The delete operator in C++ is used to deallocate memory that was previously allocated with the new operator, thereby preventing memory leaks.
int* ptr = new int; // Allocate memory
*ptr = 10; // Assign value
delete ptr; // Deallocate memory
ptr = nullptr; // Set pointer to null to avoid dangling pointer
What is the Delete Operator?
The delete operator in C++ is a crucial component of memory management, specifically designed to deallocate memory that was previously allocated using the `new` keyword. Understanding how and when to use it is essential for writing robust, efficient, and error-free code.
Differences between `delete` and `delete[]`
It's essential to differentiate between the two variants of the delete operator: `delete` and `delete[]`. The `delete` operator is used to free memory allocated for a single object, while `delete[]` is meant for arrays of objects. Using `delete` on an array can lead to undefined behavior, making it imperative to use the correct operator based on your allocation method.
Situations When to Use Delete
You should use the delete operator when you no longer need a dynamically allocated object, allowing the memory used by that object to be reallocated for other purposes. Using delete helps avoid memory leaks, which occur when allocated memory is no longer accessible but not released.
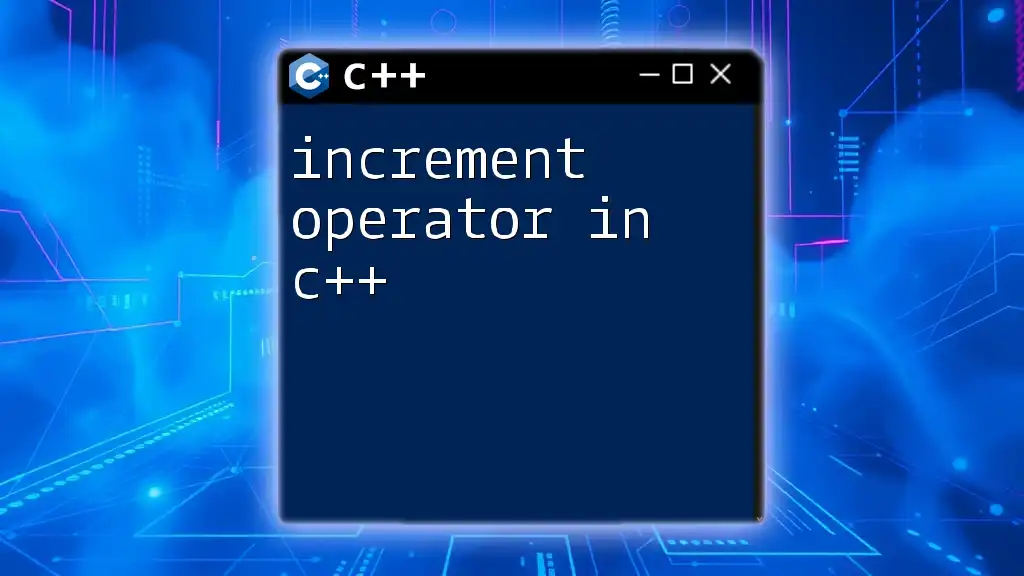
Memory Management in C++
Dynamic vs. Static Memory Allocation
In C++, memory can be allocated either statically or dynamically. Static memory allocation occurs at compile time, where the operating system allocates memory for variables based on their type and scope. On the other hand, dynamic memory allocation occurs at runtime using the `new` operator, giving you flexibility in memory management based on the program's needs.
Benefits of dynamic memory allocation include:
- The capability of allocating larger amounts of memory.
- The ability to request and release memory at any time during program execution.
- Increased adaptability for complex data structures like linked lists, trees, and graphs.
Common Memory Mismanagement Issues
C++ programmers must be vigilant to avoid common memory mismanagement issues that can lead to problems in their applications:
- Memory Leaks: Occur when memory allocated with `new` is not deallocated with `delete`, resulting in gradual memory consumption which can degrade performance.
- Dangling Pointers: These are pointers that point to memory that has already been freed, leading to potential crashes or unexpected behavior.
- Double Deletion: When a pointer is deleted multiple times, it can lead to corruption of the memory heap, causing unpredictable results.
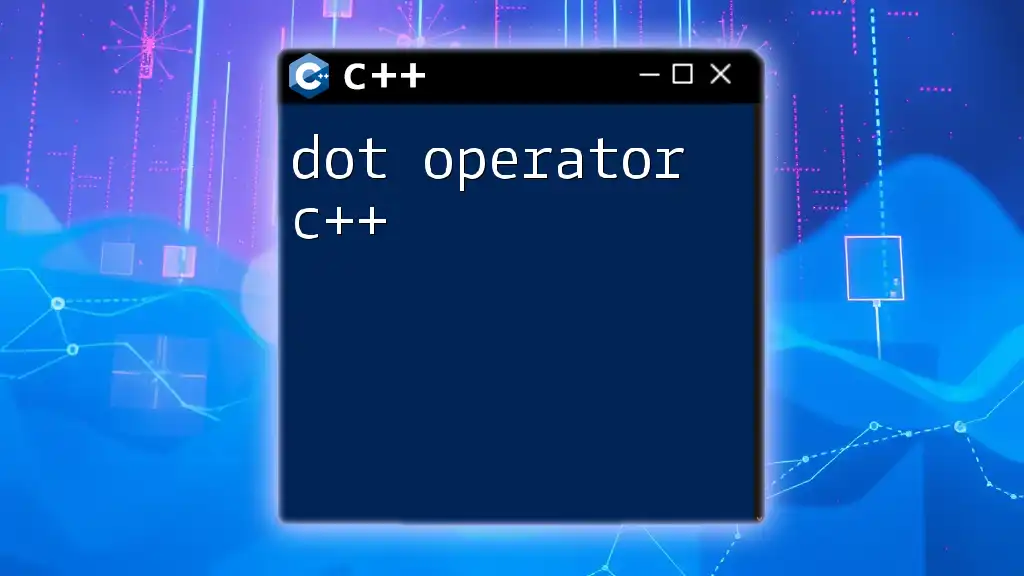
Syntax of the Delete Operator
Using `delete`
The syntax for the delete operator is straightforward:
delete pointer_variable;
Here's a brief example showcasing its use:
int* ptr = new int(5); // dynamically allocate memory for an integer
delete ptr; // deallocates memory, preventing memory leaks
Using `delete[]`
For arrays, the syntax is slightly different:
delete[] pointer_variable;
This snippet demonstrates how to correctly delete an array:
int* arr = new int[10]; // dynamically allocate an array of integers
delete[] arr; // deallocates the entire array
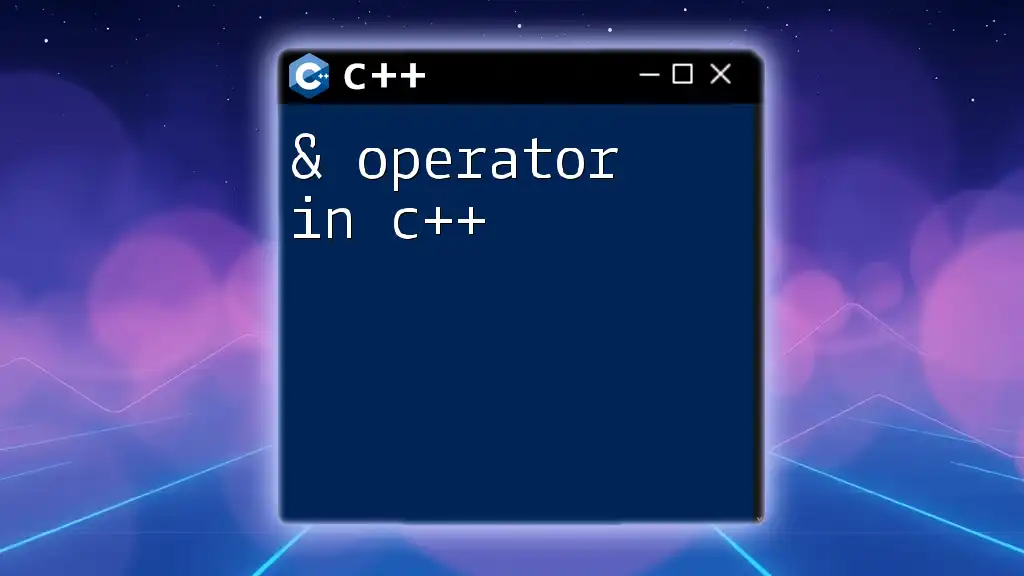
How to Properly Use the Delete Operator
Best Practices for Using Delete
Implementing best practices when using the delete operator is crucial to maintaining healthy memory management:
-
Always Check for Null Pointers: Before using `delete`, it’s wise to verify that the pointer is not `nullptr`. This prevents undefined behavior, as deleting a null pointer is safe but may lead to incorrect assumptions if the pointer was pointing to a valid object.
-
Avoid Using `delete` on Pointers Not Allocated with `new`: It's essential to delete only pointers that were allocated using `new`. Failing to do so can lead to program crashes or memory corruption.
-
Set Pointers to `nullptr` After Deletion: After deleting a pointer, it's a good practice to set it to `nullptr` to prevent accidental use:
int* ptr = new int(10); delete ptr; // proper deallocation ptr = nullptr; // prevents dangling pointer
Managing Resources in Class Constructors and Destructors
Utilizing the delete operator effectively is critical in class management. Implementing RAII (Resource Acquisition Is Initialization) ensures that resources are tied to the lifespan of objects.
Consider the following example showing proper resource management:
class MyClass {
public:
MyClass() {
// resource allocation
data = new int(42); // memory allocated
}
~MyClass() {
// resource deallocation
delete data; // memory freed in destructor
}
private:
int* data; // pointer to dynamically allocated memory
};
In this scenario, when an object of `MyClass` goes out of scope, its destructor is called, guaranteeing that the allocated memory is freed.
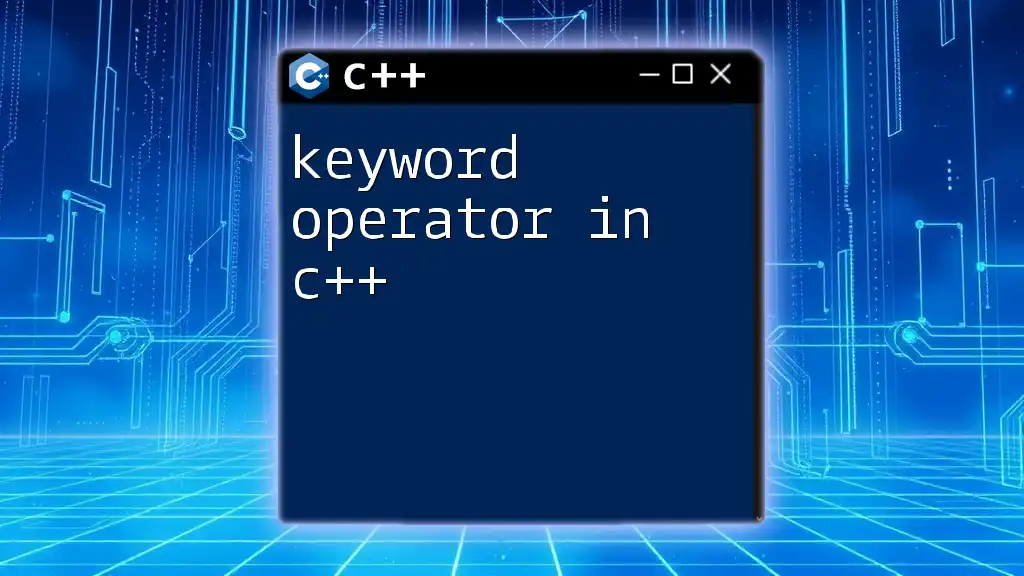
Common Mistakes with the Delete Operator
Forgetting to Delete
One of the most prevalent issues is forgetting to release allocated memory, leading to memory leaks. For instance:
int* leak = new int(100); // memory allocated
// No delete statement, leading to a memory leak
Using Delete Incorrectly
Another critical mistake is double deletion, which can lead to undefined behavior. A simple example is:
int* data = new int(30);
delete data; // first deletion
delete data; // unsafe, leads to undefined behavior
In this case, the program attempts to free already deleted memory, which is dangerous.
Managing Arrays and Pointers
It's equally important to manage arrays appropriately. Using `delete` when the correct operator is `delete[]` causes serious issues:
int* dataArray = new int[5]; // dynamic allocation of an array
delete dataArray; // incorrect, leads to undefined behavior
Here, the correct call should have been `delete[] dataArray`.
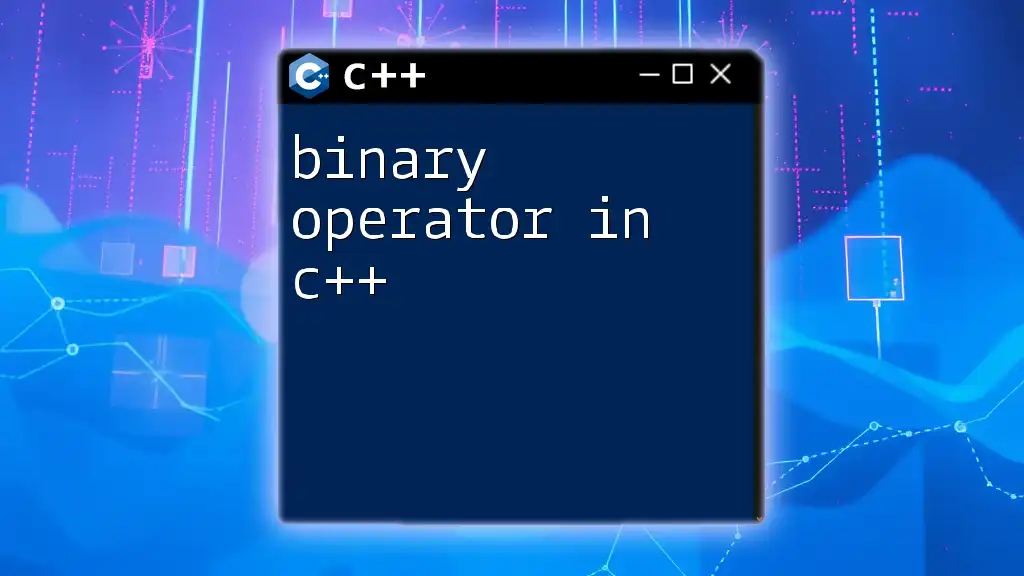
Advanced Topics
Smart Pointers as an Alternative
With the advent of smart pointers in C++11, memory management became simpler and less error-prone. Example types include `unique_ptr` and `shared_ptr`, which automatically handle memory deallocation.
Here's a simple usage example:
#include <memory>
std::unique_ptr<int> smartPtr(new int(20)); // memory automatically freed
Using smart pointers helps prevent many common memory-related errors.
Custom Deleters
Smart pointers offer the flexibility of using custom deleters for specialized deallocation needs. Here’s an example:
auto customDeleter = [](int* ptr) {
// custom deallocation logic
delete ptr;
};
std::shared_ptr<int> p(new int(50), customDeleter); // automatic memory management
This approach gives programmers control over how their resources are cleaned up while still benefiting from smart pointers’ safety.
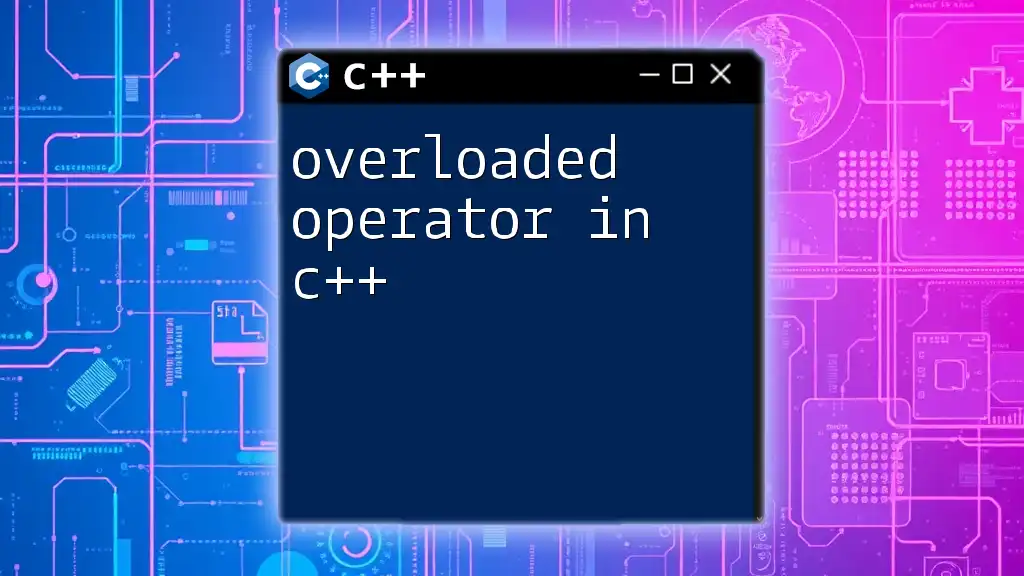
Conclusion
Understanding the delete operator in C++ is vital for effective memory management. Following best practices and avoiding common pitfalls can help you write cleaner, more efficient code. By leveraging concepts like RAII and smart pointers, you can significantly reduce the risk of memory leaks and other issues associated with manual memory management. Embrace these techniques and you'll enhance both your skills and the reliability of your C++ applications.