The `printf` function in C++ is used to output formatted text to the console, allowing for precise control over the appearance of the output.
#include <cstdio>
int main() {
int age = 25;
printf("I am %d years old.\n", age);
return 0;
}
Understanding printf in C++
The `printf` function is a standardized output function in C that has carried over to C++ as a means of displaying formatted text to the console. At its core, `printf` serves one primary purpose: to print formatted output to the standard output (usually the terminal). The function's syntax is defined as follows:
int printf(const char *format, ...);
This syntax implies that `printf` takes a format string followed by an optional list of arguments, allowing you to control how the output appears. Understanding how to leverage `printf` is crucial for any developer looking to effectively utilize C++ on legacy systems or for those who favor its straightforward functionality.
Basic Usage of printf in C++
Using `printf` is quite simple. Here is a basic example demonstrating its use to print a string:
#include <iostream>
#include <cstdio>
int main() {
printf("Hello, World!\n");
return 0;
}
In this code snippet, the text "Hello, World!" is sent to the standard output, followed by a newline character. This simplicity is one of the features that make `printf` appealing for rapid console output.
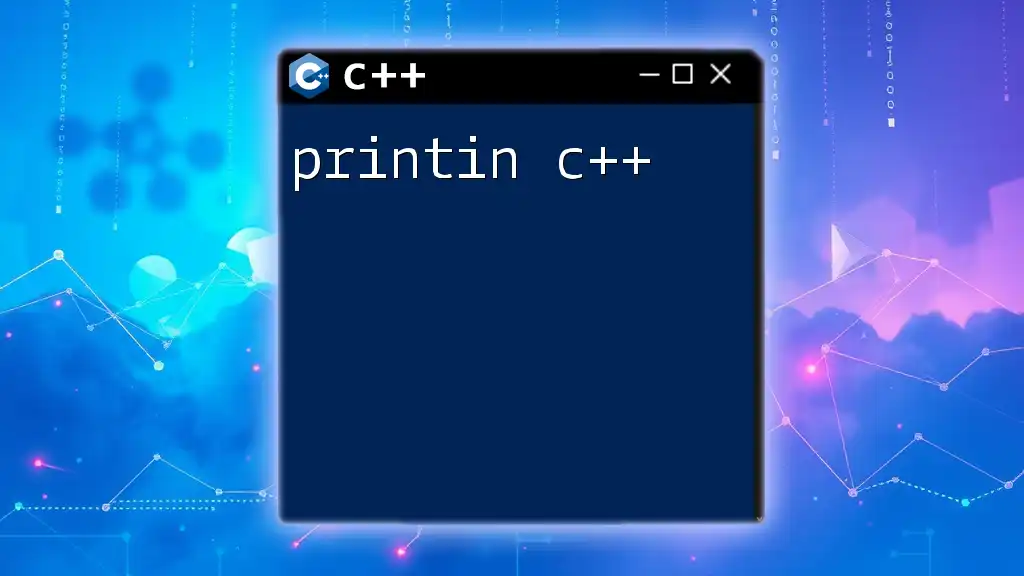
Format Specifiers in printf
The true power of `printf` lies in its format specifiers, which control how data types are represented in the output. Some of the most commonly used format specifiers include:
- `%d`: Represents signed integers.
- `%f`: Used for floating-point numbers.
- `%s`: Applies to strings.
- `%c`: Used to print individual characters.
- `%x`: Converts numbers to hexadecimal format.
Here’s an example that prints different data types:
int num = 10;
float pi = 3.14;
printf("Integer: %d, Float: %.2f\n", num, pi);
In this snippet, `num` is formatted as an integer using `%d`, and `pi` is displayed as a floating-point number with two decimal places thanks to `%.2f`.
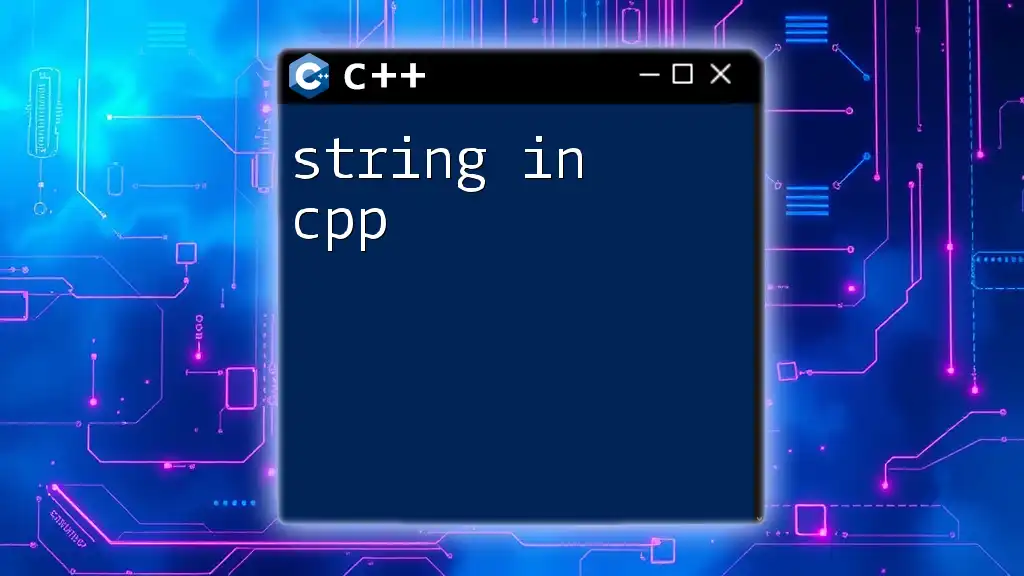
Formatting Output
When you want to control the width of your output, `printf` allows you to specify minimum field widths and precision.
Minimum Field Width
This feature is useful for creating neatly aligned columns in your output. You can set a minimum width for your formatted output as shown below:
printf("|%10d|%10.4f|\n", num, pi);
In this code, `%10d` ensures that the integer occupies at least 10 characters, while `%10.4f` specifies that the floating-point number will take 10 characters in total, with 4 characters after the decimal.
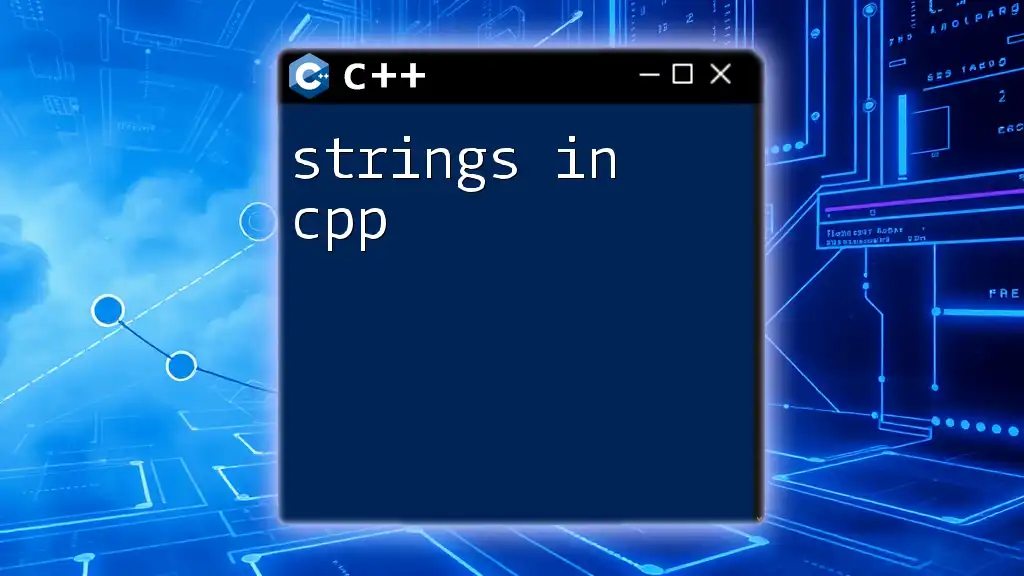
Special Characters in printf
Using special characters to enhance your output is another crucial aspect of `printf`. You can implement escape sequences to control spacing and formatting:
- `\n`: Inserts a newline.
- `\t`: Adds a tab space.
- `\\`: Outputs a backslash.
Here's an example of creating a simple table:
printf("Name\tAge\nJohn\t25\n");
This results in a neatly formatted table with names and ages aligned due to the use of the tab character.
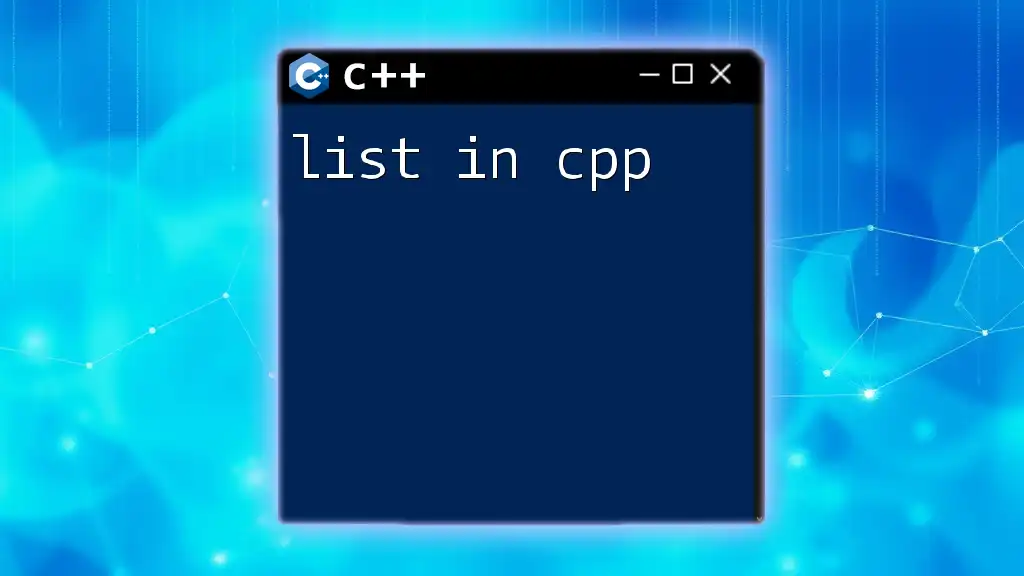
Advanced Features of printf in C++
`Printf` offers advanced formatting options through a combination of format specifiers and flags. You can use arguments to alter how your output is displayed.
Combining Format Specifiers
One useful feature is the ability to combine format specifiers. For instance, you can achieve left alignment and zero-padding in your output:
printf("%-10s %05d\n", "Item", 1);
In this code, `%-10s` left-aligns the string "Item" with a total width of 10 characters, while `%05d` pads the integer with zeros, ensuring it is always 5 digits long.
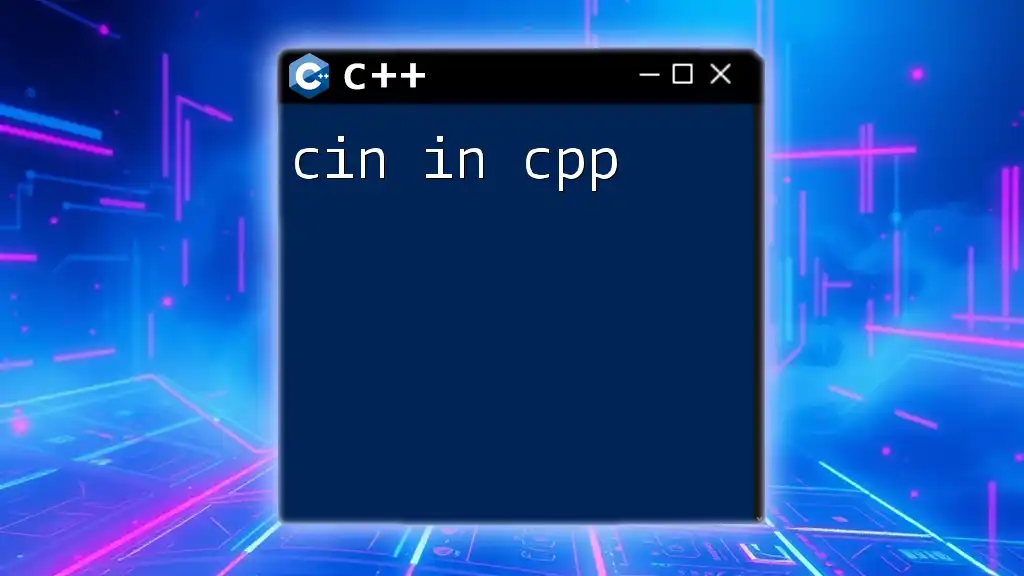
Error Handling with printf
While `printf` is powerful, it is essential to be aware of its limitations, particularly regarding error management. The function returns the total number of characters printed, which allows you to check for errors in your output:
if (printf("Hello, World!\n") < 0) {
// Handle error
}
By checking the return value, you can effectively manage any issues that arise during output.
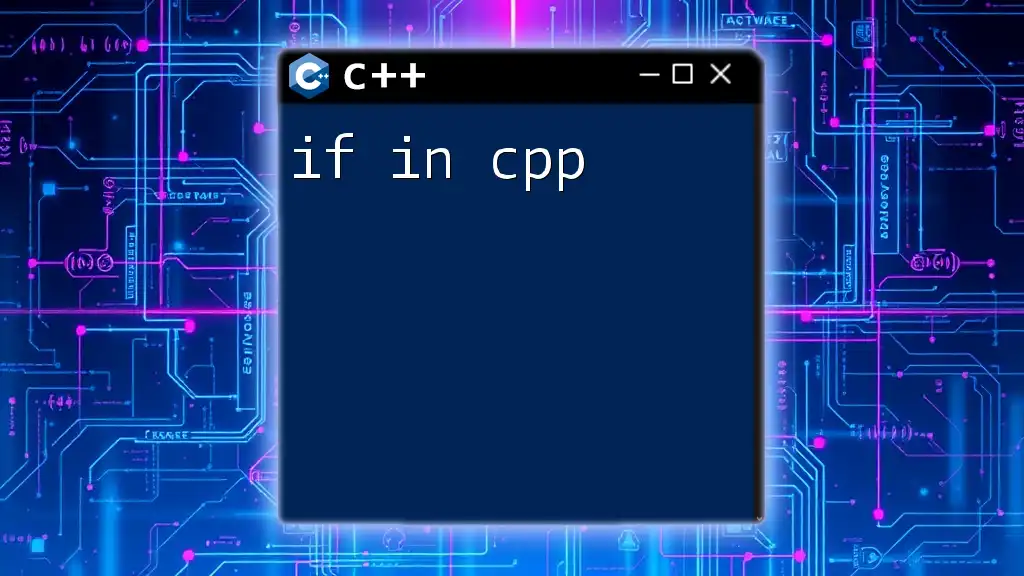
Comparison with C++ iostream
While `printf` is a versatile tool, it's important to compare it with C++'s `iostream`. Here are some differences:
- Performance: `printf` can be faster for formatted output in certain situations due to its lower overhead.
- Flexibility: `iostream` uses type safety features in C++ to streamline output, making it more consistent with modern C++ practices.
However, there are scenarios where `printf` shines. For example, when dealing with legacy codebases or when you require advanced formatting capabilities, `printf` is often the better option.
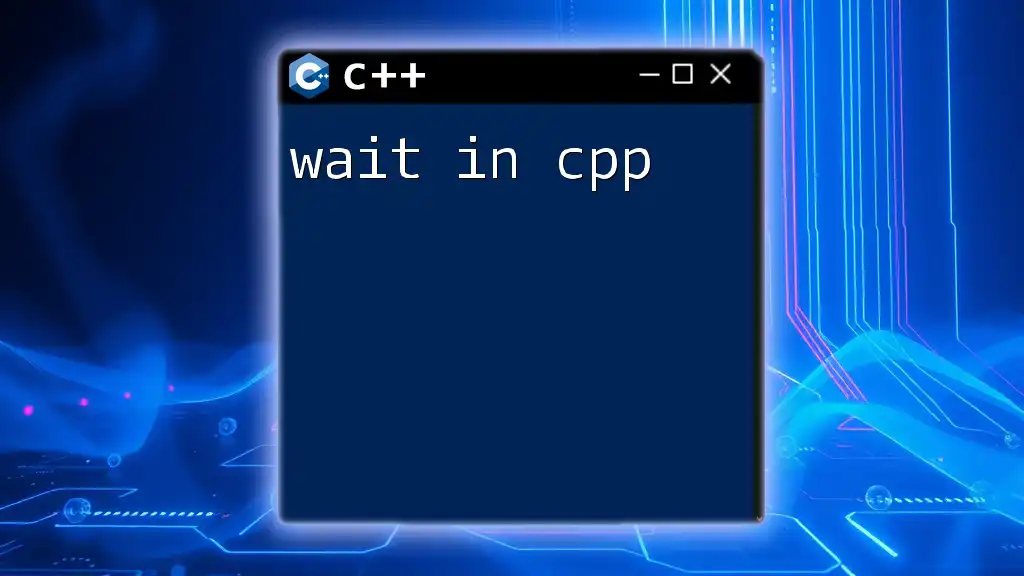
Conclusion
Mastering `printf in cpp` is an invaluable skill for any programmer. By understanding its functionalities, format specifiers, and advanced formatting techniques, you can write cleaner, more effective code. Remember the benefits of `printf`, especially in terms of speed and formatting flexibility, as you continue to develop your programming skills.
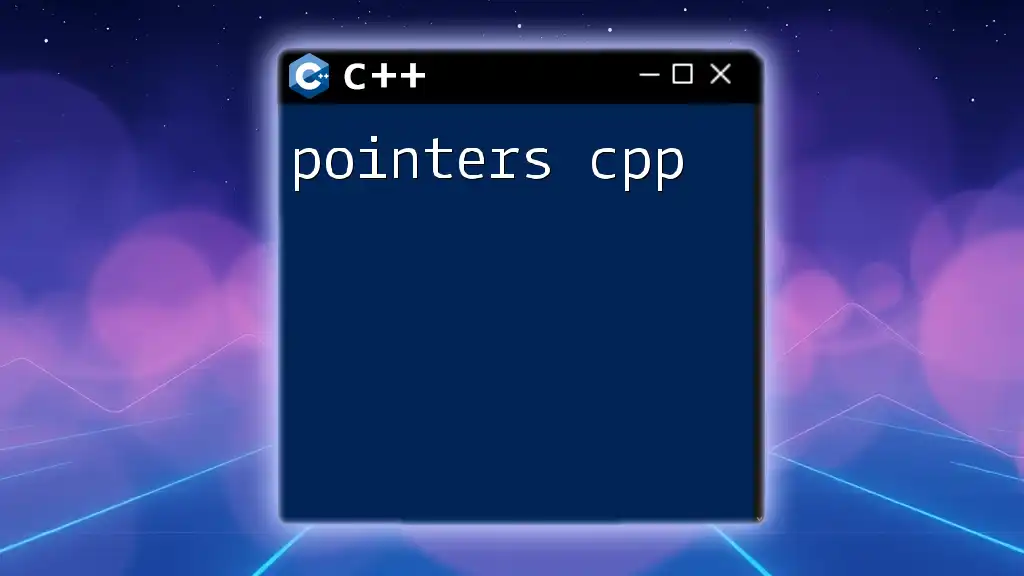
Additional Resources
For further reading, consider exploring online tutorials, recommended books, and community forums dedicated to C++ programming. Resources like Cplusplus.com or the official C++ documentation are excellent places to deepen your understanding of `printf` and other C++ functions.
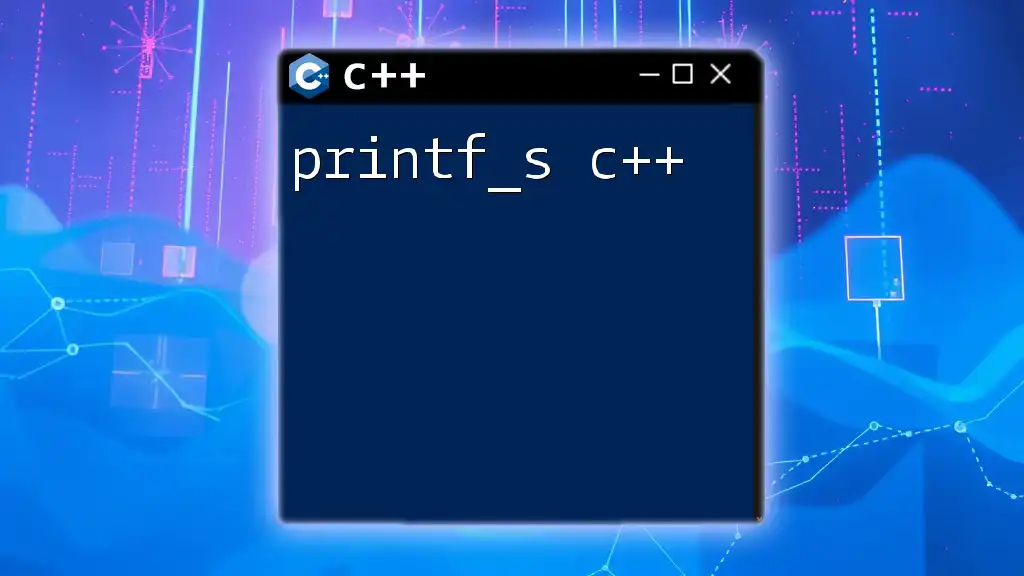
Call to Action
To enhance your C++ skills further, subscribe to our newsletter for regular tips and tutorials covering essential commands and practices. Apply the concepts learned here, and watch your proficiency in C++ soar!