Binding in C++ refers to the process of associating a name with a value, which can involve both static binding (resolved at compile time) and dynamic binding (resolved at runtime) through features such as function overloading and polymorphism.
Here's a simple code snippet illustrating both static and dynamic binding in C++:
#include <iostream>
class Base {
public:
virtual void show() { std::cout << "Base Class\n"; }
};
class Derived : public Base {
public:
void show() override { std::cout << "Derived Class\n"; }
};
void demonstrateBinding() {
Base* b = new Derived(); // Dynamic binding
b->show(); // Will call Derived's show()
Base obj; // Static binding
obj.show(); // Will call Base's show()
delete b; // Clean up
}
int main() {
demonstrateBinding();
return 0;
}
What is Binding?
Binding refers to the process of associating a function call with its corresponding function definition in a programming context. In C++, binding can affect how applications function and perform, and it is an essential concept in understanding static and dynamic behavior within object-oriented programming.
Types of Binding
Static Binding
Static binding occurs at compile time. This means that the function to be invoked is determined during compilation. It is advantageous for performance but lacks flexibility, as it does not support polymorphism.
Characteristics of Static Binding:
- Faster than dynamic binding due to early resolution.
- Suitable for non-virtual functions.
Example of Static Binding:
class Base {
public:
void display() { std::cout << "Base class display" << std::endl; }
};
class Derived : public Base {
public:
void display() { std::cout << "Derived class display" << std::endl; }
};
int main() {
Base b;
b.display(); // Calls Base class display
return 0;
}
In the example above, when we call `b.display()`, it invokes the `display()` method of the Base class. This is static binding because the function determined at compile time is the one from the Base class.
Dynamic Binding
Dynamic binding occurs at runtime. This allows C++ to support polymorphism, where the function invoked can vary based on the object type.
Characteristics of Dynamic Binding:
- Immediately resolved during program execution.
- Enables flexibility and extensibility in design.
Example of Dynamic Binding:
class Base {
public:
virtual void display() { std::cout << "Base class display" << std::endl; }
};
class Derived : public Base {
public:
void display() override { std::cout << "Derived class display" << std::endl; }
};
int main() {
Base* b = new Derived();
b->display(); // Calls Derived class display due to dynamic binding
delete b;
return 0;
}
Here, the `display()` method is defined as `virtual` in the Base class. When invoking `b->display()`, C++ uses dynamic binding to call the `display()` method from the Derived class, demonstrating polymorphism.
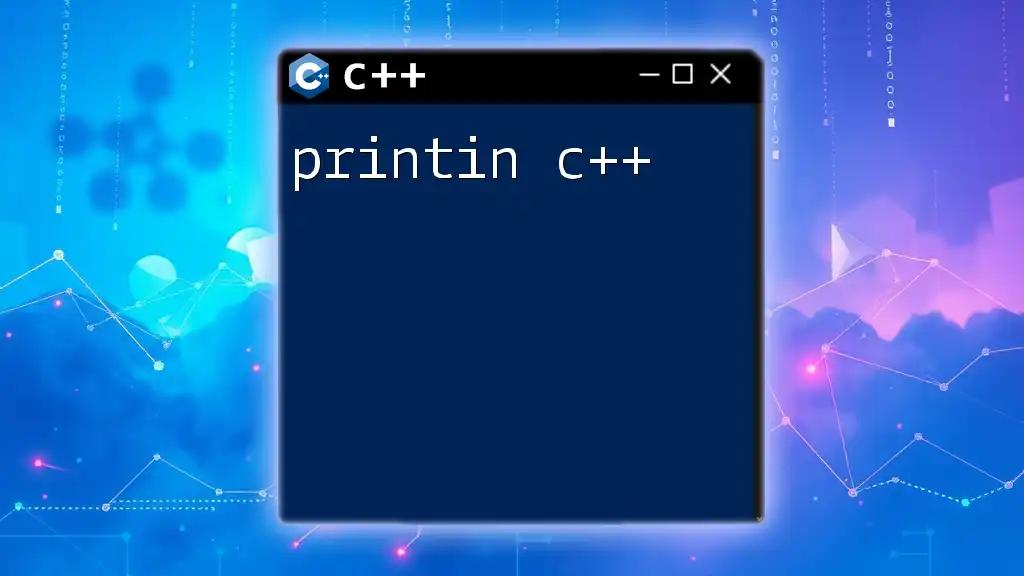
The Process of Binding
Compile-Time Binding
During compile-time binding, the compiler determines which method to bind to a function call. This mechanism is typically faster because it statically links the function's calls. The most common use cases involve simple function calls and when dealing with non-virtual functions.
Run-Time Binding
Run-time binding is more complex. It relies on the use of virtual tables (vtable) to keep track of the functions associated with a particular class. Every object of a class with virtual functions has a pointer to the vtable (known as vptr), which contains pointers to the dynamically bound functions.
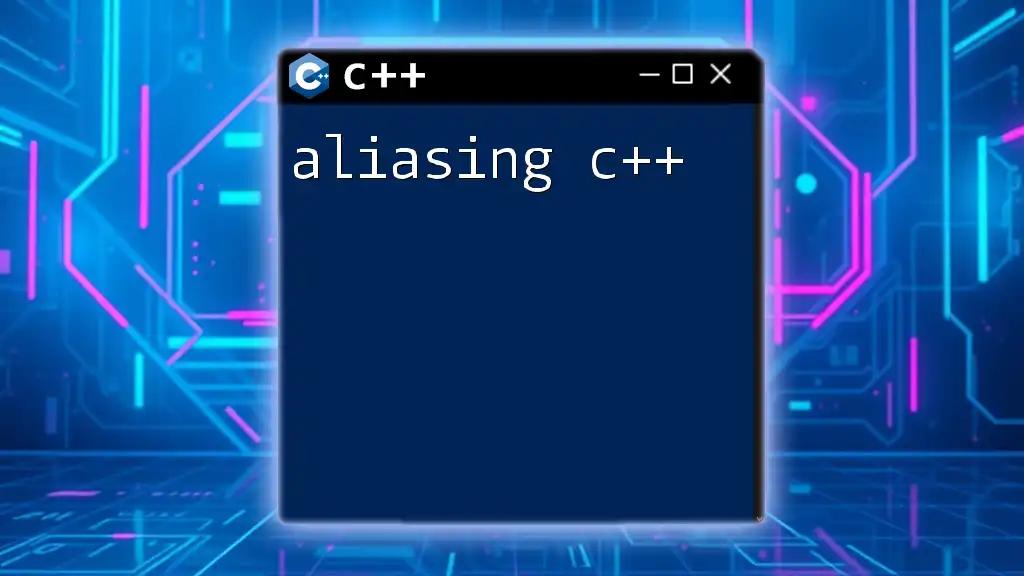
The Role of Virtual Functions
What Are Virtual Functions?
Virtual functions are functions declared within a class that can be overridden in derived classes. The primary purpose of declaring a method as virtual is to allow for dynamic binding. This leads to a more flexible code structure, enabling different behaviors for derived classes.
Creating Virtual Functions
You create a virtual function by placing the `virtual` keyword before the function declaration.
class Base {
public:
virtual void print() {
std::cout << "Base Print Function" << std::endl;
}
};
Overriding Virtual Functions
To override a virtual function, you redefine it in a derived class.
class Derived : public Base {
public:
void print() override {
std::cout << "Derived Print Function" << std::endl;
}
};
In this example, when `print()` is called on a Derived class object, it executes the overridden version, showcasing the dynamic nature of binding.
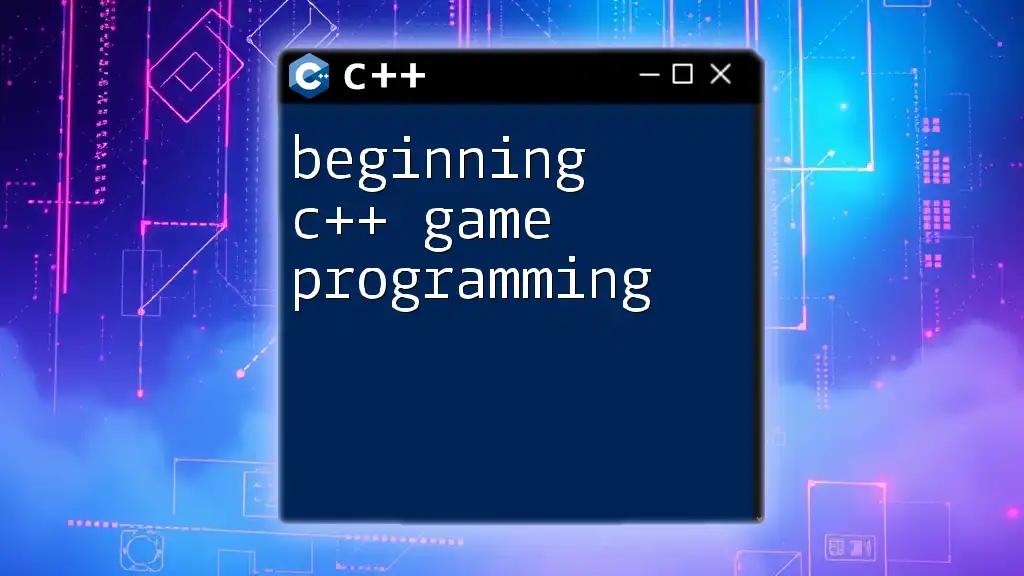
Advantages and Disadvantages of Binding
Advantages of Static Binding
- Performance: Static binding is resolved at compile-time, which results in faster function calls.
- Simplicity: It's easier to understand and implement since the behavior is predictable.
Disadvantages of Static Binding
- Lack of Flexibility: It is rigid and does not allow for changes in behavior based on object type at runtime.
Advantages of Dynamic Binding
- Flexibility and Extensibility: It allows C++ programs to decide at runtime which method to invoke. This adaptability is crucial, especially for applications that require runtime decisions based on user input or other dynamic factors.
Disadvantages of Dynamic Binding
- Performance Overhead: The process of resolving function calls at runtime can introduce latency.
- Complexity of Implementation: Managing virtual functions and understanding polymorphism can complicate the code.

Case Studies in Binding
Real-world Applications of Static Binding
Static binding is often used in scenarios where performance is critical. For example, in gaming or real-time systems where consistent response times are necessary, static binding facilitates predictable behavior without the overhead of dynamic resolution.
Real-world Applications of Dynamic Binding
Dynamic binding shines in software systems that are built with extensibility in mind. For instance, in graphical user interfaces (GUIs), you might want to invoke different event handlers based on user actions, which is efficiently managed using dynamic binding.

Best Practices for Binding in C++
Efficient Use of Binding
When designing a C++ application, consider the following guidelines:
- Use static binding when performance is the priority and polymorphism is not needed.
- Use dynamic binding when dealing with complex hierarchies where behavior must vary between derived classes.
Common Pitfalls and How to Avoid Them
- Misuse of Virtual Functions: Ensure that classes use virtual functions judiciously; unnecessary virtual functions can increase overhead.
- Object Slicing and Dynamic Binding: Be mindful of object slicing when dealing with references or pointers. Always use base pointers to handle derived objects to retain dynamic binding capabilities.
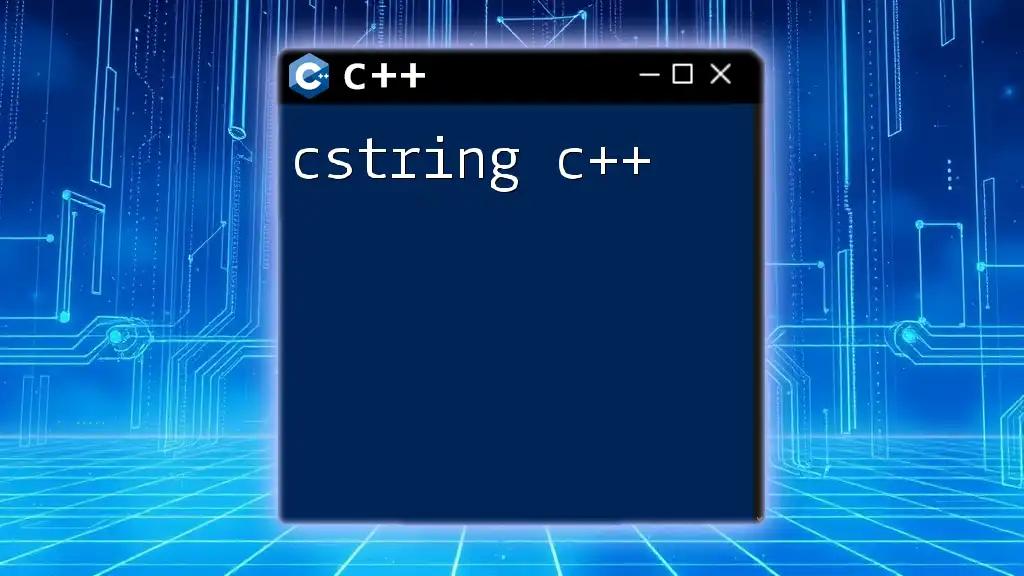
Conclusion
Understanding binding in C++ is vital for developing effective and efficient applications. Whether opting for static or dynamic binding, each has its advantages and disadvantages that must be considered in the context of your application's requirements. By mastering binding and the role of virtual functions, you can create more flexible and powerful C++ programs that harness the true potential of object-oriented programming.