In C++, a binary operator is an operator that takes two operands and performs a specific operation on them, such as addition, subtraction, multiplication, or logical comparisons.
Here's a code snippet demonstrating the use of a binary operator:
#include <iostream>
int main() {
int a = 10, b = 5;
int sum = a + b; // '+' is the binary operator for addition
std::cout << "The sum of a and b is: " << sum << std::endl;
return 0;
}
Overview of Operators in C++
In programming, operators are symbols that perform specific operations on variables and values. In C++, there are various types of operators, including unary, binary, and ternary operators. Understanding these operators is crucial for effective coding, as they form the foundation of many programming tasks.
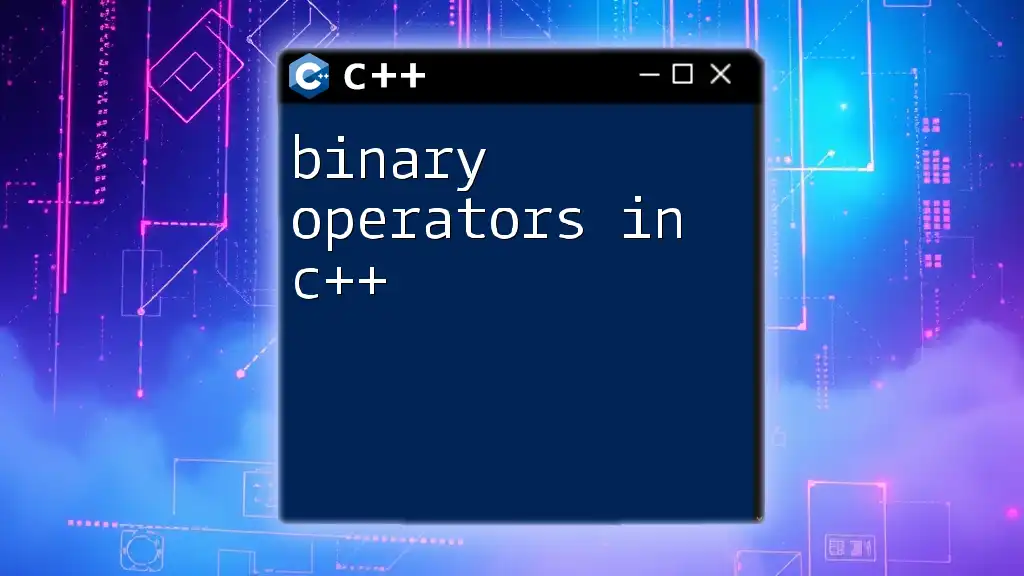
Binary Operators Explained
Binary operators are operators that require two operands to perform an operation. They take two values and apply an operation to produce a result. Every binary operator in C++ operates on two values and returns another value, making them essential for mathematical, logical, and bitwise operations.
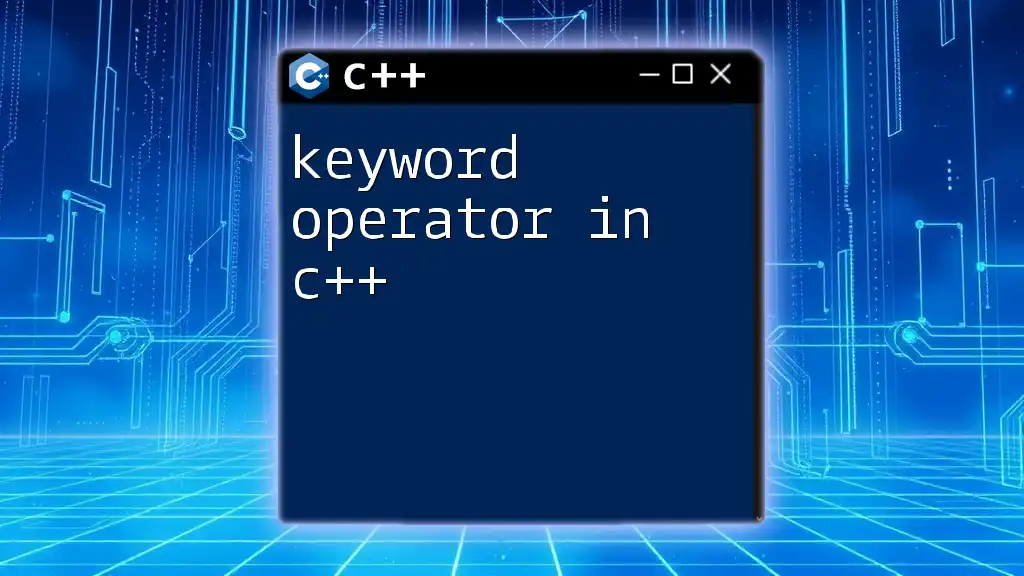
Types of Binary Operators in C++
Arithmetic Operators
Arithmetic operators perform basic arithmetic operations. They are fundamental to numerical computations in C++.
Common arithmetic binary operators include:
-
Addition (+): This operator adds two operands.
int sum = 5 + 3; // sum will be 8
-
Subtraction (-): This operator subtracts the second operand from the first.
int difference = 5 - 3; // difference will be 2
-
Multiplication (*): This operator multiplies two operands.
int product = 5 * 3; // product will be 15
-
Division (/): This operator divides the first operand by the second. If both operands are integers, it performs integer division.
int quotient = 5 / 2; // quotient will be 2
-
Modulus (%): This operator returns the remainder of a division operation.
int remainder = 5 % 2; // remainder will be 1
Relational Operators
Relational operators compare two values and return a boolean result (`true` or `false`).
Common relational binary operators include:
-
Equal to (==): Checks if two operands are equal.
bool isEqual = (5 == 5); // isEqual will be true
-
Not equal to (!=): Determines if two operands are not equal.
bool isNotEqual = (5 != 3); // isNotEqual will be true
-
Greater than (>): Checks if the first operand is greater than the second.
bool isGreater = (5 > 3); // isGreater will be true
-
Less than (<): Checks if the first operand is less than the second.
bool isLess = (5 < 3); // isLess will be false
-
Greater than or equal to (>=): Checks if the first operand is greater than or equal to the second.
bool isGreaterOrEqual = (5 >= 5); // isGreaterOrEqual will be true
-
Less than or equal to (<=): Checks if the first operand is less than or equal to the second.
bool isLessOrEqual = (3 <= 5); // isLessOrEqual will be true
Logical Operators
Logical operators are used to combine or negate conditions, providing more complex control structures.
Common logical binary operators include:
-
Logical AND (&&): Returns true if both operands are true.
bool andCondition = (5 > 3 && 2 < 3); // andCondition will be true
-
Logical OR (||): Returns true if at least one of the operands is true.
bool orCondition = (5 > 3 || 2 > 3); // orCondition will be true
-
Logical NOT (!): Returns true if the operand is false (negation).
bool notCondition = !(5 < 3); // notCondition will be true
Bitwise Operators
Bitwise operators perform operations on binary representations of integers and are crucial for low-level programming.
Common bitwise binary operators include:
-
Bitwise AND (&): Operates on each pair of bits and returns 1 only if both bits are 1.
int andResult = 5 & 3; // Bits: 0101 & 0011 = 0001 (1)
-
Bitwise OR (|): Returns 1 if at least one of the bits is 1.
int orResult = 5 | 3; // Bits: 0101 | 0011 = 0111 (7)
-
Bitwise XOR (^): Returns 1 if the bits are different.
int xorResult = 5 ^ 3; // Bits: 0101 ^ 0011 = 0110 (6)
-
Left Shift (<<): Shifts bits to the left, filling new bits with 0 and effectively multiplying the number by 2.
int leftShiftResult = 5 << 1; // 0101 becomes 1010 (10)
-
Right Shift (>>): Shifts bits to the right, discarding bits on the right and effectively performing integer division by 2.
int rightShiftResult = 5 >> 1; // 0101 becomes 0010 (2)
Assignment Operators
Assignment operators are used to assign values to variables, often in combination with arithmetic operations.
Common assignment binary operators include:
-
Simple Assignment (=): Assigns the value of the right operand to the left operand.
int x = 5; // x is assigned the value 5
-
Add and Assign (+=): Adds the right operand to the left operand and assigns the result to the left operand.
x += 3; // x is now 8
-
Subtract and Assign (-=): Subtracts the right operand from the left operand and assigns the result to the left operand.
x -= 2; // x is now 6
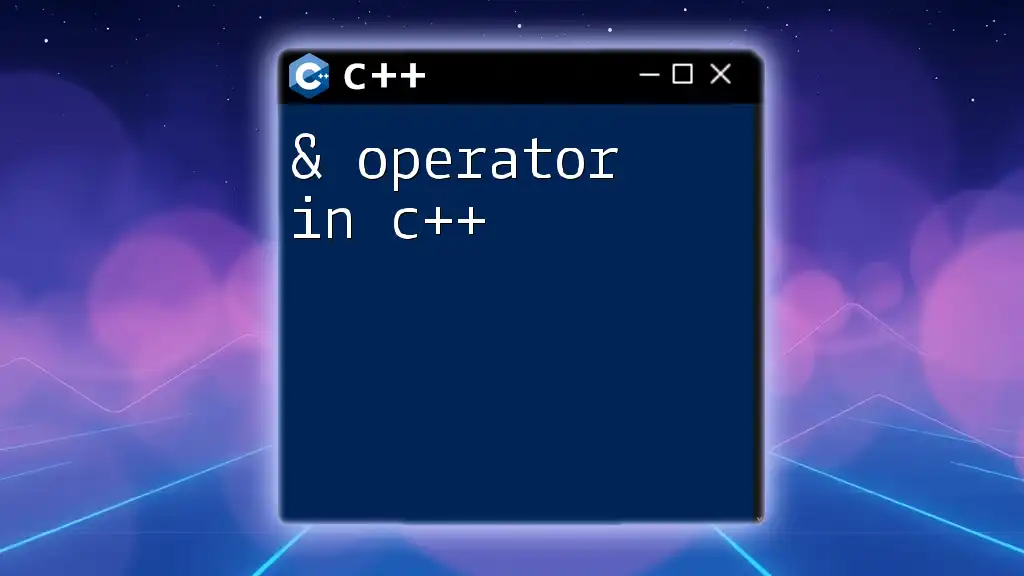
Operator Overloading in C++
Operator overloading allows you to define custom behavior for binary operators with user-defined data types. This feature enhances code readability and provides intuitive ways to interact with objects.
To overload a binary operator in C++, you define a function that specifies the operation you want to perform. Here's an example using the addition operator (+):
class Complex {
public:
int real, imag;
Complex(int r, int i) : real(r), imag(i) {}
// Overloading the + operator
Complex operator+(const Complex& obj) {
return Complex(real + obj.real, imag + obj.imag);
}
};
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
Complex c3 = c1 + c2; // c3 is now Complex(4, 6)
}
In this example, the `operator+` function defines how two `Complex` objects can be added together using the `+` symbol.
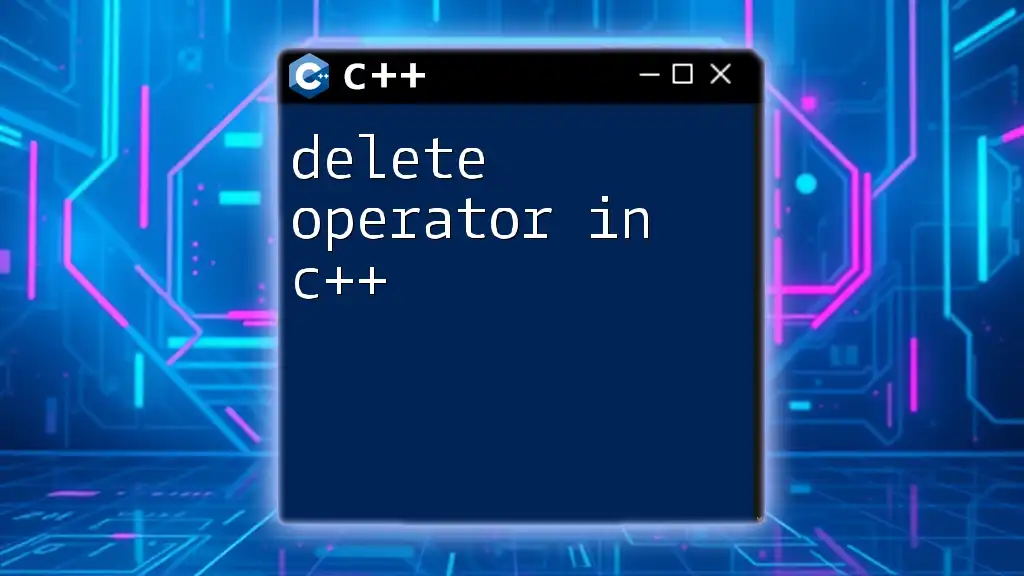
Common Mistakes with Binary Operators
When working with binary operators, it's essential to be cautious, as certain common mistakes can lead to unintended results:
-
Integer Division: When dividing two integers, be aware that C++ performs integer division. If you require a floating-point result, ensure at least one operand is a float or double.
double result = 5 / 2; // result will be 2.0, not 2.5
-
Operator Precedence: Confusing operator precedence can lead to logical errors. Use parentheses to clearly define the intended operation order.
int value = 5 + 3 * 2; // value will be 11, not 16
-
Type Mismatches: Ensure that the operands of binary operators are compatible in type. C++ will not automatically convert types in all cases.
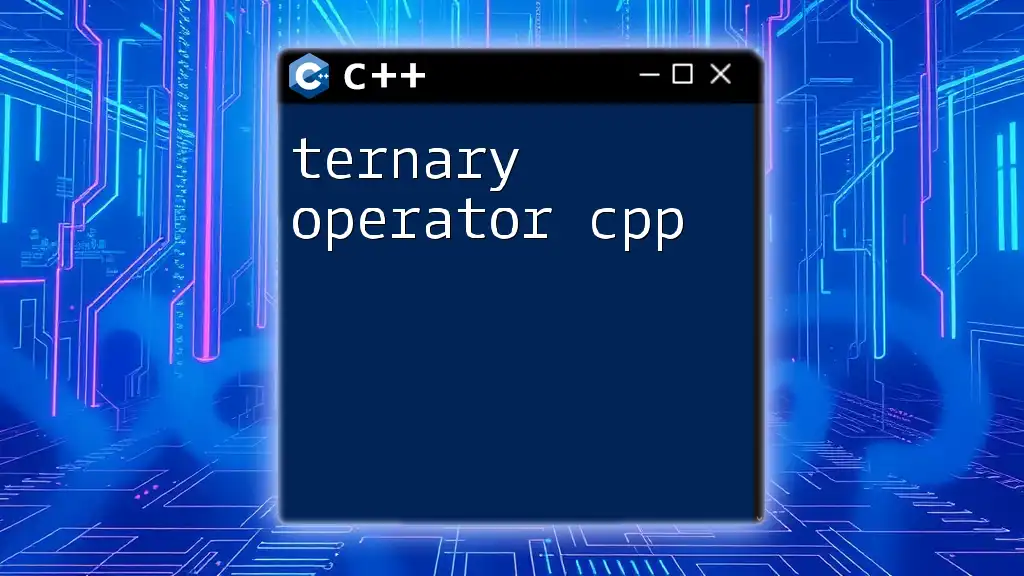
Best Practices for Using Binary Operators
To ensure clean and effective code when using binary operators, follow these best practices:
- Use parentheses judiciously to clarify the order of operations, especially in complex expressions.
- Ensure type compatibility among operands to prevent unintended behavior.
- Favor meaningful variable names and keep operations clear to enhance code readability.
- Practice modular coding by breaking down complex operations into smaller, easier-to-understand functions or expressions.
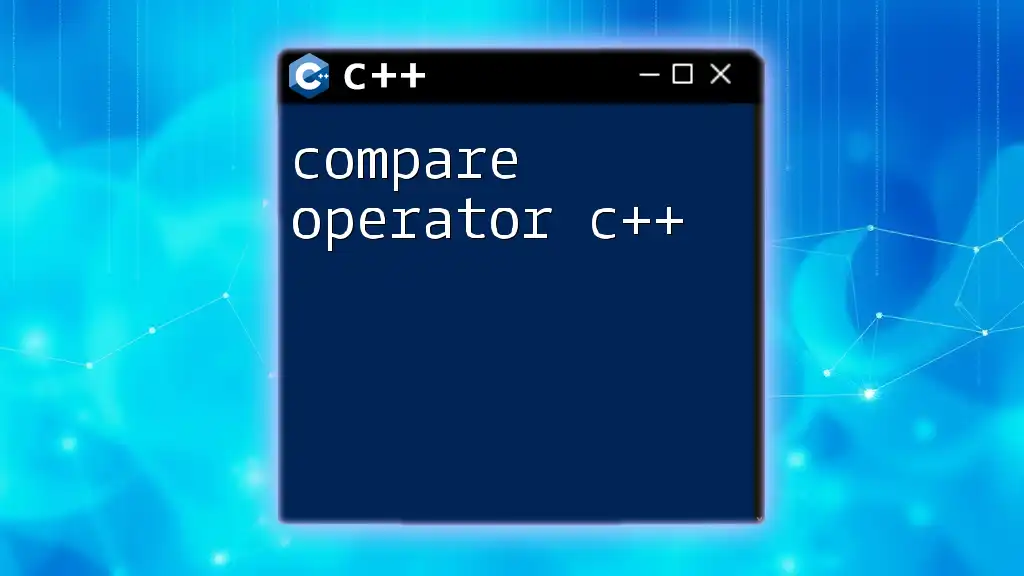
Conclusion
Understanding binary operators in C++ is crucial for any programmer. They serve as the backbone of mathematical calculations, comparisons, and logical decisions in your code. As you practice using these operators and abide by good coding practices, you will become more proficient in C++. Don't hesitate to explore more advanced topics and continue honing your skills in C++ programming.
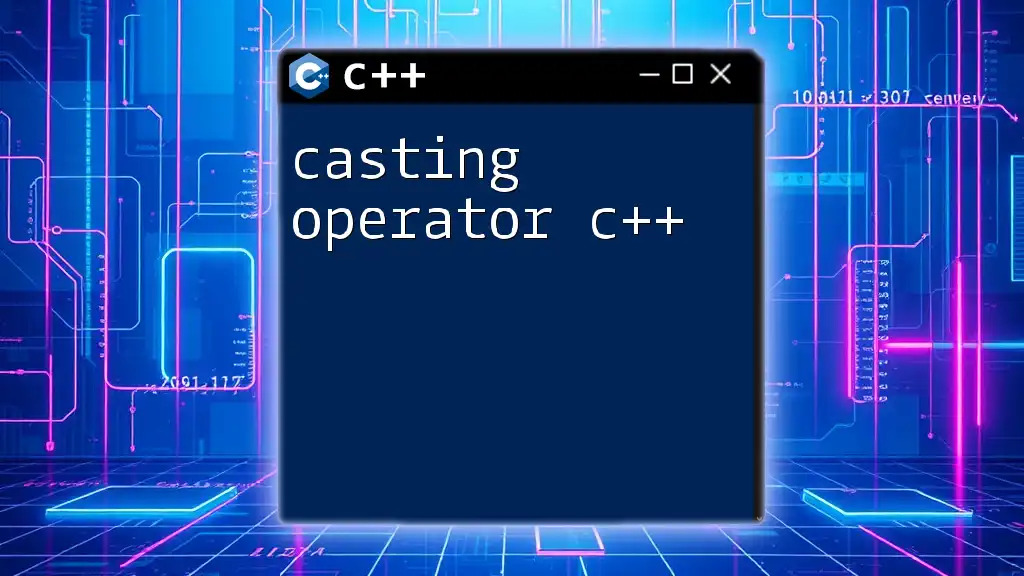
Additional Resources
To further enrich your understanding of binary operators and C++, consider exploring these resources:
- Online C++ programming courses
- Documentation from the C++ standards committee
- C++ coding communities and forums
FAQs
-
What is a binary operator in C++? A binary operator in C++ is an operator that takes two operands and performs an operation.
-
Can I overload binary operators in C++? Yes, C++ allows operator overloading, which lets you define custom behavior for binary operators with your user-defined types.
By understanding and effectively using binary operators, you empower yourself to write more efficient and dynamic code within your C++ applications.