A C++ physics engine is a software component that simulates physical systems and interactions in a virtual environment, allowing developers to create realistic movements and behaviors of objects within a game or application.
Here’s a simple code snippet that demonstrates a basic physics update loop:
#include <iostream>
#include <vector>
class Body {
public:
float mass;
float position;
float velocity;
Body(float m, float pos) : mass(m), position(pos), velocity(0) {}
void update(float deltaTime) {
position += velocity * deltaTime; // Update position based on velocity
}
};
int main() {
std::vector<Body> bodies = { Body(1.0f, 0.0f), Body(2.0f, 5.0f) };
float deltaTime = 0.016f; // Simulating a 60 FPS update
for (auto& body : bodies) {
body.update(deltaTime);
std::cout << "Body Position: " << body.position << std::endl;
}
return 0;
}
This code creates a simple structure where physical bodies can be updated each frame based on their velocity and the elapsed time.
Understanding Physics Engines
What is a Physics Engine?
A physics engine is a software component that simulates physical systems, allowing developers to create realistic interactions and animations in games and simulations. It plays a crucial role in rendering movement, collision detection, and response to forces within a simulated environment.
Types of Physics Engines
Physics engines can generally be divided into two categories: real-time and offline/simulation engines.
Real-time Engines like Bullet and Box2D are optimized for performance, making them ideal for games where quick calculations are necessary. These engines enable smooth gameplay and responsive interactions, focusing on real-time simulation.
In contrast, Offline/Simulation Engines such as PhysX and Open Dynamics Engine (ODE) are tailored for more complex simulations requiring higher accuracy over speed, commonly used in scientific simulations and cinematic effects.
Features of Physics Engines
Collision Detection is a fundamental feature of physics engines. It involves determining whether two or more objects collide, using methods such as bounding volumes which simplify calculations. Collision detection is often segmented into two phases: broad phase (quickly identifying potential colliders) and narrow phase (detecting precise collisions).
Rigid Body Dynamics refers to the simulation of solid objects that do not deform under stress. Understanding how to properly apply forces, manage torques, and impose constraints is essential for creating realistic behavior in these objects.
Soft Body Dynamics takes this further, simulating deformable objects that can change shape under forces. This creates a more flexible and lifelike representation of materials in a simulated world.
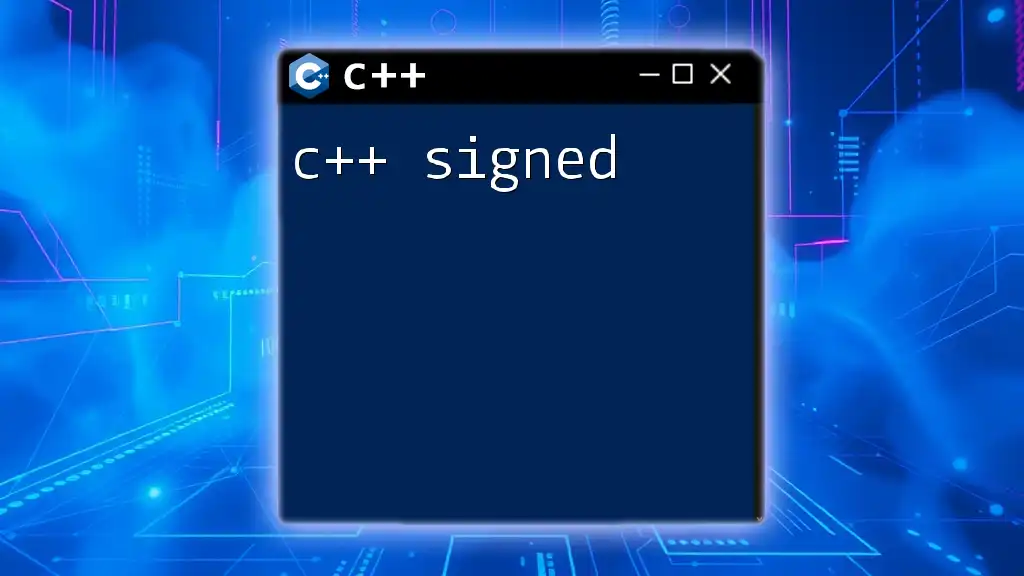
Getting Started with a C++ Physics Engine
Setting Up Your Development Environment
Before diving into coding, you need to prepare your development environment. This requires choosing the right software and tools to facilitate your project.
Required Software and Tools: IDEs like Visual Studio or Code::Blocks are popular choices among developers. For physics engines, you may consider using libraries like Bullet or Box2D, which have extensive documentation and community support.
Selecting a Physics Engine
When selecting a physics engine, consider the following factors:
- Performance: Evaluate how the engine handles scenarios demanding high frame rates.
- Ease of Integration: Check how easily it can be integrated into your existing projects.
- Community Support: A strong community can provide valuable resources and assistance.
Installation Instructions
To utilize a physics engine, you generally need to install it correctly. Here’s how you can set up Bullet as an example:
- Clone the Physics Engine Repository: Use Git to clone the Bullet repository from GitHub.
- Build the Library: Use CMake or Makefile to configure and build the library, ensuring all dependencies are resolved.
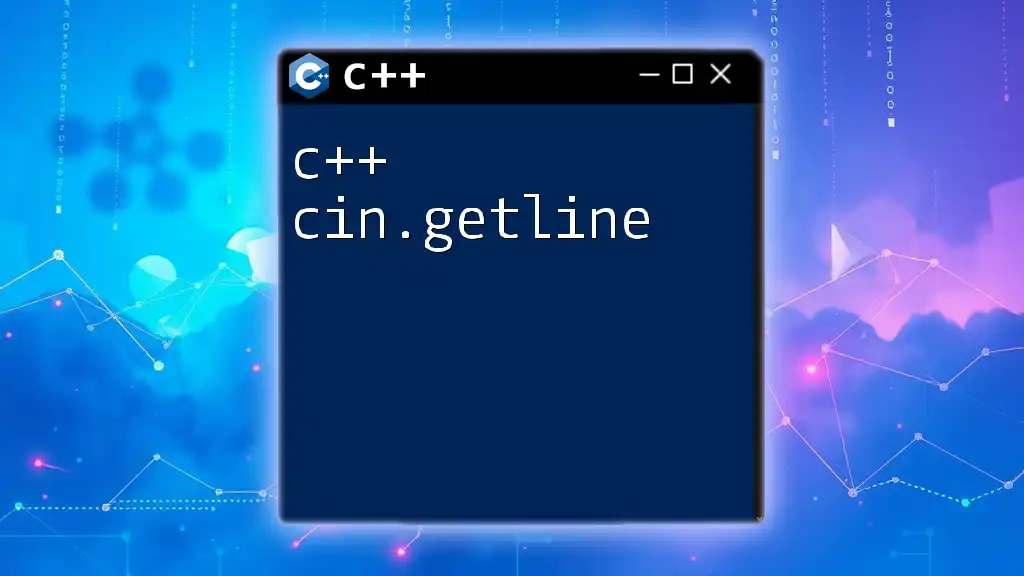
Basic Concepts in C++ Physics Engine Implementation
Core Components
The core components of a physics engine center around the world and bodies.
A World object simulates physics and is the container for all physical entities. It keeps track of the physics simulation, including all objects and their interactions.
Bodies refer to the objects in your simulation, which can be moved, rotated, and manipulated. Here’s how to create a rigid body in Bullet:
btRigidBody* createRigidBody(float mass, const btTransform& startTransform, btCollisionShape* shape) {
btVector3 inertia(0, 0, 0);
shape->calculateLocalInertia(mass, inertia);
btDefaultMotionState* motionState = new btDefaultMotionState(startTransform);
btRigidBody::btRigidBodyConstructionInfo rbInfo(mass, motionState, shape, inertia);
return new btRigidBody(rbInfo);
}
Physics Simulation Loop
Creating a physics simulation involves setting up a loop that continually updates the world and renders the objects. Here’s a simplified way to implement the simulation step:
void simulatePhysics(float deltaTime) {
dynamicsWorld->stepSimulation(deltaTime, 10);
}
This simple function will execute each frame of your simulation, keeping the physics calculations in sync with your game's timeline.
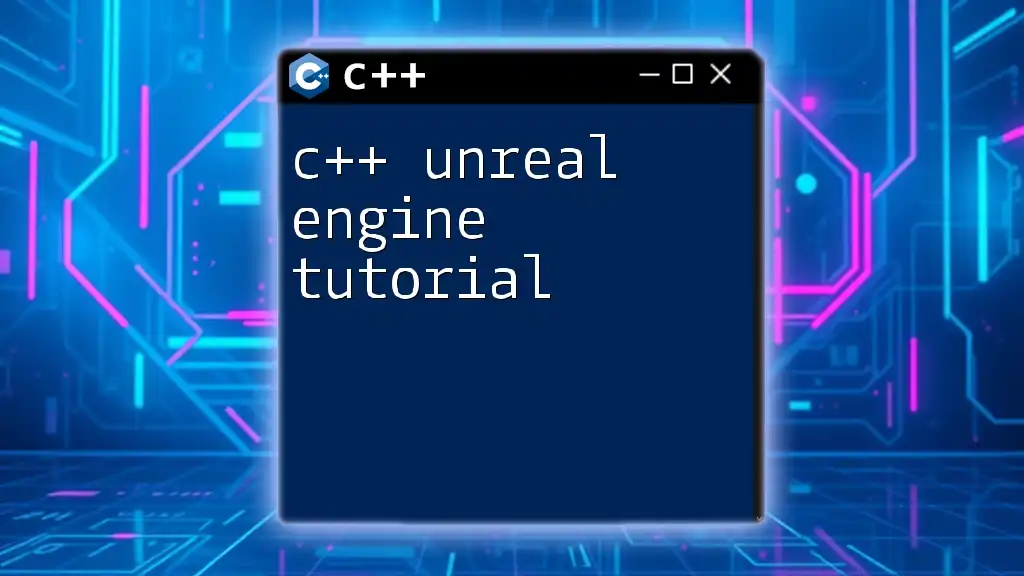
Integrating the Physics Engine into a C++ Project
Example: Building a Simple Simulation
To understand how to put it all together, let's outline the steps involved in creating a simple simulation.
Project Setup: Organize your project by creating a structured folder hierarchy to separate physics code, graphics code, and resources.
Creating Physics Objects: Use the provided code snippets to initialize basic shapes, like boxes and spheres:
btCollisionShape* boxShape = new btBoxShape(btVector3(1, 1, 1));
btCollisionShape* sphereShape = new btSphereShape(1);
Handling User Input: It is vital to allow users to manipulate the simulation. Implementing basic input handling can enable object movement. Here’s a snippet that shows how to respond to user input:
if (keyPressed == 'W') {
// Apply force to move the object
btVector3 force(0, 0, 10);
body->applyCentralForce(force);
}
Rendering the Scene
Rendering your scene involves integrating with graphics libraries such as OpenGL or DirectX. Make sure to separate the rendering logic from your physics logic for better maintainability.
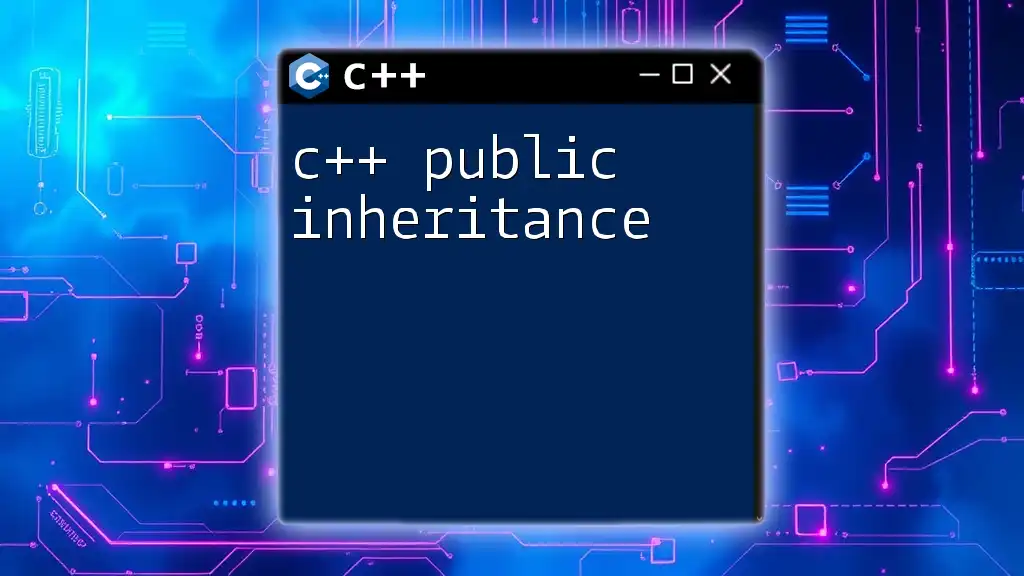
Debugging and Optimization
Common Issues in Physics Simulations
As in any development, you may encounter issues such as collision detection failures. Debugging these problems involves tracking down faulty interactions, adjusting collision shapes, or tweaking the simulation parameters.
Performance Bottlenecks can arise when managing a high number of objects. Using effective techniques like implementing broad-phase collision detection strategies can optimize performance significantly.
Best Practices
To maintain a clean and efficient codebase, it’s essential to organize and structure your code properly. Separate physics calculations from rendering logic, allowing for scalability and easier debugging.
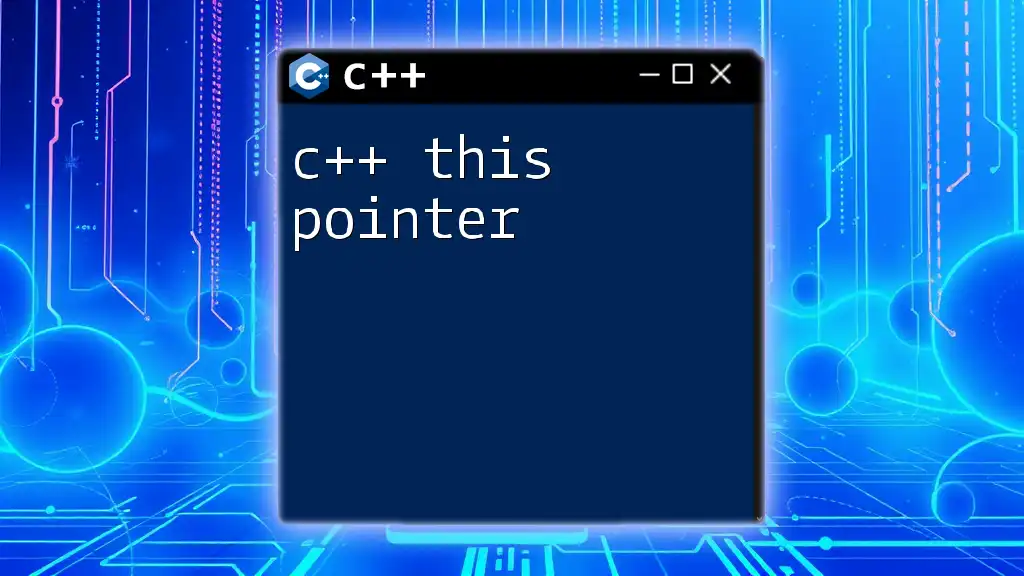
Advanced Topics in Physics Engines
Implementing Constraints and Joints
One advanced feature in physics engines is the ability to create constraints and joints. These allow for more complex interactions between objects. For example, creating a hinge joint can be done as follows:
btHingeConstraint* hinge = new btHingeConstraint(*bodyA, *bodyB, anchor, axis);
constraintSolver->addConstraint(hinge);
Continuous Collision Detection (CCD)
Continuous Collision Detection (CCD) is necessary for objects moving at high speeds. This technique helps prevent the occurrence of tunneling effects, ensuring collisions are accurately detected even for fast-moving bodies.
Soft Body Physics
Exploring soft body physics opens up new possibilities for simulating flexible materials, like cloth or jelly. Implementing these simulations can be more challenging but adds significant realism to your projects.
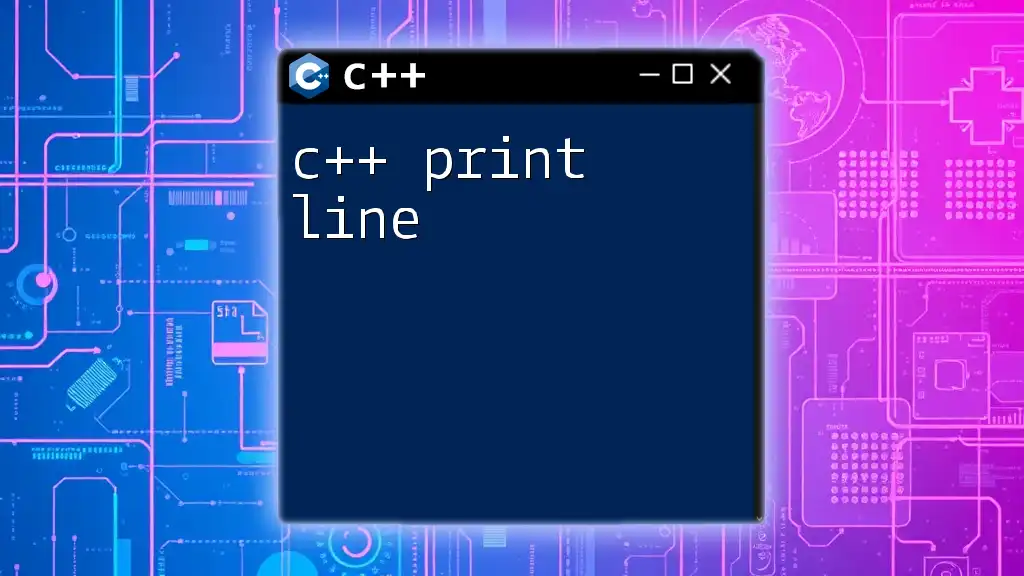
Conclusion
In summary, the power of a C++ physics engine cannot be overstated in game and simulation development. The ability to simulate realistic interactions enhances the user experience and brings virtual worlds to life. With the foundational knowledge shared in this guide, you have the tools and insights required to start implementing physics in your projects successfully.
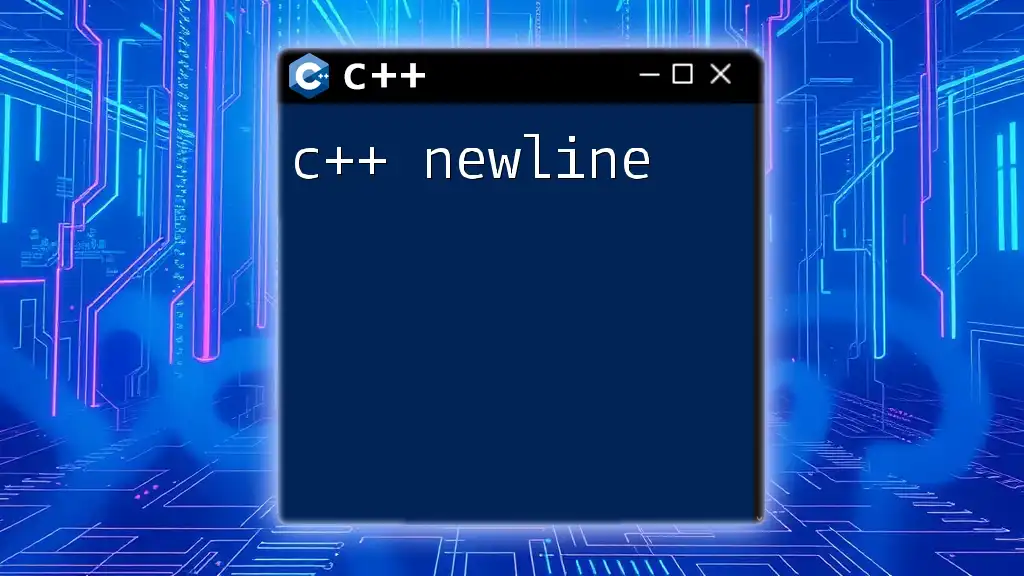
Call to Action
Join the C++ Physics community and share your progress or questions in forums. Follow for more tips and insights to enhance your development endeavors!