In C++, operator precedence determines the order in which operators are evaluated in expressions, influencing how calculations are performed.
Here’s an example demonstrating operator precedence:
#include <iostream>
int main() {
int a = 5, b = 3, c = 2;
int result = a + b * c; // Multiplication has higher precedence than addition
std::cout << "Result: " << result << std::endl; // Outputs: Result: 11
return 0;
}
Understanding Operators in C++
What Are Operators in C++?
In C++, operators are special symbols or keywords that tell the compiler to perform specific mathematical, relational, or logical manipulations on data. They operate on one or more operands, such as variables or constants, to produce a result. Understanding operators is crucial as they form the essence of programming logic.
Categories of C++ Operators
C++ categorizes operators based on their functionality. Here are the primary types of operators you'll encounter:
- Arithmetic Operators: For performing mathematical calculations (e.g., +, -, *, /).
- Relational Operators: For comparing values (e.g., ==, !=, >, <).
- Logical Operators: For logical operations (e.g., &&, ||, !).
- Bitwise Operators: For manipulating bits of data (e.g., &, |, ^).
- Assignment Operators: For assigning values (e.g., =, +=, -=).
- Increment/Decrement Operators: For changing value by a specific amount (e.g., ++, --).
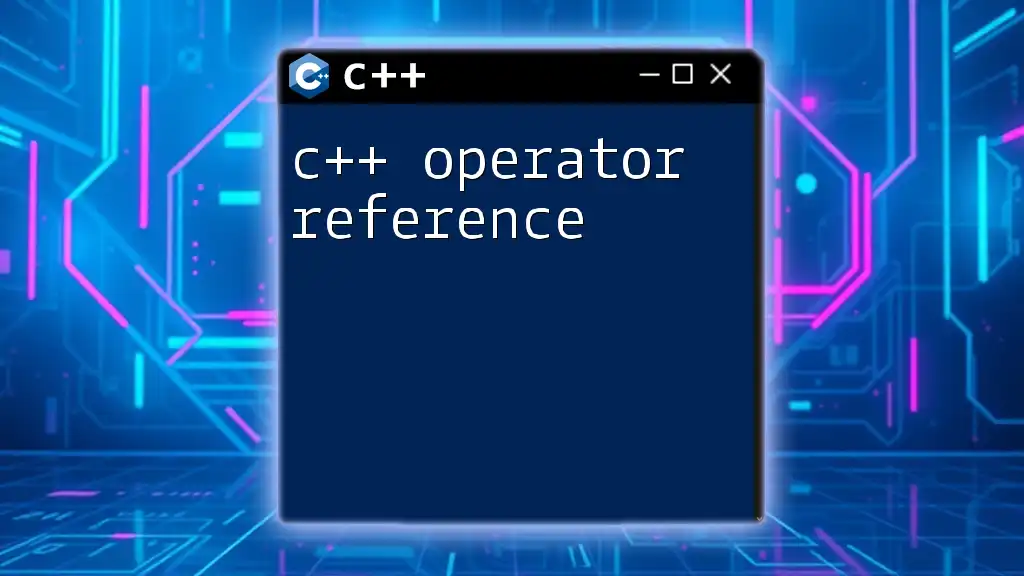
The Concept of Precedence in C++
What is Precedence?
Precedence refers to the rules that dictate the order in which operators are evaluated in expressions. In simpler terms, precedence determines which operation is performed first when multiple operators are involved. This is vital to understand because it directly influences the final outcome of complex expressions.
Why Is Operator Precedence Important?
Comprehending operator precedence is essential to avoid logical errors in your code. Misunderstanding the order of operations can lead to unintended results, making your programs behave unpredictably. By mastering the concept of precedence, you can enhance the reliability and accuracy of your code.
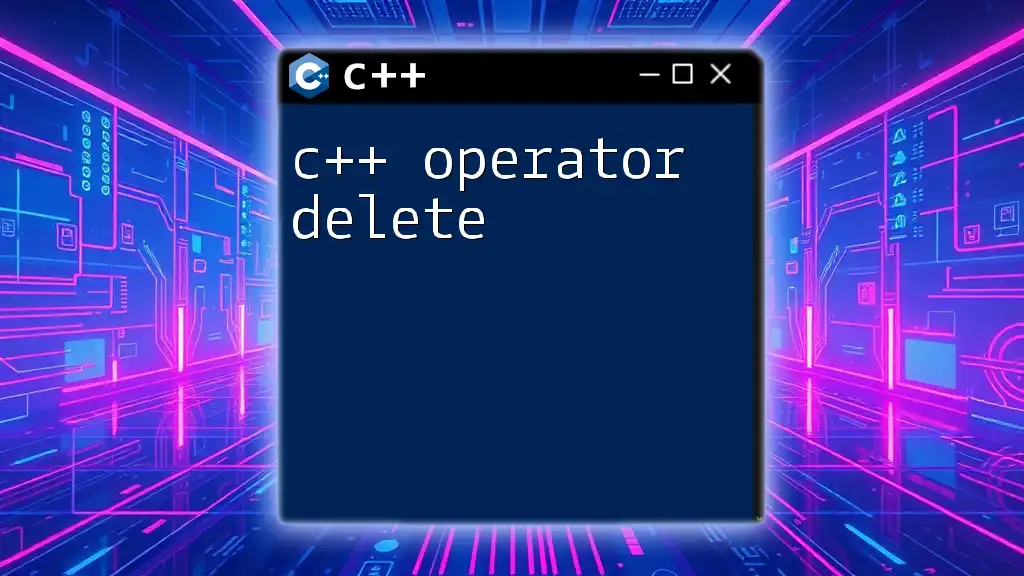
C++ Operator Precedence Rules
The Order of Operations in C++
In C++, certain operators take precedence over others. This order determines how an expression is evaluated. Operators with higher precedence are evaluated before those with lower precedence.
The C++ Precedence Table
Below is a summary of operator precedence in C++, organized from highest to lowest:
Operator | Description | Precedence Level |
---|---|---|
`::` | Scope resolution | Highest |
`()` | Function call operator | |
`[]` | Array subscript operator | |
`.` | Member access operator | |
`->` | Member access via pointer | |
`++` / `--` | Increment/Decrement (prefix) | |
`-` / `+` | Unary negation/positive | |
`*` | Dereference operator | |
`**` | Exponentiation (if used in libraries) | |
`*`, `/`, `%` | Multiplication, Division, Modulus | |
`+`, `-` | Addition and Subtraction | |
`<<`, `>>` | Bitwise shift left/right | |
`<`, `<=`, `>`, `>=` | Relational operations | |
`==`, `!=` | Equality and inequality comparisons | |
`&` | Bitwise AND | |
`^` | Bitwise XOR | |
` | ` | Bitwise OR |
`&&` | Logical AND | |
` | ` | |
`? :` | Ternary conditional operator | |
`=` | Simple assignment | Lowest |
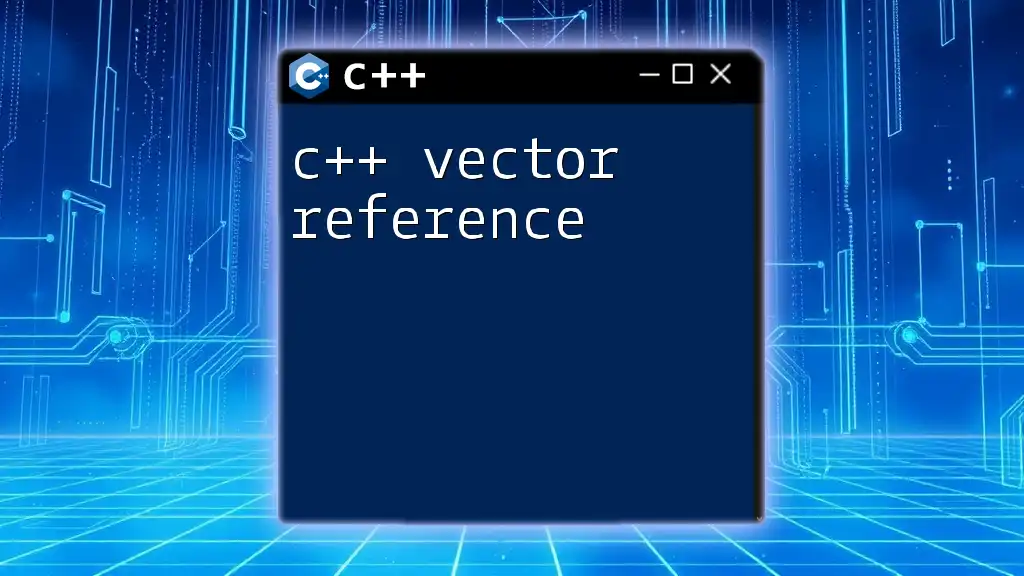
Detailed Breakdown of C++ Operator Precedence
Highest Precedence Operators
Function Calls and Member Access
The function call operator `()` and member access operators `.` and `->` have the highest precedence in C++. This means they will be evaluated before other operators in an expression. For example:
class Example {
public:
void show() { std::cout << "Hello, World!\n"; }
};
Example obj;
obj.show(); // Member access takes precedence over other operations
Mid-Level Precedence Operators
Arithmetic Operations
C++ performs arithmetic operations in a specific order. Multiplication and division are evaluated before addition and subtraction. This means that in an expression like `2 + 3 * 4`, the multiplication is performed first, resulting in:
int result = 2 + 3 * 4; // Evaluates to 14, since 3 * 4 equals 12, then 2 + 12
Lowest Precedence Operators
Assignment Operators
Assignment operators, such as `=`, have the lowest precedence. This means they are evaluated last in expressions. For example:
int a = 5;
int b = 3 + a; // Here, '3 + a' is evaluated first, then assigned to b
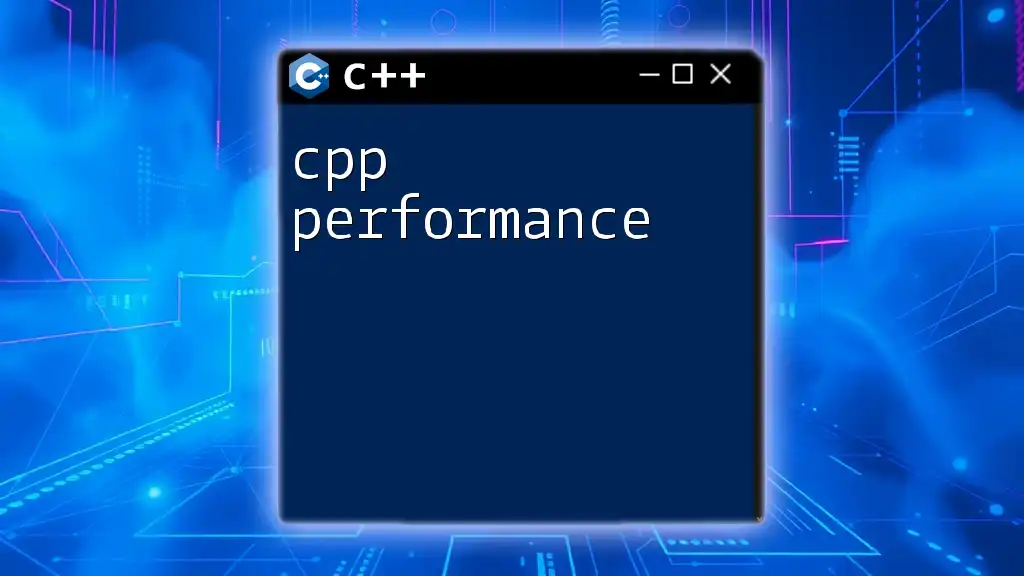
Operator Precedence in Complex Expressions
Nested Expressions
When working with nested expressions, the impact of operator precedence becomes even more critical. Consider the example:
int result = (2 + 3) * (4 - 1); // Correctly evaluates to 15
Here, the expressions within parentheses are computed first due to their higher precedence, leading to a clear understanding of how the final value is derived.
Parentheses and Their Impact on Precedence
Using parentheses can significantly affect the evaluation order of an expression. For instance:
int result = 2 + (3 * 4); // Changes the order to 2 + 12, resulting in 14
The presence of parentheses ensures that the multiplication occurs before the addition, which clarifies intent and ensures accuracy.
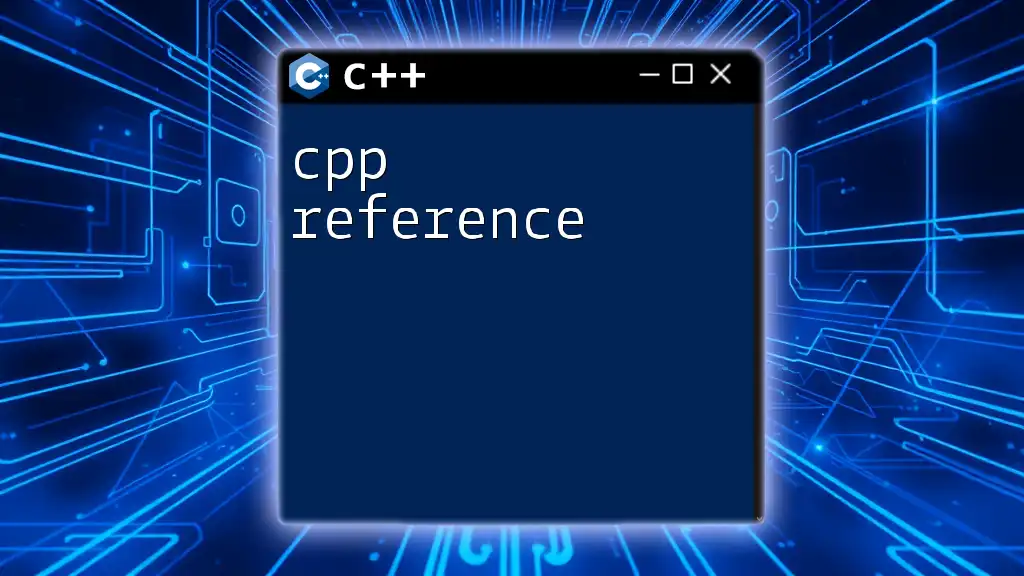
Common Mistakes with Operator Precedence
Programming errors often stem from misinterpreting operator precedence. It’s common to assume a certain order, leading to unexpected results. For example:
int value = 5 + 3 * 2; // This evaluates to 11, not 16 as some might expect
Being aware of precedence rules helps prevent such mistakes and enhances code reliability.
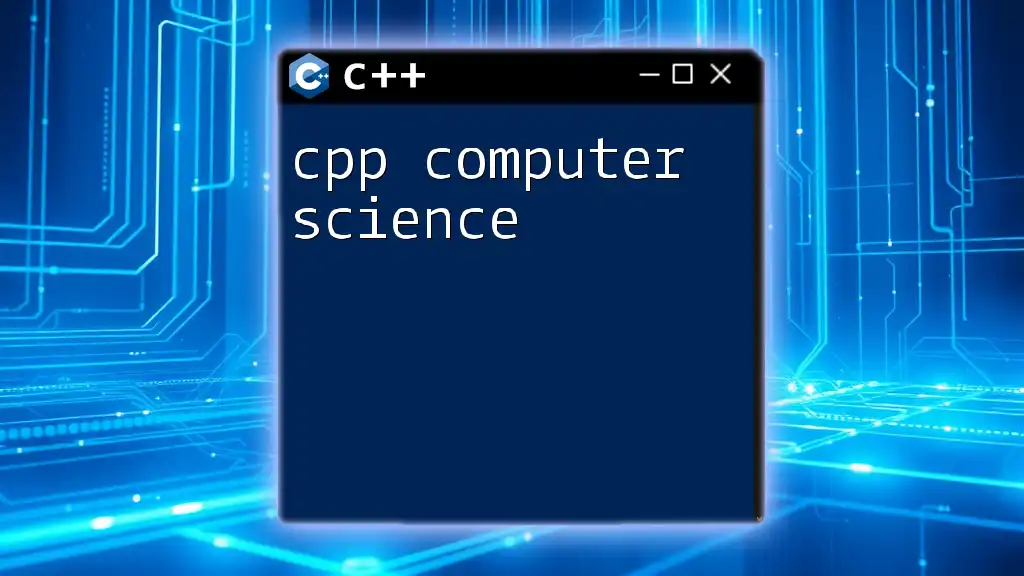
Best Practices for Managing Operator Precedence in C++
Use of Parentheses for Clarity
When in doubt about the order of operations, use parentheses to clarify expressions. Not only does this help the compiler understand your intent, but it also makes your code more readable for others. For instance:
int total = (a + b) * (c - d); // This expression is much clearer with parentheses
Maintaining Readability in Code
Keeping your code simple and readable should always be a priority. By understanding and properly applying operator precedence, you can write expressions that convey your logic clearly. Avoid overly complex expressions, and break down calculations into smaller, easily digestible parts if necessary.
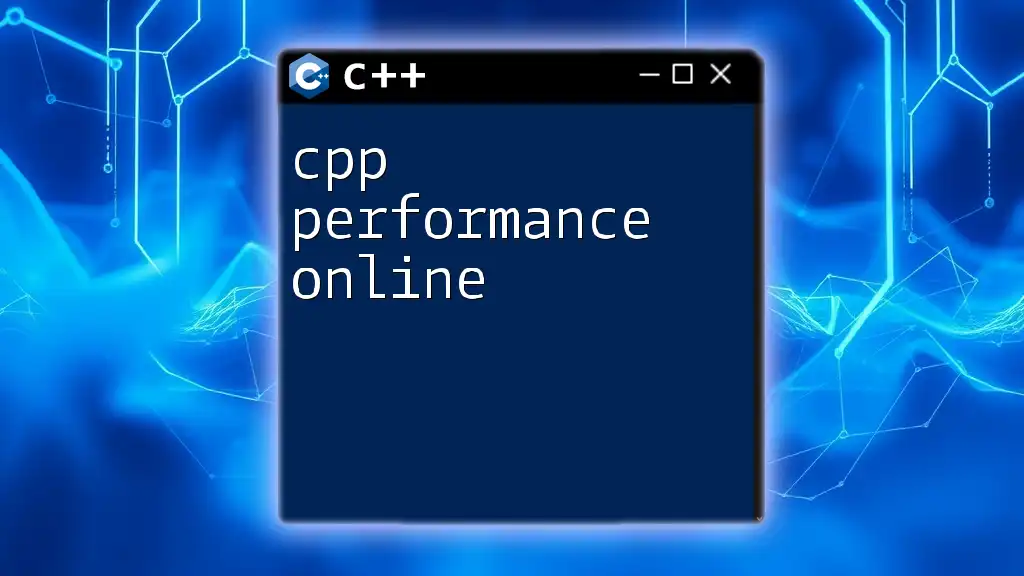
Conclusion
Understanding cpp operator precedence is vital for producing correct and efficient C++ programs. By mastering how different operators interact based on their precedence, you can write better code that performs as expected. Practicing with various expressions will solidify your understanding and help you avoid common pitfalls. Consider joining our program for more insights and tips on mastering C++ commands!
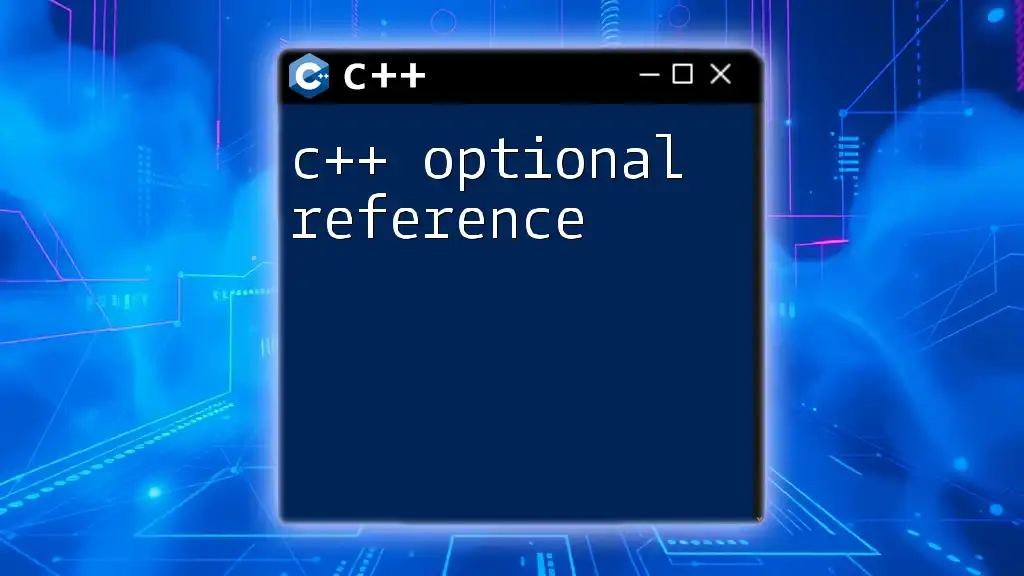
Additional Resources
For those eager to delve deeper into C++ operators and precedence, consider exploring documentation, tutorials, and coding exercises tailored to reinforce these concepts. Engaging with these resources will provide you with a stronger foundation in C++ programming.