In C++, operator overloading allows developers to redefine the way operators function for user-defined types, enabling intuitive operations on objects of those types.
Here’s a simple example of overloaded addition (`+`) operator for a `Point` class:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
// Overloading the + operator
Point operator+(const Point& p) {
return Point(x + p.x, y + p.y);
}
};
int main() {
Point p1(1, 2);
Point p2(3, 4);
Point p3 = p1 + p2; // Using the overloaded operator
std::cout << "Point p3: (" << p3.x << ", " << p3.y << ")" << std::endl; // Output: Point p3: (4, 6)
return 0;
}
Understanding Operator Overloading
Operator overloading is a powerful feature in C++ that allows developers to define custom behaviors for operators when they are used with user-defined types (like classes). This enhances the readability and usability of the code by allowing intuitive expressions like `a + b` when `a` and `b` are objects of a user-defined class.
Benefits of Operator Overloading
The benefits of operator overloading are considerable, including:
- Enhanced Code Readability: Overloading makes the code more intuitive. Instead of calling a method like `add(v1, v2)`, one can simply write `v1 + v2`.
- Simplified Syntax: Users can work with complex objects using simple operators, reducing the learning curve and making the code easier to follow.
- Improved Usability: Operator overloading allows user-defined types to function as built-in types, enabling seamless integration into existing C++ code.

How to Overload Operator in C++
Basic Syntax of Operator Overloading
Overloading an operator in C++ generally adheres to specific syntax. The operator is defined as a function, either a member function of a class or a friend function.
Here is the basic syntax for overloading an operator:
- The function must be declared with the keyword `operator` followed by the operator symbol.
- The function can be declared either as a member function or a friend function.
For example:
ReturnType operatorOperatorSymbol(ParameterType1 parameter1, ParameterType2 parameter2) {
// Function Definition
}
Overloading Operators: The Fundamentals
Operators can be classified into unary (operating on one operand) and binary (operating on two operands). Understanding the distinctions is crucial when implementing overloaded operators.
- Member Function vs. Friend Function:
- Member Function: The first operand is the object itself. It is generally used for unary operators.
- Friend Function: Does not belong to any class but can access private members. Often used for binary operators.
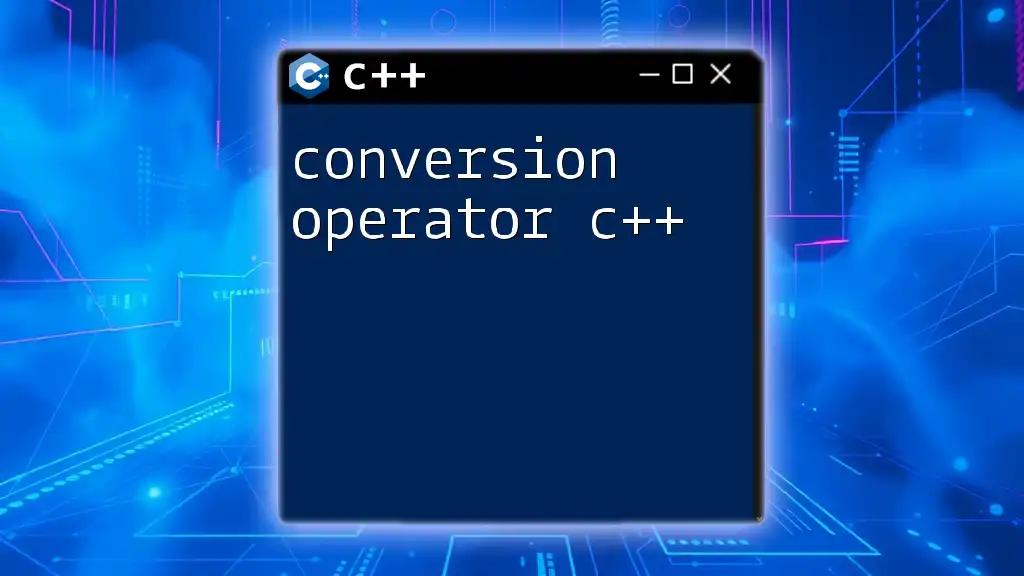
Examples of Overloading Operators
Overloading the Addition Operator
Using Member Function
Let's look at an example of overloading the addition operator (`+`) using a member function. This example creates a simple class `Vector` to represent points in a 2D space and adds two vectors together:
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
Vector operator+(const Vector& v) {
return Vector(x + v.x, y + v.y);
}
};
In this code, the `operator+` function takes another `Vector` as a parameter, adds the corresponding components, and returns a new `Vector` object representing the sum.
Using Friend Function
Alternatively, the addition operator can be overloaded using a friend function, which is beneficial if we need to work with two objects that are not explicitly tied to a single class's member context:
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
friend Vector operator+(const Vector& v1, const Vector& v2) {
return Vector(v1.x + v2.x, v1.y + v2.y);
}
};
In this case, `operator+` is a non-member function, allowing for more flexibility and clarity in certain situations.
Overloading the Stream Insertion Operator
The stream insertion operator (`<<`) allows us to output our custom objects easily. This example shows how to overload it for a `Point` class:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
friend std::ostream& operator<<(std::ostream& os, const Point& p) {
os << "(" << p.x << ", " << p.y << ")";
return os;
}
};
By overloading the `<<` operator, we can now simply use `std::cout << point;` to output the `Point` object conveniently.
Overloading the Subtraction Operator
The subtraction operator (`-`) can also be overloaded in a similar fashion to addition. Here's how you would do it for the `Vector` class:
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
Vector operator-(const Vector& v) {
return Vector(x - v.x, y - v.y);
}
};
This implementation allows us to use the subtraction operator intuitively to subtract one vector from another.
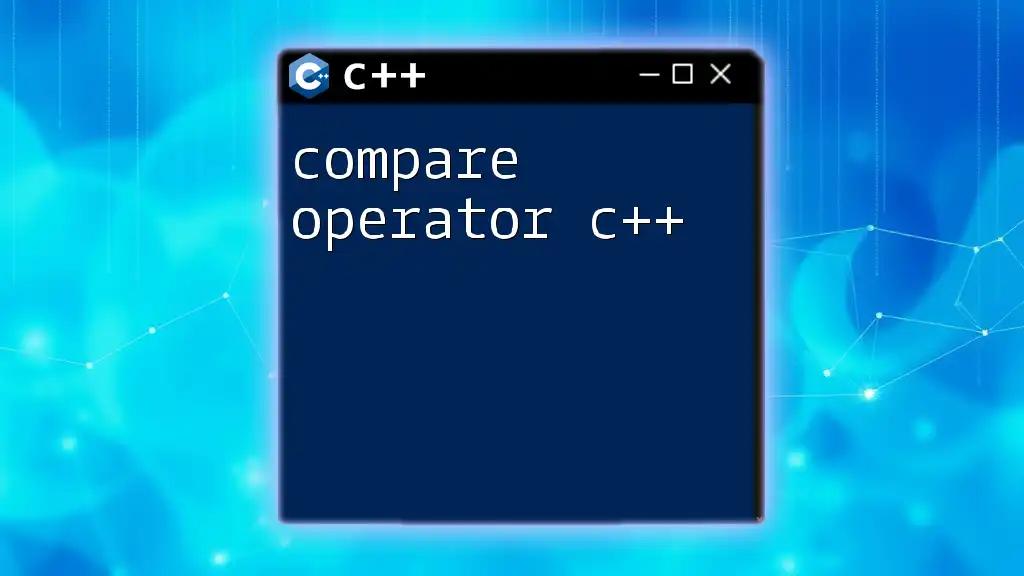
Best Practices for Operator Overloading
When to Overload Operators
While operator overloading can yield significant benefits, developers must exercise discretion. Generally, it is wise to overload operators when they:
- Serve an intuitive purpose with user-defined types.
- Align logically with their built-in counterparts, providing clear functionality.
Achieving Consistency in Overloaded Operators
Consistency is crucial for a user-friendly interface. The overloaded operators should align with the expected behavior in C++, so it feels natural to use them. For instance, if `a + b` is overloaded to perform vector addition, then `a - b` should act according to the expectations of mathematical vectors. This ensures that users can intuitively understand the operations being performed.
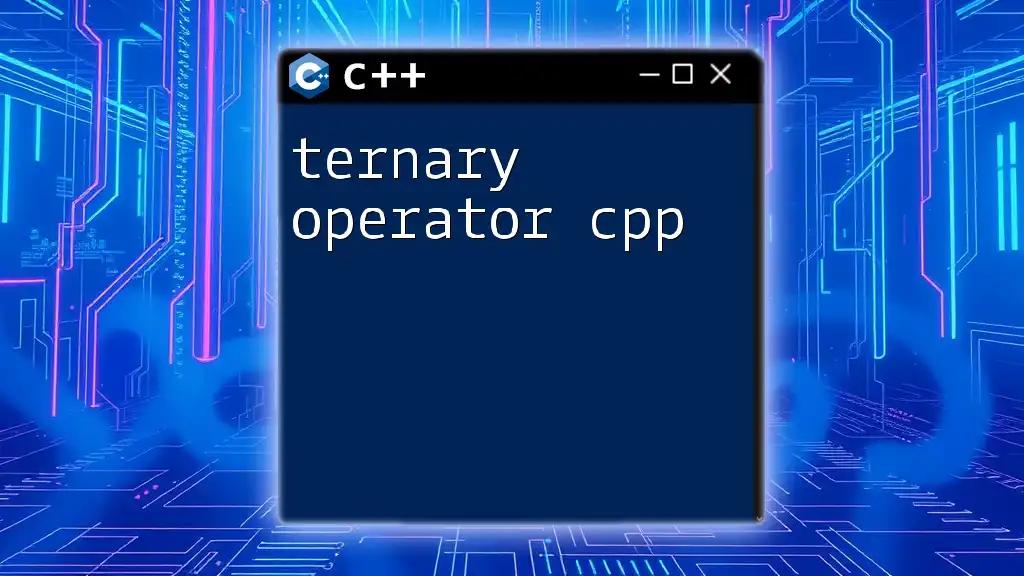
Conclusion
To recap, operator overloading in C++ is a powerful and indispensable feature that increases code readability and usability. By adopting this technique, C++ programmers can create flexible and understandable interfaces around their custom data types.
Next Steps for Further Learning
For those looking to deepen their understanding of operator overloading, consider exploring additional resources such as books, online tutorials, and forums dedicated to C++. Practicing by creating more overloaded operators in various contexts will also provide invaluable experience.
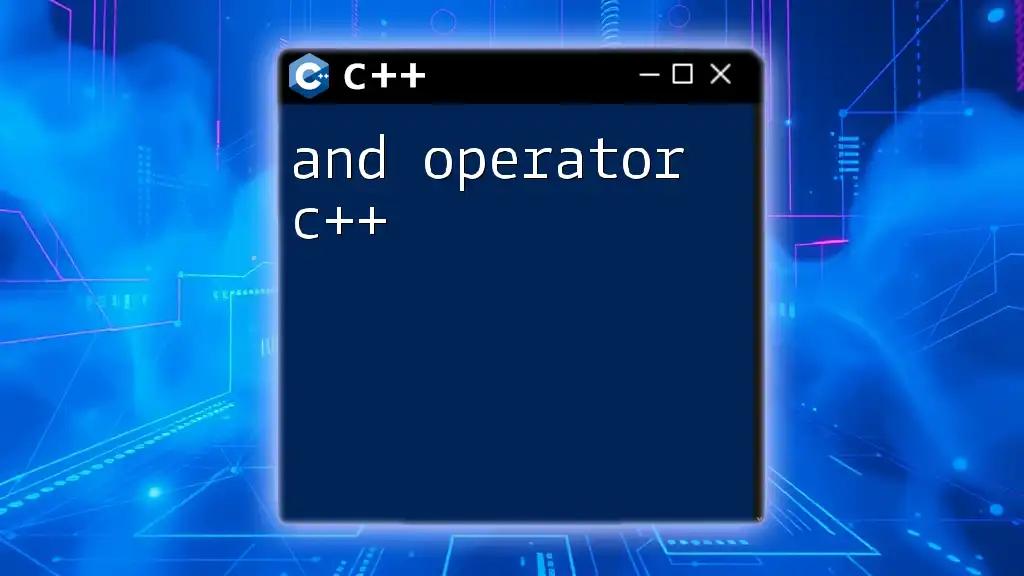
FAQs about Operator Overloading
Common Questions
- What operators can be overloaded? Most operators can be overloaded, except for certain operators like `::`, `.*`, and `?.`.
- Can constructors be overloaded? While you can overload constructors, operator overloading refers to changing the behavior of existing operators. It’s vital to distinguish these two concepts.
- What limitations exist with operator overloading? Operator overloading can lead to complexity or confusion if used improperly. Therefore, maintain clear documentation and use overloads sparingly to enhance usability rather than hinder it.