C++ plays a pivotal role in aerospace engineering by enabling the development of high-performance software for simulations, control systems, and data analysis in aerospace applications.
Here's a simple code snippet demonstrating a basic C++ program that calculates the thrust needed for a rocket:
#include <iostream>
int main() {
double mass; // mass of the rocket in kg
double acceleration; // desired acceleration in m/s^2
std::cout << "Enter the mass of the rocket (kg): ";
std::cin >> mass;
std::cout << "Enter the desired acceleration (m/s^2): ";
std::cin >> acceleration;
double thrust = mass * acceleration; // F = ma
std::cout << "Required thrust (N): " << thrust << std::endl;
return 0;
}
Understanding C++ for Aerospace Engineering
C++ is integral to the aerospace engineering field, offering a blend of performance and flexibility. As a powerful programming language, C++ encapsulates features that are essential for creating complex aerospace systems.
Characteristics of C++
Performance is crucial in aerospace applications, where efficiency can mean the difference between successful missions and failures. The performance capabilities of C++ make it a preferred choice for applications requiring high computational speeds, such as flight simulations and real-time data processing.
Object-Oriented Programming (OOP) is another hallmark of C++. This paradigm is particularly suitable for aerospace engineering, where complex systems, such as drones or spacecraft, can be modeled using classes and objects. OOP enables engineers to create modular and reusable code, streamlining code maintenance and scalability.
Portability is vital in aerospace, where software must often be deployed across various platforms. C++ provides the versatility needed to develop applications that function seamlessly on different hardware, from embedded systems in aircraft to desktop applications for design and simulation.
Applications of C++ in Aerospace
C++ finds its footing in various aerospace applications, including flight simulation, embedded systems in aircraft, and software for spacecraft control. These applications leverage C++'s capacity to manage real-time data effectively and execute complex algorithms.

Key Concepts of C++ Relevant to Aerospace Engineering
Classes and Objects
Classes in C++ allow engineers to define complex data types, encapsulating both data and functions. This is especially useful in aerospace, where systems can be intricate.
For example, consider a basic class representing an aircraft:
class Aircraft {
private:
int altitude;
string name;
public:
void setAltitude(int a) { altitude = a; }
string getName() { return name; }
};
In this example, the Aircraft class defines properties and behaviors pertinent to any generic aircraft. This encapsulation allows for the organized management of attributes such as altitude and name.
Inheritance and Polymorphism
C++ supports inheritance, enabling the creation of class hierarchies. This means that a new class can inherit attributes and methods from an existing class, making it easier to extend functionality.
Consider the following example with a derived class:
class FighterJet : public Aircraft {
private:
int missileCapacity;
public:
void setMissileCapacity(int m) { missileCapacity = m; }
};
Here, the FighterJet class builds upon the Aircraft class, adding its unique properties like missile capacity. Polymorphism allows for methods to be used interchangeably, enabling dynamic behavior at runtime and enforcing a unified interface for varied objects.
Templates and Generic Programming
Templates in C++ help create functions and classes that operate with any data type, enhancing the versatility of aerospace software.
For instance:
template <typename T>
class Sensor {
private:
T data;
public:
void setData(T d) { data = d; }
};
In this case, the Sensor class can manage data of any type, be it integers for temperature sensors or floats for pressure sensors. This flexibility is crucial in aerospace, where different aspects of flight—like temperature, pressure, and altitude—must be monitored.

C++ Libraries for Aerospace Engineering
Numerous libraries enhance C++'s capabilities in aerospace engineering, providing tools specifically designed for simulations, graphics, and data structures.
Popular C++ Libraries
Open CASCADE Technology (OCCT)
OCCT is a comprehensive library for 3D CAD, providing tools for geometric modeling and visualization. This library is widely used in aerospace design and simulation applications, facilitating the construction of complex geometric models.
OpenGL
OpenGL is a robust graphics library for rendering 2D and 3D graphics. In aerospace, it is often utilized to visualize flight paths or simulate environmental conditions. A simple setup for rendering triangles in OpenGL looks like this:
glBegin(GL_TRIANGLES);
glVertex3f(0.0f, 1.0f, 0.0f);
glVertex3f(-1.0f, -1.0f, 0.0f);
glVertex3f(1.0f, -1.0f, 0.0f);
glEnd();
This snippet demonstrates how to draw basic shapes, which can be scaled and expanded upon for complex visualizations.
Boost C++ Libraries
This collection of libraries offers utilities that extend the functionality of the C++ standard library. It provides solutions for various tasks, including multi-threading, which is essential for processing real-time aviation data, ensuring responsiveness in various applications.
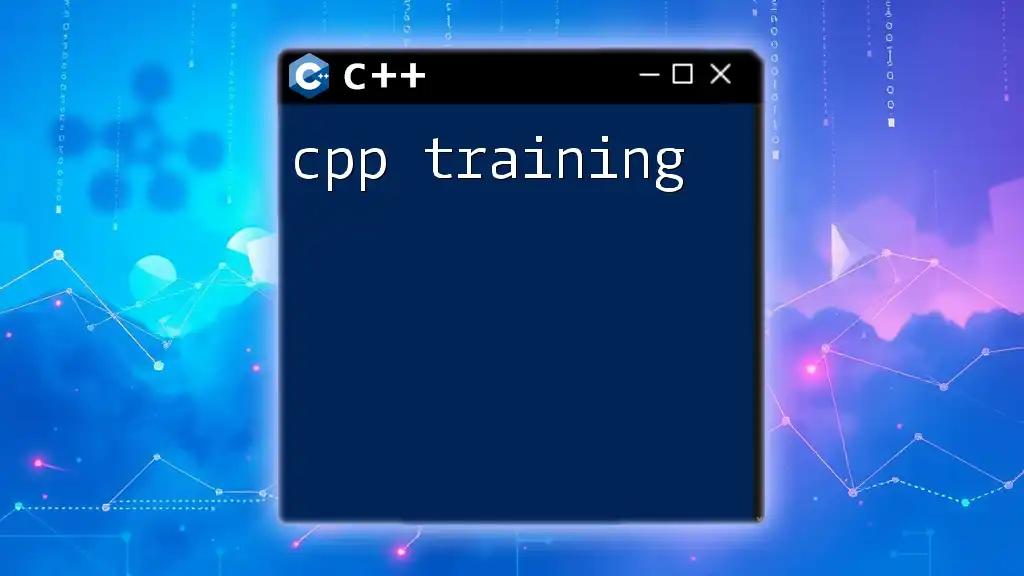
C++ for Embedded Systems in Aerospace
Challenges in Embedded Systems Programming
Embedded systems are crucial in aerospace since they often control essential operations in aircraft. These systems face unique challenges, including stringent resource constraints and the need for real-time performance.
C++ in Embedded Systems
C++'s advantages over C in embedded systems include enhanced type safety and abstraction, making it with a clean object-oriented model practical for managing complex operations. Software in avionics systems, which must meet high reliability and safety standards, benefits significantly from C++'s structured approach.

Case Studies: C++ in Aerospace Engineering
Flight Simulation Software
C++ is heavily used in developing flight simulation software, offering the necessary speed and efficiency to create realistic scenarios. This software often combines physics engines with user interface components, showcasing the versatility of C++ in building complex systems.
Real-time Processing in UAVs (Unmanned Aerial Vehicles)
In UAVs, C++ is employed for navigation and flight control. Real-time data processing is essential for operating UAVs effectively, requiring multi-threaded applications that can manage various input sources simultaneously. A basic example of multi-threading in C++ for navigation calculations looks like this:
#include <thread>
void calculateNavigation() {
// Navigation algorithm
}
int main() {
std::thread navThread(calculateNavigation);
navThread.join();
return 0;
}
By leveraging multi-threading, engineers can ensure that navigation updates occur continuously, enhancing the reliability of UAV operations.
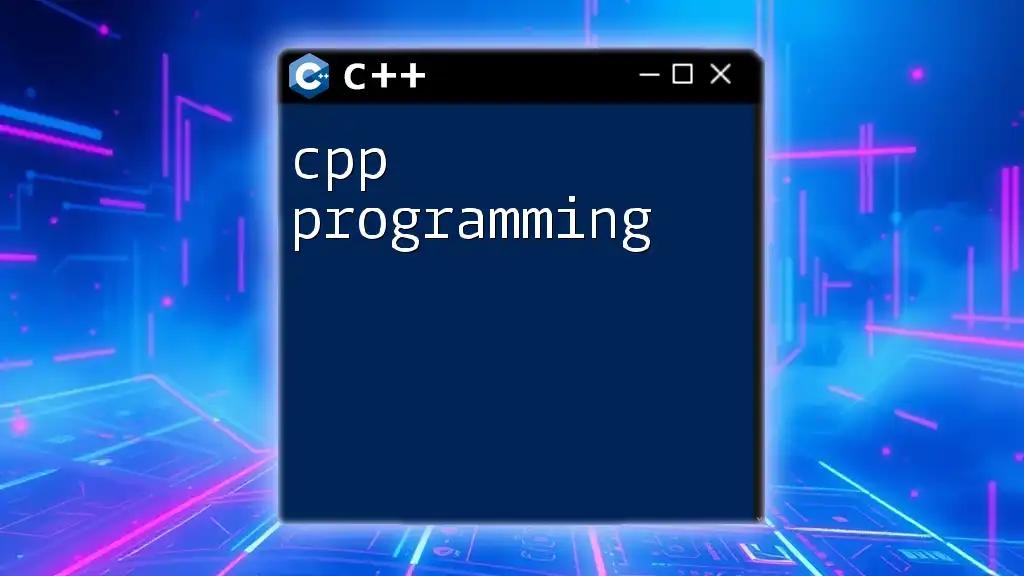
Best Practices for Using C++ in Aerospace
Coding Standards
Maintaining readable and maintainable code is critical in aerospace applications. Following established coding standards, such as those from MISRA for C and C++, ensures that codebases remain understandable and less prone to errors—critical in safety-sensitive applications.
Testing and Debugging Techniques
Testing and debugging are paramount in aerospace software development. Implementing unit testing frameworks enables systematic validation of code components. Simulation environments provide a safe space to validate software in conditions that mimic real-world scenarios, thereby enhancing reliability and safety.

Conclusion
C++ is a fundamental tool in the field of aerospace engineering, allowing for the development of robust software systems that meet the industry's dynamic demands. By mastering C++ and leveraging its characteristics, professionals in aerospace engineering can create more efficient, reliable, and scalable systems. With the continuous evolution of technology, C++ will undoubtedly remain a key language for innovative aerospace applications.
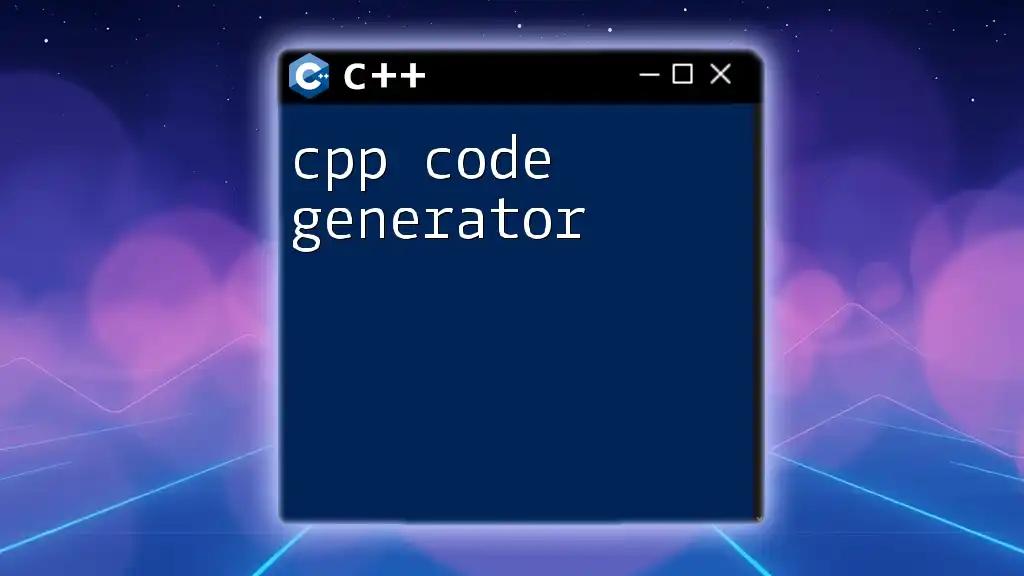
Further Reading and Resources
For those looking to deepen their knowledge of C++ in aerospace engineering, numerous resources, including books, online courses, and educational websites, can provide valuable insights and skills for success in this exciting field.