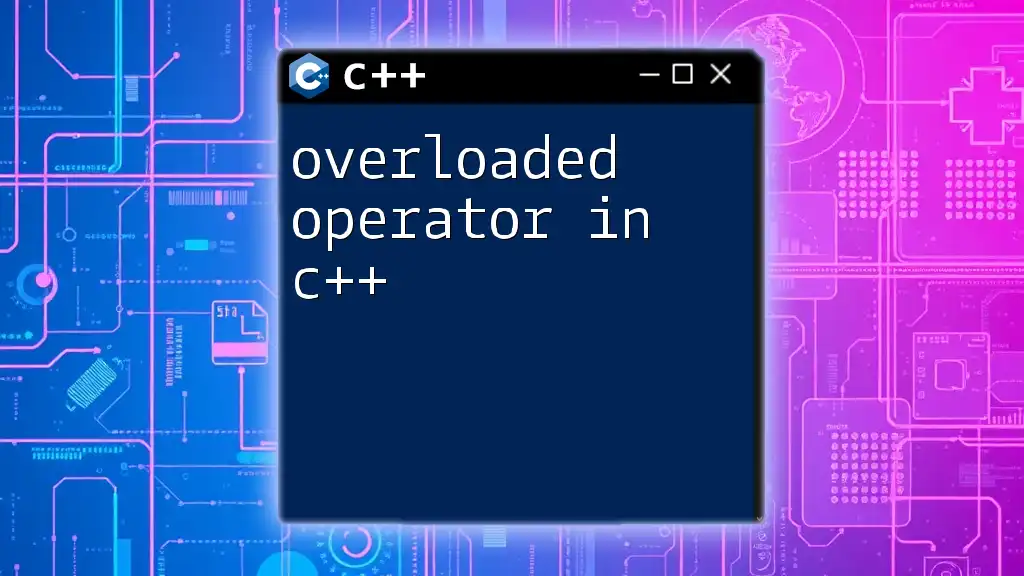
Special Keyword Operators in C++
Overloading Operators
Operator overloading allows C++ developers to redefine how operators work with user-defined types. This capability enhances code readability and usability.
Example Code: Overloading the '+' Operator
class Complex {
public:
float real;
float imag;
Complex operator+(const Complex &obj) {
Complex temp;
temp.real = real + obj.real;
temp.imag = imag + obj.imag;
return temp;
}
};
In this example, the `+` operator has been overloaded to work with `Complex` objects.
Pointer Operators
Pointer operators are essential in C++ for memory management. The two primary pointer operators are:
- Dereference Operator (`*`): Used to access the value at the address pointed to by a pointer.
- Address-of Operator (`&`): Returns the address of a variable.
Example Code: Using `*` and `&` Operators
int var = 20;
int *ptr = &var; // ptr now holds the address of var
int value = *ptr; // value is 20
Scope Resolution Operator
The scope resolution operator allows access to global variables, class members, or functions that are hidden due to name conflicts.
Example Code: Using the '::' Scope Resolution Operator
int x = 5;
namespace MyNamespace {
int x = 10;
}
int main() {
cout << ::x; // Outputs: 5
cout << MyNamespace::x; // Outputs: 10
}
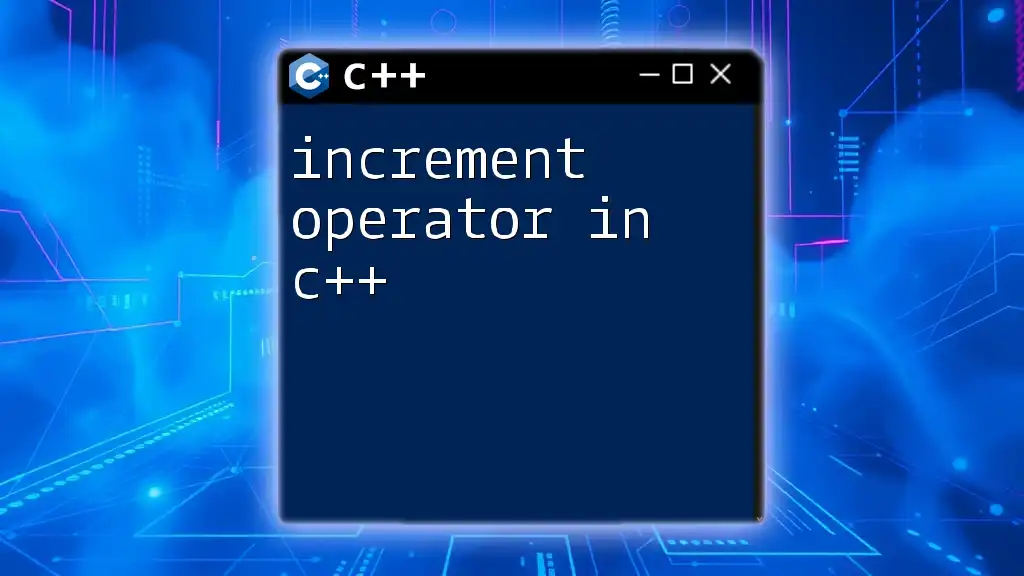
Common Mistakes and Best Practices
Misusing Keyword Operators
One common pitfall is misunderstanding operator precedence, which can lead to unexpected results. It's crucial to know that operations with higher precedence are evaluated first, and failing to recognize this can produce errors in logic.
How to Avoid These Mistakes
To avoid common mistakes, follow these guidelines:
- Familiarize yourself with operator precedence and associativity.
- Use parentheses to clarify operations when in doubt.
Best Practices for Using Keyword Operators
- Write clear and understandable code using keyword operators.
- Avoid overly complex expressions; favor simpler, more straightforward implementations.
- Comment on tricky logic to ensure maintainability.
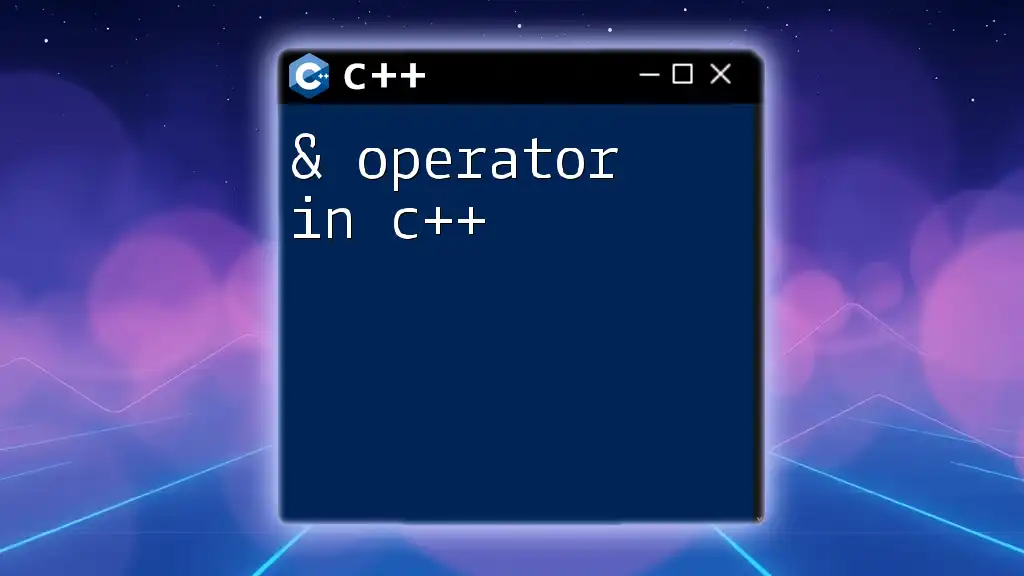
Performance Considerations
Efficiency of Keyword Operators
Using keyword operators efficiently can significantly enhance program performance. However, some operators, such as overloaded ones, can incur overhead if not used judiciously.
Example Code: Benchmark Comparisons of Keyword Operators
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Perform operations using keyword operators
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> diff = end - start;
std::cout << "Elapsed time: " << diff.count() << " seconds\n";
}
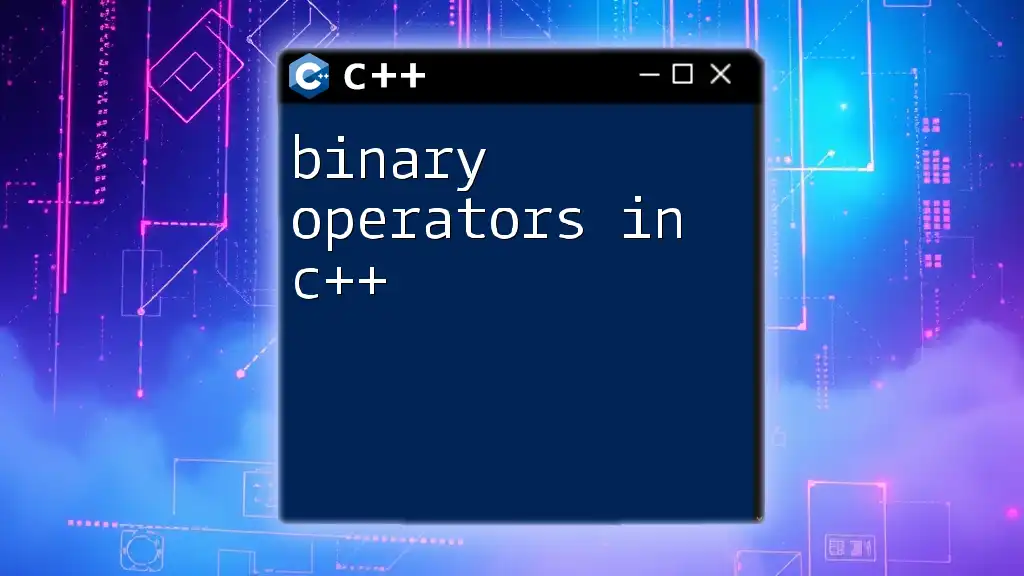
Conclusion
Understanding the keyword operator in C++ is vital for any C++ developer. By mastering the various types of keyword operators, their syntax, and common practices, programmers can write efficient, readable code. Not only do keyword operators provide clarity, but they also facilitate complex operations that are common in today's programming tasks. To truly excel in C++, practice using these operators in various scenarios and explore more about them.
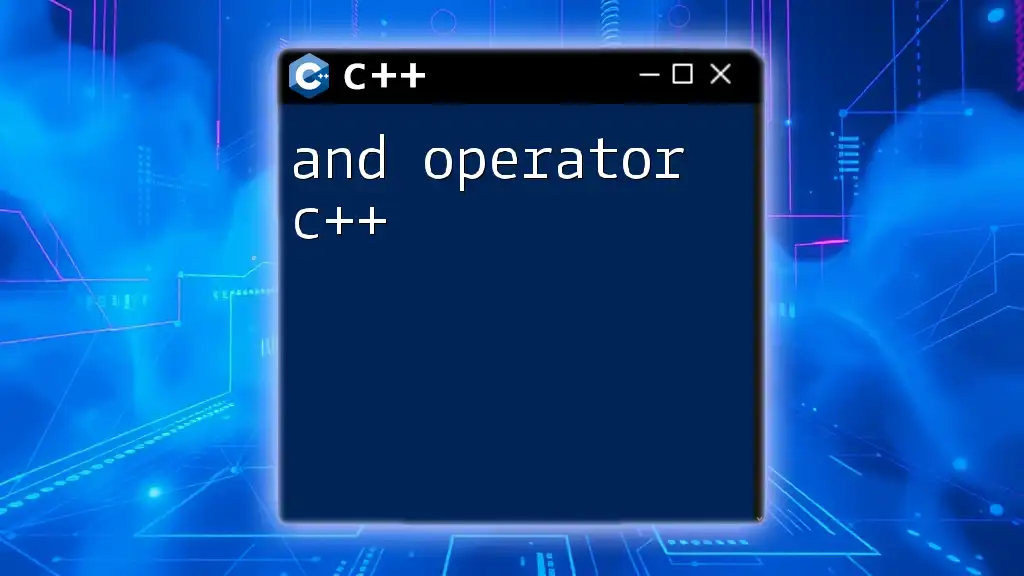
Additional Resources
Recommended Readings
Consider reading resources such as "The C++ Programming Language" by Bjarne Stroustrup for a comprehensive dive into C++ keywords and operators.
Online Courses and Tutorials
Explore platforms like Coursera and Udacity, which offer structured courses on C++ programming, focusing on keywords and operator usage.
Community and Forums
Engage with C++ forums like Stack Overflow or Reddit’s r/cpp community where you can ask questions, share knowledge, and learn from fellow enthusiasts.