In C++, you can split a string by a specific character using the `std::stringstream` class along with the `getline` function, as shown in the example below:
#include <iostream>
#include <sstream>
#include <vector>
std::vector<std::string> splitString(const std::string &str, char delimiter) {
std::vector<std::string> tokens;
std::stringstream ss(str);
std::string token;
while (std::getline(ss, token, delimiter)) {
tokens.push_back(token);
}
return tokens;
}
int main() {
std::string input = "hello,world,this,is,c++";
std::vector<std::string> result = splitString(input, ',');
for (const auto &s : result) {
std::cout << s << std::endl;
}
return 0;
}
Understanding Strings in C++
Overview of C++ Strings
In C++, strings are handled using the `std::string` class provided by the Standard Library. This class offers a flexible and powerful way to work with text. Unlike C-style strings (character arrays), which require manual handling for memory management and string manipulation, `std::string` abstracts these complexities, allowing developers to focus on functionality rather than implementation details.
Why Split Strings?
The ability to split strings is fundamental in programming. It plays a vital role in various scenarios, from parsing data formats (like CSV files) to breaking down user-inputted strings for processing. When you need to extract meaningful components from a string, such as tokens or keywords, splitting becomes essential.
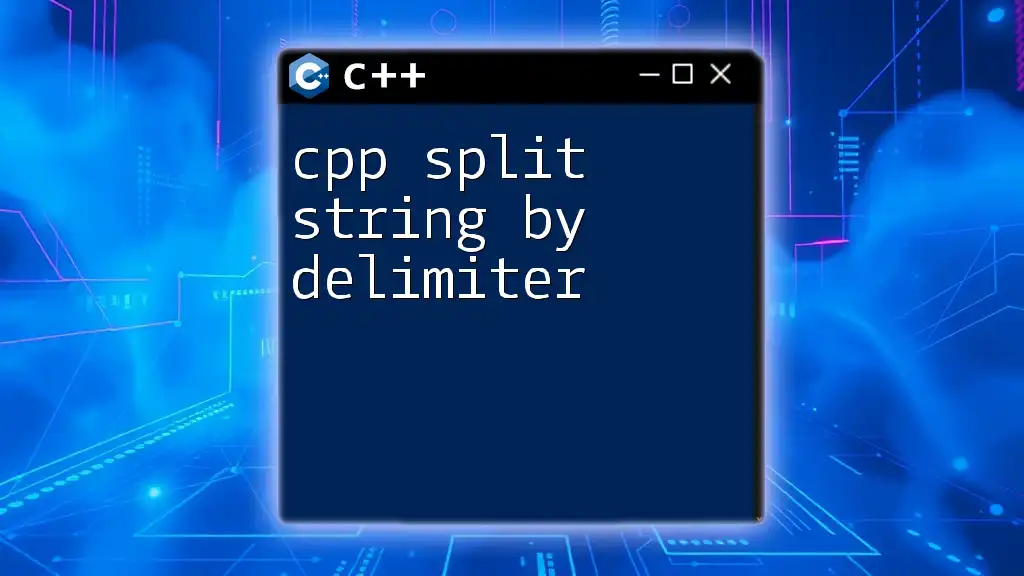
The Concept of Splitting Strings in C++
What Does it Mean to Split a String?
Splitting a string involves breaking it into substrings based on specified delimiters—characters that indicate where a split should occur. Common delimiters include commas, spaces, and semicolons. Understanding how to manipulate strings in this manner can make data handling much more effective.
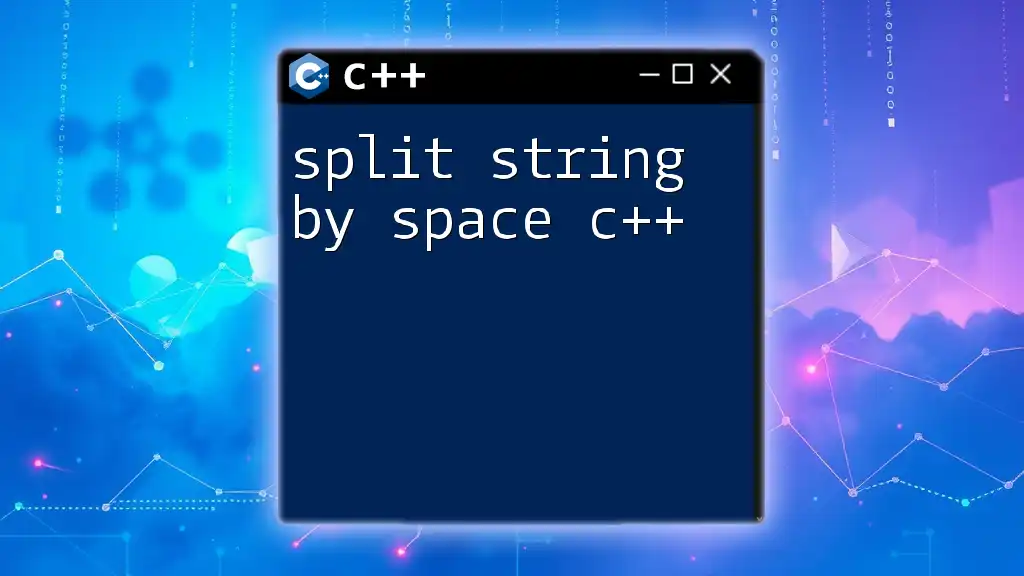
Different Methods to Split Strings in C++
Using Standard Library Functions
Using `std::istringstream` and `std::getline`
One of the simplest ways to split strings in C++ is by using `std::istringstream` along with `std::getline`. This approach leverages the power of stream operations to read segments of the string efficiently.
Here’s an example of how to implement this:
#include <iostream>
#include <sstream>
#include <vector>
std::vector<std::string> splitString(const std::string &input, char delimiter) {
std::istringstream iss(input);
std::string item;
std::vector<std::string> tokens;
while (std::getline(iss, item, delimiter)) {
tokens.push_back(item);
}
return tokens;
}
int main() {
std::string str = "apple,banana,cherry";
char delimiter = ',';
std::vector<std::string> result = splitString(str, delimiter);
for (const auto &token : result) {
std::cout << token << std::endl;
}
return 0;
}
In this example, `std::getline` reads from the input string until it encounters the specified delimiter, adding each segment to the resulting vector. This method is efficient and keeps your code clean and readable.
Using `std::string::find` and `std::string::substr`
Another method to split a string manually involves using `std::string::find` in conjunction with `std::string::substr`. This technique gives you more control, allowing for custom splitting logic.
Here’s how you can implement it:
#include <iostream>
#include <vector>
#include <string>
std::vector<std::string> splitString(const std::string &input, char delimiter) {
std::vector<std::string> tokens;
size_t start = 0, end = 0;
while ((end = input.find(delimiter, start)) != std::string::npos) {
tokens.push_back(input.substr(start, end - start));
start = end + 1;
}
tokens.push_back(input.substr(start));
return tokens;
}
In this code, `find` locates the delimiter's position in the string. The `substr` method then extracts the portion of the string from the last start position to the found delimiter, appending each token to the vector.
Custom Split Function Example
Writing a Flexible Split Function
Creating a flexible split function can enhance your string manipulation capabilities. By allowing multiple delimiters, you can accommodate various data formats.
For example:
#include <iostream>
#include <vector>
#include <string>
std::vector<std::string> splitString(const std::string &input, const std::string &delimiters) {
std::vector<std::string> tokens;
size_t start = 0, end = 0;
while ((end = input.find_first_of(delimiters, start)) != std::string::npos) {
if (end > start) {
tokens.push_back(input.substr(start, end - start));
}
start = end + 1;
}
tokens.push_back(input.substr(start));
return tokens;
}
This function utilizes `find_first_of`, allowing a string of delimiters. This adds versatility, making it suitable for more complex string formats and parsing scenarios.
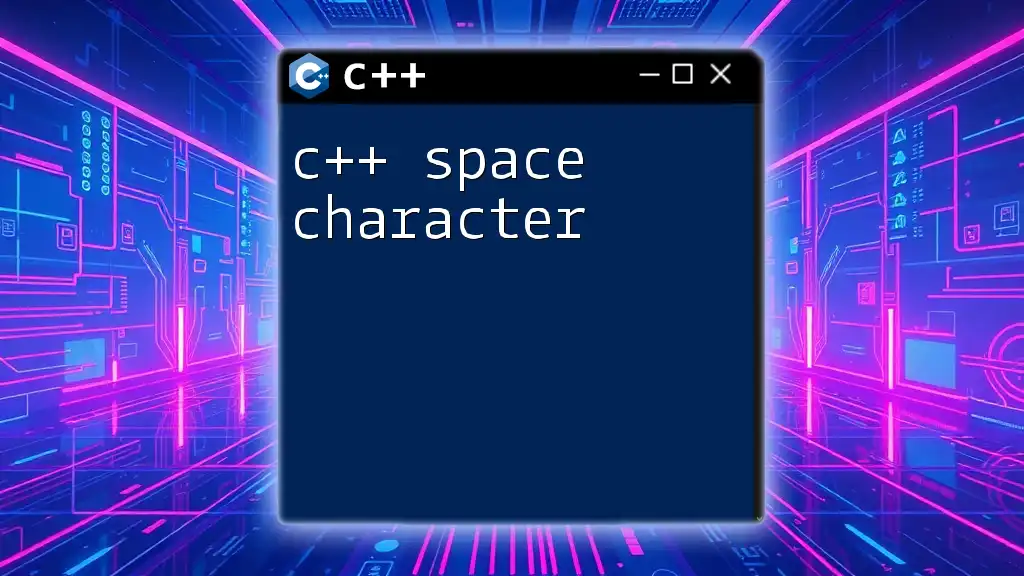
Performance Considerations
Comparing Approaches: Efficiency and Usability
When choosing between using standard library functions and crafting a custom implementation, consider the following factors:
-
Standard Library Functions: These are typically optimized and well-tested, ensuring robustness and reliability. They also make your code easier to read, which can be a significant advantage in collaborative environments.
-
Custom Implementations: While potentially more flexible, a manually crafted solution might sacrifice some performance and readability. However, they can be valuable for specific needs where more control is required.
Ultimately, your choice should be informed by your project's requirements and the specific scenarios you intend to handle.
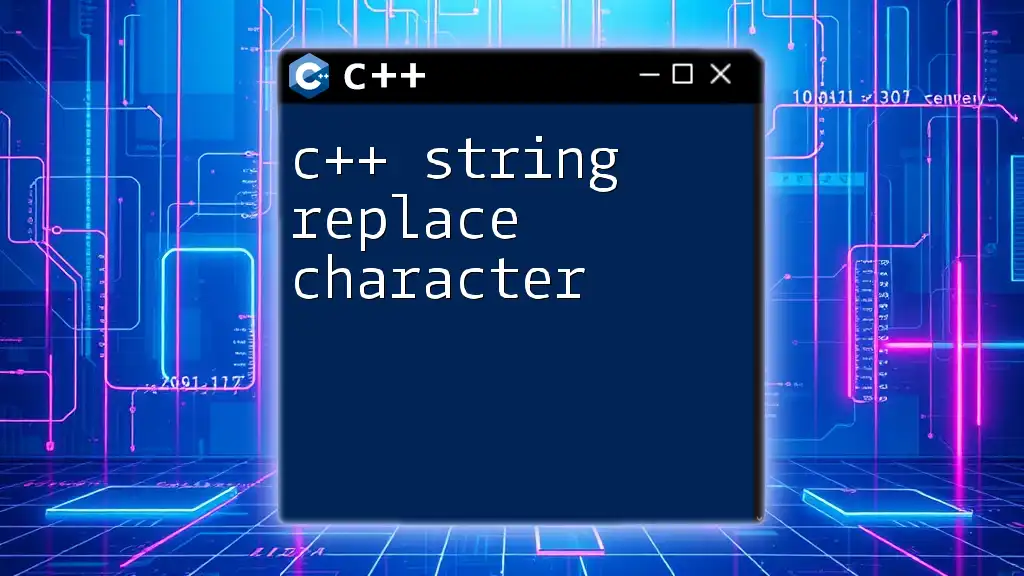
Real-World Use Cases
Practical Applications of Splitting Strings
Splitting strings finds applications across many domains:
-
Data Processing: In applications that handle data import, such as CSV readers, splitting strings is crucial for correctly capturing and processing each field.
-
Configuration Parsing: Many applications read configuration files where settings are specified in a delimited format. Splitting these strings provides a way to easily access and manipulate various parameters.
-
Log Analysis: Analyzing log files often involves extracting relevant tokens for each log entry, helping in identifying issues or patterns.
Each of these scenarios can leverage the techniques discussed, illustrating the importance of mastering string manipulation in C++.
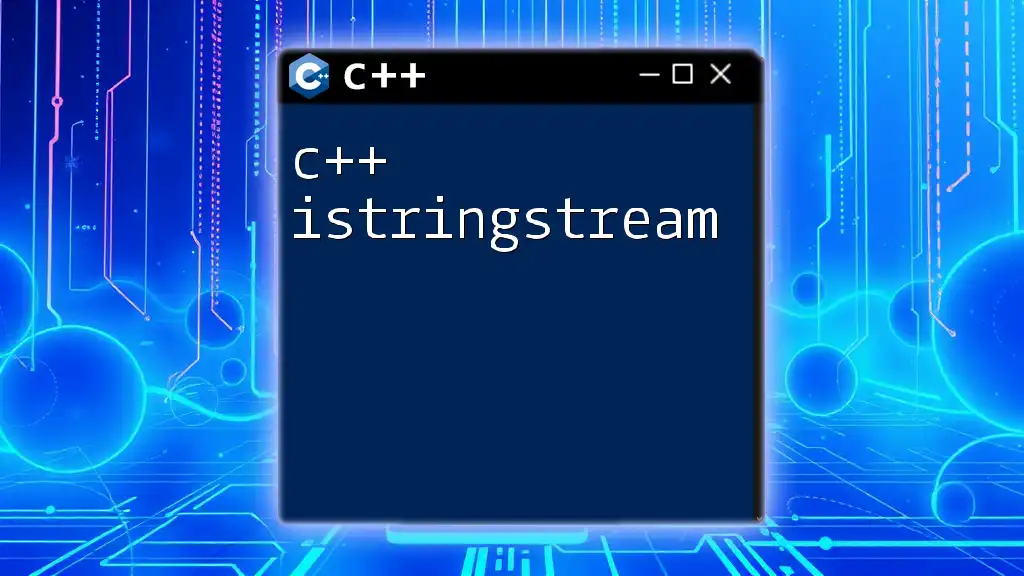
Conclusion
Understanding how to c++ split string by character is an essential skill for any C++ programmer. From using standard library functions to implementing custom solutions, the approaches laid out in this guide provide a comprehensive toolkit for string manipulation.
As you continue to practice and explore, don't hesitate to experiment with these methods in your projects. Each implementation enhances your ability to manage text data effectively, making you a more proficient developer. Keep learning, and stay tuned for more concise C++ tutorials and tips that will help you on your programming journey!
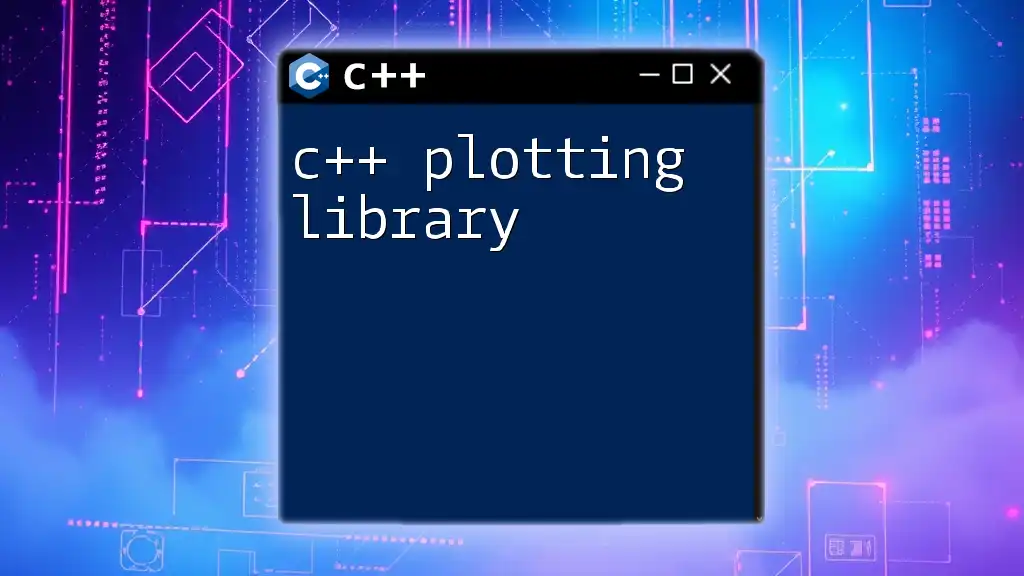
Additional Resources
To further enrich your understanding, consider exploring the official C++ documentation for in-depth insights, recommended programming books, and online courses tailored for advancing your C++ skills. Engage with communities and forums where you can share insights and learn from diverse experiences in string manipulation and beyond.