To create a directory in C++ only if it does not already exist, you can use the following code snippet that utilizes the `<sys/stat.h>` header for checking the directory status and making the directory.
#include <sys/stat.h>
#include <iostream>
void createDirectoryIfNotExists(const std::string& dir) {
struct stat info;
if (stat(dir.c_str(), &info) != 0) { // Check if the directory exists
if (mkdir(dir.c_str(), 0777) != 0) // Create the directory
std::cerr << "Error creating directory: " << dir << std::endl;
} else if (info.st_mode & S_IFDIR) { // It's a directory
std::cout << "Directory already exists: " << dir << std::endl;
} else {
std::cerr << "Not a directory: " << dir << std::endl;
}
}
Understanding the Basics of C++ File System
What is the File System?
A file system is a method of organizing and storing files on a storage medium. It manages how data is stored and retrieved, allowing us to create a structured hierarchy where files reside in directories (or folders). In C++, working with file systems often involves manipulating files and directories, making tasks like creating and checking for the existence of directories essential.
C++ File System Library
Starting with C++17, the language introduced the `<filesystem>` header, empowering developers to interact with files and directories in a more intuitive way. This library includes a set of functions for handling file system paths, making it easier to create directories, check if they exist, and manage files.
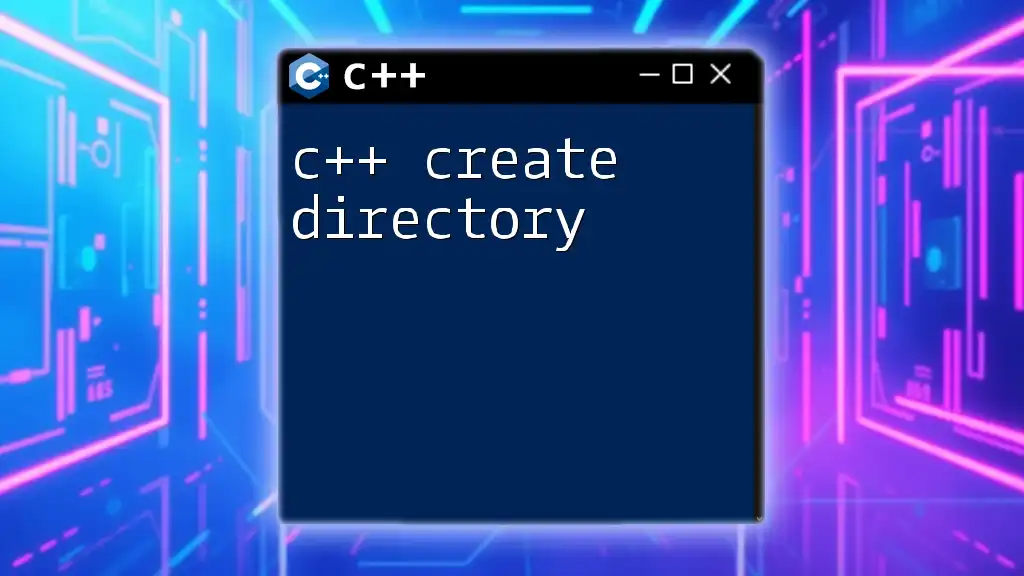
Reasons to Create Directories
Creating directories in C++ is crucial for various reasons:
-
Data Organization: Organizing files into directories helps maintain clarity within projects, especially as the number of files grows. Having structured folders can significantly enhance project organization.
-
Preventing Errors: By checking if a directory exists before attempting to create it, you avoid potential runtime errors, making your applications more robust and user-friendly.
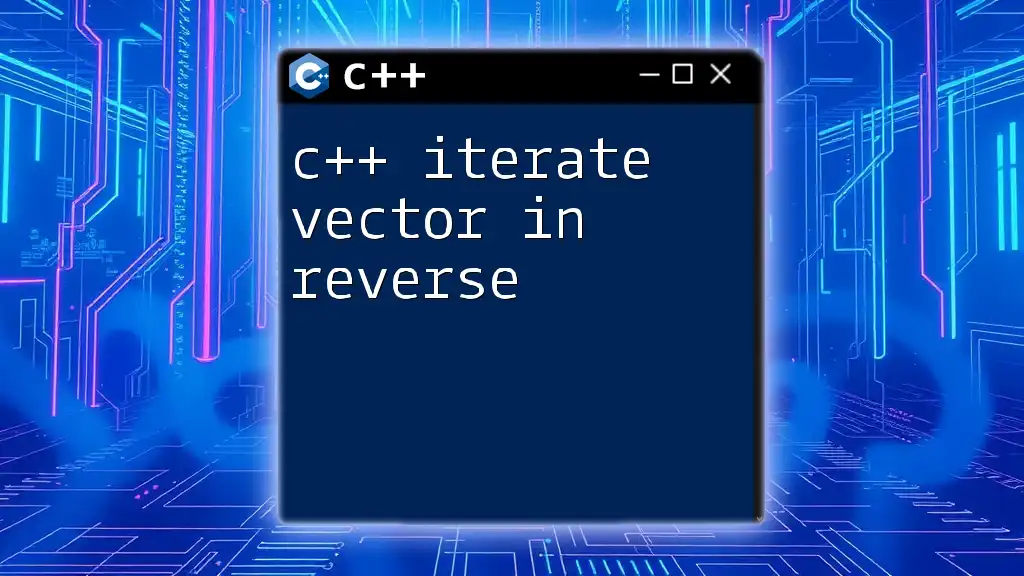
Using the C++ File System Library
Including the Necessary Headers
To utilize the features of the filesystem library, you need to include the appropriate headers. This sets the groundwork for any file system operation in C++.
#include <iostream>
#include <filesystem>
Namespace Considerations
The functionality of the `<filesystem>` library is encapsulated in the `std::filesystem` namespace. For convenience, you can create an alias to streamline your code. This makes it easier to call functions without having to repeatedly specify the full namespace.
namespace fs = std::filesystem;
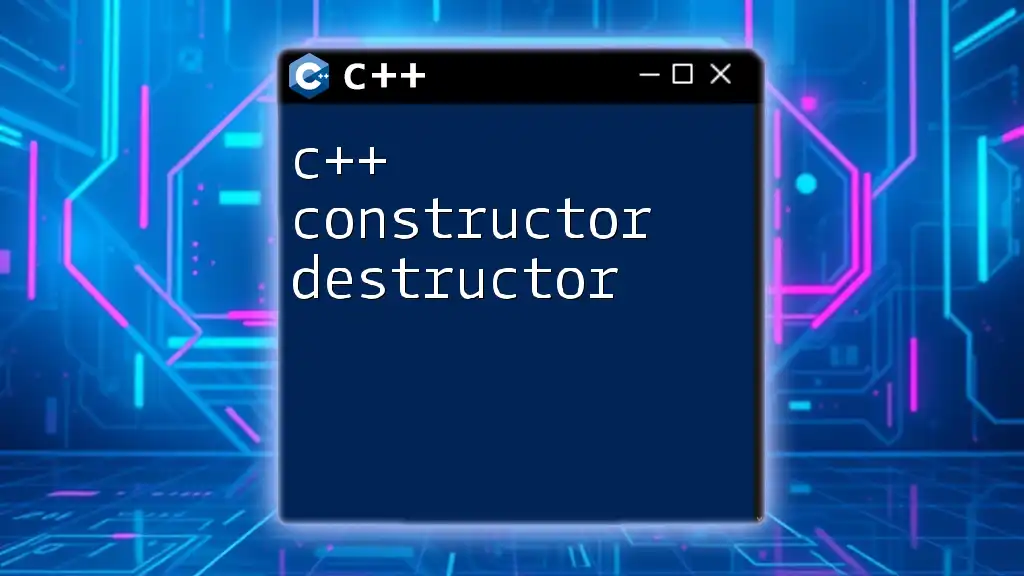
Checking if a Directory Exists
Function to Check Directory Existence
Before creating a directory, it's important to verify whether it already exists. This can be done using the `exists()` and `is_directory()` functions provided by the filesystem library.
bool directoryExists(const std::string& path) {
return fs::exists(path) && fs::is_directory(path);
}
Explanation
The `exists()` function checks if the specified path exists on the file system, while `is_directory()` verifies if that path is a directory. Both these functions work in tandem to confirm the existence and type of the specified path, ensuring that we only proceed to create a directory when it is genuinely necessary.
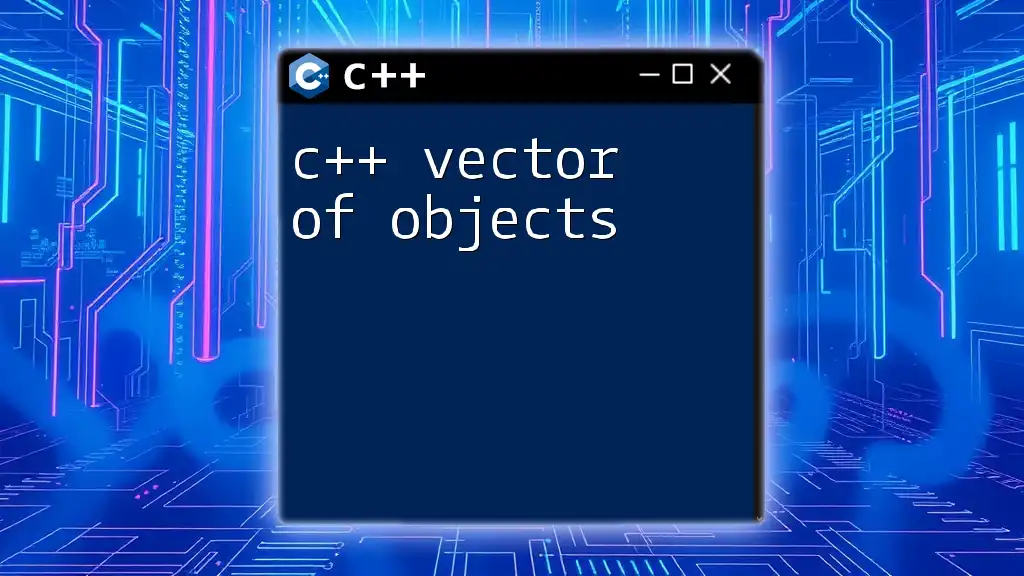
Creating the Directory if it Does Not Exist
Using the `create_directory()` Function
Once you've confirmed that the desired directory does not yet exist, you can proceed to create it using the `create_directory()` function. This is a straightforward method that successfully creates a directory at the specified path.
void createDirectoryIfNotExists(const std::string& path) {
if (!directoryExists(path)) {
fs::create_directory(path);
std::cout << "Directory created: " << path << std::endl;
} else {
std::cout << "Directory already exists: " << path << std::endl;
}
}
Complete Example
To illustrate the process, here's how it all fits together in a simple program. This example checks for the existence of a directory and creates it if it does not exist.
int main() {
std::string dirPath = "example_directory";
createDirectoryIfNotExists(dirPath);
return 0;
}
In this example, if you run the program, it will check for `example_directory`. If it isn't present, the program will create it and print a confirmation message to the console. If it already exists, you'll receive a message indicating this.
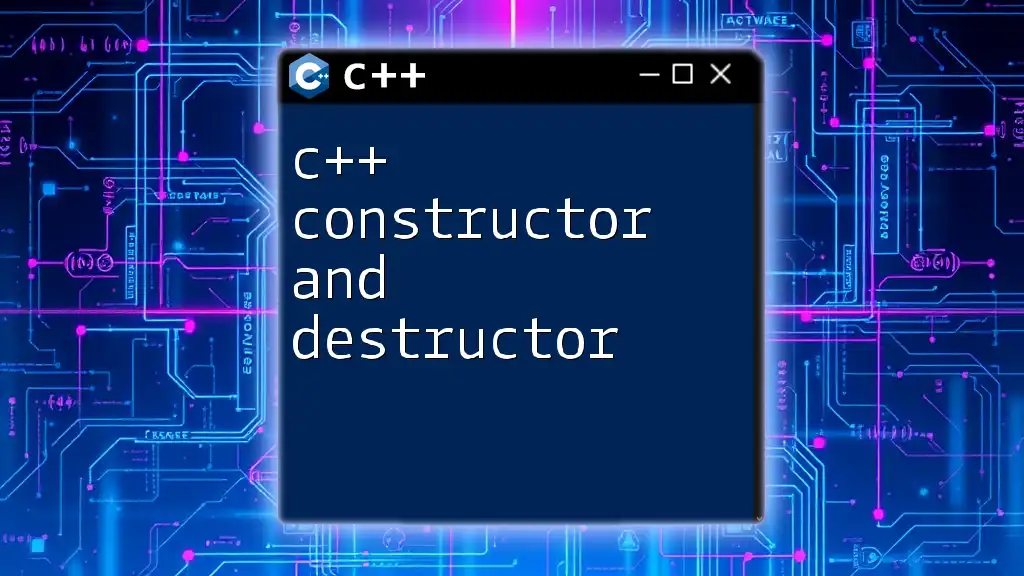
Error Handling and Best Practices
Handling Exceptions
When dealing with file systems, it is essential to anticipate and gracefully handle exceptions that may occur. Using try-catch blocks helps catch exceptions that arise from operations like creating directories.
try {
fs::create_directory(path);
} catch (const fs::filesystem_error& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
This approach not only prevents crashes but also provides the user with valuable feedback on what went wrong, which is crucial for debugging and maintaining a robust application.
Best Practices
When creating directories, consider the following best practices:
- Naming Conventions: Use clear and consistent naming conventions for your directories to avoid confusion and make navigation easier.
- Path Safety: Be cautious with file paths to ensure compatibility across different operating systems. Using relative paths rather than absolute paths can often enhance portability.
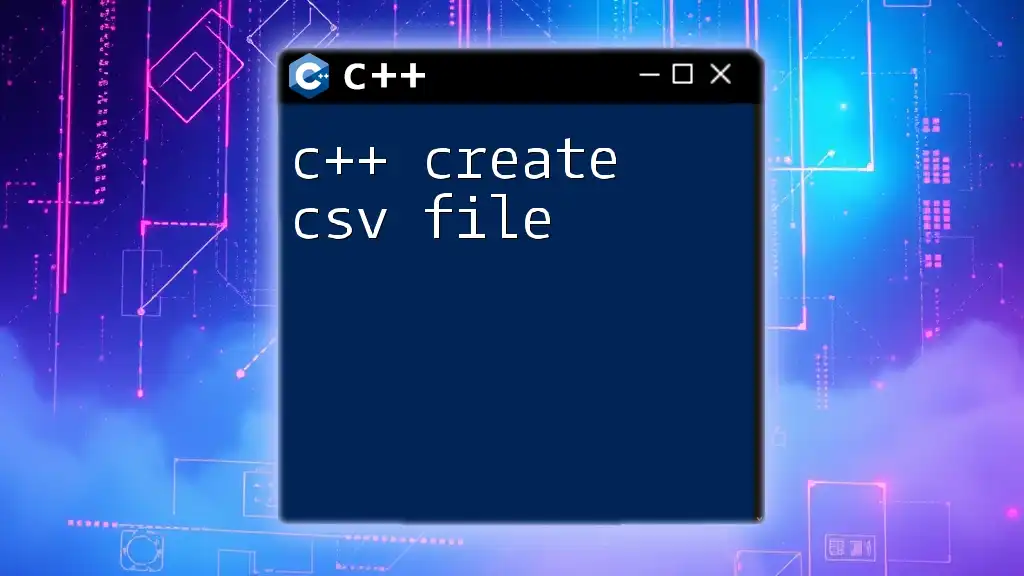
Conclusion
In summary, being able to c++ create directory if not exists is a fundamental aspect of effective file management within your application. Not only does it help in organizing your files better, but it also enhances the resilience of your application against runtime errors. Now that you've learned the basics of using the C++ file system library, you can implement these practices in your projects for cleaner, more efficient code.
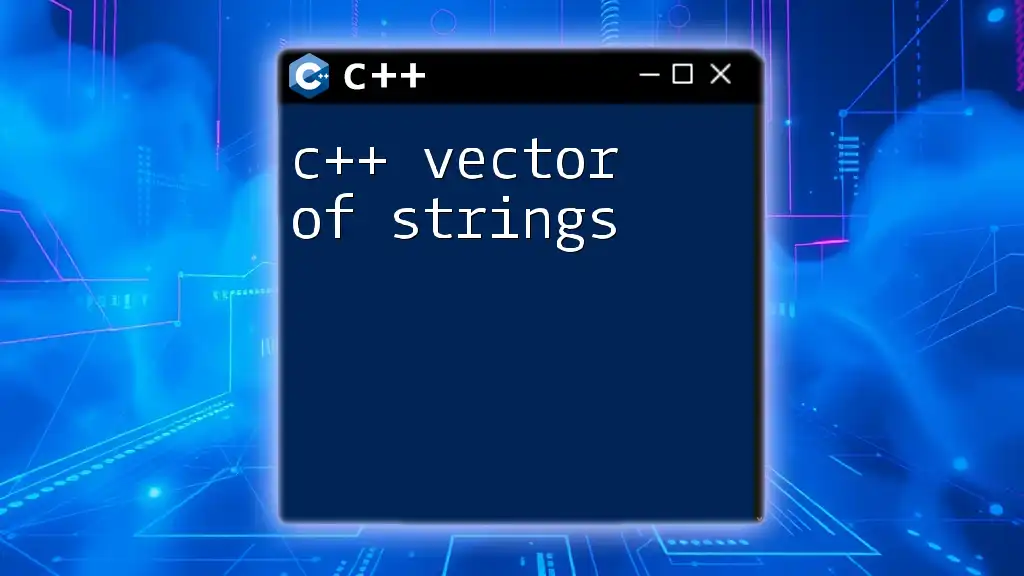
Additional Resources
For further reading and exploration, consider checking the official C++ documentation on the `<filesystem>` library to deepen your understanding of file operations and capabilities. Additionally, you might want to explore open-source projects that illustrate advanced usage of file system manipulation in C++.
Feel free to share your experiences or questions regarding directory management in C++, and stay tuned for more tips on mastering C++ programming!