Public inheritance in C++ allows a derived class to inherit the public and protected members of a base class, promoting an "is-a" relationship while maintaining access control.
Here’s a simple code snippet demonstrating public inheritance:
#include <iostream>
class Base {
public:
void show() {
std::cout << "Base class method." << std::endl;
}
};
class Derived : public Base {
public:
void display() {
std::cout << "Derived class method." << std::endl;
}
};
int main() {
Derived obj;
obj.show(); // Calling inherited method from Base class
obj.display(); // Calling method from Derived class
return 0;
}
What is Public Inheritance?
Definition
Public inheritance is a key concept within C++ that allows a class (referred to as the "derived class") to inherit properties and behaviors from another class (the "base class"). In this hierarchical relationship, the derived class expands upon the functionality provided by the base class. With public inheritance, all public members of the base class remain accessible by the derived class and any objects of the derived class.
Characteristics of Public Inheritance
In public inheritance, several characteristics define how the classes operate:
-
Accessibility of Base Class Members: All public members of the base class are accessible by the derived class. Private members remain inaccessible, while protected members can still be accessed but are not available outside of the derived class hierarchy.
-
How Derived Classes Utilize Base Class Components: The derived class can extend or modify the behavior established by the base class, creating a flexible relationship that promotes code reuse.
Consider the following snippet that illustrates a simple class hierarchy:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Barking..." << std::endl;
}
};
In this example, the `Dog` class inherits from the `Animal` class, gaining access to the `eat()` method while adding its own behavior with `bark()`.
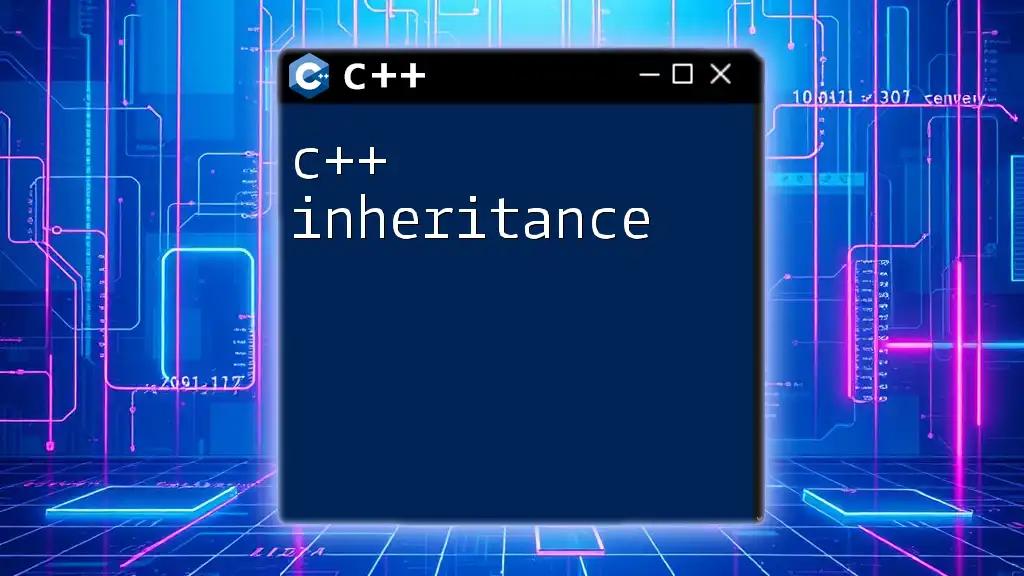
Why Use Public Inheritance?
Code Reusability
A primary advantage of public inheritance is code reusability. By inheriting from a base class, a derived class can reuse existing methods and properties, minimizing code duplication. This leads to cleaner and more maintainable code.
For example, if you have a `Shape` base class with methods for calculating area and perimeter, you can create specialized derived classes like `Circle` and `Square`, each of which reuses the shared functionality.
Polymorphism
Public inheritance lays the foundation for polymorphism in C++. This means that a pointer or reference to a base class can refer to an object of a derived class. This capability can be extremely powerful, allowing functions to work with different classes using common interfaces.
class Base {
public:
virtual void show() {
std::cout << "Base class." << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class." << std::endl;
}
};
void display(Base &b) {
b.show(); // Calls the appropriate show() based on the object type
}
In this example, calling `display()` with an object of `Derived`, will invoke the `show()` method from `Derived`, showcasing polymorphic behavior.
Design Principles
Public inheritance allows you to adhere to significant design principles in software development, specifically the Liskov Substitution Principle (LSP). According to LSP, objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. This ensures that derived classes maintain the integrity of base class interfaces, resulting in robust and flexible code architecture.

Implementing Public Inheritance in C++
Basic Syntax
The syntax for implementing public inheritance in C++ is straightforward. You simply declare a derived class following the base class name and specify the inheritance type with the keyword `public`.
class Base {
public:
void display() {
std::cout << "Base class display function." << std::endl;
}
};
class Derived : public Base {
public:
void show() {
std::cout << "Derived class show function." << std::endl;
}
};
In this syntax, `Derived` is publicly inheriting from `Base`, meaning all public members of `Base` are accessible in `Derived`.
Access Control
Member Accessibility
Access control in public inheritance is crucial. The followed rules are:
- Public Members: Accessible through both the base and derived classes.
- Protected Members: Accessible in the derived classes, but not outside of them.
- Private Members: Inaccessible from the derived class.
Consider the following illustration of member accessibility:
class Base {
public:
void publicFunction() {
std::cout << "Public function called." << std::endl;
}
protected:
void protectedFunction() {
std::cout << "Protected function called." << std::endl;
}
private:
void privateFunction() {
std::cout << "Private function called." << std::endl;
}
};
class Derived : public Base {
public:
void accessFunctions() {
publicFunction(); // Accessible
protectedFunction(); // Accessible
// privateFunction(); // Not accessible
}
};
Here, `Derived` can access `publicFunction()` and `protectedFunction()`, but cannot access `privateFunction()` from the `Base` class.

Overriding Virtual Functions
What are Virtual Functions?
Virtual functions are special functions declared in a base class that can be overridden in a derived class. They are declared using the `virtual` keyword and enable dynamic polymorphism, allowing for the behavior of a derived class to be invoked instead of the base class function at runtime.
Overriding in Public Inheritance
When a derived class overrides a virtual function, it provides its specific implementation. The override can be achieved through the `override` specifier, which improves code readability and helps catch errors.
class Base {
public:
virtual void speak() {
std::cout << "Base speaking." << std::endl;
}
};
class Derived : public Base {
public:
void speak() override {
std::cout << "Derived speaking." << std::endl;
}
};
In the example above, the `speak()` function in the `Derived` class overrides the same function in the `Base` class, allowing customized behavior when invoked.

The "is-a" Relationship
Explanation of the "is-a" Concept
In object-oriented programming, the "is-a" relationship is a fundamental principle that indicates a derived class is a more specific version of the base class. Public inheritance establishes this kind of relationship.
Real-World Examples
To better understand this concept, consider the real-world relationships represented in C++:
- Animal and Dog: A `Dog` "is-a" `Animal`. Hence, it can inherit properties like `eat()` while defining its specific behaviors, such as `bark()`.
- Vehicle and Car: A `Car` "is-a" `Vehicle`, gaining access to functions like `drive()` while adding its own features.
This relationship encourages intuitive class hierarchies that benefit from clear abstractions.

Common Pitfalls and Best Practices
Avoiding Diamond Problem
One common pitfall with public inheritance is the diamond problem, which occurs in multiple inheritance scenarios. This happens when two base classes inherit from the same parent class. When a derived class inherits from both base classes, ambiguity arises regarding which base class function should be invoked.
To avoid this, C++ uses a virtual inheritance mechanism, allowing for a single shared instance of the base class. Implementing it requires using the `virtual` keyword in the base class declaration.
Best Practices
To make the most of public inheritance, consider these best practices:
- Prefer using composition over inheritance when the relationship is not naturally an "is-a" relationship. This results in more flexible and decoupled code.
- Balance between code reuse and maintainability. While inheritance promotes reuse, be cautious not to create excessively complex hierarchies.
- Ensure that derived classes appropriately adhere to the base class's interface and behavior, maintaining the integrity of polymorphism.

Conclusion
Public inheritance in C++ is a powerful tool for creating flexible, reusable, and maintainable code structures. By understanding its principles, developers can leverage the capabilities of inheritance to build complex systems while avoiding common pitfalls. As you explore the world of C++, practice implementing these concepts through real-world examples, enhancing both your skillset and your software designs.

Further Reading
For deepening your understanding of C++ and object-oriented programming, consider exploring resources that cover private and protected inheritance, interfaces, and design patterns. There’s a wealth of knowledge waiting to help you become a more proficient C++ developer!

Code Samples Repository
Access a comprehensive repository of code samples discussed throughout this article to enhance your learning and experimentation.