In C++, you can print a map by iterating through its elements using a `for` loop to access both the keys and values. Here's a simple example:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap = {{"apple", 1}, {"banana", 2}, {"orange", 3}};
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
What is a Map in C++?
A map in C++ is a part of the Standard Template Library (STL) that stores elements in key-value pairs. Each key is unique, and it is associated with one specific value. This data structure provides fast retrieval of data based on the key, making it an attractive option for various programming needs.
Comparison with other data structures reveals that maps are particularly useful when you need to associate a unique identifier (key) with a specific value and efficiently look it up, unlike vectors or arrays, which use a sequential index.
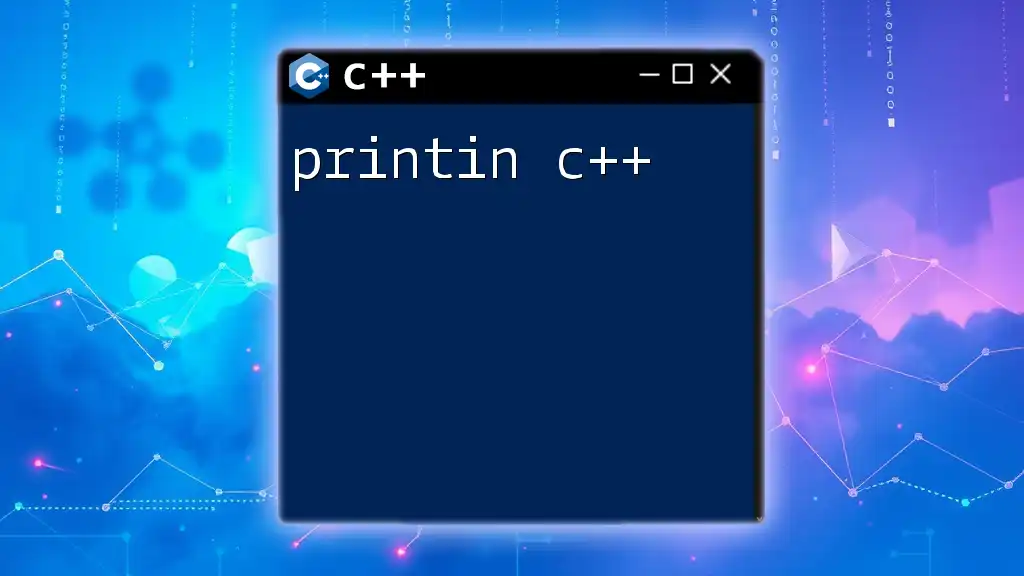
Importance of Printing a Map
Printing a map is a crucial skill for any C++ programmer. It serves multiple purposes:
- Debugging: Easily inspect the contents of a map to ensure it holds the expected data.
- Data Visualization: Presenting the data in a human-readable format can help understand the relationships between keys and values.
- Development: During the development phase, frequent map printing can reveal potential issues and guide adjustments.
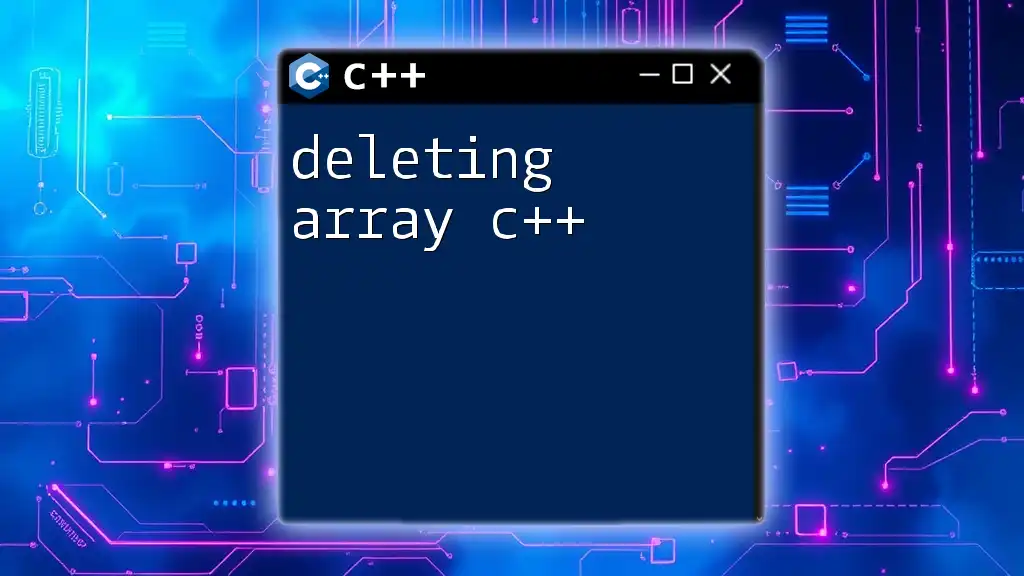
How to Create a Map in C++
To get started with map printing, you must first know how to create a map in C++.
Declaring and Initializing a Map
You can declare a map using the `std::map` declaration. Here's the typical syntax for creating a map:
#include <iostream>
#include <map>
using namespace std;
int main() {
map<string, int> studentGrades;
studentGrades["Alice"] = 90;
studentGrades["Bob"] = 85;
// Printing the map can be done here or in other functions
return 0;
}
In this example, we create a map named `studentGrades` where student names (as `string` types) are the keys, and their respective grades (as `int` types) are the values.
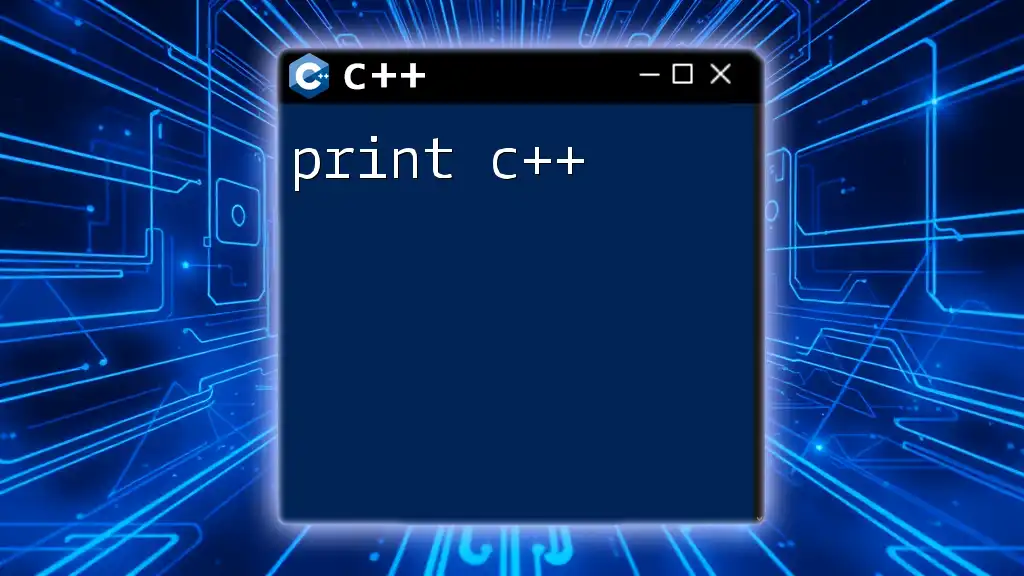
Different Ways to Print a Map in C++
Once you've created a map, the next logical step is knowing how to effectively print it.
Using a Simple Loop
One of the simplest methods of printing a map in C++ is through a standard loop.
Explanation of the Iteration Process
You can use either an iterator or a range-based for loop. However, the range-based for loop provides a cleaner and more concise syntax.
Example: Printing a Map with a Simple Loop
Here's how to print the contents of the map using a range-based for loop:
for (const auto& pair : studentGrades) {
cout << pair.first << ": " << pair.second << endl;
}
When you run this code, the output will be:
Alice: 90
Bob: 85
Using `for_each` and Lambda Functions
Another modern approach for printing a map in C++ is to utilize the `std::for_each` function in conjunction with lambda functions.
What is `for_each`?
`std::for_each` applies a function to a range of elements. This allows for elegant formatting while printing.
Example: Printing a Map with `for_each`
Here's an example of how to implement this method:
#include <algorithm>
for_each(studentGrades.begin(), studentGrades.end(), [](const pair<string, int>& p) {
cout << p.first << ": " << p.second << endl;
});
This method promotes better readability and a more functional programming style.
Custom Formatting with Print Functions
Sometimes, clarity is key in your output. Creating a custom print function helps manage that.
Creating a Custom Print Function
A custom print function allows you to maintain consistency while providing enhanced formatting options.
Example: Custom Print Function
void printMap(const map<string, int>& myMap) {
for (const auto& pair : myMap) {
cout << "Student: " << pair.first << " - Grade: " << pair.second << endl;
}
}
How to Call the Function
You can call this function from your main function like so:
int main() {
// Create the map and populate it
printMap(studentGrades);
return 0;
}
This implementation ensures that the output is structured neatly:
Student: Alice - Grade: 90
Student: Bob - Grade: 85
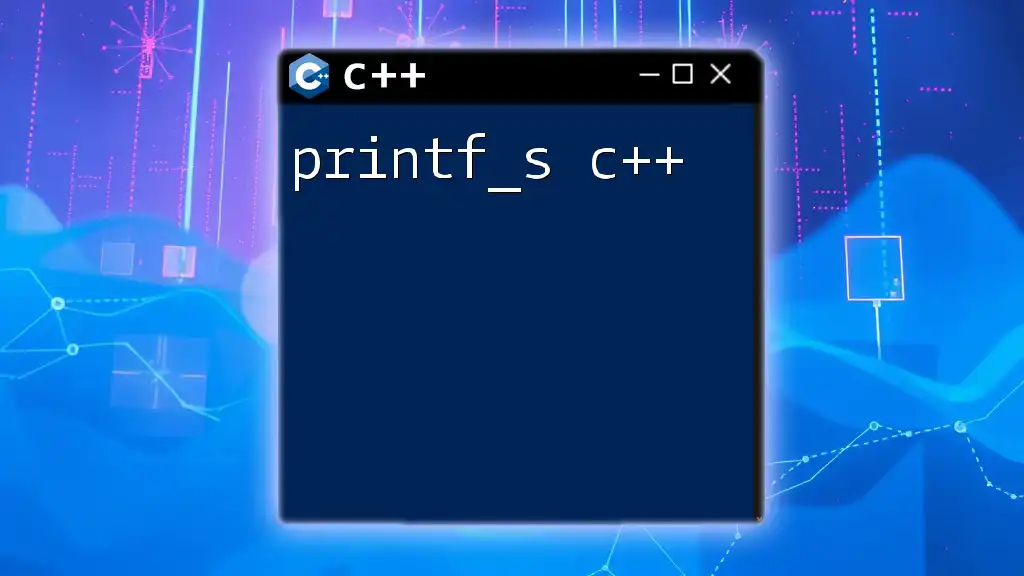
Special Cases in Map Printing
Printing Nested Maps
Nested maps are maps that contain other maps as values. They are useful when you want to categorize your data further.
What Are Nested Maps?
For instance, consider a map where each subject (key) points to another map of students and their grades (values).
Example: Printing a Nested Map
Here's how you can print a nested map:
map<string, map<string, int>> nestedMap = { {"Math", {{"Alice", 90}, {"Bob", 85}}} };
for (const auto& outerPair : nestedMap) {
cout << outerPair.first << ":\n";
for (const auto& innerPair : outerPair.second) {
cout << " " << innerPair.first << ": " << innerPair.second << endl;
}
}
The output will show:
Math:
Alice: 90
Bob: 85
Printing Maps with Complex Data Types
Handling complex data types can also appear challenging but offers flexibility.
Overview of Complex Data Types in Maps
Maps in C++ can hold complex data types, including custom structs or classes.
Custom Print Function for Complex Types
For example, we can define a `Student` struct and create a map based on student IDs:
struct Student {
string name;
int age;
};
map<int, Student> studentMap;
To print this map, define a custom print function:
void printComplexMap(const map<int, Student>& myMap) {
for (const auto& pair : myMap) {
cout << "ID: " << pair.first << ", Name: " << pair.second.name << ", Age: " << pair.second.age << endl;
}
}
This makes your output even more informative.
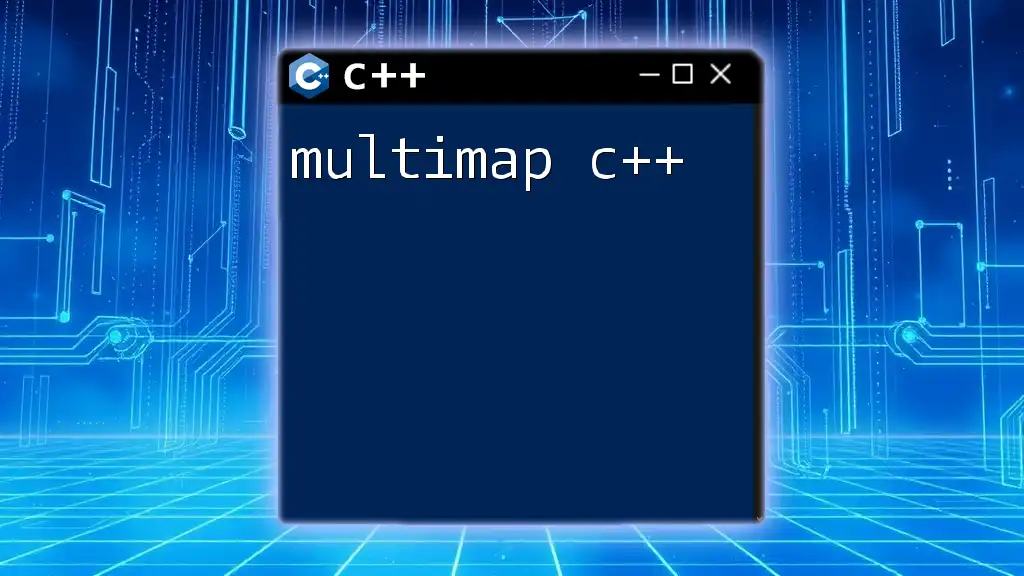
Common Mistakes and Tips
Common Mistakes When Printing Maps
When you're printing a map in C++, certain pitfalls can arise:
- Forgetting to include necessary libraries: Always ensure `#include <map>` and `#include <iostream>` are present.
- Not properly iterating through the map: Using incorrect loop structures can lead to missing data.
Best Practices for Map Printing
When shaping your practice, consider the following tips:
- Use of `const` with iterators: Enhances safety by preventing modifications to the map during iteration.
- Keeping code modular: Creating reusable print functions will save time and facilitate better readability.
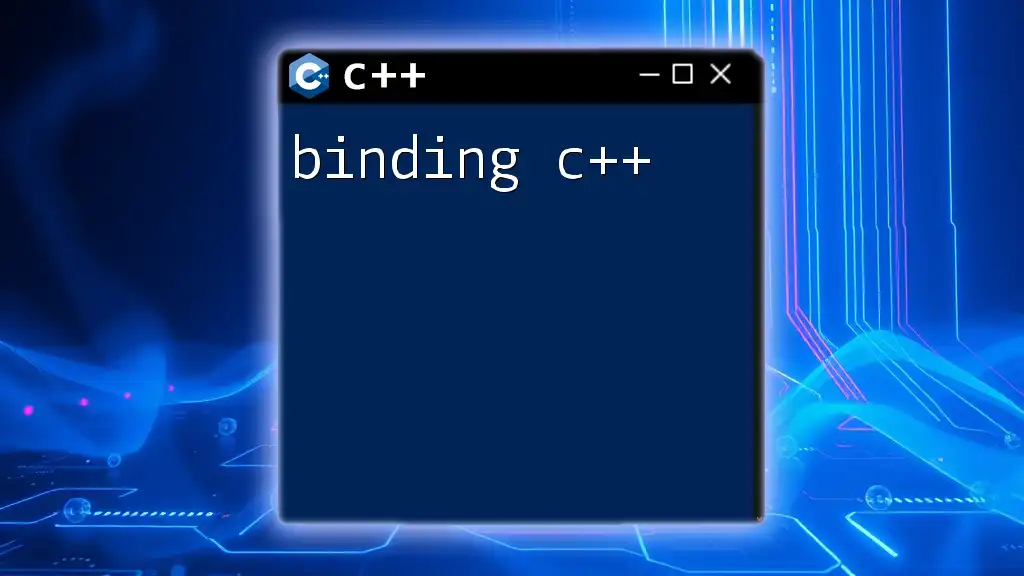
Conclusion
In summary, the ability to effectively print a map in C++ is essential for debugging and data presentation. Understanding how to create, iterate, and format your map outputs can significantly improve your programming experience.
Encouraging further exploration can lead you into more complex C++ structures and methods. Don't hesitate to dive deeper into the STL and discover the vast functionalities available in C++.
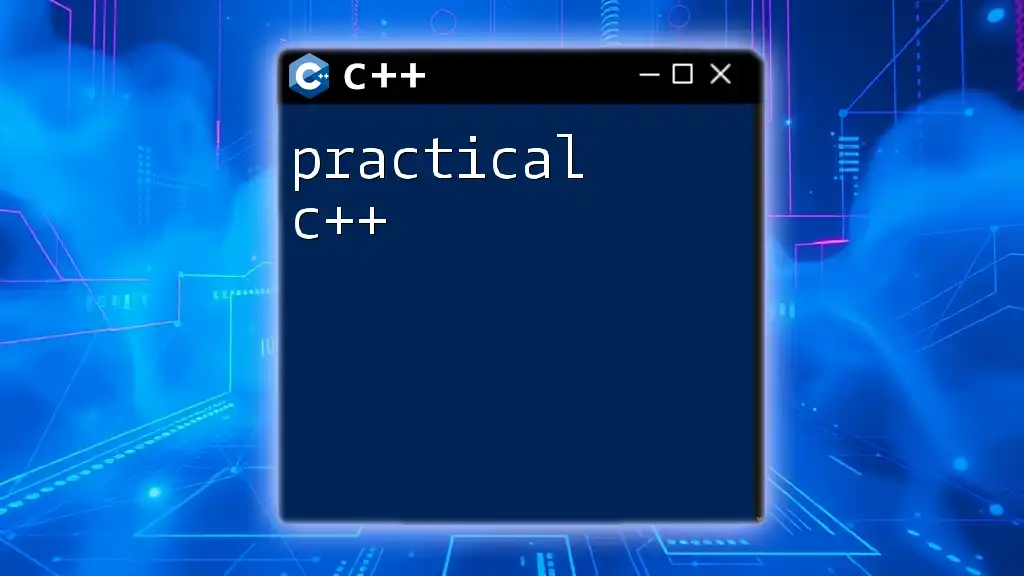
Call to Action
If you find this guide helpful and want to master C++ commands through structured lessons and tutorials, sign up with us. Join our community, and elevate your C++ programming skills today!