In C++, string copying can be accomplished using the `strcpy()` function from the `<cstring>` library to copy C-style strings, or by using the assignment operator for `std::string` objects.
Here's a simple example using both methods:
#include <iostream>
#include <cstring>
int main() {
// C-style string copying
char source[] = "Hello, World!";
char destination[20];
strcpy(destination, source);
std::cout << "C-style string copy: " << destination << std::endl;
// C++ string copying
std::string str1 = "Hello, C++!";
std::string str2 = str1; // Using assignment operator
std::cout << "C++ string copy: " << str2 << std::endl;
return 0;
}
Understanding C-style Strings
C-style strings are arrays of `char` that are terminated with a null character (`\0`). This null-termination is how the program knows where the string ends.
You can declare a C-style string like this:
char str1[20] = "Hello, World!";
In this example, `str1` can hold up to 19 characters plus the terminating null character. It’s essential to keep this in mind when performing string operations, as exceeding the boundary of the allocated memory can lead to undefined behavior.
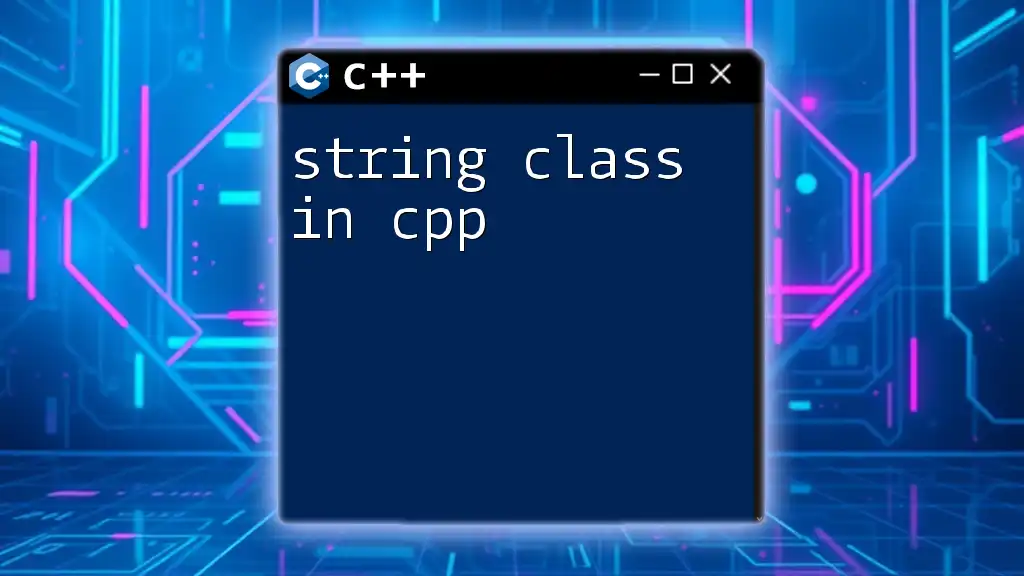
Using `strcpy` for String Copy Operations
The `strcpy` function is a common way to copy C-style strings. It is defined in the `<cstring>` header and requires you to follow certain conventions.
Syntax of `strcpy`:
char *strcpy(char *dest, const char *src);
- Parameters:
- `dest`: Pointer to the destination where you want to copy the string.
- `src`: Pointer to the source string you are copying from.
Example of Using `strcpy`:
char source[] = "Hello";
char destination[20];
strcpy(destination, source);
In this example, `strcpy` copies the content of `source` into `destination`. After this operation, `destination` will contain the string "Hello".
Beware of Buffer Overflow
Buffer overflow is a significant concern when using `strcpy`. If the size of the `src` string exceeds the allocated size of the `dest` buffer, you could overwrite memory, leading to undefined behavior or crashes.
Consider this risky scenario:
char shortDest[5];
strcpy(shortDest, "Hello"); // Risk of overflow!
In this case, "Hello" is 6 characters long, including the null terminator, while `shortDest` is only 5 characters long. This leads to overflow and undefined behavior.
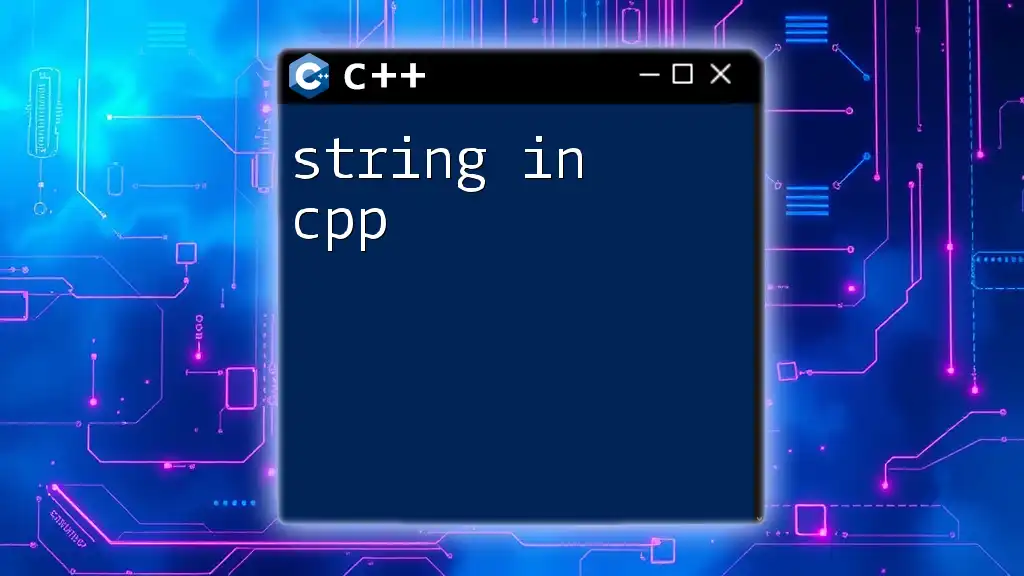
Safe Alternatives to `strcpy`
Introduction to `strncpy`
To mitigate risks associated with copying strings, you can use `strncpy`. This function allows you to specify the maximum number of characters to copy, making it safer.
Usage of `strncpy`:
strncpy(destination, source, sizeof(destination) - 1);
destination[sizeof(destination) - 1] = '\0'; // Ensuring null termination
In this code, the `sizeof(destination) - 1` ensures that we do not exceed the buffer limit, as we leave space for the null terminator.
When to Use `strncpy`
Use `strncpy` when you are unsure of the source string's length or when dealing with untrusted input. However, be cautious, as `strncpy` does not automatically null-terminate the destination string if the source string's length exceeds the specified limit.
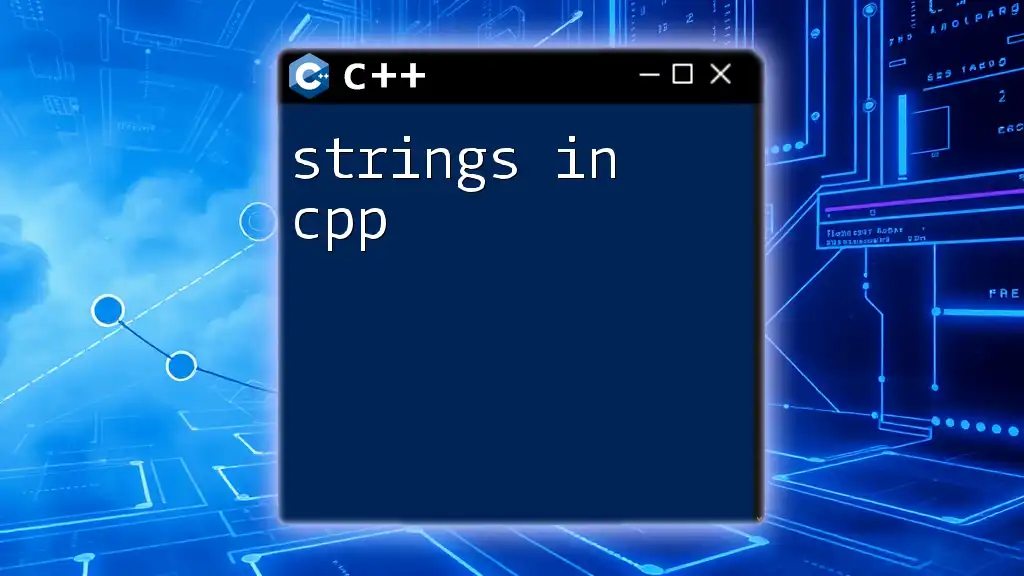
Working with `std::string`
What is `std::string`?
`std::string` is part of the C++ Standard Library and offers a higher-level abstraction compared to C-style strings. It manages memory automatically, reduces the chances of errors, and provides various member functions for manipulation.
Copying Strings in `std::string`
To copy strings using `std::string`, you can employ the assignment operator (`=`) or the copy constructor.
Example:
std::string str1 = "Hello";
std::string str2 = str1; // Using the copy constructor
After this assignment, both `str1` and `str2` will contain the string "Hello".
Using the `assign` Method
You can also use the `assign` method to copy strings:
std::string str1 = "Hello";
std::string str2;
str2.assign(str1);
This assignment method is especially useful when you want to copy a substring or when the source type isn't compatible with the assignment operator.
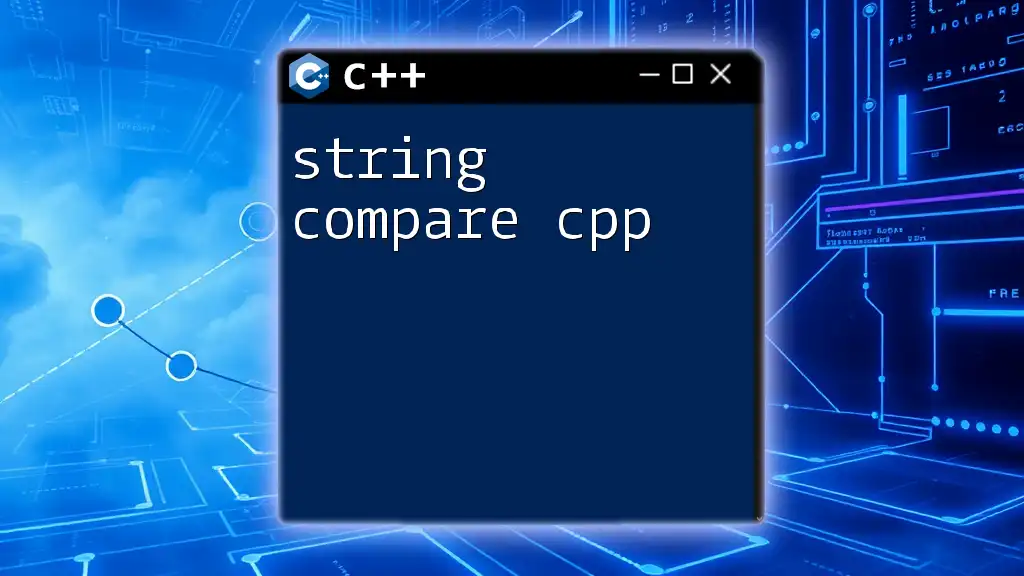
Advanced String Copy Techniques
Using `std::copy` Algorithm
For advanced copying mechanisms, you can leverage the `<algorithm>` library's `std::copy` function. This approach can be beneficial when dealing with raw arrays derived from a `std::string`.
Example:
std::string source = "Hello";
char destination[20];
std::copy(source.begin(), source.end(), destination);
destination[source.size()] = '\0'; // Null-termination
This snippet copies each character from `source` to `destination`, ensuring you handle null termination explicitly.
Using Move Semantics in C++11 and Later
With the advent of C++11, move semantics greatly enhance efficiency. When you copy an `std::string`, you can avoid excessive copying by using move semantics, which transfer ownership instead of duplicating data.
Example:
std::string a = "Hello";
std::string b = std::move(a); // 'a' is now empty
In this example, the contents of string `a` are transferred to `b`, leaving `a` empty, thus improving performance by reducing unnecessary copying.
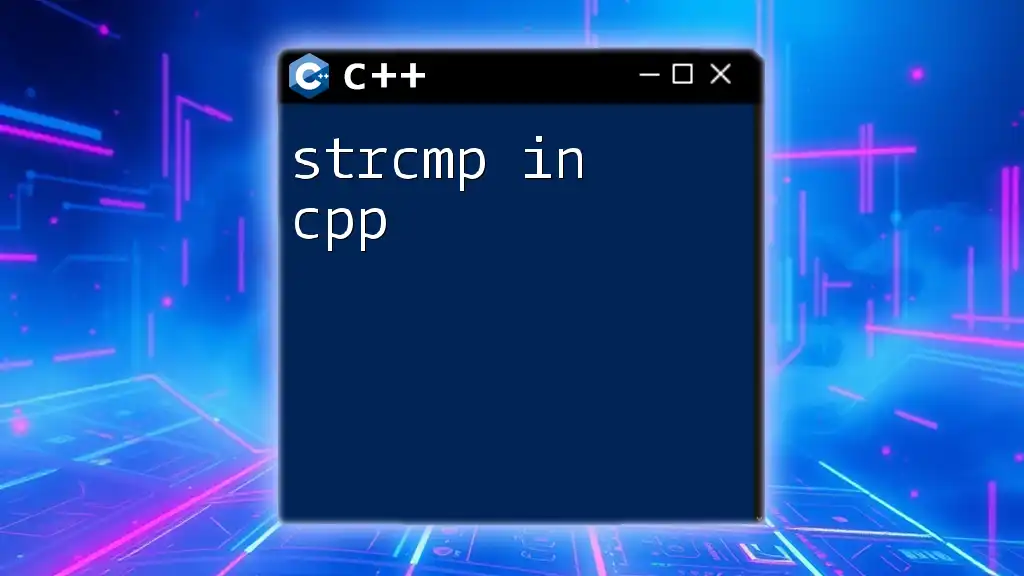
Common Pitfalls When Copying Strings
Null Pointer Dereferencing
Always ensure that your pointers are valid before dereferencing them. Attempting to copy from or to a null pointer will lead to runtime errors.
Potential Memory Leaks
When using C-style strings and dynamic memory allocation, always make sure to `free` allocated memory to prevent leaks. In contrast, `std::string` automatically manages memory, significantly reducing this risk.
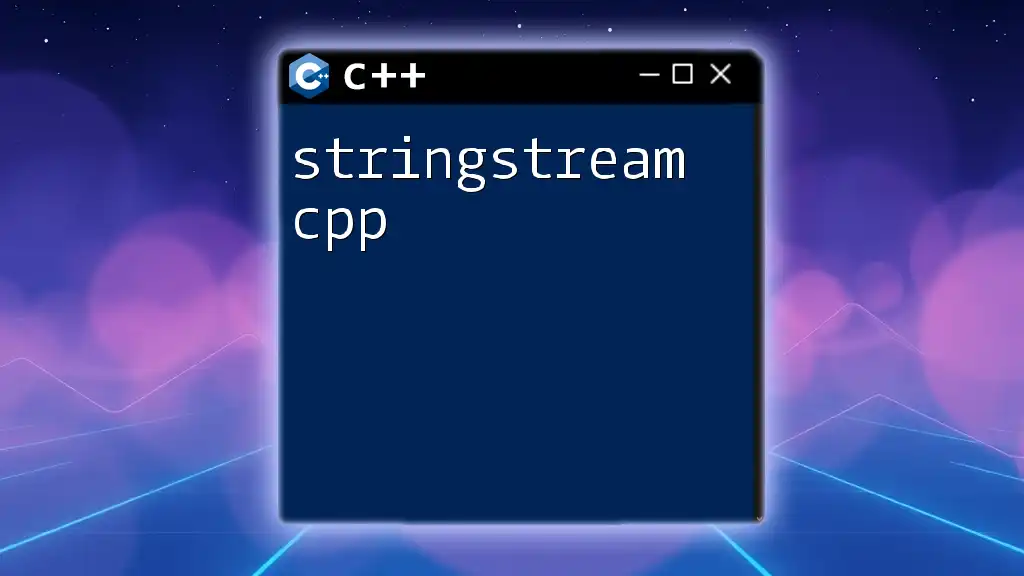
Conclusion
Understanding string copying in C++—whether using C-style strings or `std::string`—is vital for effective memory management and program stability. By following safe practices like using `strncpy` and leveraging modern C++ features such as move semantics, you can manipulate strings efficiently and safely.
Final Thoughts on Best Practices
Always prioritize safe string manipulation to avoid common pitfalls. Mastering string copy methods will not only improve your coding skills but also enhance the quality and stability of your applications.