In C++, access specifiers `public`, `private`, and `protected` control the visibility of class members, determining how they can be accessed from outside the class or by derived classes.
class Base {
public:
int publicVar; // Accessible from anywhere
protected:
int protectedVar; // Accessible in derived classes
private:
int privateVar; // Accessible only within this class
};
Understanding Access Specifiers in C++
Importance of Access Specifiers
Access specifiers are a fundamental feature in C++ that controls the visibility and accessibility of class members. They are essential for achieving data encapsulation and secure programming, preventing unintended interference with internal states and behavior. By effectively using access specifiers, developers can expose only those aspects of a class that are necessary, ensuring a clean and understandable interface.
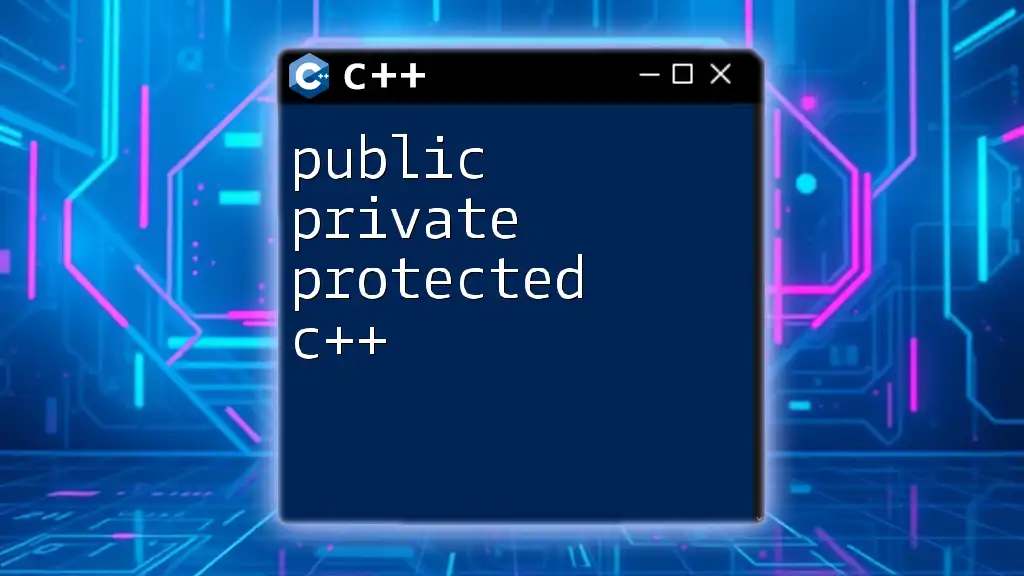
Understanding Public, Private, and Protected
What is Public in C++?
The public access specifier allows members of a class to be accessible from any part of the program. This means that both member variables and member functions declared as public can be accessed outside the class. This is particularly useful for functions that are intended to be part of the class's interface.
Example:
class PublicExample {
public:
int publicVariable;
void publicMethod() {
// Function logic here
}
};
In this example, `publicVariable` and `publicMethod()` can be accessed anywhere in the code that has visibility to `PublicExample`, allowing external code to interact with these members freely.
What is Private in C++?
The private access specifier restricts access to class members so that they can only be accessed within the class itself. This is a key element of encapsulation as it protects the internal state of the class and prevents external code from making arbitrary alterations.
Example:
class PrivateExample {
private:
int privateVariable;
void privateMethod() {
// Function logic here
}
friend class FriendClass; // Example of friend class access
};
In this case, `privateVariable` and `privateMethod()` cannot be accessed from outside `PrivateExample`, ensuring that only internal methods can interact with them.
What is Protected in C++?
The protected access specifier is a middle ground between public and private. Members declared as protected can be accessed within the class itself and by derived classes. This allows for a controlled way of accessing class internals in the context of inheritance.
Example:
class ProtectedExample {
protected:
int protectedVariable;
public:
void setProtectedVariable(int value) {
protectedVariable = value; // Accessible within the class and its derived classes
}
};
Here, `protectedVariable` can be accessed in `ProtectedExample` itself and any class that derives from it, but it remains hidden from the global scope.
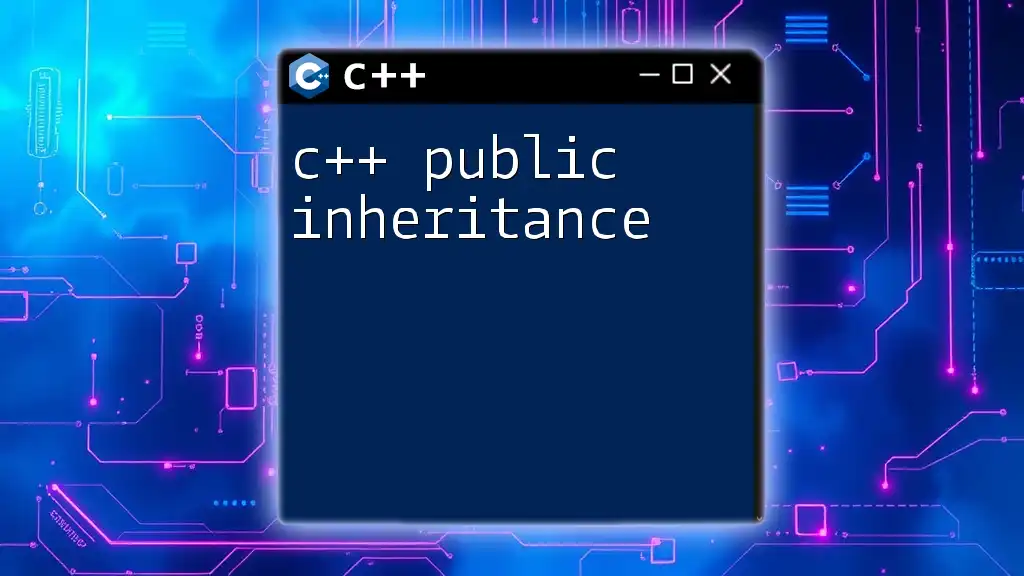
Access Specifier Hierarchies
Public Members
Public members form the interface of the class, allowing external components to interact with the class. They serve as the primary means to access functionality and data of the class without exposing the internals.
Private Members
Private members are crucial for maintaining the integrity and security of the class’s data. By preventing external access, developers can safely encapsulate logic that should not be exposed, reducing the risk of unintended behavior from outside the class.
Protected Members
Protected members provide a way for derived classes to access certain data from their base class without exposing it completely to the outside world. This is particularly useful in designs that utilize inheritance, allowing subclasses to build upon constraints established by their parent class.
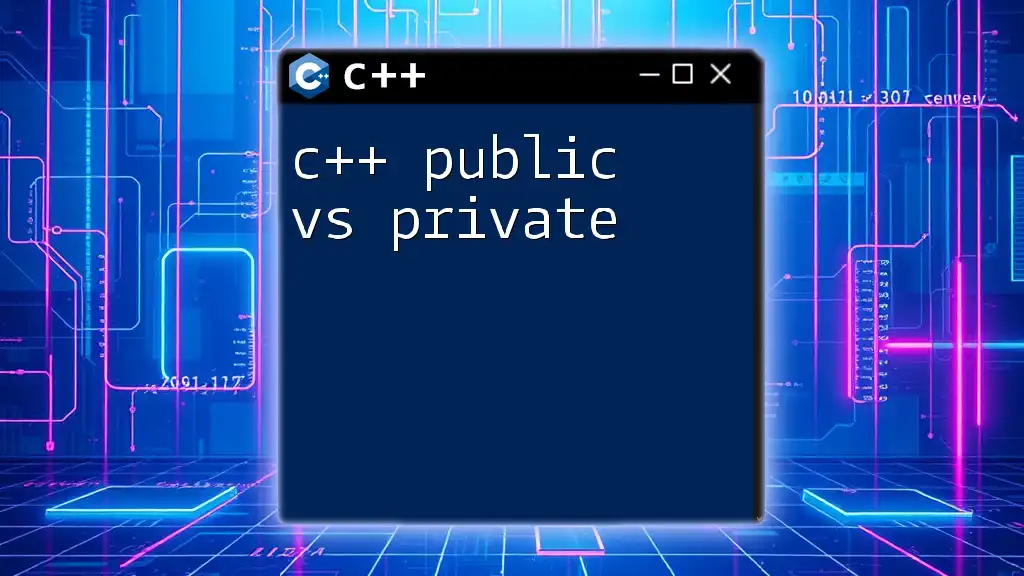
Access Specifiers in Inheritance
Public Inheritance
Public inheritance allows base class members designated as public to be accessible from derived classes as well. In this model, the derived class can extend the functionality of the base class while maintaining its interface.
Example:
class Base {
public:
void basePublic() {
// Base class public function logic
}
};
class Derived : public Base {
public:
void derivedFunction() {
basePublic(); // Accessible
}
};
In this scenario, `derivedFunction()` can call `basePublic()` without restriction.
Protected Inheritance
When a class is inherited as protected, all public and protected members of the base class remain accessible from derived classes, but they cannot be accessed publicly. This is less common and is typically used to create a more restricted interface in inheritance.
Private Inheritance
Private inheritance creates a tight coupling between classes, where the base class members become inaccessible to the outside world and are accessible only to the derived class. It’s often used in terms of class composition rather than class hierarchy.
Example:
class Derived : private Base {
public:
void derivedFunction() {
basePublic(); // Not Accessible outside Derived
}
};
Here, outside of `Derived`, there’s no access to `basePublic()` or any other members of `Base`.
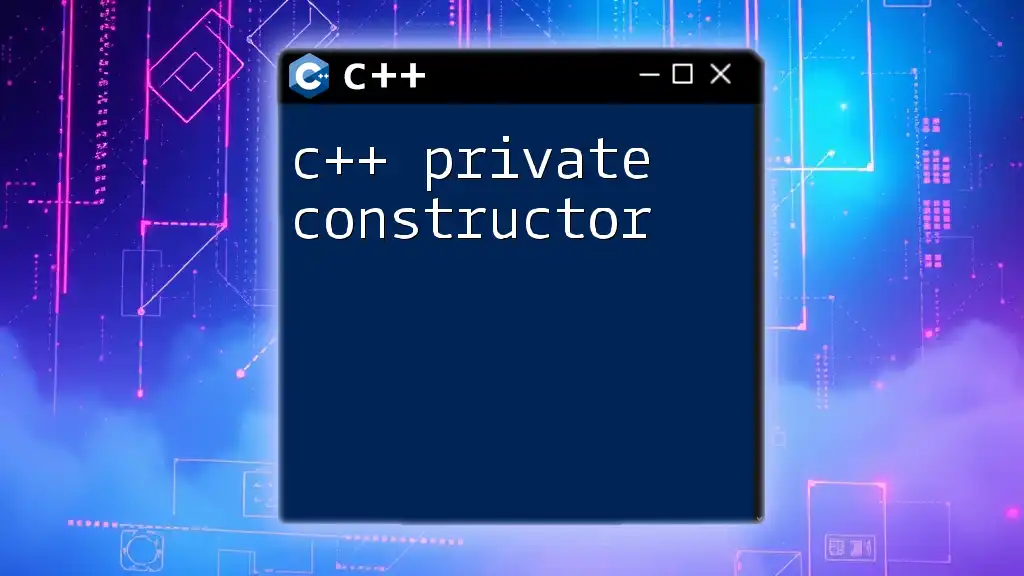
Differences Between Public, Private, and Protected
Summary of Key Differences
The differences between public, private, and protected can be summarized in terms of accessibility:
- Public: Accessible from anywhere.
- Private: Accessible only within the class itself.
- Protected: Accessible within the class and its derived classes.
Use Cases for Each Access Specifier
- Use public for methods that are intended to be widely used as part of the class interface.
- Use private for internal variables and methods that should not be exposed, ensuring the class maintains its integrity.
- Use protected when developing a class hierarchy that requires certain members to be accessible by derived classes while hiding them from the outer world.
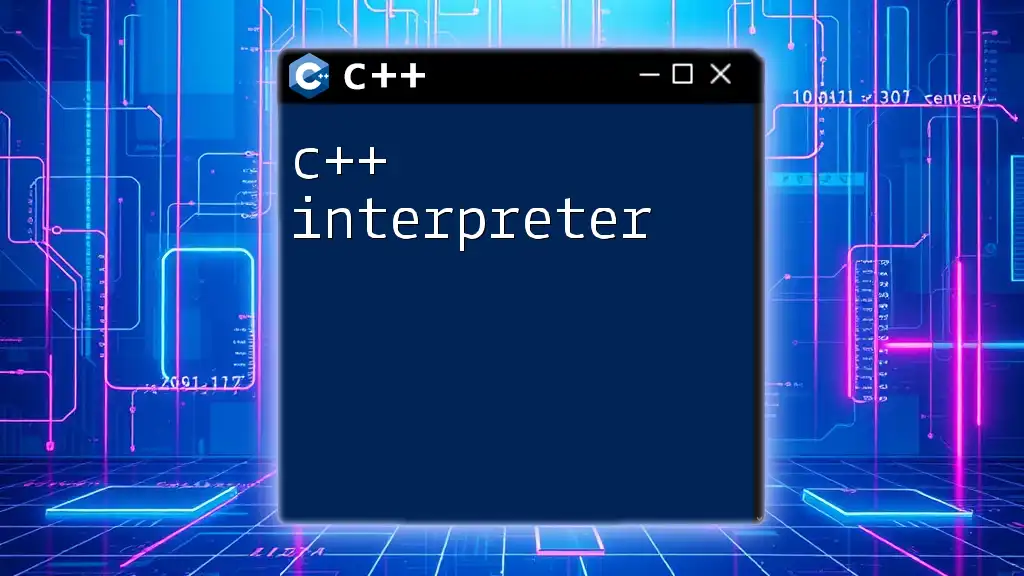
Best Practices for Using Access Specifiers
Use of Getters and Setters
To ensure controlled access to private member variables, consider implementing getters and setters. This approach upholds encapsulation and allows validation checks before assigning or retrieving values.
Example:
class Encapsulated {
private:
int number;
public:
void setNumber(int num) {
number = num; // Additional validation can be added here
}
int getNumber() {
return number; // Simple access to the private variable
}
};
Favoring Private Over Public
As a best practice, lean towards making class members private whenever possible. This approach reduces the risk of introducing bugs from unintended interactions and achieves a cleaner separation of concerns.
Leveraging Protected in Base Classes
Use protected access for members that are meant to be shared with derived classes but should remain hidden from the general public. This can simplify class relationships while ensuring the underlying implementation remains secure.
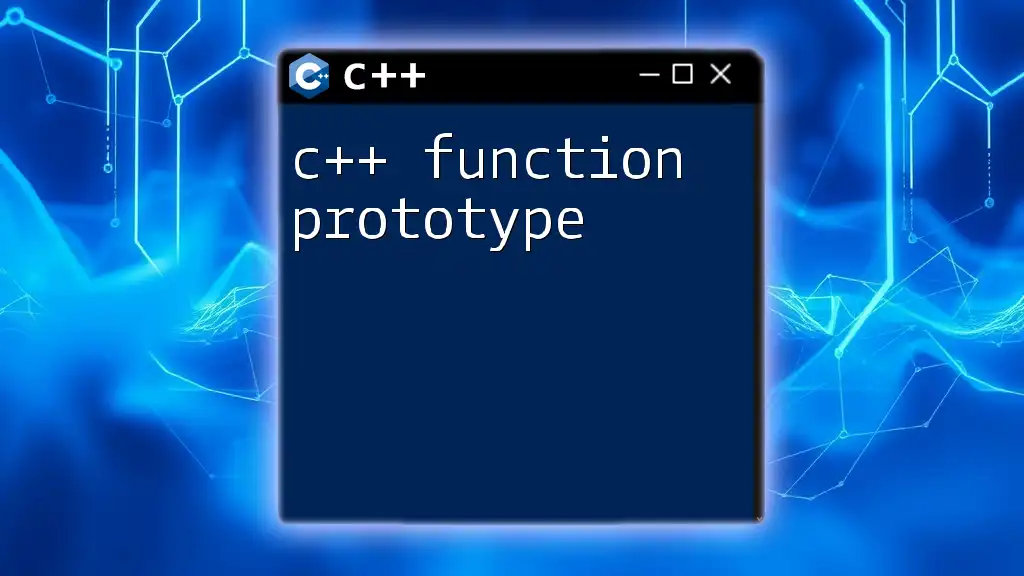
Conclusion
Access specifiers—public, private, and protected—are integral to managing visibility and accessibility in C++. Proper use enhances security, enforces encapsulation, and enables clear communication and functionality between classes. By mastering these concepts, developers will strengthen their design choices in C++, paving the way for robust, maintainable code.
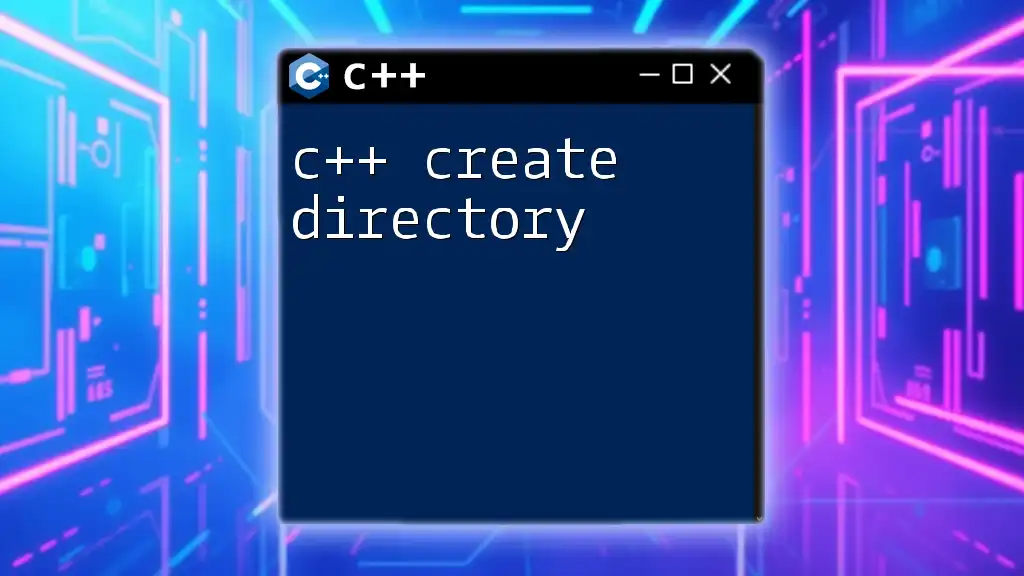
Additional Resources
For further reading and exploration of C++ access specifiers, consider diving into recommended books, online tutorials, and the official C++ documentation. Knowledge in this area is critical for advancing your skills as a proficient C++ programmer.