To create a directory in C++, you can use the `mkdir` function from the <sys/stat.h> header, as shown in the following code snippet:
#include <sys/stat.h>
#include <iostream>
int main() {
const char* dirName = "new_directory";
if (mkdir(dirName, 0777) == 0) {
std::cout << "Directory created successfully!" << std::endl;
} else {
perror("Error creating directory");
}
return 0;
}
Understanding Directories in C++
What is a Directory?
A directory, also known as a folder, is a container used to organize files within a file system. It allows users to structure their storage hierarchically, making it easier to manage files. In programming, working with directories is essential when dealing with file I/O operations.
Directory Structure in C++
C++ provides ways to interface with the operating system’s file structure. Understanding how the C++ language interacts with directories is crucial for effectively managing files and directories in your application.
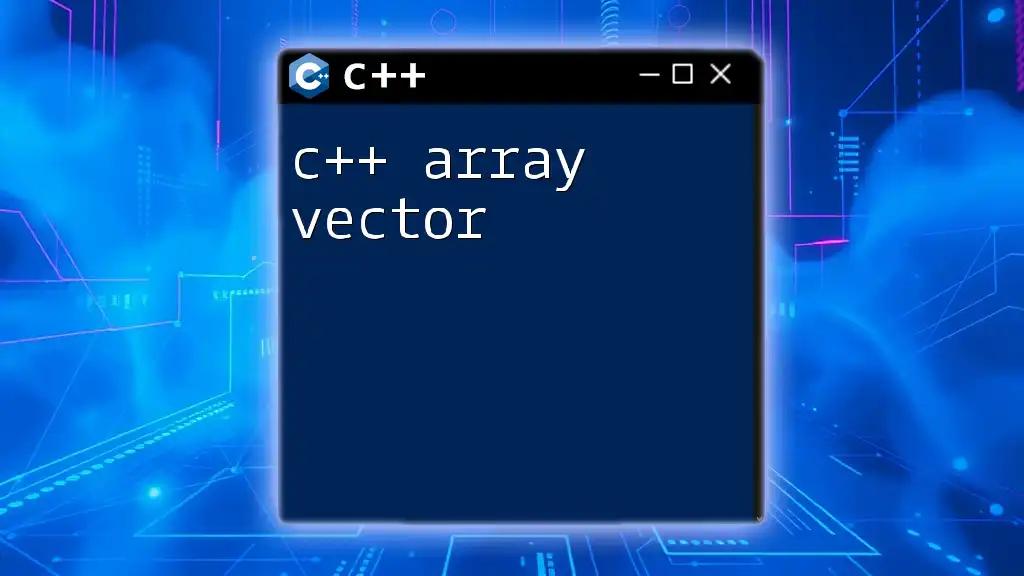
Libraries Required for Creating Directories
Standard Library Overview
Starting from C++17, the `<filesystem>` library was introduced, enriching the standard library with numerous features to work with files and directories efficiently. It allows developers to create, remove, and manipulate directories easily.
Backward Compatibility
For those using older versions of C++, system calls can be utilized to create directories. However, this method is less portable and may differ based on operating systems.

Setting Up the Environment
Compiler Requirements
To fully leverage C++17 features, ensure that your compiler supports these standards. Popular compilers like GCC, Clang, and MSVC have added support for the `<filesystem>` library. Check your compiler's documentation to enable C++17 features:
- GCC: Use the flag `-std=c++17`
- Clang: Use the flag `-std=c++17`
- MSVC: No additional flags are needed if you are using a recent version.
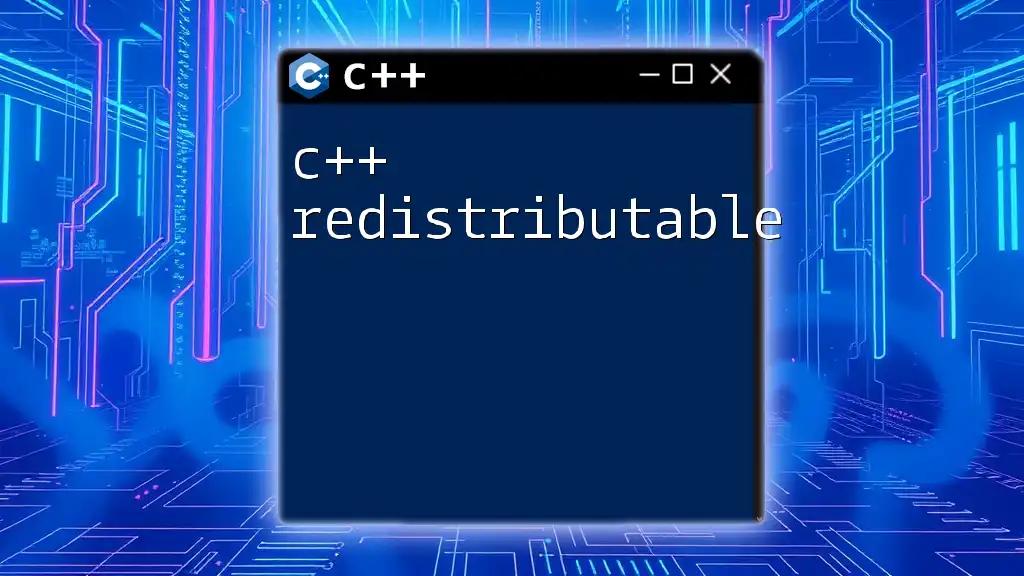
Creating a Directory Using C++17 `<filesystem>`
Basic Syntax
To create a directory, you can utilize the `std::filesystem::create_directory` function, which checks for the existence of the directory before creating it.
Code Example
#include <iostream>
#include <filesystem>
int main() {
std::filesystem::path dirPath("new_directory");
if (std::filesystem::create_directory(dirPath)) {
std::cout << "Directory created successfully: " << dirPath << std::endl;
} else {
std::cout << "Directory already exists or could not be created" << std::endl;
}
return 0;
}
In this example, we create a new directory named `new_directory`. The output informs the user whether the directory was successfully created or if it already exists.
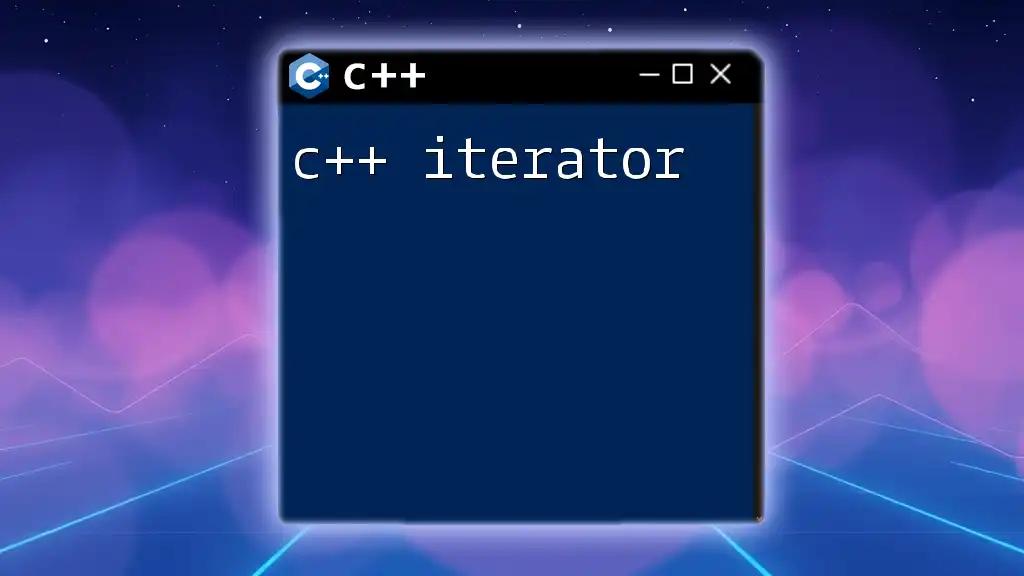
Handling Errors While Creating Directories
Common Errors
When creating directories, you may encounter issues, such as permission errors or trying to create a directory that already exists. It's essential to handle these gracefully to enhance user experience.
Error Handling in C++
Using exceptions effectively helps manage errors that arise during directory creation. Surround your directory creation logic with a try-catch block to catch and handle any exceptions.
Code Example for Error Handling
#include <iostream>
#include <filesystem>
int main() {
std::filesystem::path dirPath("another_directory");
try {
if (std::filesystem::create_directory(dirPath)) {
std::cout << "Directory created successfully: " << dirPath << std::endl;
}
} catch (const std::exception& e) {
std::cerr << "Error occurred: " << e.what() << std::endl;
}
return 0;
}
In this example, if an error occurs during the directory creation process, it is caught, and an error message is displayed, giving insight into what went wrong.
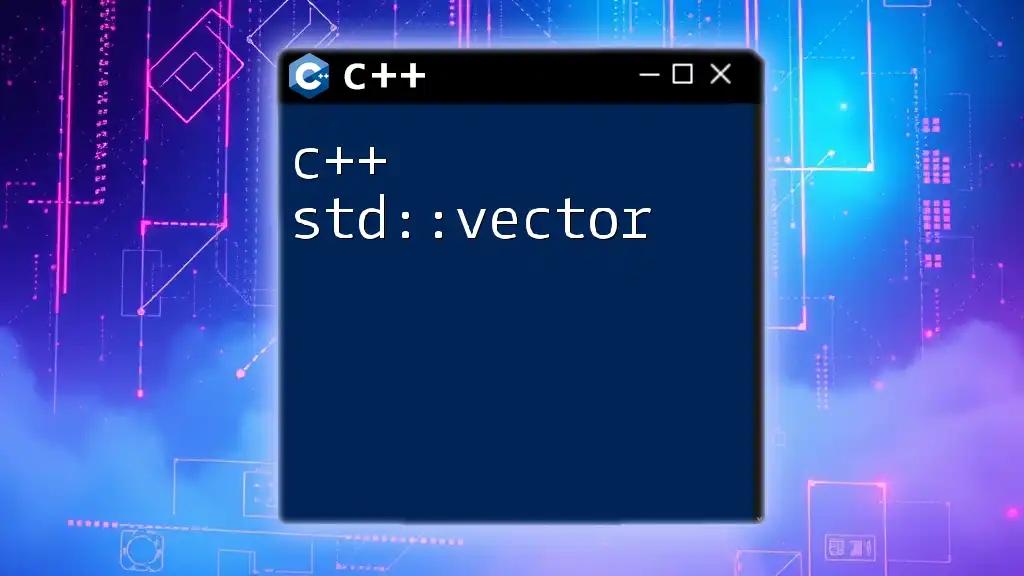
Creating Nested Directories
Using `create_directories`
The `std::filesystem::create_directories` function allows you to create nested directories efficiently. If the parent directory does not exist, it will create it, making it ideal for structuring files in a hierarchy.
Code Example
#include <iostream>
#include <filesystem>
int main() {
std::filesystem::path nestedDirPath("parent_dir/child_dir");
if (std::filesystem::create_directories(nestedDirPath)) {
std::cout << "Nested directories created successfully: " << nestedDirPath << std::endl;
}
return 0;
}
This code snippet creates both `parent_dir` and `child_dir` if they do not already exist. Proper handling of nested directories is critical for organizing files logically.

Alternative Ways to Create Directories
Using System Calls (For Compatibility)
If you are working with older C++ standards or need an alternative approach, you can use system calls. This method invokes shell commands to create directories but lacks the portability and safety of the `<filesystem>` approach.
Code Example
#include <cstdlib>
int main() {
system("mkdir new_directory"); // For UNIX/Linux
// system("md new_directory"); // For Windows
return 0;
}
This example uses the `system` function to execute the `mkdir` command in UNIX/Linux or `md` in Windows. While this is quick and simple, it may not handle errors as gracefully and can lead to platform dependencies.
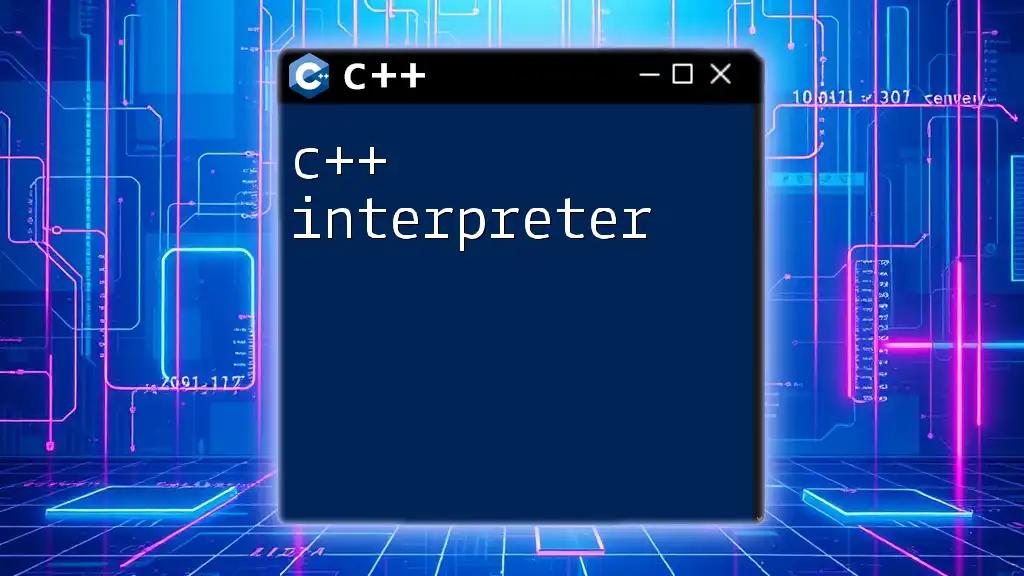
Best Practices for Directory Management
Checking Directory Existence Before Creation
It is good practice to check if a directory exists before attempting to create it. This prevents unnecessary errors and can improve performance.
Permissions and Security Compliance
When creating directories, consider the user's permissions. Ensuring that your application adheres to security guidelines when managing directories will help prevent unauthorized access and maintain system integrity.
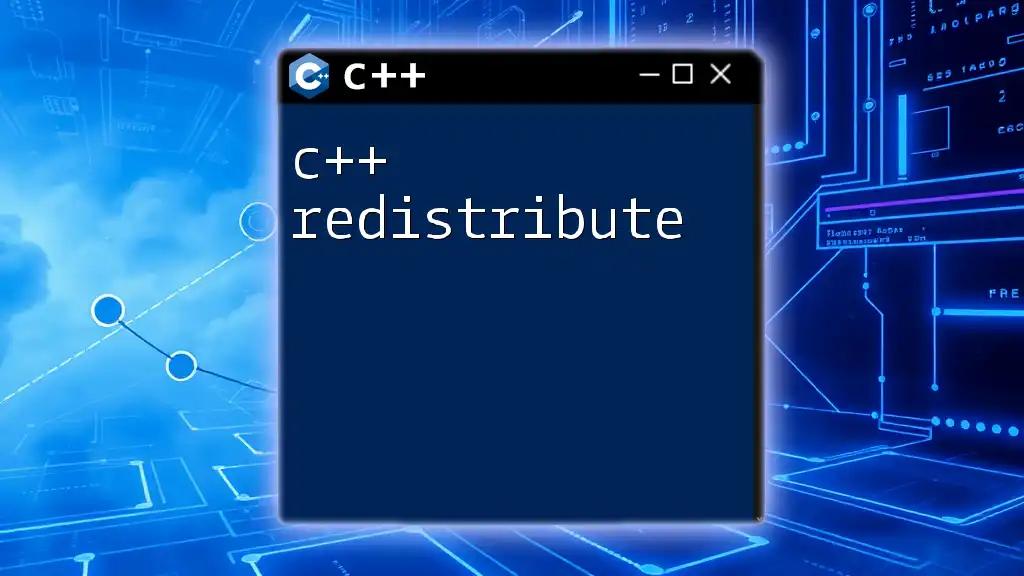
Conclusion
The ability to create and manage directories in C++ is a powerful tool that helps in structuring data and managing files effectively. By understanding the various methods available, including the modern `<filesystem>` library and alternative system calls, you can choose the best approach for your application needs. Explore this capability and experiment with your directory management today!
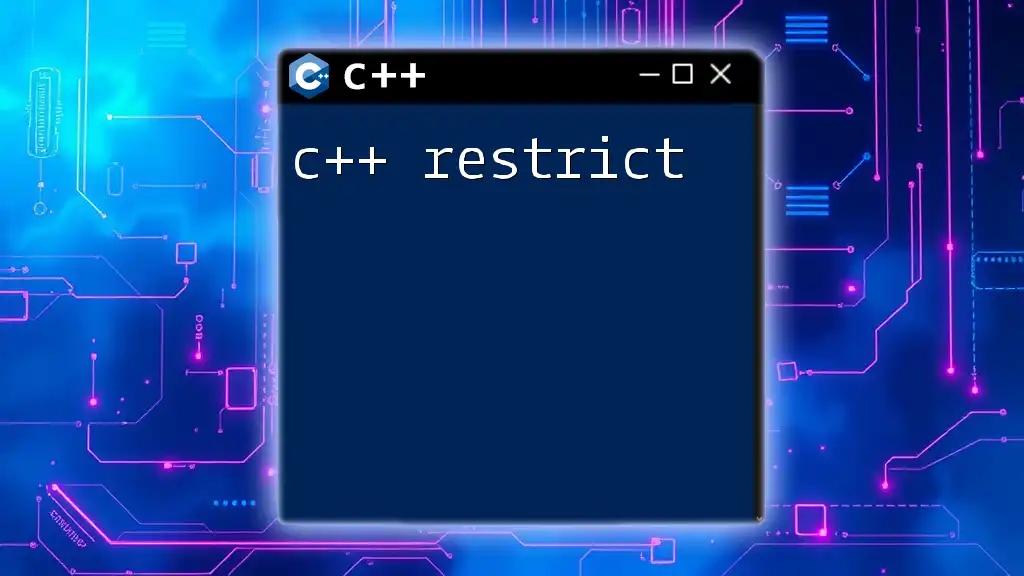
Call to Action
Join us for more in-depth tutorials and practical guides on C++. Let’s enhance your programming skills and build robust applications together!