Hashing in C++ involves converting data into a fixed-size value that represents the original input, commonly used in data structures like hash tables for efficient data retrieval. Here's a simple example using the `std::unordered_map` from the C++ Standard Library:
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
std::unordered_map<std::string, int> hashMap;
hashMap["apple"] = 1;
hashMap["banana"] = 2;
std::cout << "Hash for 'apple': " << hashMap["apple"] << std::endl;
return 0;
}
Understanding the Basics of Hashing
What is a Hash Function?
A hash function is a mathematical function that converts an input (or 'key') into a fixed-size string of bytes. The output, typically called a hash code, is designed to uniquely represent the original input. The primary purpose of a hash function is to ensure that data can be efficiently retrieved and stored.
A good hash function has three main characteristics:
- Deterministic: The same input will always produce the same output.
- Fast computation: The function should return the hash value quickly.
- Uniform distribution: Hash values should be evenly distributed across the possible output range to minimize collisions.
Types of Hash Functions
Hash functions fall into two main categories based on their applications: cryptographic hash functions and non-cryptographic hash functions.
Cryptographic Hash Functions
These functions are designed to be secure against various attacks. They generate hash codes that are difficult to replicate or reverse-engineer. Common cryptographic hash functions include MD5, SHA-1, and SHA-256. They are often used in security applications, such as password storage and digital signatures.
Non-Cryptographic Hash Functions
These functions prioritize speed and performance rather than security. They are used in hash tables, data structures, and algorithms where quick access to data is required. Examples include MurmurHash and FNV.
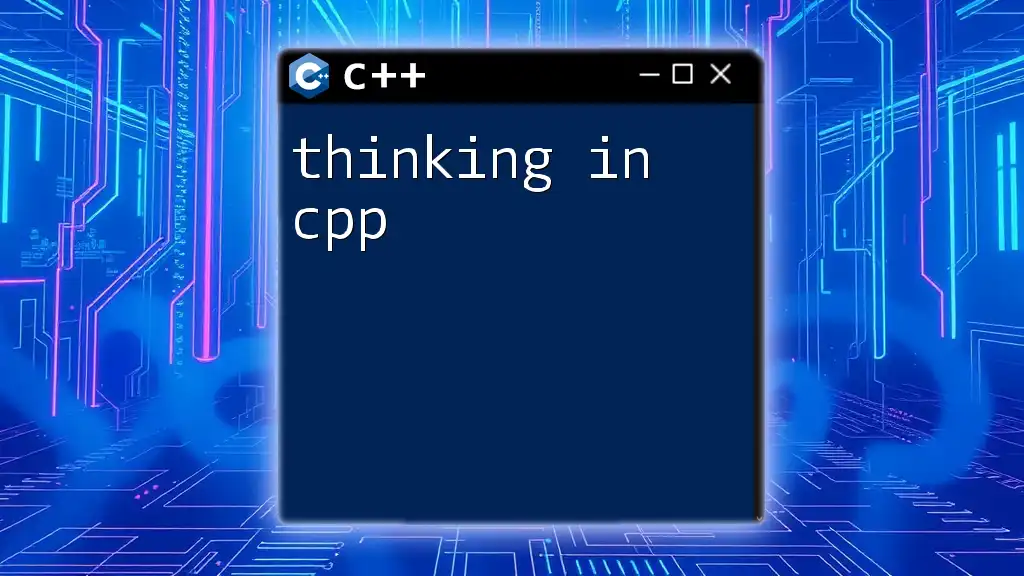
How Hashing Works in C++
Using Hash Functions with STL
C++ provides a comprehensive Standard Template Library (STL), which includes data structures like `std::unordered_map` and `std::unordered_set` that utilize hashing. These collections automatically manage hashing and may even allow you to specify your own hash function.
Here's a brief overview:
- `std::unordered_map`: A hash table based implementation that allows you to store key-value pairs. Access and modification typically happen in constant time, O(1).
- `std::unordered_set`: A collection that contains unique keys. It is also implemented using a hash table, ensuring that duplicate entries are not allowed.
Creating Custom Hash Functions
When using STL hash-based containers, you might sometimes need a custom hash function. This is especially the case when using complex data types.
Defining a Custom Hash Function Creating a custom hash function allows you to define how unique keys should be represented. Below is an example of a custom hash function for a `struct` that contains two integers.
#include <iostream>
#include <unordered_map>
struct CustomKey {
int a;
int b;
};
// Custom hash function
struct CustomHash {
std::size_t operator()(const CustomKey& k) const {
return std::hash<int>()(k.a) ^ std::hash<int>()(k.b);
}
};
// Usage
std::unordered_map<CustomKey, int, CustomHash> myMap;
In this example, the `CustomHash` struct overloads the `operator()`, allowing it to act as a hash function for `CustomKey`. The hash values are computed using the XOR operation to combine the hashes of the individual fields.
Handling Collisions
Despite the careful design of hash functions, collisions occur when different keys produce the same hash value. It is crucial to implement methods for handling these collisions effectively.
Chaining
In chaining, each entry in the hash table points to a linked list of entries that hash to the same index. This allows multiple values to coexist at the same index.
Open Addressing
In open addressing, when a collision occurs, the hash table finds the next available slot using various probing methods:
- Linear probing: Incrementing the index sequentially until finding an empty slot.
- Quadratic probing: Using a quadratic function to find the next index.
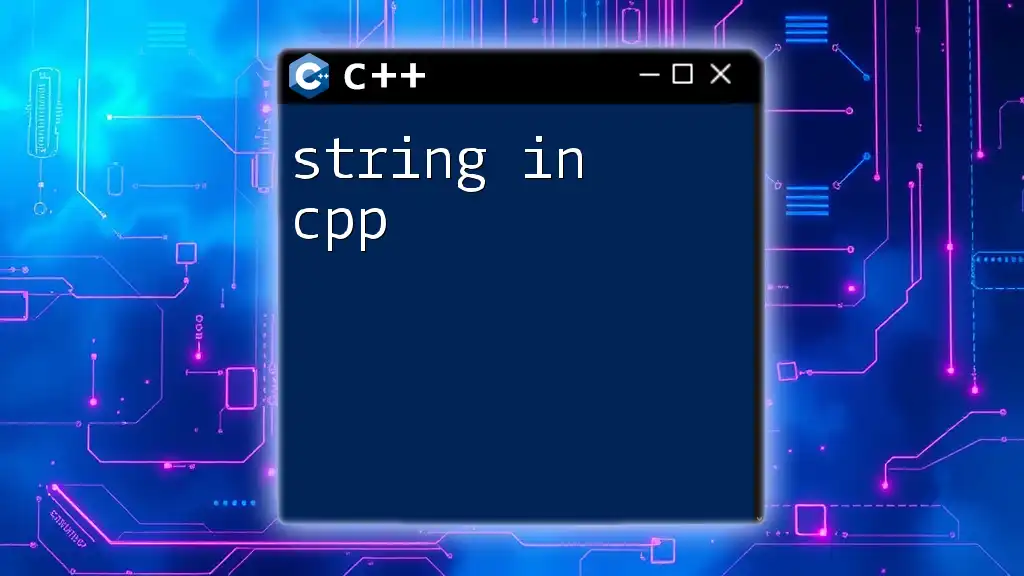
Implementing Hashing in C++
Step-by-Step Guide to Creating a Hash Table
Implementing your own hash table is a practical way to understand how hashing works. The following example demonstrates a simple hash table that stores integers.
Creating a Basic Hash Table
#include <vector>
#include <list>
#include <iostream>
class HashTable {
public:
HashTable(int size) : table(size) {}
void insert(int key) {
int index = key % table.size();
table[index].push_back(key);
}
bool search(int key) {
int index = key % table.size();
for (auto& element : table[index]) {
if (element == key) {
return true;
}
}
return false;
}
private:
std::vector<std::list<int>> table;
};
// Usage
HashTable ht(10);
ht.insert(5);
ht.search(5);
In this implementation, `HashTable` uses a vector of lists to handle entries. The `insert` function calculates the index based on the modulus operation, and the `search` function checks for the presence of a key.
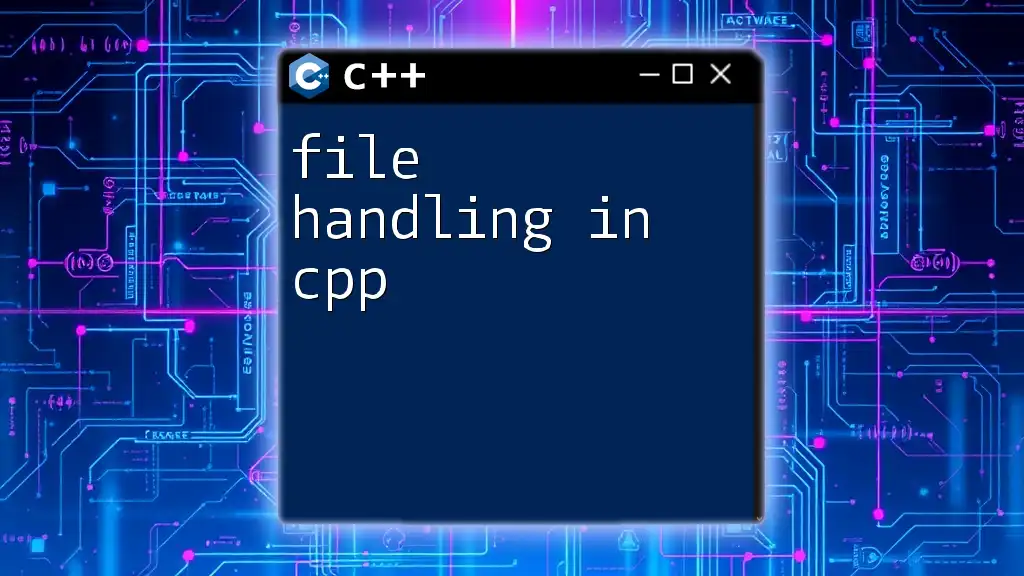
Advanced Hashing Techniques
Cryptography and Hashing
Cryptographic hashing is becoming increasingly important in today's digital world due to the growing need for data protection. C++ libraries like OpenSSL or Crypto++ allow developers to easily implement cryptographic hashing to secure sensitive information.
For example, using OpenSSL to hash a string might look like this:
#include <openssl/sha.h>
#include <iostream>
#include <iomanip>
void hashString(const std::string& input) {
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
SHA256_Update(&sha256, input.c_str(), input.size());
SHA256_Final(hash, &sha256);
std::cout << "SHA-256: ";
for (int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
std::cout << std::hex << std::setw(2) << std::setfill('0') << (int)hash[i];
}
std::cout << std::endl;
}
// Usage
hashString("Hello, World!");
This example demonstrates how to use the OpenSSL library to calculate the SHA-256 hash of a string, highlighting a practical use of cryptographic hashing.
Performance Considerations
When implementing hashing in C++, keep in mind both time and space complexity:
- Time Complexity: The average-case time complexity for insertion, deletion, and access is O(1) for hash tables. However, in the worst case, it can degrade to O(n) if many collisions occur.
- Space Complexity: Hash tables may require additional memory for pointers in lists (for chaining) or to manage probing sequences (for open addressing). Efficient memory management is crucial for performance.
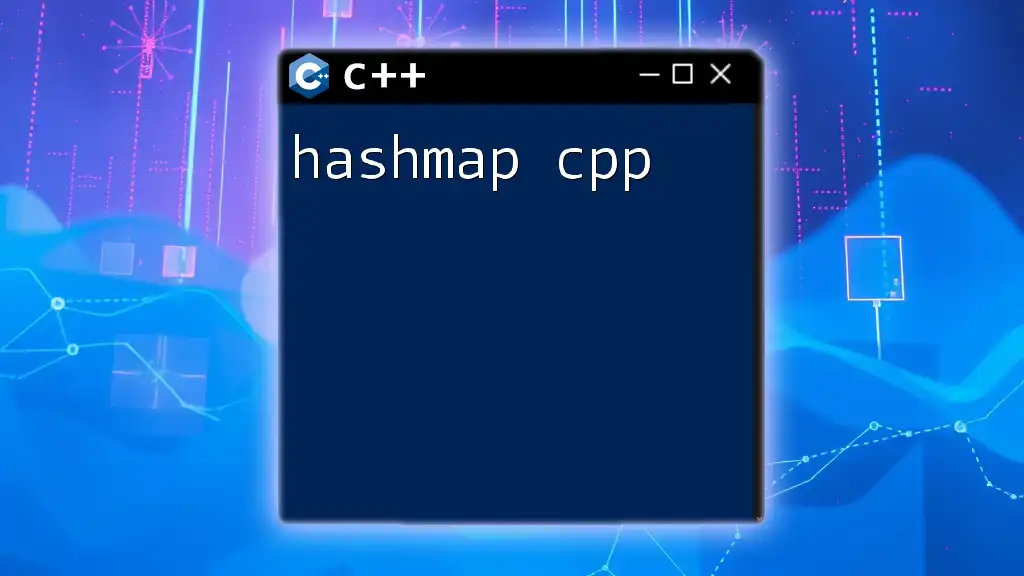
Real-World Applications of Hashing in C++
Hashing is predominantly used in various sectors and applications, including:
- Caching: By using hash tables, you can store frequently accessed data so that it can be retrieved faster.
- Data Deduplication: Hashing helps identify duplicate records by generating unique identifiers for blocks of data and comparing them.
- Password Storage and Security: Instead of storing raw passwords, applications use hashing to store hashed versions, enhancing security in case of data breaches.
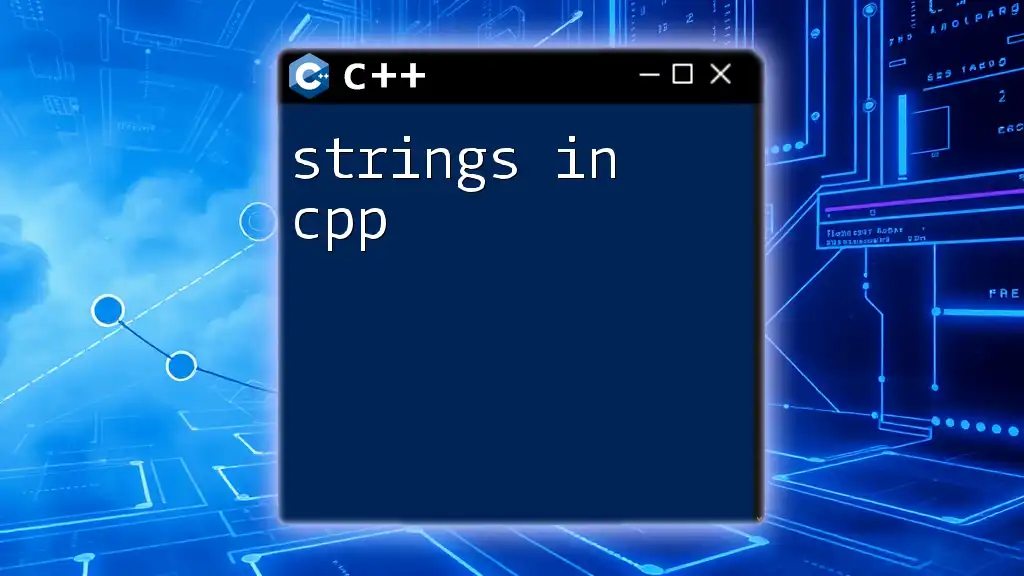
Conclusion
Hashing is an essential concept in C++ programming, impacting data retrieval, storage efficiency, and security significantly. As you continue to practice and explore the use of hashing in C++, you'll discover its versatility and power in problem-solving across various domains.
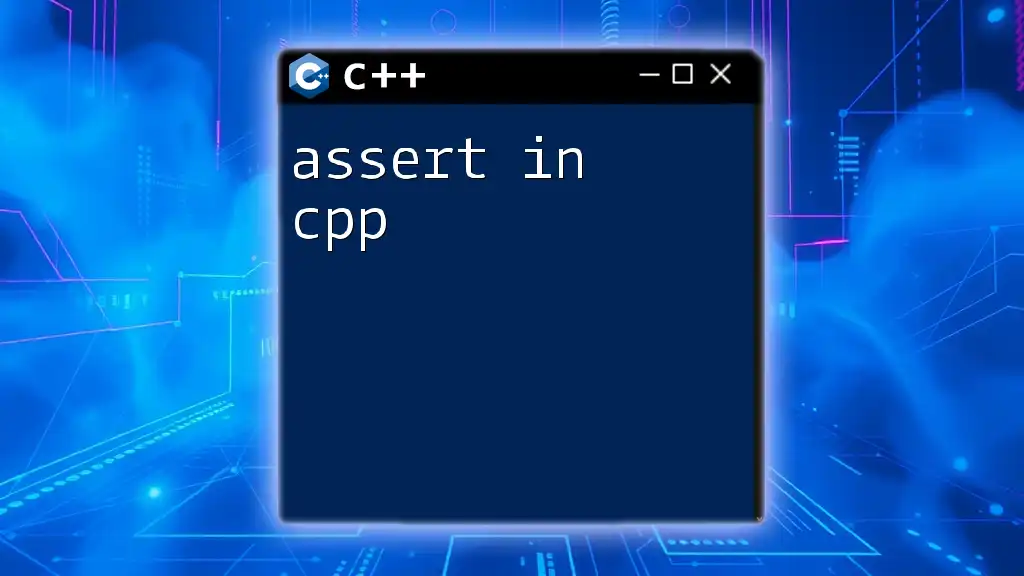
Additional Resources
To further enhance your understanding of hashing in C++, consider exploring books dedicated to algorithms and data structures, joining online programming communities, or participating in forums to address your questions and share knowledge. Additionally, look for tutorials and video courses that specifically cover hashing techniques in C++.
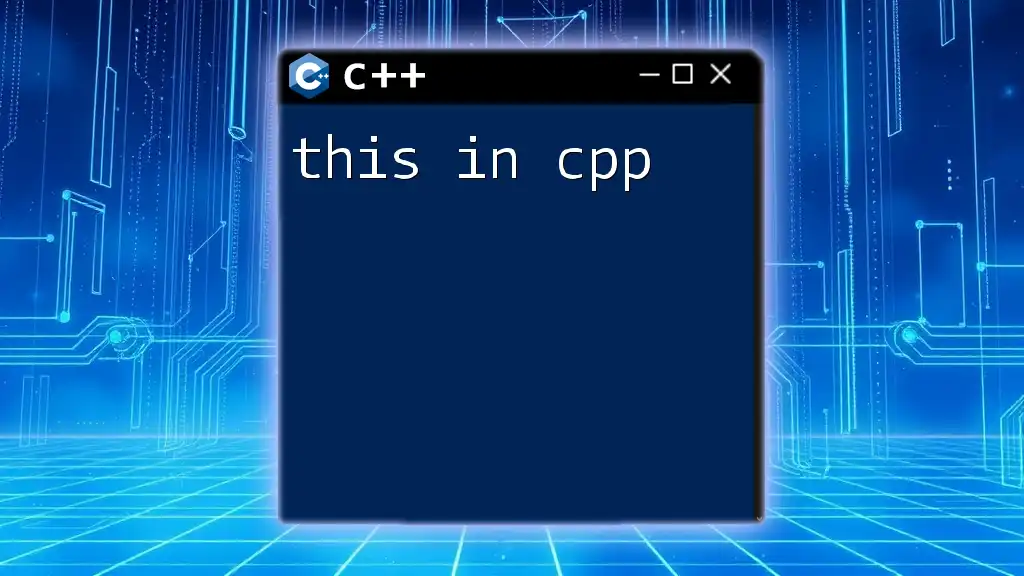
FAQs About Hashing in C++
-
What is a hash table? A hash table is a data structure that implements an associative array, mapping keys to values for efficient data access.
-
Are hash functions reversible? No, hash functions are designed to be one-way, meaning you cannot easily retrieve the original input from its hash value.
-
What is a collision in hashing? A collision occurs when two different inputs generate the same hash value, requiring strategies to handle these situations.
By familiarizing yourself with hashing in C++, you'll significantly improve your skills in writing efficient and sophisticated applications.