In C++, the `strcmp` function is used to compare two strings lexicographically and returns 0 if they are equal, a positive value if the first string is greater, and a negative value if the second string is greater.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cstring>
int main() {
const char* str1 = "Hello";
const char* str2 = "World";
int result = strcmp(str1, str2);
if (result == 0) {
std::cout << "Strings are equal." << std::endl;
} else if (result < 0) {
std::cout << "str1 is less than str2." << std::endl;
} else {
std::cout << "str1 is greater than str2." << std::endl;
}
return 0;
}
Understanding Strings in C++
In C++, strings can be handled in two primary ways: C-style strings and C++ strings (specifically `std::string`). C-style strings are simply arrays of characters terminated by a null character (`'\0'`). They require manual memory management and string manipulation functions from the C standard library.
On the other hand, `std::string`, part of the C++ Standard Library, provides a more user-friendly and efficient way of handling strings with built-in functionalities like resizing, copying, and concatenation. This article will primarily focus on `std::string`, as it is more commonly used in modern C++ programming.

Overview of C++ String Comparison
String comparison is a fundamental operation in programming that allows developers to check if two strings are equal, and determine their ordering (e.g., which comes first lexicographically). Understanding how to appropriately compare strings is crucial for tasks such as input validation, searching, and sorting.

Basic String Comparison in C++
Using Equality Operators
The most straightforward way to compare strings in C++ is to use the equality operator (`==`). This operator checks whether two strings contain the same sequence of characters.
Code Snippet: Basic Comparison
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "World";
if (str1 == str2) {
std::cout << "Strings are equal." << std::endl;
} else {
std::cout << "Strings are not equal." << std::endl;
}
return 0;
}
In this example, the output will indicate that the strings are not equal, as "Hello" and "World" contain different characters. It's important to note that the `==` operator takes care of null terminators and handles memory automatically.
Common Pitfalls: One common mistake occurs when trying to compare string literals directly using `==`, which may lead to comparison errors if you mistakenly treat them as pointers instead of actual strings.
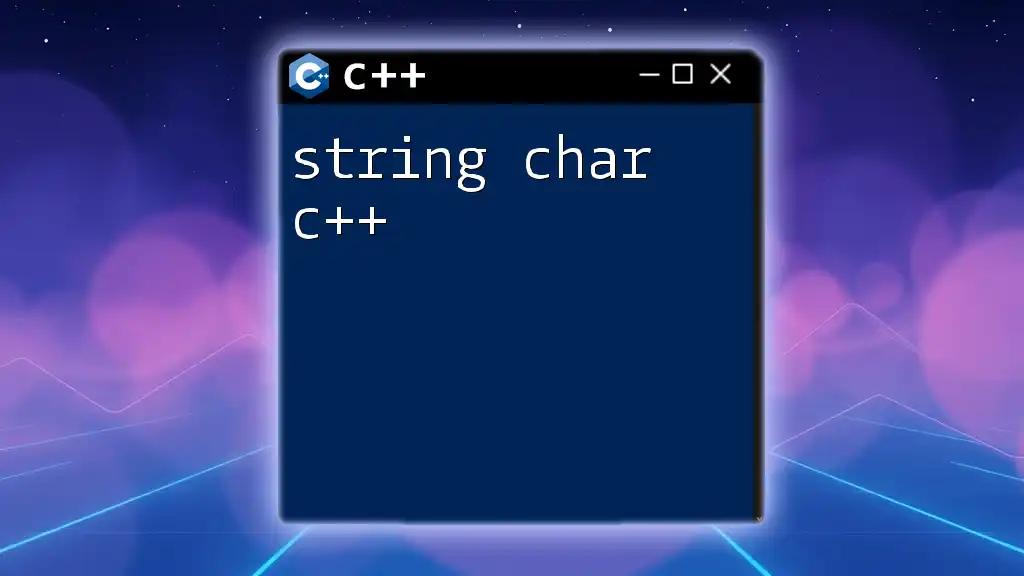
Advanced String Comparison Techniques
Using `std::string::compare()`
The `compare` method provides another way to compare strings. It returns an integer that can indicate whether the first string is less than, equal to, or greater than the second string.
Code Snippet: Using std::string::compare()
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "Hello";
int result = str1.compare(str2);
if (result == 0) {
std::cout << "Strings are equal." << std::endl;
} else if (result < 0) {
std::cout << "str1 is less than str2." << std::endl;
} else {
std::cout << "str1 is greater than str2." << std::endl;
}
return 0;
}
In this case, since both strings are equal, the output will confirm their equality. The `compare` method works as follows:
- Return 0: If the strings are equal.
- Return negative value: If the first string is less than the second.
- Return positive value: If the first string is greater than the second.
Use cases for `compare()` include sorting, searching, and complex validation tasks where knowing the lexicographical order is necessary.
Comparing Strings Using `strcmp()`
For those who still prefer or require C-style strings, the `strcmp()` function from the C standard library is an option. While it operates on C strings (`const char*`), it follows a similar logic to `std::string::compare()`.
Code Snippet: Using strcmp
#include <iostream>
#include <cstring>
int main() {
const char* str1 = "Hello";
const char* str2 = "World";
int result = strcmp(str1, str2);
if (result == 0) {
std::cout << "Strings are equal." << std::endl;
} else if (result < 0) {
std::cout << "str1 is less than str2." << std::endl;
} else {
std::cout << "str1 is greater than str2." << std::endl;
}
return 0;
}
In this example, since "Hello" is lexicographically less than "World", the corresponding output would indicate this.
Pros and cons of using `strcmp()` include the need for manual memory management and a lack of safety features compared to `std::string`.
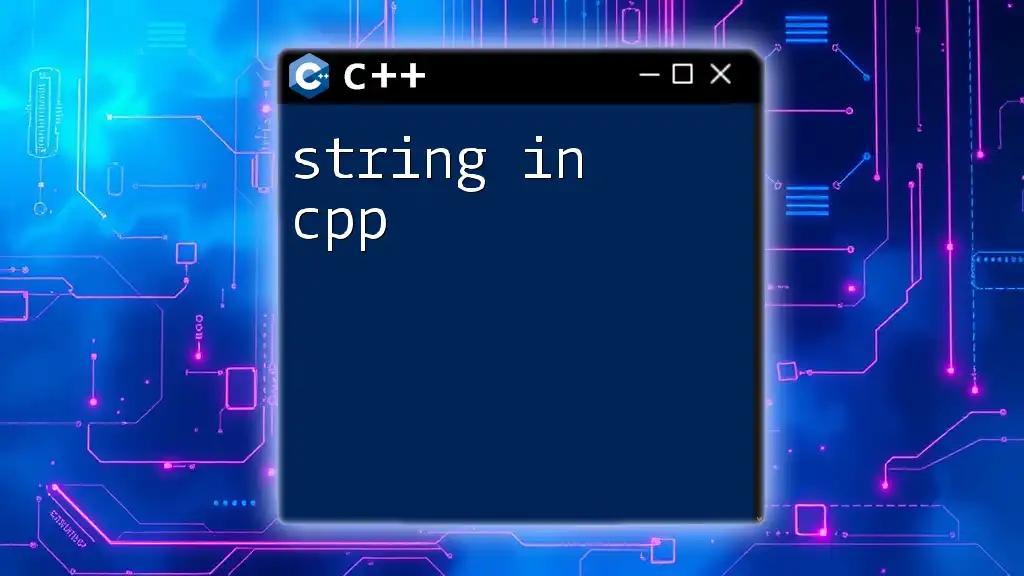
Substring Comparison in C++
How to Compare Substrings
Sometimes, you may only need to compare part of a string. This is easily done using the `substr()` method available in `std::string`.
Code Snippet: Substring Comparison
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string subStr = str.substr(0, 5);
if (subStr == "Hello") {
std::cout << "Substring matches!" << std::endl;
}
return 0;
}
In this case, the program extracts the first five characters of `str` and compares it with "Hello". The output would confirm that the substring matches.
Substrings are particularly useful for validating user input or searching within larger strings. When comparing substrings, always be sure to handle cases where the substring may be longer than the main string to avoid errors.

Best Practices for Comparing Strings
When it comes to string comparison, here are some best practices:
- Use `std::string` over C-style strings (`const char*`) whenever possible. This minimizes memory management issues.
- Preferred methods for comparing strings include the `==` operator and `std::string::compare()`. They are straightforward and safe.
- Be aware of case sensitivity. If you require case-insensitive comparisons, consider converting both strings to the same case using `std::transform()` or `std::tolower()` before comparing.
- Avoid potential pitfalls such as comparing pointers instead of string values, which may lead to unexpected behavior.

Conclusion
Understanding how to effectively compare strings in C++ is fundamental for successful software development. Whether you're employing operators, methods like `compare()`, or relying on functions such as `strcmp()`, each technique has its purposes and best-use scenarios. Practice these techniques to enhance your C++ programming skills, and rest assured that mastering `string compare cpp` will prove invaluable in your coding journey.
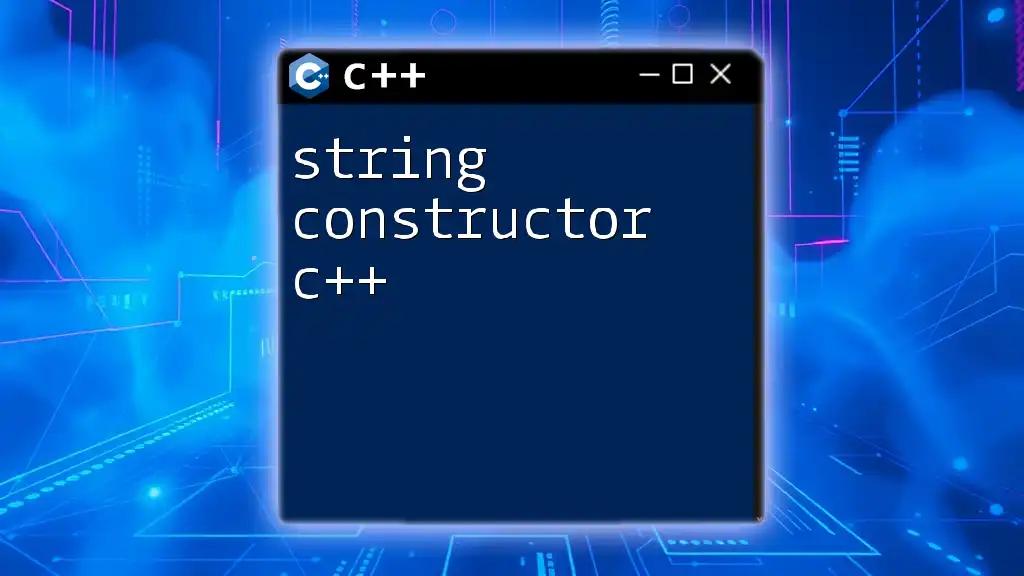
Resources and Further Reading
For further insight, refer to comprehensive documentation such as cppreference.com for more examples and in-depth explanations on string handling in C++. Explore exercises that challenge your understanding and help consolidate your skills in string comparison.