A map iterator in C++ is used to traverse elements in a `std::map` container, allowing access to key-value pairs in a sorted order.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
Understanding C++ Map
What is a Map in C++?
A map in C++ is a part of the Standard Template Library (STL) that stores elements in key-value pairs. Each key in a map is unique, and it's associated with a specific value. Maps are typically implemented as balanced binary trees, making them efficient for searching, inserting, and deleting entries.
The primary advantages of using maps include:
- Automatic sorting: When you insert items into a map, they are stored in sorted order based on the keys.
- Fast lookups: Maps generally provide logarithmic time complexity for operations like inserting, accessing, and deleting elements.
Here's a basic example of how to declare and initialize a map:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> age;
age["Alice"] = 30;
age["Bob"] = 25;
return 0;
}
Features of C++ Maps
Maps in C++ have several compelling features:
- Unique Keys: Each key is unique; if a key already exists, inserting a new value will simply update the existing key's value.
- Automatic sorting: Maps manage the keys in a sorted order based on their values.
- Performance: Through underlying tree structures, maps enable operations like insertion and deletion at logarithmic time complexity.
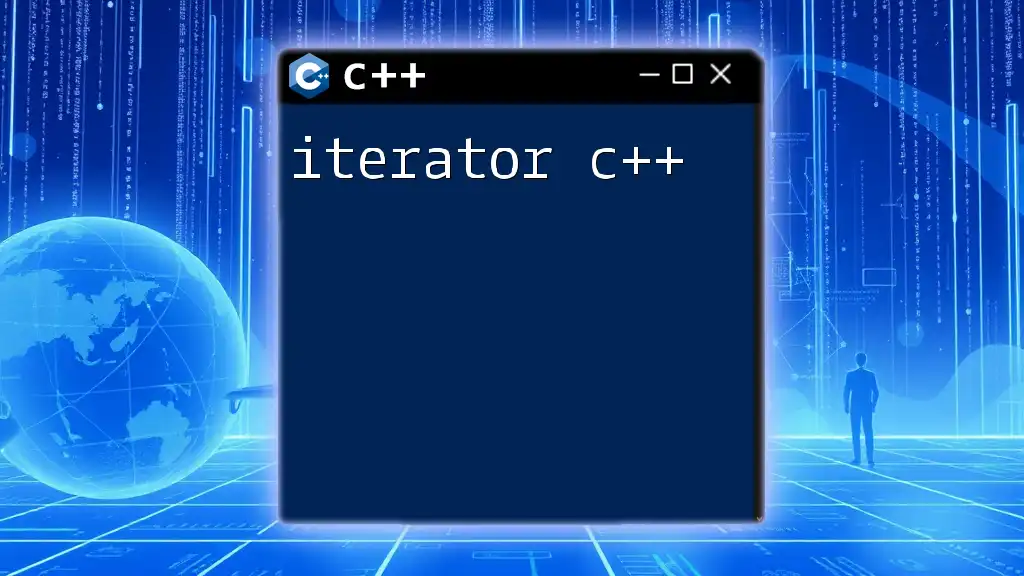
Introduction to Map Iterators
What are Iterators?
Iterators serve as a bridge between algorithms and containers. They act as pointers to elements within a container, allowing developers to traverse the data structures without needing to understand the internal representations of those structures.
Map Iterators in C++
Map iterators are specialized iterators designed to navigate through the elements of a `map` container. They allow you to efficiently access both keys and their associated values, providing the flexibility to read and, in some cases, modify the occurrences within the map.
Comparatively, map iterators are different from vector indices or list iterators in that they are inherently linked to the key-value pair nature of a map.
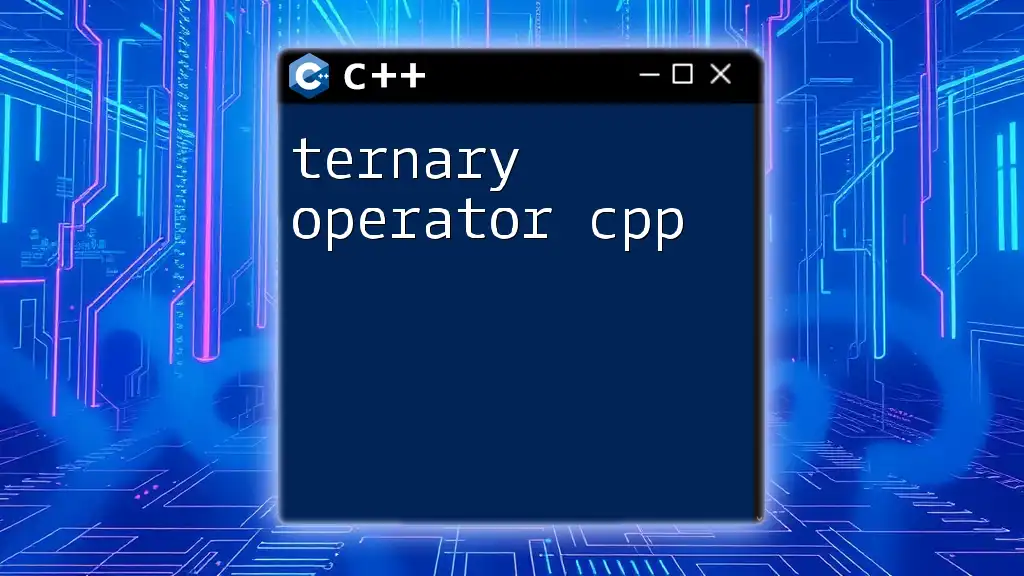
Types of Map Iterators
Const Iterators
Const iterators are used when you want to traverse a map while ensuring that the elements are not modified. They guarantee that the content of the map remains unchanged.
Here’s a simple function that proves the usefulness of const iterators:
#include <iostream>
#include <map>
void printMap(const std::map<std::string, int>& myMap) {
for (auto it = myMap.cbegin(); it != myMap.cend(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
}
Non-Const Iterators
In contrast, non-const iterators allow you to traverse and modify the elements in a map. Using a non-const iterator can also facilitate actions like incrementing values.
Here’s an example where we use a non-const iterator to increment the age stored in our map:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap{{"Alice", 30}, {"Bob", 25}};
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
it->second++; // Incrementing value
}
}
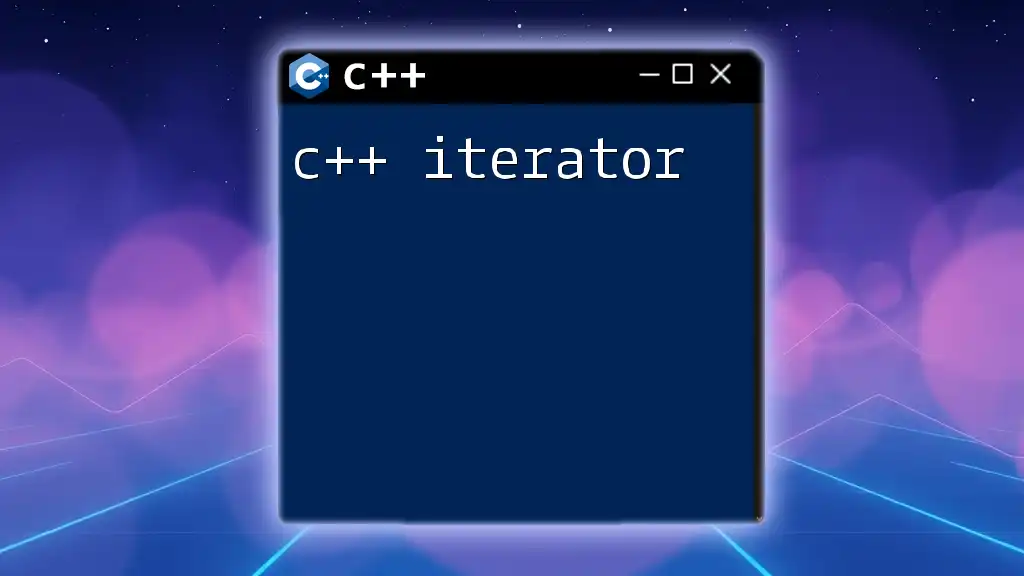
Using Map Iterators
Basic Operations with Map Iterators
Iterating Through a Map
An essential functionality of map iterators is to efficiently loop through the key-value pairs. Using an iterator enables you to access both keys and values without relying on indices, which you cannot use with maps.
Example of iterating over a map:
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
Inserting Elements
Map iterators can also aid in inserting new elements into a map. Elements can be added dynamically during iteration, though one should handle the insertion carefully to prevent invalidating the iterator.
Example of inserting elements:
myMap.insert(std::make_pair("Charlie", 20));
Erasing Elements
Another essential operation is using iterators to erase elements from a map. Using an iterator for deletion is safer and allows for seamless continuation of looping.
Example of erasing an element:
myMap.erase("Alice");
Advanced Operation: Reverse Iterators
Reverse iterators are particularly useful when traversing maps from the last element to the first, allowing you to access elements in reverse order.
You can utilize reverse iterators in the following way:
for (auto it = myMap.rbegin(); it != myMap.rend(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
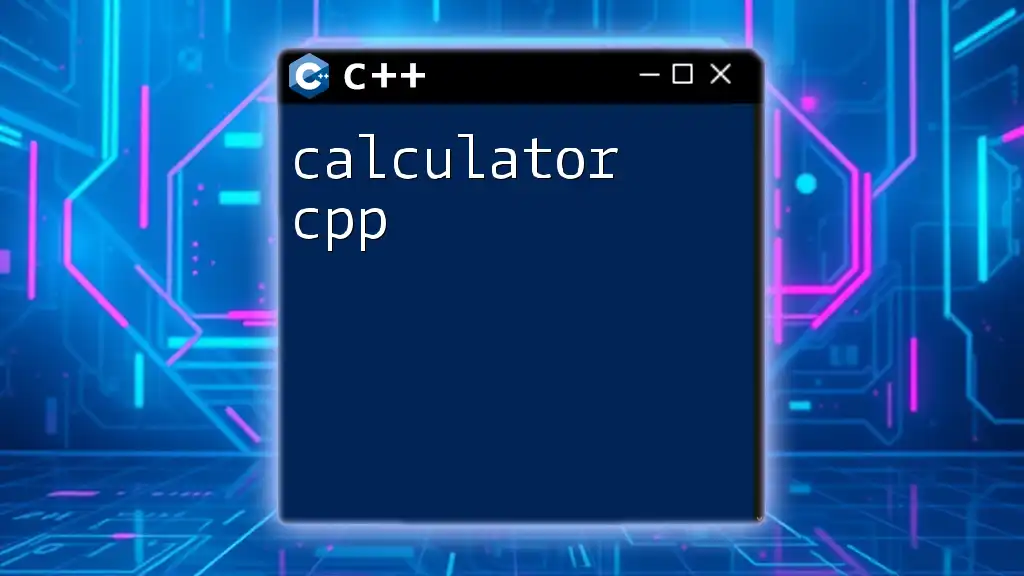
Practical Applications of Map Iterators
Use Cases in Real-Life Applications
Map iterators can be extremely beneficial in a variety of real-world applications, including implementing caches, counting frequencies of elements, or maintaining configurations where key-value relationships are crucial. Their automatic sorting can also enable easy retrieval of data based on ordered keys, enhancing searching capabilities.
Common Mistakes to Avoid
While using map iterators, avoid these common pitfalls:
- Out-of-bounds access: Be cautious when incrementing iterators; always ensure that they remain within valid bounds.
- Invalidating iterators: Inserting or erasing elements can invalidate iterators, so always ensure that your iterator remains valid post-operation.
These thoughtful approaches will help prevent errors that can lead to runtime crashes or unintended behaviors.
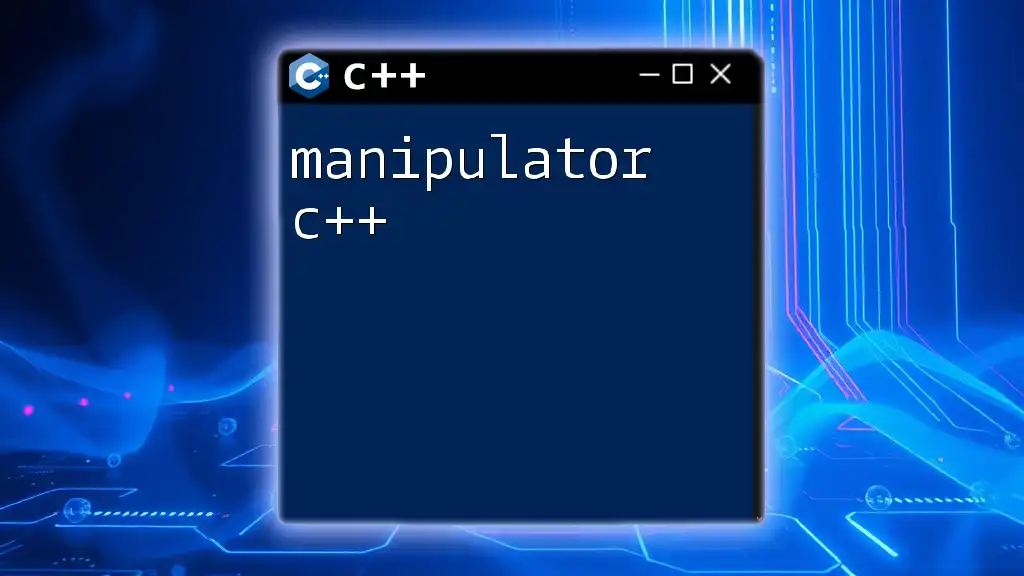
Conclusion
In conclusion, understanding and utilizing map iterators in C++ opens up powerful functionalities in working with key-value pairs efficiently. Whether you're navigating through a map, inserting elements, or erasing them, knowing how to manipulate and traverse through maps using iterators is an essential skill for any C++ developer. As you continue to grow your skills, remember that practice is vital in mastering the nuances of C++ maps and iterators together.
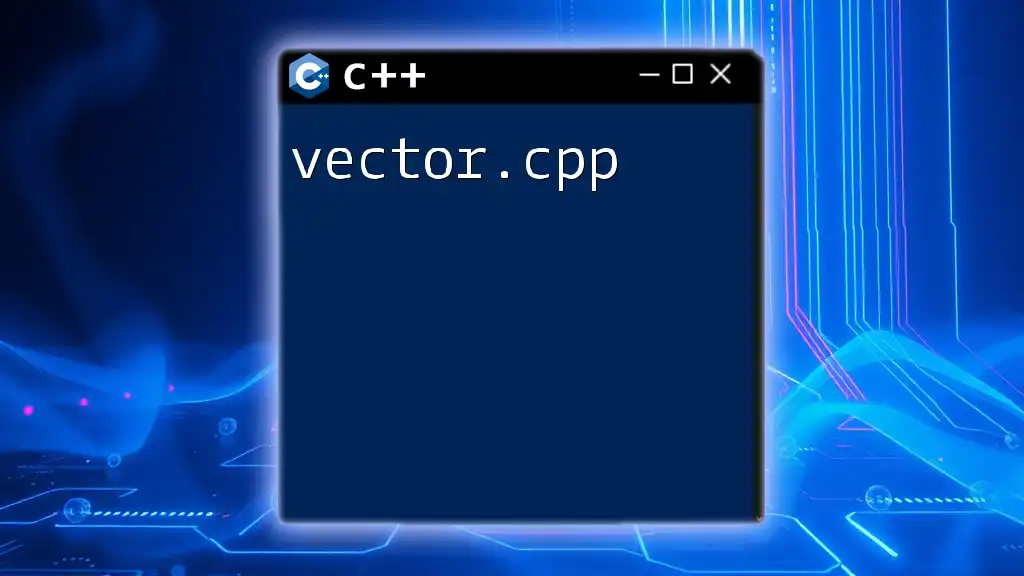
Additional Resources
To deepen your understanding of C++ maps and iterators, consider exploring further readings, helpful online communities, and tutorials that provide additional guidance and real-world applications of these concepts. Engaging with official C++ documentation can also enhance your knowledge and avoid common pitfalls as you move forward in your programming journey.