Overloaded operators in C++ allow you to define custom behavior for operators (like +, -, *, etc.) when they are used with user-defined data types (classes).
Here's a simple code snippet demonstrating operator overloading for a `Vector` class:
#include <iostream>
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
// Overloading the + operator
Vector operator+(const Vector& v) {
return Vector(x + v.x, y + v.y);
}
};
int main() {
Vector v1(1, 2);
Vector v2(3, 4);
Vector v3 = v1 + v2; // Calls the overloaded operator+
std::cout << "Result: (" << v3.x << ", " << v3.y << ")" << std::endl; // Outputs: Result: (4, 6)
return 0;
}
What is Operator Overloading?
Operator overloading allows developers to define custom behaviors for C++ operators when they are used with user-defined types. This feature enhances the language by making it more intuitive and readable, particularly for instances in which the natural operations of mathematical objects are applicable. With overloaded operators, you can manipulate your custom classes and structs just as you would with built-in types.
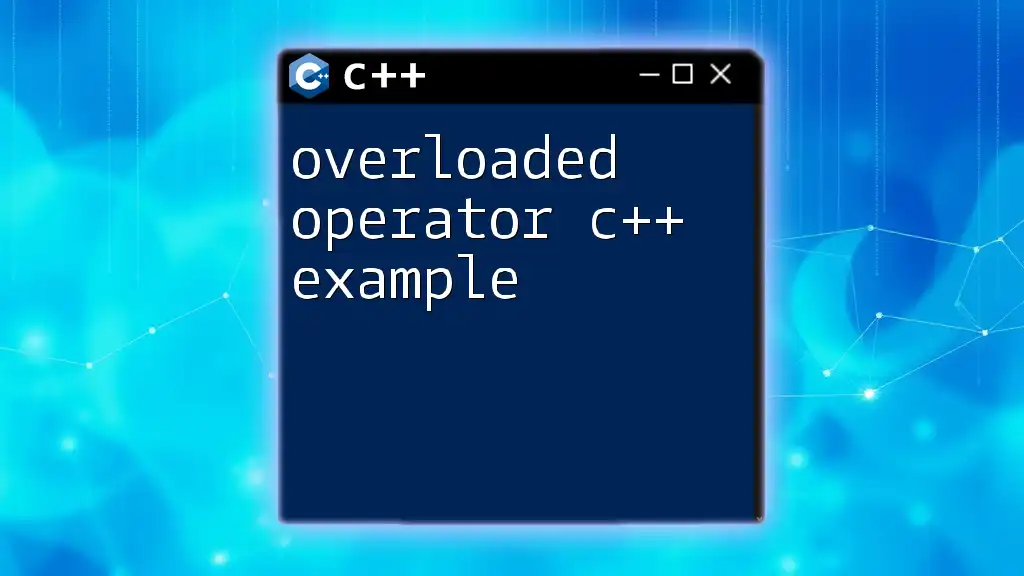
Importance of Operator Overloading in C++
Overloading operators bring several advantages to your code:
-
Enhancing Code Readability: By using operator overloading, you can define operations for custom types that resemble operations of fundamental types. This leads to clearer and more understandable code. For instance, how intuitive would it be to add two complex numbers using the `+` operator rather than a dedicated method?
-
Creating Intuitive Interfaces: Operators add familiarity for anyone using your classes. Instead of method calls, users can perform operations directly using syntax they are accustomed to, like `a + b` instead of `a.add(b)`.
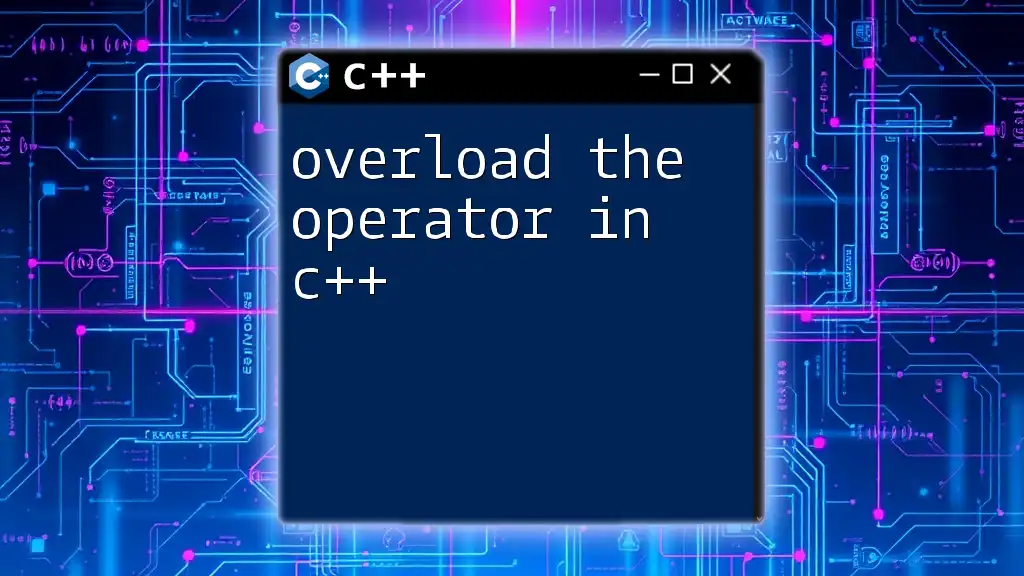
Understanding C++ Operators
Types of Operators
C++ permits the overloading of a variety of operators. Here’s a list of some common categories that you can work with:
- Arithmetic Operators: `+`, `-`, `*`, `/`, etc.
- Relational Operators: `==`, `!=`, `<`, `>`, etc.
- Increment/Decrement Operators: `++`, `--`
- Assignment Operators: `=`, `+=`, `-=`, etc.
- Stream Operators: `<<`, `>>`
Keep in mind that certain operators, such as `::`, `.`, `.*`, and those related to type conversion, cannot be overloaded.
How C++ Handles Operators
In C++, operators are defined to work with specific types. When you try to use an operator with types it doesn’t handle, the compiler will generate an error. For example, trying to multiply two `std::string` objects directly results in a compilation error since C++ doesn’t natively support that operation.
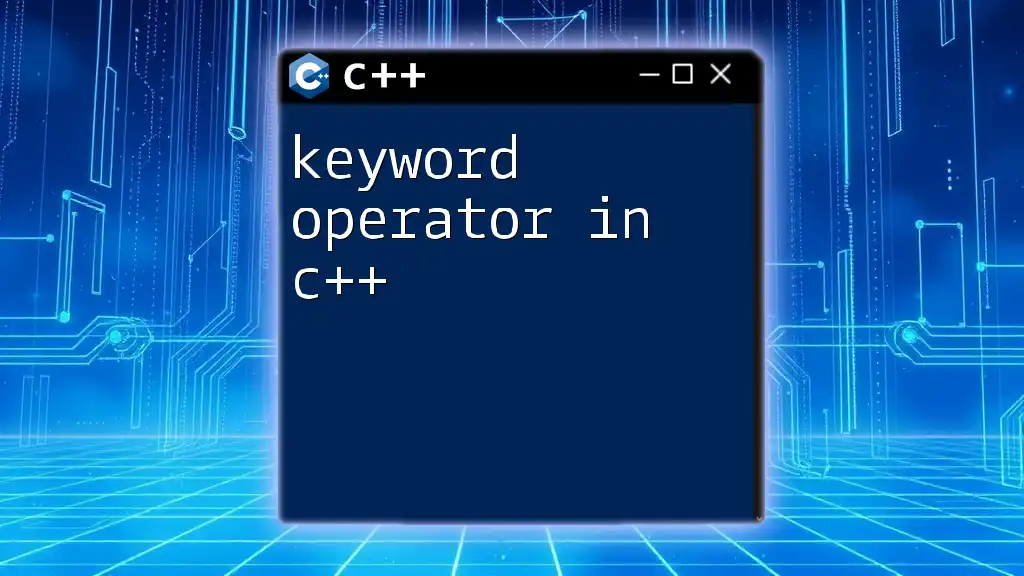
Basics of Overloading Operators
Syntax of Overloading Operators
The general syntax for overloading an operator in C++ involves defining a function with the keyword `operator` followed by the symbol you wish to overload. Here’s a simple example where we create a `Point` class and overload the `+` operator.
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
Point operator+(const Point& other) {
return Point(x + other.x, y + other.y);
}
};
In this example, when two `Point` objects are added together, the result will be a new `Point` that holds the sum of their `x` and `y` coordinates.
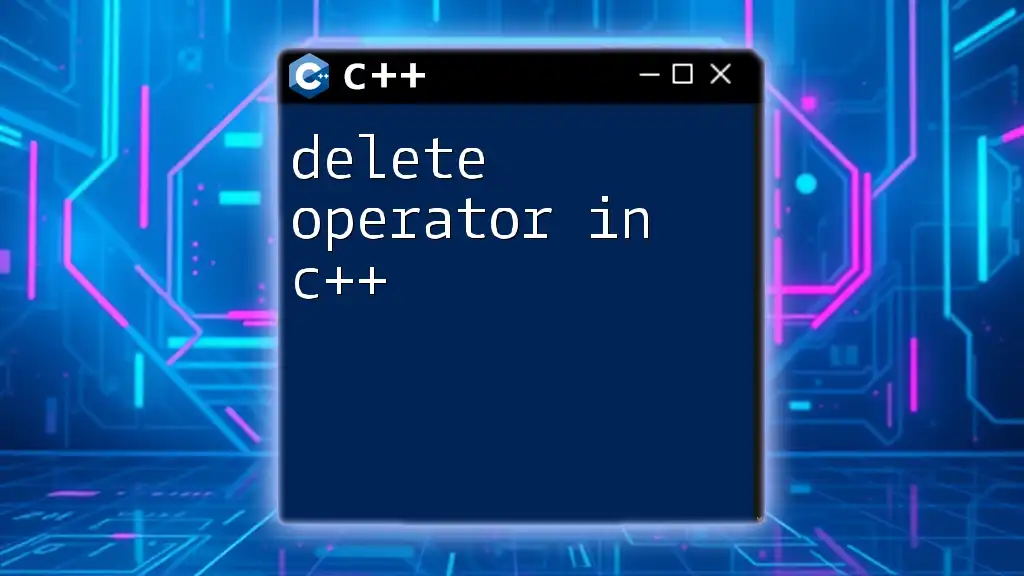
Detailed Look at Operator Overloading
Overloading Unary Operators
Unary operators operate on only one operand, such as `-`, `++`, and `--`.
Example: Overloading the Negation Operator
In the following example, we define a `Complex` class and overload the negation operator to return the negative of a complex number.
class Complex {
public:
double real, imag;
Complex(double r, double i) : real(r), imag(i) {}
Complex operator-() {
return Complex(-real, -imag);
}
};
If you create a `Complex` object and apply the negation operator, it will return the object with flipped real and imaginary parts.
Overloading Binary Operators
Binary operators require two operands. They can also be user-friendly when we define them for custom classes.
Example: Overloading the Addition Operator
Continuing with the `Complex` class, here’s how we overload the `+` operator:
Complex operator+(const Complex& other) {
return Complex(real + other.real, imag + other.imag);
}
When using this operator, you can seamlessly add two `Complex` objects together, and it will return a new `Complex` object with the resultant values.
Overloading Assignment Operator
When dealing with class objects, correctly managing assignment is crucial to avoid problems with shallow copies.
Importance of Copy vs Move Assignment
Copy assignment creates a new object as a copy of another. Move assignment takes ownership of the resources of another object, which is more efficient in terms of resource management.
Example: Overloading Assignment Operator
Complex& operator=(const Complex& other) {
if (this != &other) {
real = other.real;
imag = other.imag;
}
return *this;
}
This code safely manages self-assignment and assigns values to the `real` and `imag` members.
Handling Stream Operators
Operators for input and output are particularly useful when managing user interaction.
Overloading the Insertion Operator
#include <iostream>
std::ostream& operator<<(std::ostream& os, const Complex& c) {
os << c.real << " + " << c.imag << "i";
return os;
}
This allows easy printing of `Complex` objects. For example, you can use `std::cout << c;` when `c` is a `Complex` object.
Overloading the Extraction Operator
std::istream& operator>>(std::istream& is, Complex& c) {
is >> c.real >> c.imag;
return is;
}
With this operator, users can enter complex numbers directly into objects using standard input.
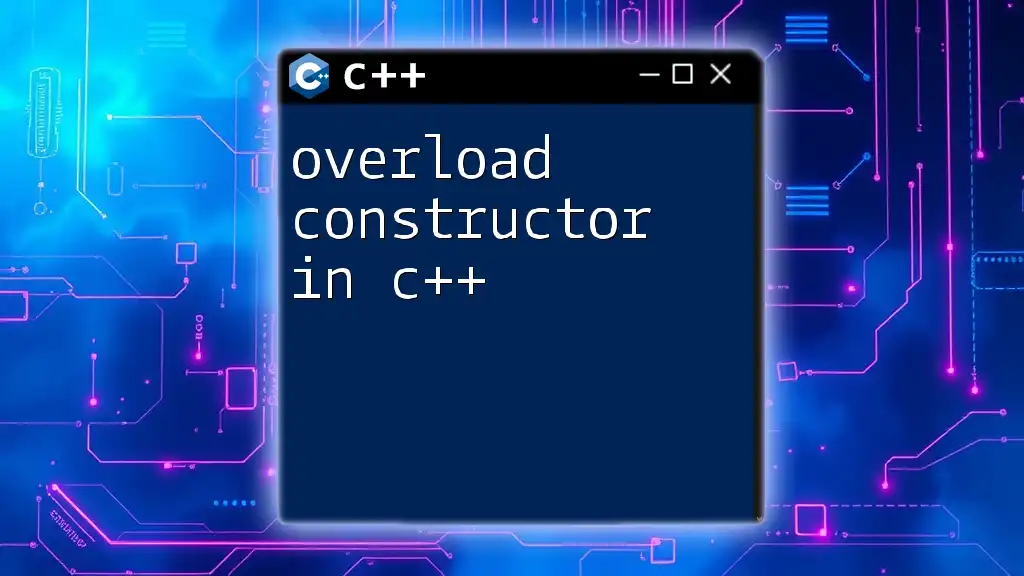
Best Practices for Operator Overloading
Maintain Intuitive Behavior
When overloading operators, you should ensure that they maintain intuitive behavior. A user should always expect the overloaded operator to behave similarly to how it functions with built-in types.
Respecting Operation Conventions
Following conventional behaviors of operators prevents confusion. For example, the `+` operator should always imply addition; avoid using it for operations like subtraction, as this leads to unexpected results.
Avoiding Overloading for Complex Operations
Sometimes simplicity is better. Avoid using operator overloading for highly complex operations that could confuse users. Maintain clarity and ease of use.
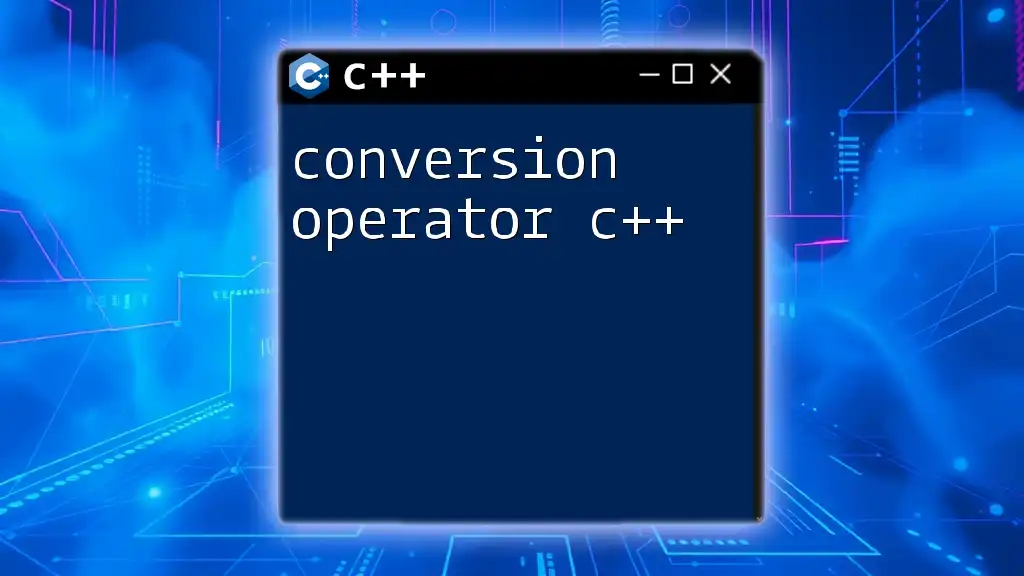
Common Mistakes and How to Avoid Them
Forgetting to Handle Self-Assignment
It’s a common mistake to neglect checking for self-assignment in the assignment operator, which can lead to adverse effects. Always check if the current object is the same as the object being assigned to it.
Incorrect Reference Management
Overloading operators incorrectly with respect to pointers and dynamic memory can lead to memory leaks or crashes. Always use smart pointers where appropriate to prevent memory management issues.
Failing to Return Correct Types
Returning incompatible types or failing to return `*this` for assignment operators will lead to compilation errors or runtime issues. Ensure you understand the return type expectations.
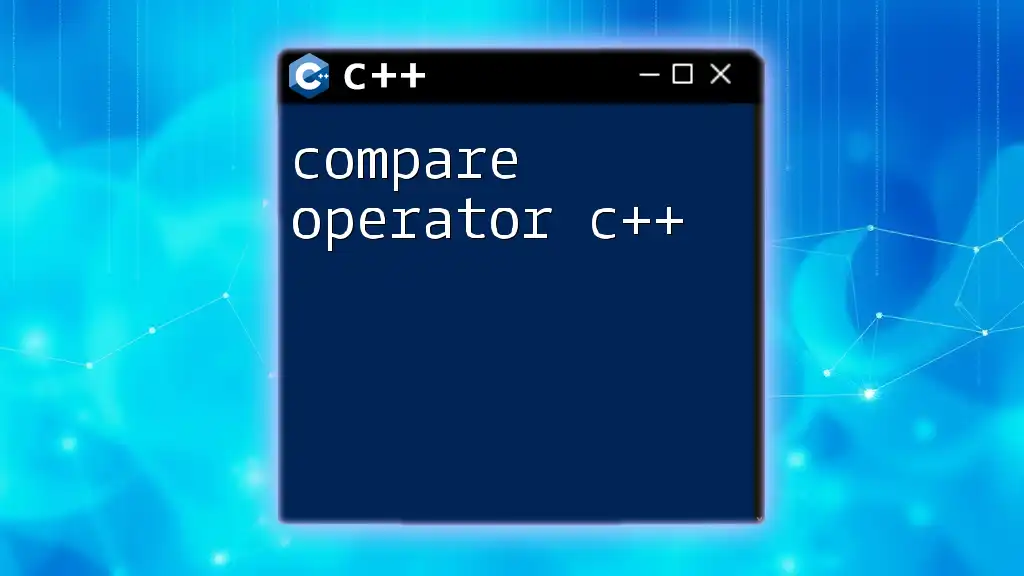
Conclusion
Overloaded operators in C++ play a crucial role in bringing readability and intuitiveness to the manipulation of user-defined types. By leveraging operator overloading wisely, you can create classes that are not only powerful but also easy to use. Practice and experiment with the examples provided to deepen your understanding of this vital C++ feature.
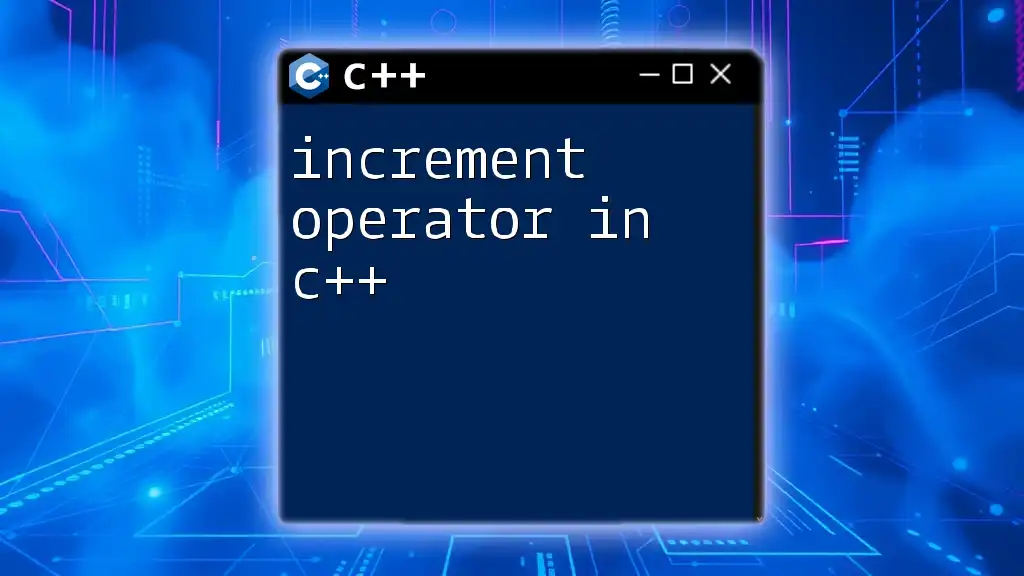
Further Reading
For further exploration of overloaded operators in C++, consider diving into advanced topics such as move semantics, templates, and operator overloading with smart pointers. Numerous resources—books, online tutorials, and communities—are available to support your continued learning journey in C++.