To check if a string represents a number in C++, you can use the `std::all_of` function combined with `isdigit` from the `<cctype>` header.
#include <iostream>
#include <string>
#include <algorithm>
#include <cctype>
bool isNumber(const std::string& str) {
return !str.empty() && std::all_of(str.begin(), str.end(), ::isdigit);
}
int main() {
std::string input = "12345";
std::cout << (isNumber(input) ? "Is a number" : "Not a number") << std::endl;
return 0;
}
Understanding the Basics of Strings and Numbers in C++
Before diving into the methods to c++ check if string is number, it’s essential to understand the core concepts of strings and numbers in C++.
What are Strings in C++?
In C++, a string is a sequence of characters. Strings are widely used for representing textual data and are available through the C++ Standard Library with the `std::string` type. This data type provides multiple built-in functionalities for manipulation, making it easier for developers to work with text-based information.
Numeric Data Types in C++
C++ offers various numeric data types, such as int, float, and double. Each has its own range and precision, crucial for different programming scenarios. Recognizing whether a user input is a number allows you to ensure that calculations and data manipulations proceed without errors.
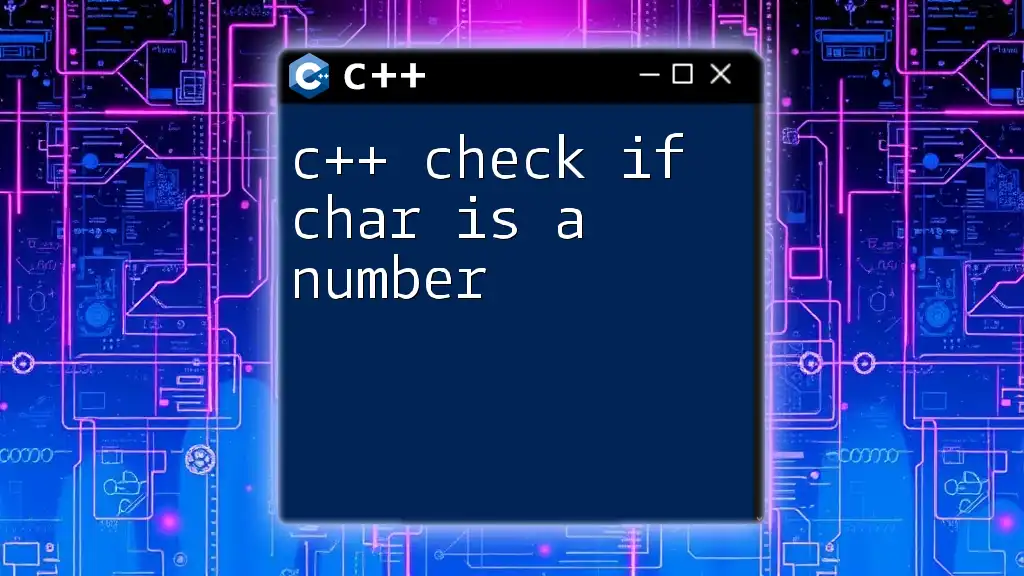
Why Check If a String is a Number?
Edge Cases in User Input
In practice, user inputs can often lead to exceptions and program failures if not properly validated. For instance, if your program expects a numeric input but receives a string with letters, an error will occur during parsing. Validating input is crucial to maintain robustness and improve user experience.
Applications in Data Processing
In fields like data analysis, finance, and scientific computing, parsing and validating numbers from strings is a common requirement. Errors in number identification can lead to incorrect results, making this check indispensable for accurate data processing.
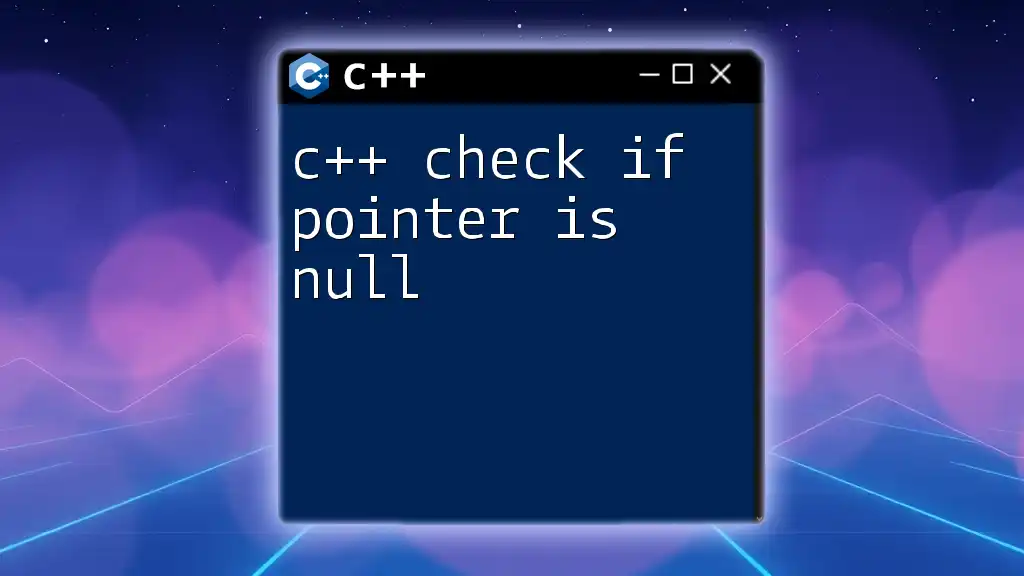
Methods to Check If String is a Number in C++
Using `std::isdigit`
What is `std::isdigit`?
`std::isdigit` is a function from the `<cctype>` library that checks if a character is a decimal digit (0-9). It is often used in loops to examine each character in a string.
Code Snippet Example:
#include <iostream>
#include <cctype>
#include <string>
bool isNumber(const std::string &str) {
for (char c : str) {
if (!std::isdigit(c)) return false; // Return false if any character is not a digit
}
return true; // Return true if all characters are digits
}
int main() {
std::string input = "12345";
std::cout << isNumber(input); // Will output 1 (true)
}
This method is efficient for whole numbers without any signs or decimal points. However, it does not handle negative numbers or decimal values.
Using `std::stringstream`
What is `std::stringstream`?
`std::stringstream` allows you to read from and write to strings as if they were streams. It excels at converting strings to various types, making it suitable for checking if a string can be interpreted as a number.
Code Snippet Example:
#include <iostream>
#include <sstream>
#include <string>
bool isNumber(const std::string &str) {
std::stringstream ss(str);
double d; // Create a variable to hold the converted double
char c; // Create a character variable to check for leftover characters
return !(ss >> d).fail() && !(ss >> c); // Check if conversion to double succeeded and no leftover characters
}
int main() {
std::string input = "123.45";
std::cout << isNumber(input); // Will output 1 (true)
}
This method accommodates both integers and floating-point values. However, it requires that the entire string is convertible to a number; otherwise, it returns false.
Using Regular Expressions
Introduction to Regular Expressions in C++
Regular expressions (regex) are patterns used to match character combinations in strings. They offer a powerful way to validate string formats, making them a great option for checking if a string is a number.
Code Snippet Example:
#include <iostream>
#include <regex>
#include <string>
bool isNumber(const std::string &str) {
std::regex e("^-?\\d+(\\.\\d+)?$"); // Regular expression pattern for both integers and decimals, allowing optional negative sign
return std::regex_match(str, e); // Match the entire string against the regex
}
int main() {
std::string input = "-123.45";
std::cout << isNumber(input); // Will output 1 (true)
}
Regular expressions provide flexibility, allowing you to include patterns for negatives, decimals, etc. However, they can be slower than other methods for simple checks.
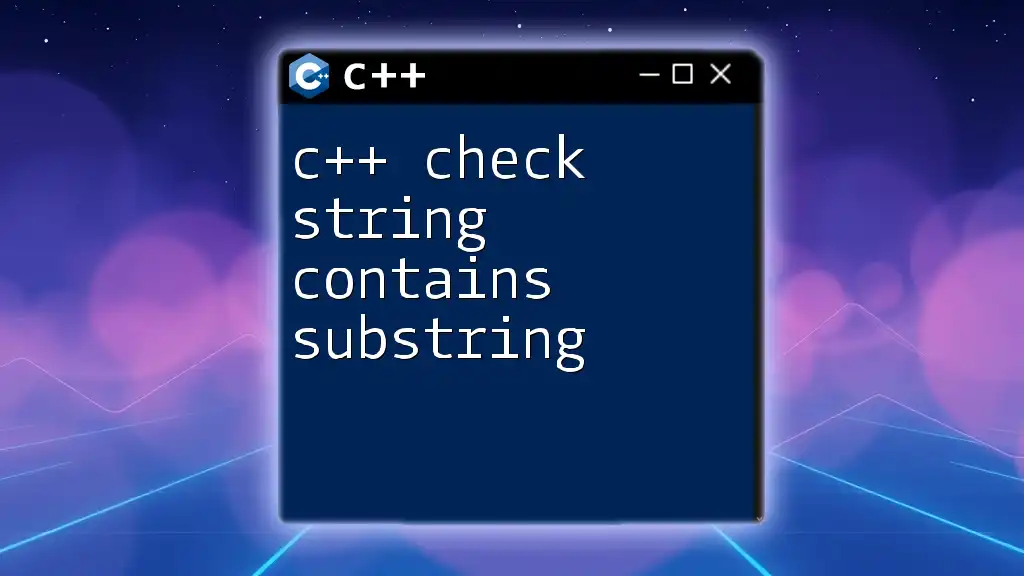
Performance Considerations
When considering performance, it is crucial to choose the right method based on your needs:
- Using `std::isdigit` can be fast for whole numbers but does not accommodate any other format.
- `std::stringstream` is versatile and handles different numeric types but may introduce overhead in performance.
- Regular expressions, while powerful, may be less efficient compared to other methods, particularly for large datasets or frequent validations.
Choosing the right method depends on the specific context of your application. For instance, if your primary input is a whole number, `std::isdigit` is straightforward. If you need to handle multiple numeric formats, regex or `std::stringstream` may be the better options.
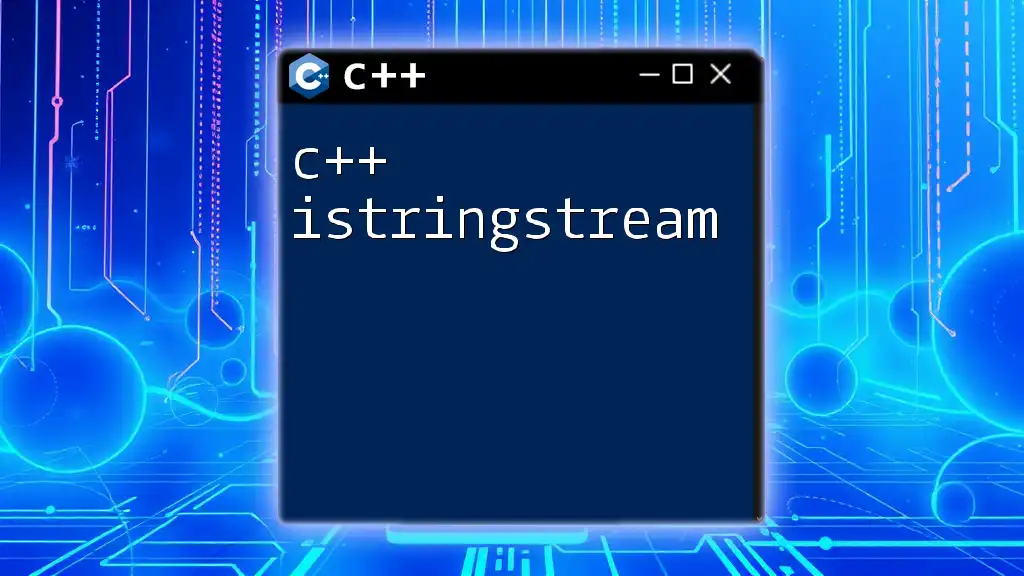
Conclusion
In summary, there are multiple ways to c++ check if string is number. By understanding the differences among methods like `std::isdigit`, `std::stringstream`, and regular expressions, you can select the most suitable approach for your application’s requirements.
Implementing these methods will not only make your code more robust but also improve performance and user trust. As you work with strings and numbers in C++, integrating proper validation checks will pave the way for writing reliable and maintainable code.
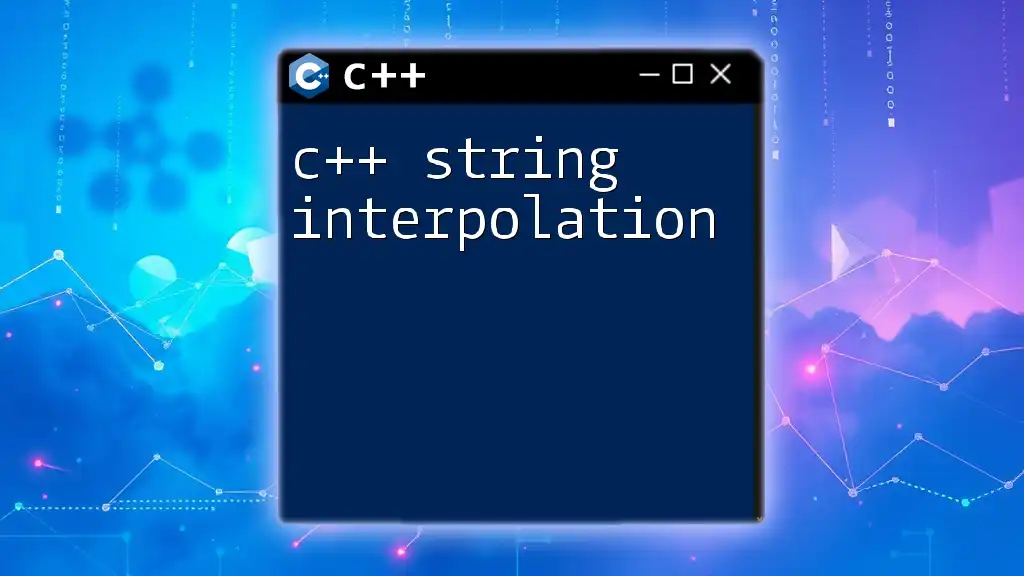
Additional Resources
For further reading, consider exploring the C++ Standard Library documentation for `std::string`, `std::stringstream`, and regular expressions. Each of these areas holds valuable details that can enhance your overall understanding of handling data in C++.
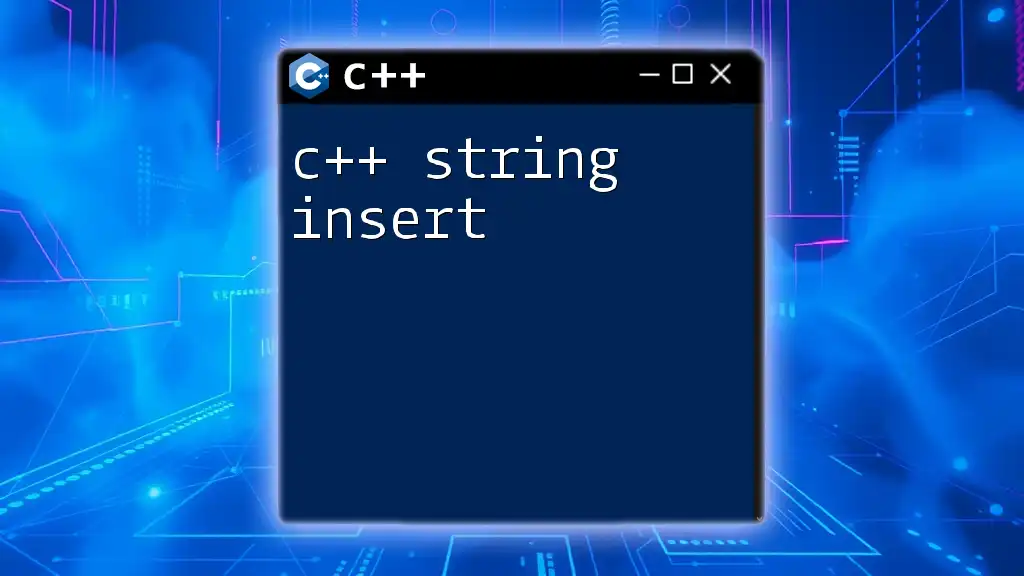
FAQs
-
Can I use `std::stoi` instead of these methods?
While `std::stoi` is useful for converting strings to integers, it does not inherently check if the string is valid. It will throw an exception if the conversion fails. -
How can I handle exceptions for invalid input?
Using `try-catch` blocks around your conversion functions can help you gracefully manage exceptions related to invalid input. -
Can I check for formatted numbers (e.g., currency)?
Yes, but you may need to use regular expressions or custom string parsing to account for specific formats, such as currency symbols.