The ternary operator in C++ is a concise way to perform conditional statements that returns one of two values based on a boolean expression, using the syntax `condition ? value_if_true : value_if_false`.
Here’s a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 10, b = 20;
int max = (a > b) ? a : b; // Returns the greater of a and b
std::cout << "The maximum value is: " << max << std::endl;
return 0;
}
Understanding the Syntax of C++ Ternary Operator
The ternary operator is a compact syntax for making simple decisions based on a condition. In C++, the syntax of the ternary operator is written as follows:
condition ? expression1 : expression2;
Components of the Syntax
- Condition: This is a boolean expression that evaluates to either `true` or `false`.
- Expression if true: This expression is executed if the condition is `true`.
- Expression if false: This expression is executed if the condition is `false`.
For example, consider the following code snippet:
int a = 10, b = 20;
int max = (a > b) ? a : b; // max will hold the value of 20
In this example, the condition `(a > b)` evaluates to `false`, so the value of `b` is assigned to `max`.
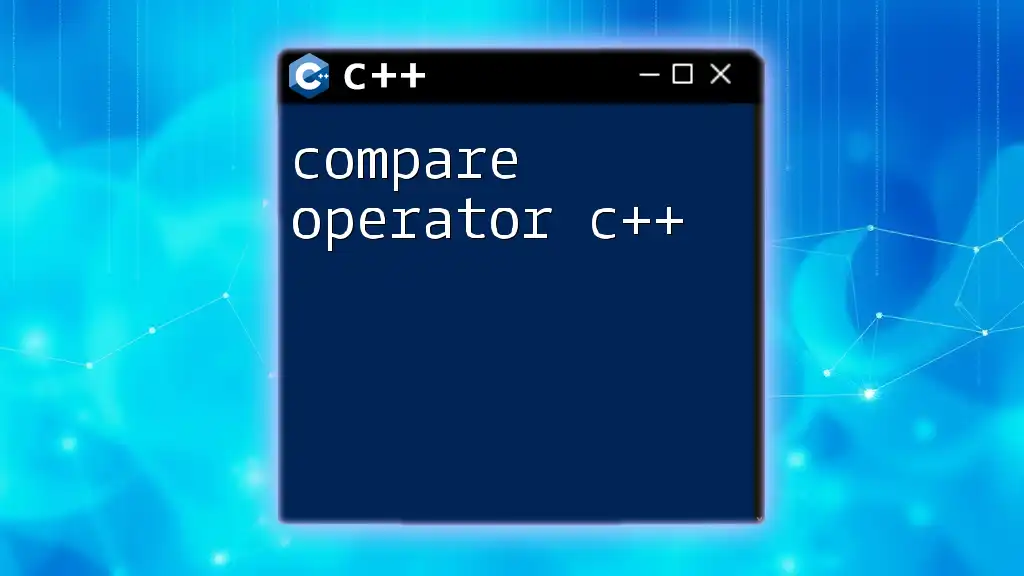
How Ternary Operator Works in C++
Understanding how the ternary operator works can help you write more concise and efficient C++ code. The evaluation process is straightforward: C++ evaluates the condition first. If the condition is true, the first expression is executed; if false, the second expression is executed.
Example Code Snippet
Here’s another example to illustrate its functionality clearly:
int x = 5;
int result = (x > 10) ? x + 10 : x - 10; // result will be -5
In this scenario, since `x` is not greater than 10, the expression `x - 10` is executed, and the value of `result` is `-5`.
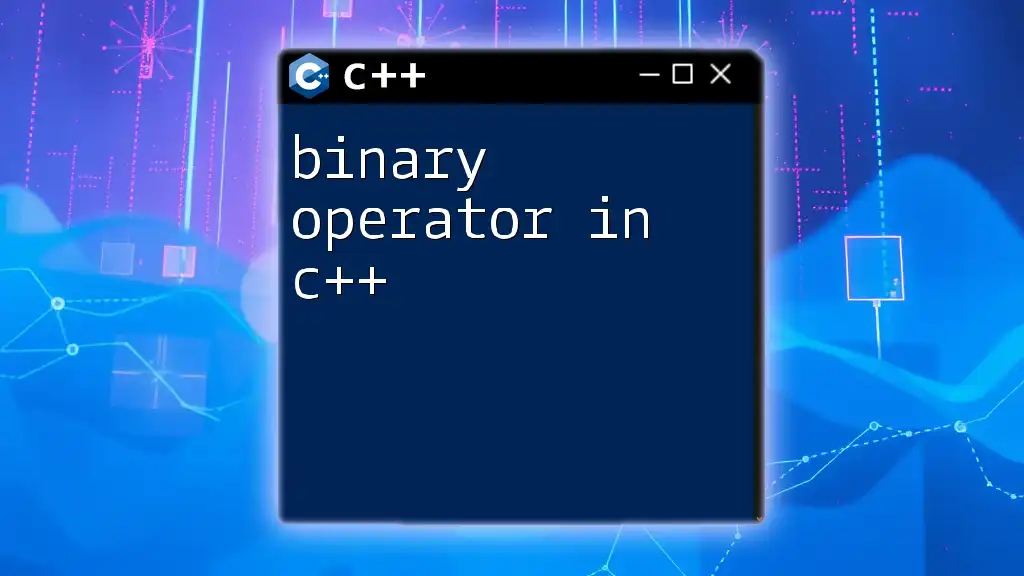
Use Cases for Ternary Operator in C++
Using the ternary operator effectively can simplify your code and make it more readable. Here are some common use cases:
-
Inline Assignment: You can use the ternary operator to assign values based on a condition in a single line.
int temperature = 30; string weather = (temperature > 25) ? "Warm" : "Cool";
-
Returning Values from Functions: A function can use a ternary operator to return values conditionally.
bool isEven(int number) { return (number % 2 == 0) ? true : false; }
In this example, the function checks if a number is even. If it is, it returns `true`; otherwise, it returns `false`. This single line replaces a potential five-lined if-else statement.
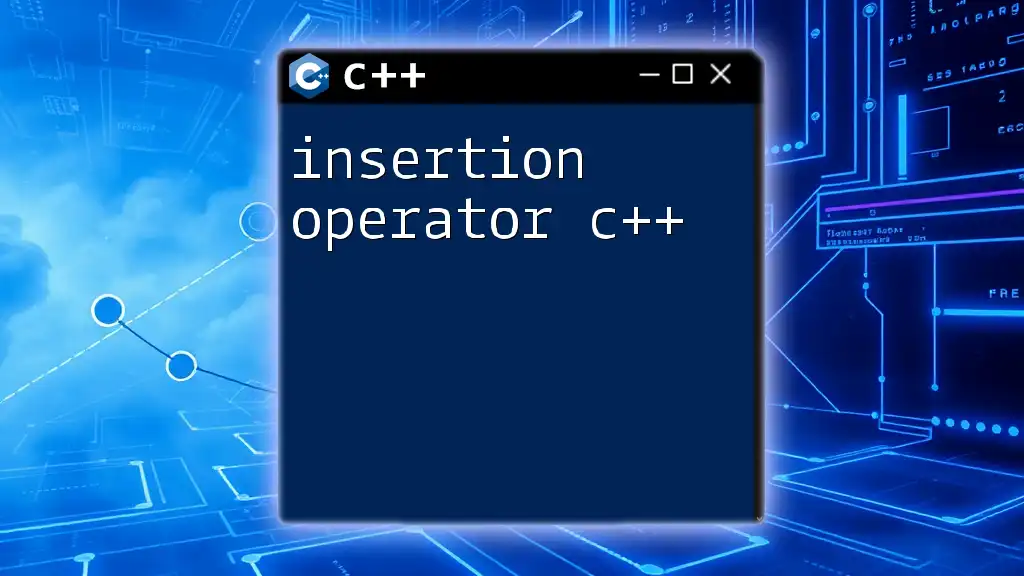
Advantages of Using the C++ Ternary Operator
The ternary operator offers several advantages, especially when used wisely:
-
Conciseness: It allows you to reduce the amount of code by replacing multi-line if-else statements with a shorter format.
-
Readability: When used properly, it can make your code cleaner and easier to understand, particularly in simple conditions.
-
Performance: The ternary operator can influence performance by reducing the number of lines of code the compiler needs to parse, though this is typically negligible for small expressions.
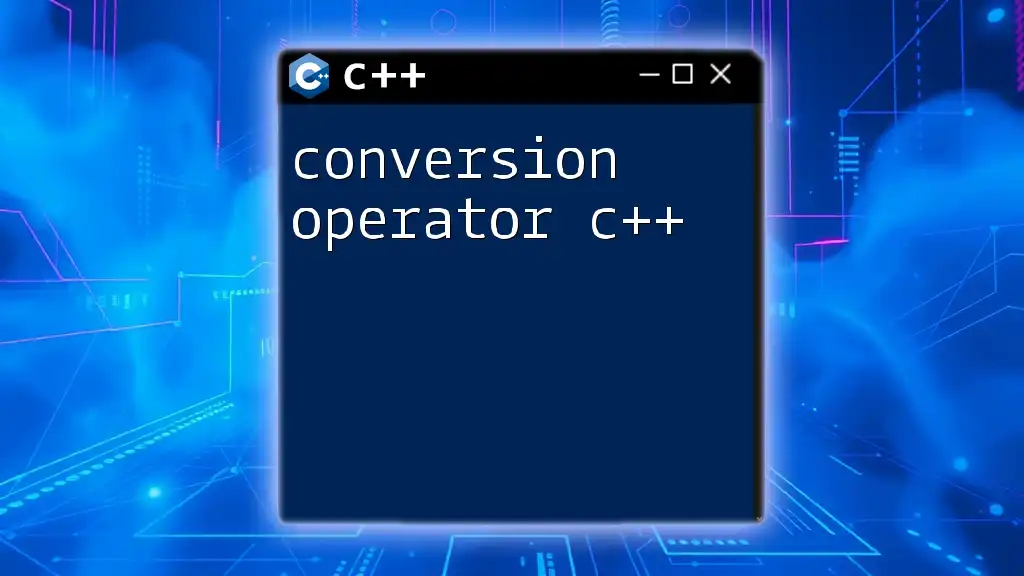
Common Mistakes with Ternary Operator in C++
Despite its advantages, many programmers make mistakes when using the ternary operator. Here are some of the common pitfalls:
-
Overcomplicating Expressions: One of the frequent issues is nesting ternary operators excessively, leading to unclear and hard-to-read code. For example:
int value = (a > b) ? (a < c ? a : c) : (b < c ? b : c);
This line quickly becomes overwhelming. It can often be clearer and simpler to use traditional if-else statements when the logic becomes complex.
-
Advice on Readability: If your expression requires more than a couple of operations, consider using an if-else statement for clarity.
-
Debugging Challenges: Debugging a ternary operator can be tricky, especially if the logic isn't straightforward. Always ensure readability trumps brevity when complexity increases.
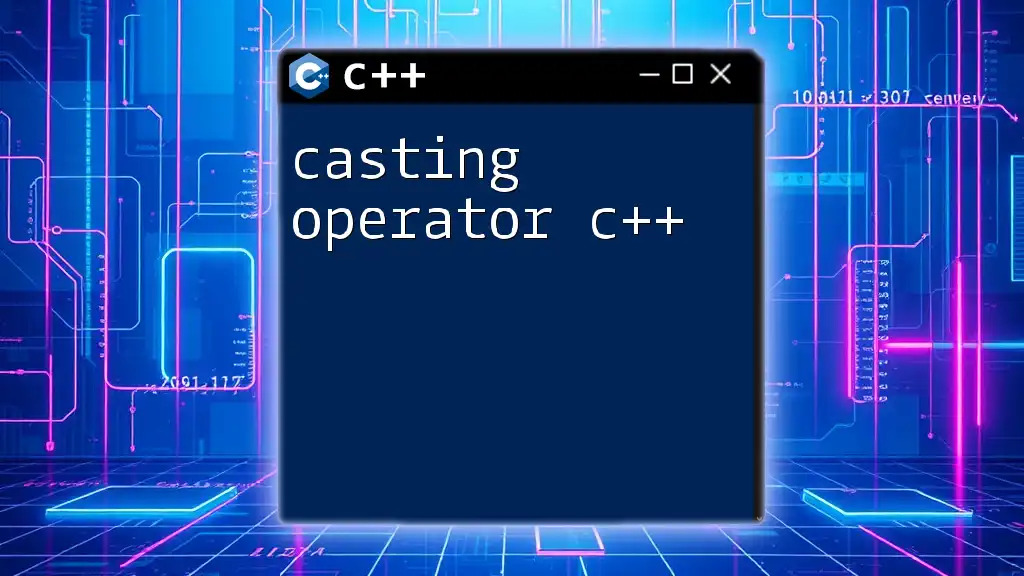
Nesting Ternary Operators
While nesting ternary operators can be done, caution should be exercised. Nesting allows you to evaluate multiple conditions, but at the cost of readability. The syntax for nesting is similar to that of a single ternary operator:
int a = 10, b = 20, c = 30;
int max = (a > b) ? (a > c ? a : c) : (b > c ? b : c);
Here, you are evaluating the maximum among three numbers. Although it works, the logical flow becomes less clear the more you nest. It is thus advisable to limit complexity or revert to traditional condition checking in such cases.
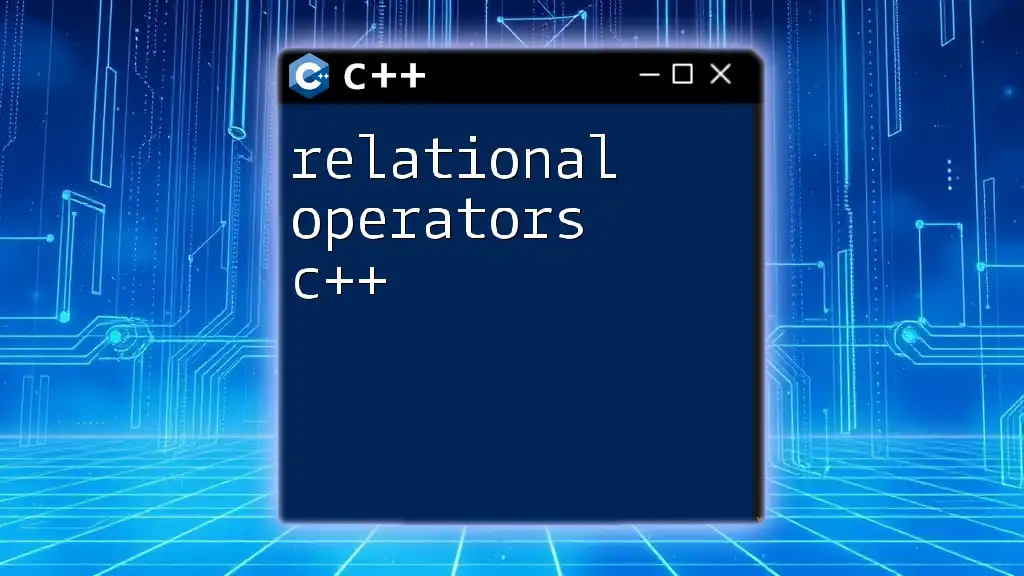
Best Practices for Using Ternary Operator in C++
To make the best use of the ternary operator in C++, consider the following best practices:
-
When to Use: Utilize the ternary operator when the condition is simple, allowing for a quick decision or value assignment without losing clarity.
-
When to Avoid: If multiple conditions or operations must be evaluated, or if it makes your code hard to read, opt for traditional if-else structures.
-
Code Readability Tips: Always prioritize readability. Use whitespace effectively, and avoid excessive nesting. If an expression takes more than a few seconds to digest, simplify the logic.
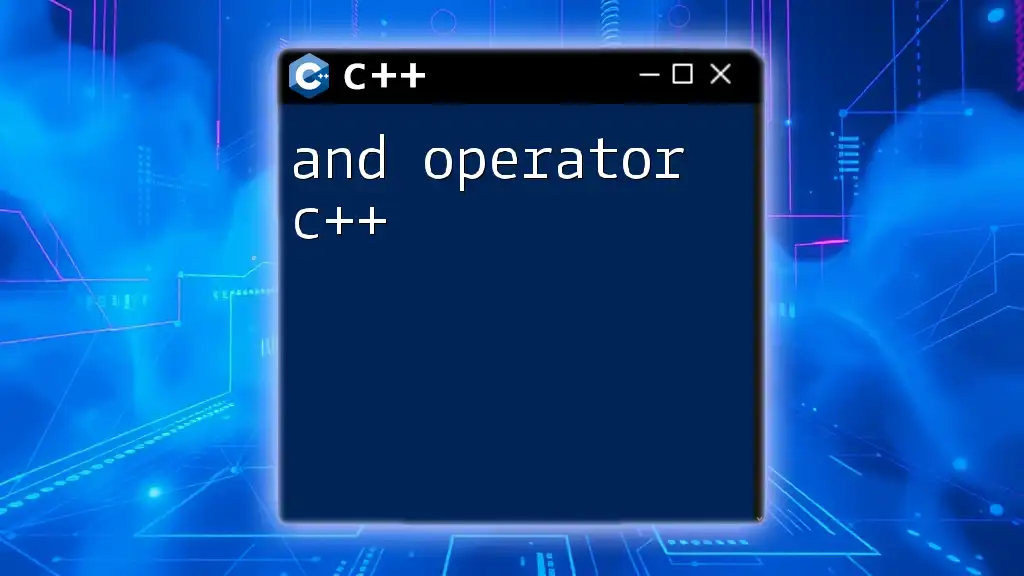
Conclusion
In summary, the ternary operator cpp offers a concise way to handle conditional expressions, but it must be used with an understanding of its implications. While it can simplify your code and enhance performance, maintain clarity and readability as a priority. Regular practice and thoughtful application of the ternary operator will enhance your coding efficiency in C++.
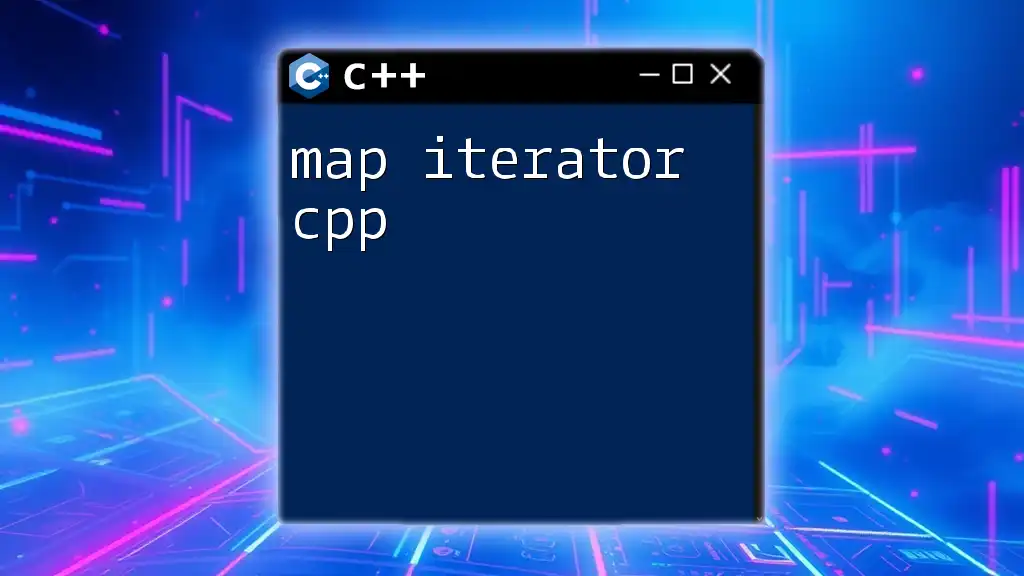
Additional Resource and Further Reading
As you continue to hone your C++ skills, consider exploring the various resources available. Books and articles focused on advanced C++ concepts may provide deeper insights into conditional operators and their application.
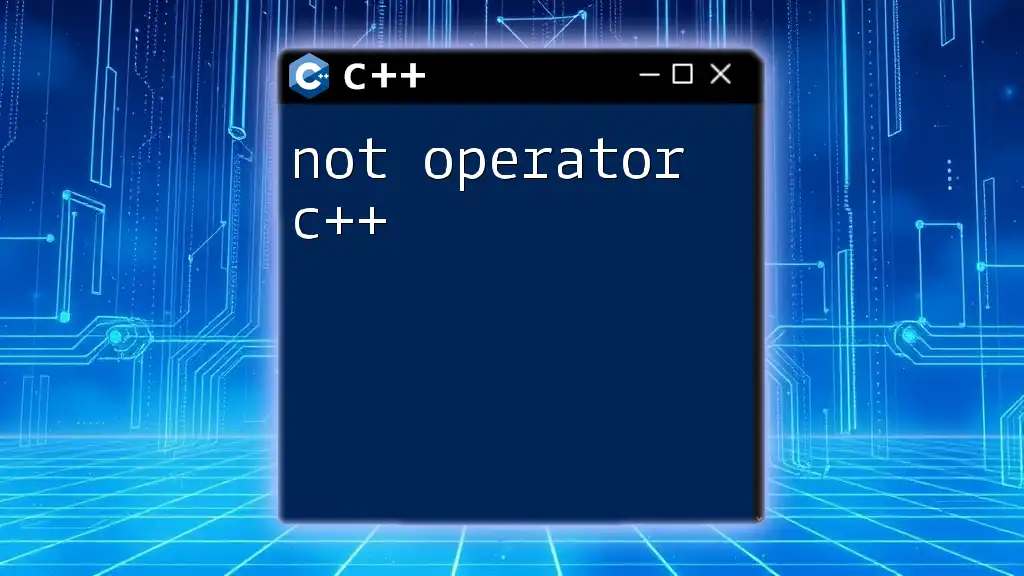
Code Playground
To reinforce your understanding, I encourage you to experiment with the provided examples in your IDE or use an online compiler. Try modifying conditions and expressions to see firsthand how they behave. This hands-on practice will help solidify your understanding of the ternary operator in C++.