In C++, you can split a string by spaces using the `std::istringstream` class along with the `std::getline` function, which allows you to extract words from the string into a vector.
Here's a code snippet demonstrating this:
#include <iostream>
#include <sstream>
#include <vector>
int main() {
std::string str = "Split this string by spaces";
std::istringstream iss(str);
std::vector<std::string> words;
std::string word;
while (iss >> word) {
words.push_back(word);
}
for (const auto& w : words) {
std::cout << w << std::endl;
}
return 0;
}
Understanding Strings in C++
In C++, the string data type is represented by the `std::string` class, which provides a flexible and efficient way to manage sequences of characters. Unlike C-style strings which are terminated with a null character (`'\0'`), `std::string` automatically handles memory allocation, making string handling easier and less error-prone.
Using `std::string` over traditional character arrays offers several benefits:
- Dynamic Size: You can easily modify the size of a string without worrying about buffer overflow.
- Integrated Methods: `std::string` includes various helper methods that simplify common tasks, such as concatenation, substring extraction, and replacement.

Why Split Strings?
Splitting a string by space is a common requirement in many programming applications. Whether you are parsing user input, reading data files, or processing commands, being able to break a string into its constituent parts allows for more manageable data handling.
Real-life examples of when you might need to split strings include:
- User Input Parsing: When accepting input commands, it's essential to extract different arguments from a single input line.
- Data Parsing: In applications that process log files or CSV data, splitting strings can help isolate relevant information from larger datasets.

Methods to Split a String by Space in C++
Using `std::istringstream`
One of the most straightforward methods to split a string by space in C++ is by using the `std::istringstream` class from the `<sstream>` library. `std::istringstream` allows you to treat strings as input streams, thus giving you the flexibility to read formatted input.
Here’s a code example demonstrating how to use `std::istringstream` to split a string:
#include <iostream>
#include <sstream>
#include <vector>
std::vector<std::string> splitString(const std::string& str) {
std::istringstream iss(str);
std::vector<std::string> tokens;
std::string token;
while (iss >> token) {
tokens.push_back(token);
}
return tokens;
}
In this example, `iss >> token` reads each word from the input string separated by spaces and stores it in the `tokens` vector. This method effectively handles varying lengths of whitespace without additional code.
Using `std::getline` with a Stringstream
Another effective approach is to use `std::getline` in combination with a `std::stringstream`. This method allows you to specify the delimiter directly, which can be useful if you want to customize string splitting in the future.
Here’s how you can implement this:
#include <iostream>
#include <sstream>
#include <vector>
std::vector<std::string> splitString(const std::string &str) {
std::vector<std::string> tokens;
std::stringstream ss(str);
std::string token;
while (std::getline(ss, token, ' ')) {
tokens.push_back(token);
}
return tokens;
}
Here, the `std::getline(ss, token, ' ')` method fetches each token from the stream separated by spaces. It's an intuitive method that performs well in typical scenarios where spaces are the delimiter.
Using `std::string` find and substr
For those looking for a more manual approach or who might need advanced customization, using `std::string` functions like `find` and `substr` can be beneficial. This method offers the most control over the string-splitting process.
As an example, consider the following code:
#include <iostream>
#include <string>
#include <vector>
std::vector<std::string> splitString(const std::string &str) {
std::vector<std::string> tokens;
size_t start = 0, end = 0;
while ((end = str.find(' ', start)) != std::string::npos) {
tokens.push_back(str.substr(start, end - start));
start = end + 1;
}
tokens.push_back(str.substr(start));
return tokens;
}
This implementation uses `find` to locate the spaces and `substr` to extract tokens between them. It allows you to easily manage the logic behind string splitting, which can be particularly advantageous in complex applications.

Handling Edge Cases
Empty Strings
When dealing with user input or data processing, it's crucial to account for empty strings. Each of the previously mentioned methods will naturally return an empty vector if the input is an empty string, which is the expected behavior.
Multiple Spaces
In cases where the string contains multiple consecutive spaces, the `std::istringstream` and `std::getline` methods will skip empty tokens, making them robust for such scenarios. However, if you use `find` and `substr`, you may need additional logic to handle these cases effectively.
Leading and Trailing Spaces
It’s also wise to trim leading and trailing spaces before splitting the string. While the aforementioned methods will still work without modifications, ensuring a clean input can enhance performance and accuracy, particularly in user-facing applications.
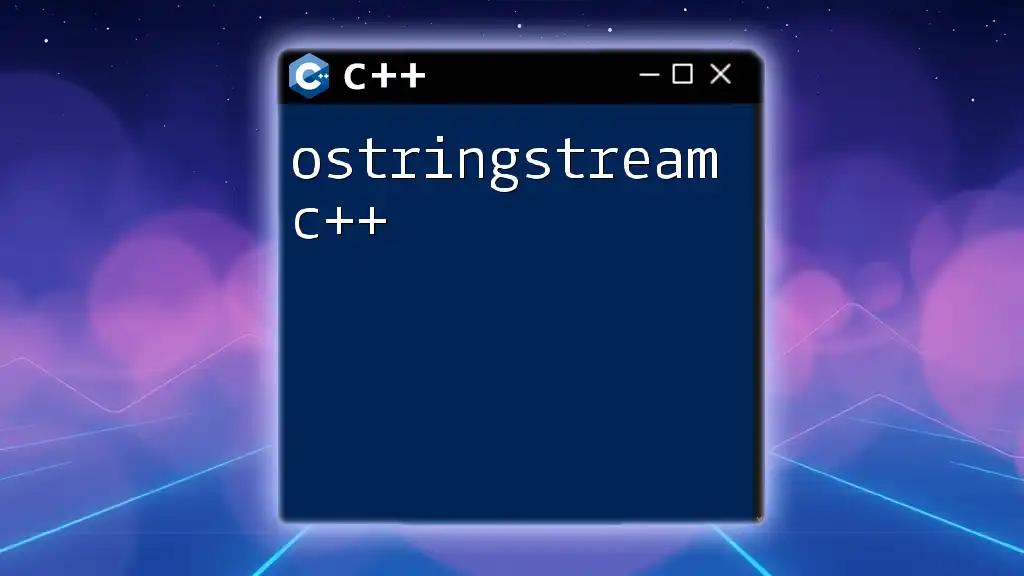
Performance Considerations
The choice of method for splitting strings can significantly influence performance, especially in scenarios dealing with large datasets or high-frequency calls. While `std::istringstream` and `std::getline` are generally more performant for simple use cases, the `find` and `substr` approach may be more appropriate for complex and customizable string manipulations.
When working with performance-sensitive applications, always benchmark these methods to determine which one fulfills your needs best.
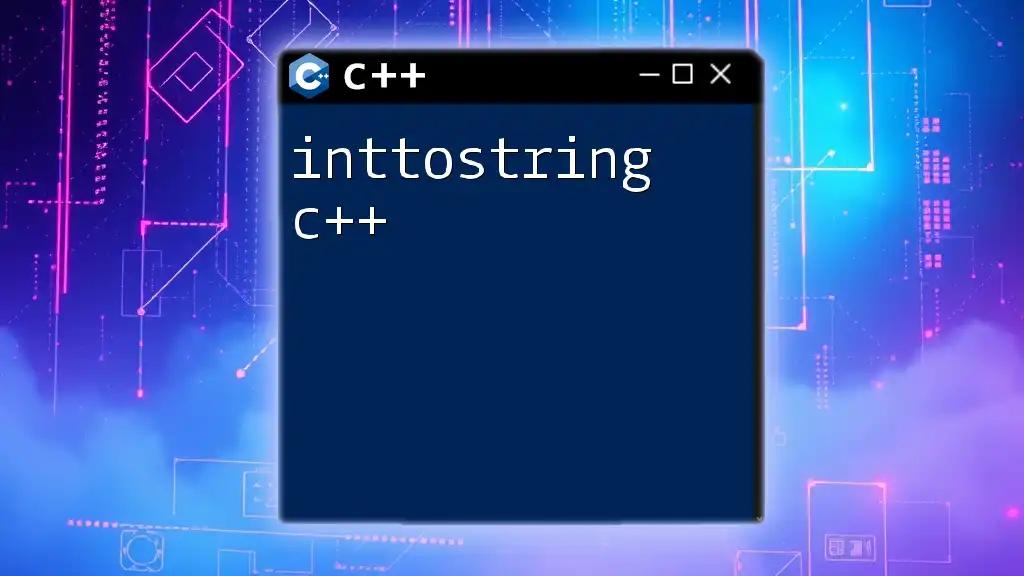
Practical Applications
User Input Parsing
In command-line applications, efficiently splitting strings can enhance your parsing logic, allowing you to extract arguments quickly and accurately. For example, if a user types a command followed by options, you can easily separate them into their respective components.
Data Processing
Splitting strings finds extensive use in data processing. For instance, when reading a log file, splitting each line by spaces can help isolate timestamps, log levels, and messages quickly, making it easier to analyze logs for errors or specific events.

Conclusion
In C++, efficiently splitting strings by space is an essential skill that can significantly improve your data handling capabilities. By understanding the different methods available such as using `std::istringstream`, `std::getline`, and the manual `find` and `substr` approach, you can choose the best technique for your particular needs. Practicing these string manipulation techniques will not only enhance your coding skills but also prepare you for real-world programming challenges.

Further Resources
For those interested in deepening their knowledge of C++ string manipulations, consider exploring a variety of resources, including books, online courses, and tutorials. They can offer additional insights and complex use cases to enrich your understanding of string processing in C++.