In C++, you can make the program pause execution for a specified duration using the `std::this_thread::sleep_for` function from the `<thread>` library.
Here's an example code snippet:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Waiting for 3 seconds..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(3));
std::cout << "Done waiting!" << std::endl;
return 0;
}
Understanding "Wait" in C++
In programming, the term "wait" refers to pausing a thread's execution until a specific condition is met or a certain amount of time passes. This concept is fundamental in various applications, such as UI interactions, scheduling tasks, syncing threads, and even managing network requests. Using wait commands in C++ allows developers to control the flow of their programs effectively, ensuring that resources are used appropriately and that processes occur in the desired sequence.
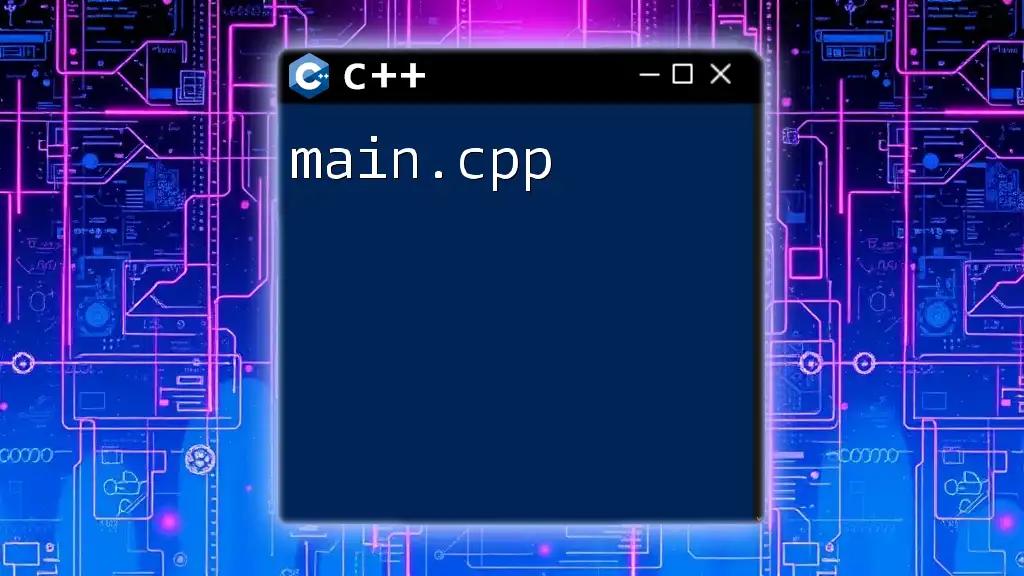
Types of Wait in C++
System Sleep
A straightforward method to make a thread pause is using system sleep. The C++ Standard Library provides several ways to implement this. The `std::this_thread::sleep_for` function is one of the most commonly used methods.
Example Usage:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Waiting for 2 seconds..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Continuing now!" << std::endl;
return 0;
}
In this example, the program outputs a message, pauses for 2 seconds, and then continues. The `sleep_for` function takes a `std::chrono` duration object, allowing you to specify the time to wait clearly.
User Input Wait
Another common wait method involves pausing the program until the user provides input. This is particularly useful in console applications where you expect user interaction.
Example Usage:
#include <iostream>
int main() {
std::cout << "Press Enter to continue...";
std::cin.get();
std::cout << "Continuing now!" << std::endl;
return 0;
}
Here, the program will stop executing until the user presses Enter. This type of wait is particularly useful in scenarios such as command-line interfaces where the program should not proceed until user input is received.
Conditional Wait (Busy Wait)
Conditional wait typically involves a situation where a thread pauses execution based on specific conditions being met. This can lead to inefficient CPU usage if not handled carefully, as busy waiting keeps checking for conditions without relinquishing control.
Example Usage:
#include <iostream>
#include <thread>
#include <chrono>
bool conditionMet = false;
void checkCondition() {
std::this_thread::sleep_for(std::chrono::seconds(5));
conditionMet = true;
std::cout << "Condition is now met!" << std::endl;
}
int main() {
std::thread conditionThread(checkCondition);
while (!conditionMet) {
std::cout << "Waiting for condition..." << std::endl;
std::this_thread::sleep_for(std::chrono::milliseconds(500));
}
conditionThread.join();
return 0;
}
In this scenario, the program runs a thread that simulates a condition being met after 5 seconds. The main thread uses a loop to check this condition every half-second, demonstrating how a busy wait works. However, employing this strategy can increase CPU load; thus, it should be utilized cautiously.
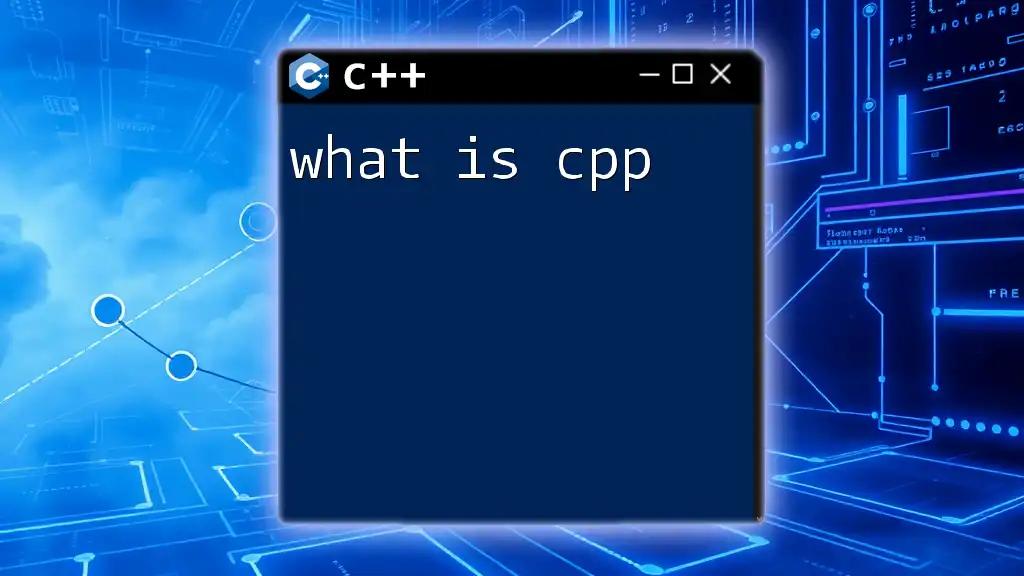
Using the C++ Standard Library for Wait Commands
`std::this_thread::sleep_for`
This function allows developers to pause the execution of a thread for a specified duration. The versatility of `sleep_for` lies in its ability to accept various time units: seconds, milliseconds, and even microseconds. This flexibility enables developers to tailor waiting durations to suit their application needs conveniently.
`std::this_thread::sleep_until`
When you want to pause until a specific point in time instead of waiting for a fixed duration, `sleep_until` becomes a handy tool.
Example Usage:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
auto now = std::chrono::steady_clock::now();
std::cout << "Sleeping for 3 seconds using sleep_until...\n";
std::this_thread::sleep_until(now + std::chrono::seconds(3));
std::cout << "Awake now!" << std::endl;
return 0;
}
In this case, the program determines the current time and then sleeps until 3 seconds later. This approach can be especially beneficial in applications requiring precise timing.

Practical Applications of Wait in C++
Animations
In graphical applications or games, wait commands are crucial for creating smooth animations. By inserting short pauses in loops, developers can control the speed at which animations are rendered, ensuring a visually appealing experience.
Network Requests
Waiting can also manage delays in network requests. For instance, when making API calls, programmers may need to incorporate wait commands to allow for response time from external servers. This prevents the application from hanging without feedback to users.
Game Development
In game development, a frequent use of wait commands is in the game loop. Each iteration of the loop may include wait commands to maintain a consistent frame rate, enabling smooth gameplay while allowing for user actions and rendering processes.
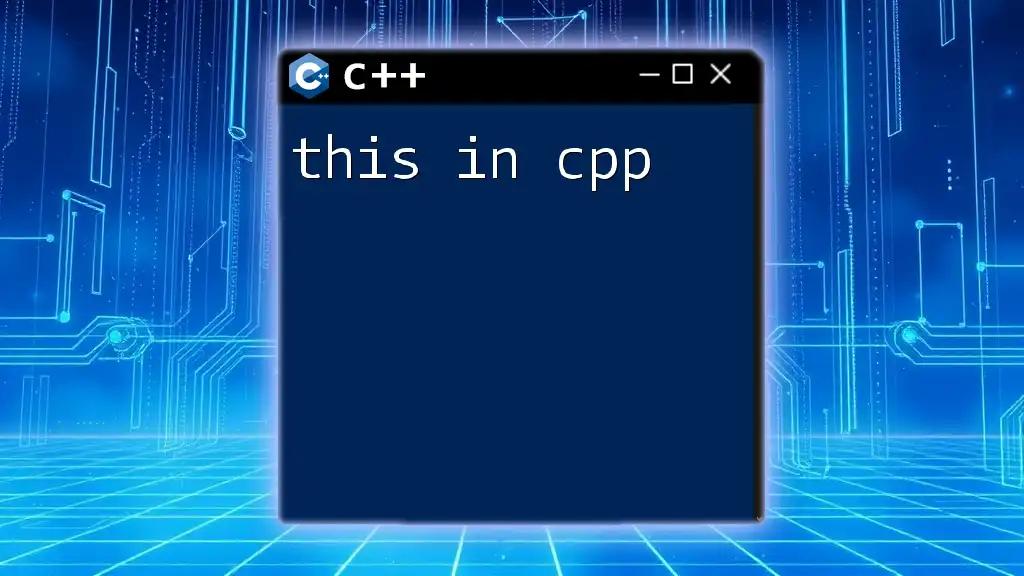
Best Practices for Using Wait in C++
When using wait commands in C++, it is vital to avoid busy waiting whenever possible, as it leads to inefficient resource usage and increased CPU load. Instead, focus on using built-in functions like `sleep_for` and `sleep_until`, which provide clean and efficient pauses.
It's essential to choose the right waiting method based on your application's requirements. Use `sleep_for` for fixed delays and `sleep_until` for more time-sensitive operations. Always ensure thread safety when implementing waiting in multi-threaded environments to prevent unexpected behavior.
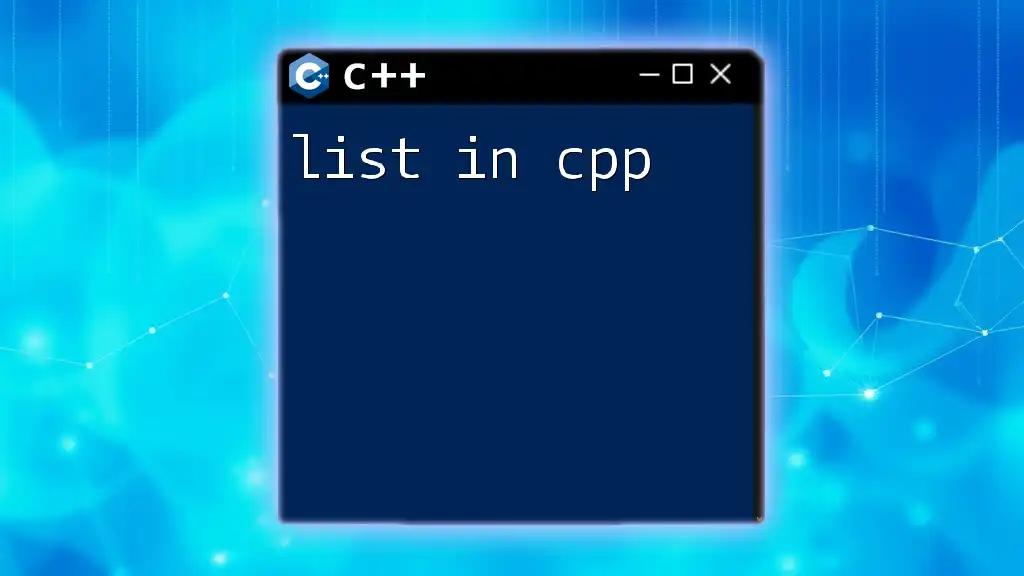
Conclusion
Understanding how to implement and utilize wait commands in C++ is crucial for maintaining the structured flow of applications. By leveraging the various C++ Standard Library functions for timing and synchronization, developers can create responsive and efficient programs that interact seamlessly with users and other systems.
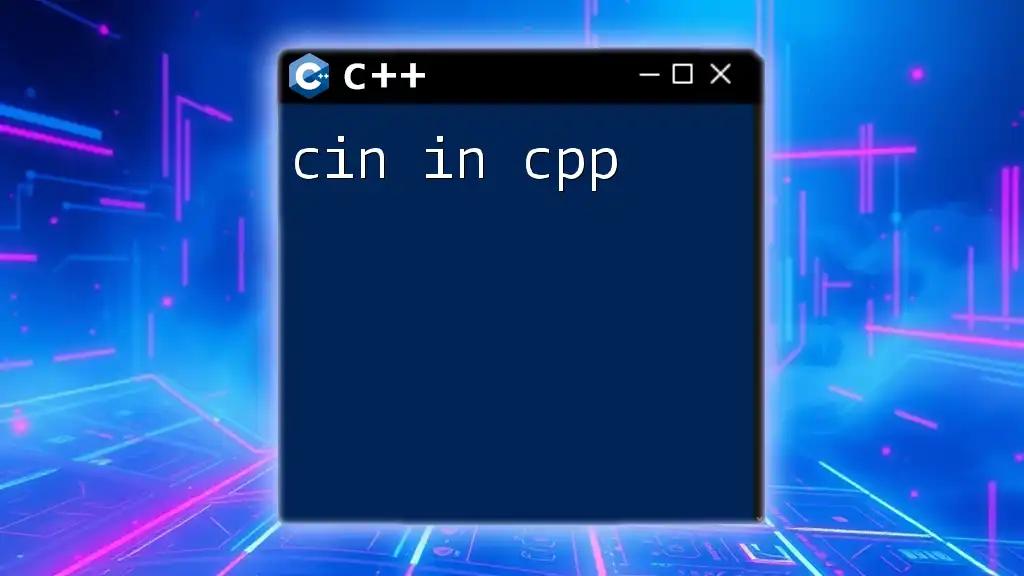
Additional Resources
For deeper exploration into C++ timing mechanisms, you may refer to the official documentation for `std::this_thread`, `chrono`, and relevant topics surrounding multithreading and time management. These resources will further enhance your understanding and application of wait commands in C++.