C++ (often referred to as cpp) is a powerful, high-level programming language that supports object-oriented, procedural, and generic programming, making it widely used for system/software development and game programming.
Here is a simple code snippet demonstrating the syntax of a basic C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful general-purpose programming language created by Bjarne Stroustrup in the early 1980s. It is an extension of the C programming language, designed to provide both low-level performance and high-level abstraction. This duality makes C++ ideal for system programming while also allowing developers to create more abstract, object-oriented programs.
History and Evolution
C++ has undergone significant evolution since its inception. It built upon the foundations of C while adding features that support object-oriented programming (OOP). Over the years, several versions of C++ have been introduced, including:
- C++98: The first standardized version of C++, which established the foundation for future improvements.
- C++11: Introduced features such as auto keyword, lambda expressions, and smart pointers, enhancing productivity and performance.
- C++14: Minor updates and bug fixes to C++11, with small improvements.
- C++17: Added new features like the optional and variant types, and more improvements to the STL (Standard Template Library).
- C++20: Includes concepts, ranges, coroutines, and enhanced support for compile-time programming.
These advancements ensure that C++ remains relevant and powerful in a fast-evolving tech landscape.
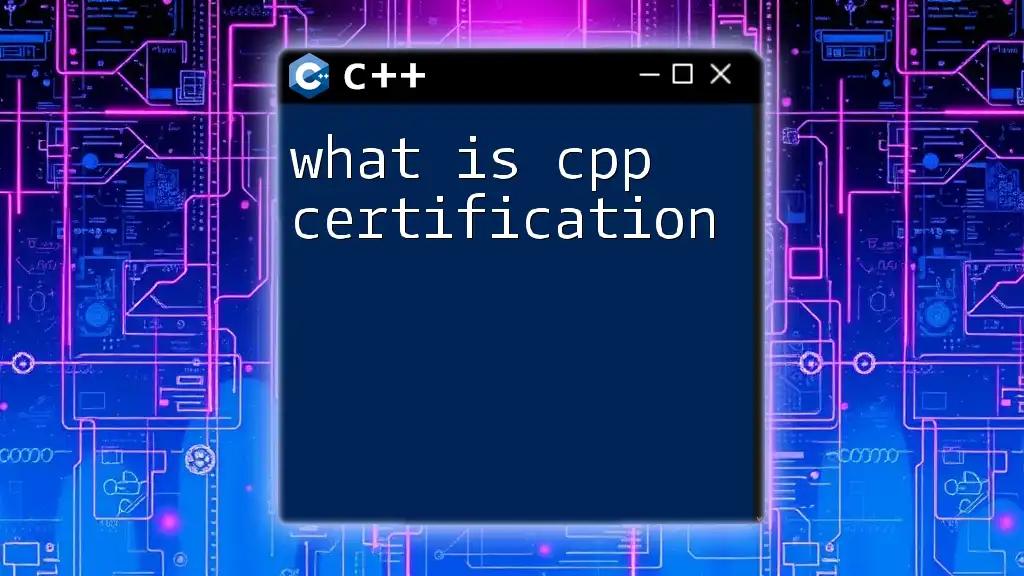
Key Features of C++
Object-Oriented Programming (OOP)
One of the most significant features of C++ is its support for object-oriented programming (OOP), which centers around four main principles:
Encapsulation
Encapsulation means bundling the data (attributes) and methods (functions) that operate on that data into a single unit, or class. This ensures that sensitive data is hidden from outside interference.
Example:
class Account {
private:
double balance; // private attribute
public:
void deposit(double amount) {
balance += amount; // method to modify the balance
}
double getBalance() {
return balance; // method to access the balance
}
};
Inheritance
Inheritance allows a class (derived class) to acquire properties and behaviors (methods) from another class (base class), promoting code reuse.
Example:
class Vehicle {
public:
void start() {
// Common start method for all vehicles
}
};
class Car : public Vehicle {
public:
void honk() {
// Car-specific method
}
};
Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon, primarily achieved through function overriding.
Example:
class Shape {
public:
virtual void draw() {
// Default draw implementation
}
};
class Circle : public Shape {
public:
void draw() override {
// Circle-specific draw function
}
};
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful feature of C++ that provides a collection of generic classes and functions. It includes:
- Containers: Data structures (e.g., vectors, lists, maps) that store collections of objects.
- Algorithms: Functions that operate on containers, like sorting and searching.
- Iterators: Objects that allow for the traversal of container elements efficiently.
Example of using a vector in STL:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " "; // Output the elements
}
return 0;
}
Memory Management
C++ provides manual memory management, granting developers control over memory allocation and deallocation, primarily through the use of `new` and `delete` keywords.
Example of manual memory management:
int* ptr = new int; // Allocating memory
*ptr = 10;
delete ptr; // Deallocating memory
To enhance safety and reduce memory leaks, C++ also offers smart pointers like `std::unique_ptr` and `std::shared_ptr`.
Example of using smart pointers:
#include <memory>
void createObject() {
std::unique_ptr<int> ptr = std::make_unique<int>(10);
// Memory is automatically freed when 'ptr' goes out of scope
}
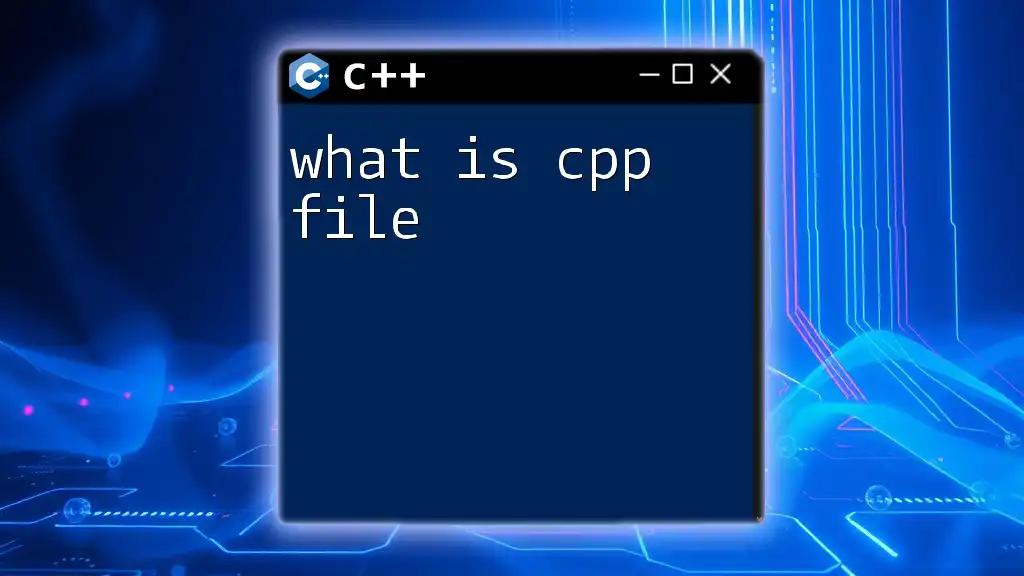
C++ Syntax and Structure
Basic Syntax
C++ syntax includes:
- Data Types: Fundamental types include `int`, `char`, `float`, and `double`.
- Variables and Constants: Variables can be modified; constants cannot.
Example:
const double PI = 3.14; // Constant declaration
int radius = 5; // Variable declaration
- Control Structures: C++ uses control structures like `if`, `switch`, and loops (for, while) to manage flow.
Example of a loop:
for (int i = 0; i < 5; i++) {
std::cout << i << " "; // Output numbers 0 to 4
}
Functions
Functions in C++ help structure code and promote reusability. They can be defined and declared, allowing for abstraction and functionality.
Inline Functions
These functions are small and can be expanded in-line to save the overhead of a function call.
inline int square(int x) {
return x * x;
}
Function Overloading
C++ allows multiple functions with the same name but different parameters (number or type).
Example:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Classes and Objects
C++ is class-based; classes define new types that encapsulate data and functions. An instance of a class is called an object.
Example of a class definition:
class Dog {
public:
void bark() {
std::cout << "Woof! Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Output: Woof! Woof!
return 0;
}
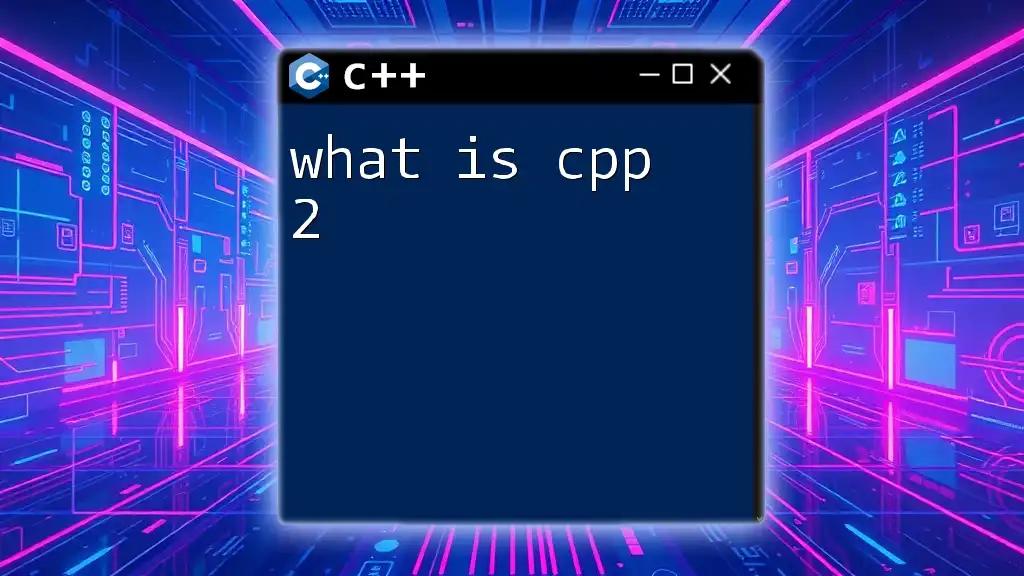
Common Uses of C++
Application Domains
C++ is prevalent in various application domains due to its performance and versatility:
- Game Development: High-performance graphics and real-time interaction make C++ popular in the gaming industry (e.g., Unreal Engine).
- Systems Programming: Operating systems often utilize C++ for its access to low-level system resources while providing high abstraction.
- Embedded Systems: C++ supports hardware-level programming, making it suitable for developing software in embedded devices.
- Web Development: Although not as common as other languages for web development, C++ can be used on the server side, especially for high-performance web applications.
Performance and Efficiency
C++ is known for its performance and efficiency. By allowing low-level memory management and minimal abstraction overhead, C++ is ideal for performance-critical applications, including high-frequency trading platforms and scientific computing.
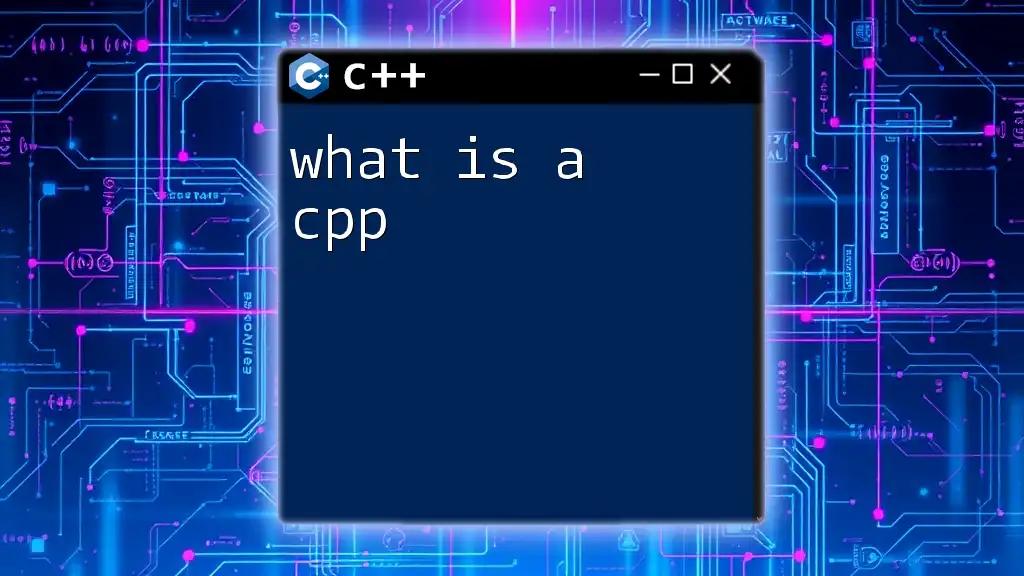
How to Get Started with C++
Setting Up the Development Environment
To begin programming in C++, set up a development environment. You can install a C++ compiler (such as GCC or Clang) and an Integrated Development Environment (IDE) like Visual Studio, Code::Blocks, or CLion. Following the installation, configure your environment by adding necessary paths and libraries.
Writing Your First C++ Program
Once your environment is set up, you can write your first C++ program. A simple "Hello, World!" example is a great starting point.
Example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Compile and run this code to see the output and understand the compilation process.
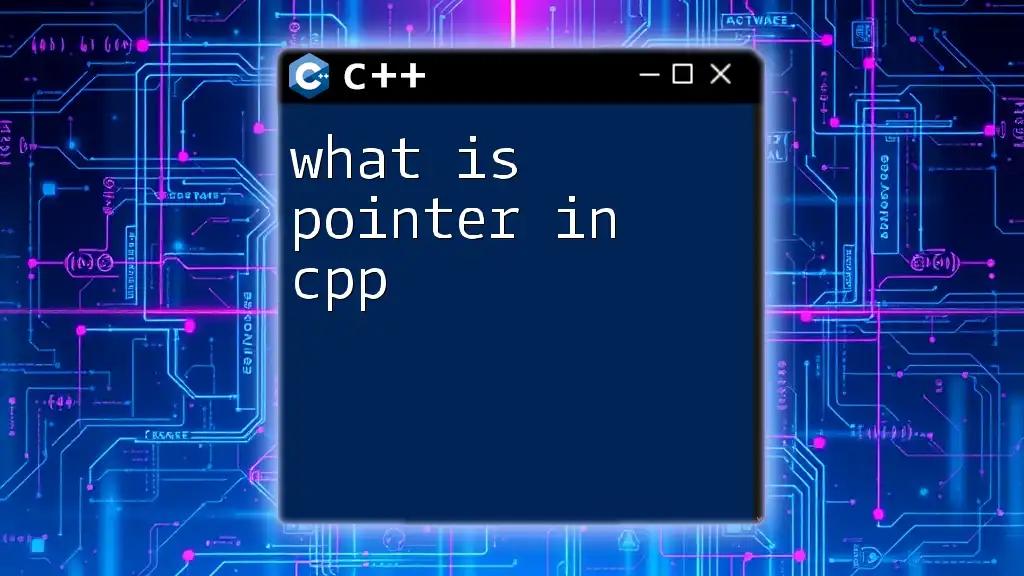
Resources for Learning C++
Online Courses and Tutorials
To deepen your understanding of C++, consider reputable online platforms, where structured courses are available. Websites like Coursera, Udacity, and Codecademy offer comprehensive resources.
Books on C++
Books can provide in-depth knowledge and insights from industry experts. Some recommended titles include:
- The C++ Programming Language by Bjarne Stroustrup
- Effective C++ by Scott Meyers
Community and Forums
Engaging with the C++ community can vastly improve your learning experience. Explore forums such as Stack Overflow or Reddit's r/cpp to ask questions, share knowledge, and network with other developers.
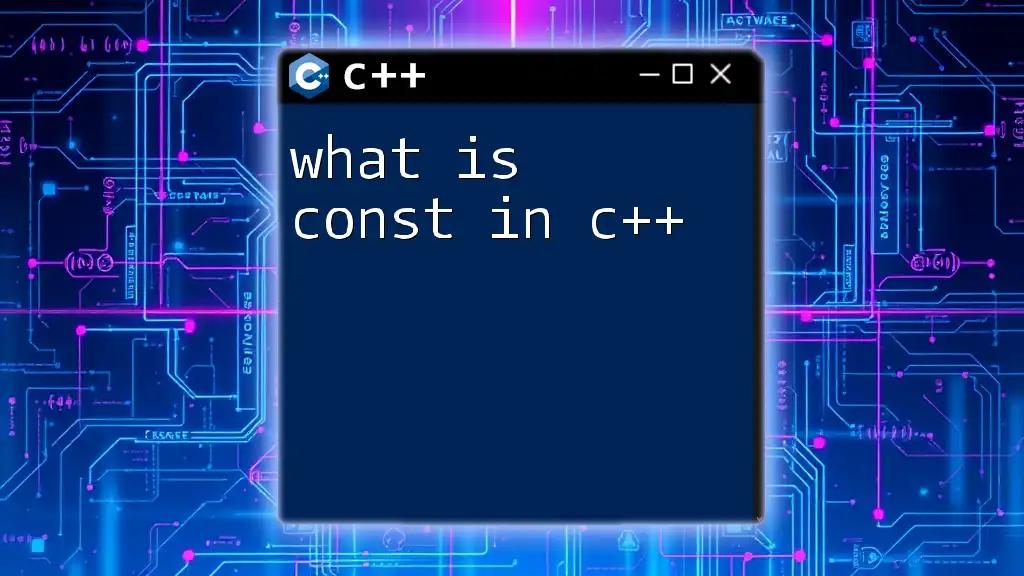
Conclusion
In summary, understanding what is C++ is pivotal for anyone interested in programming. C++ combines the performance of low-level programming with high-level abstractions, making it a versatile language suitable for various applications. With its powerful features, rich history, and widespread use, C++ is a language worth mastering.
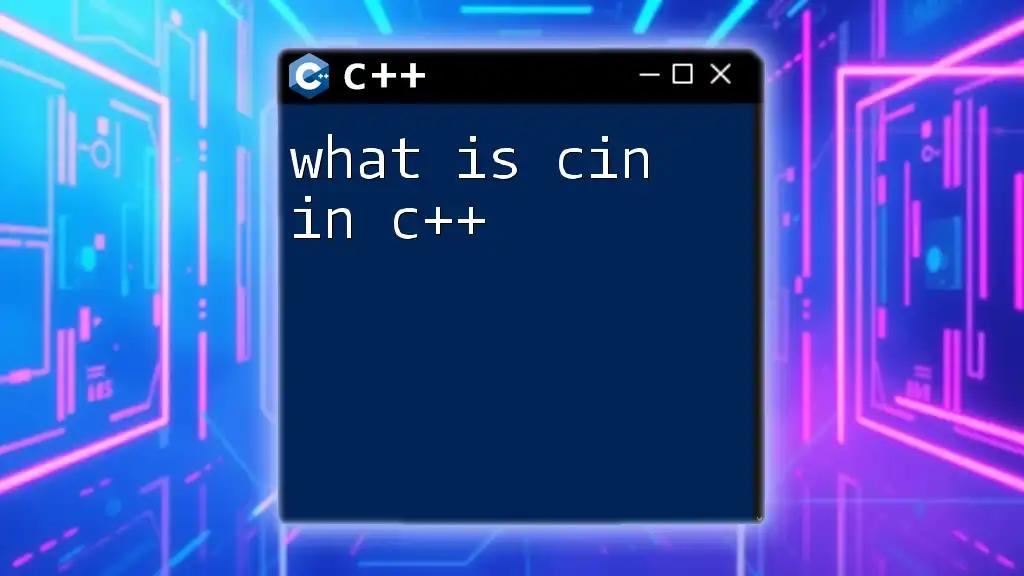
Call to Action
If you're eager to delve deeper into C++ programming and learn how to use its commands quickly and concisely, follow us for more tutorials and tips! Start your journey today and unlock the full potential of C++.