Microsoft C++ is a programming language and development environment provided by Microsoft that allows developers to create applications using the C++ programming language, leveraging its features alongside the Windows operating system’s capabilities.
Here’s a simple code snippet demonstrating a "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a general-purpose programming language that has been crucial in the development of modern software. Originating from the early 1980s, it was created by Bjarne Stroustrup at Bell Labs, primarily as an enhancement to the C programming language by adding object-oriented features. Over the decades, C++ has undergone numerous revisions, evolving through standards such as C++98, C++11, C++14, C++17, and C++20. This evolution showcases its adaptability and continued relevance in software engineering.
The importance of C++ lies in its performance, versatility, and ability to leverage both high-level and low-level programming features. It is widely used in fields ranging from systems programming and game development to high-performance applications like simulations and real-time systems.
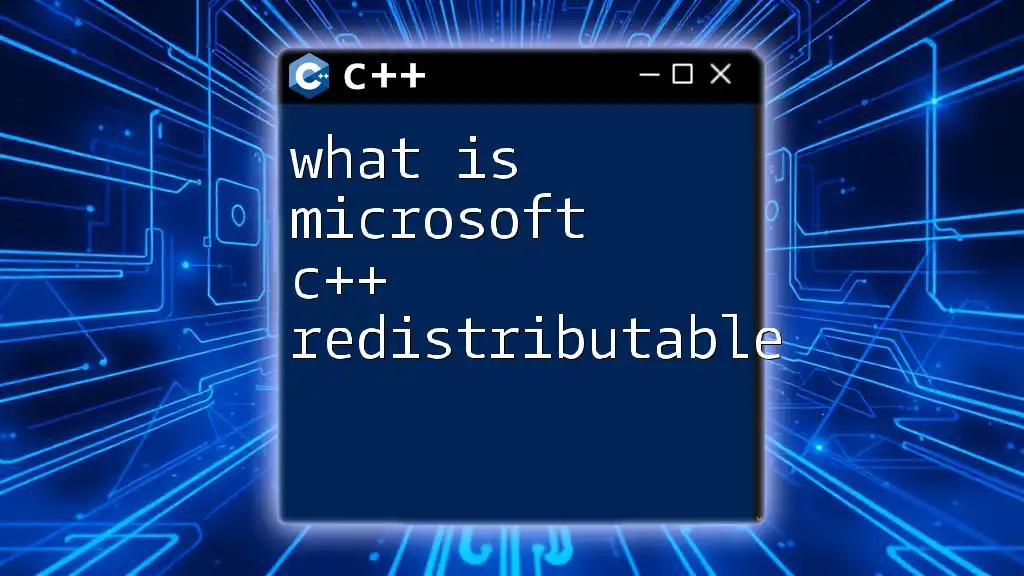
Understanding Microsoft C++
Microsoft C++ refers to Microsoft's implementation of the C++ programming language, primarily through its Visual Studio IDE. Visual Studio is a powerful development environment that simplifies the process of writing, debugging, and compiling C++ applications for Windows.
Microsoft C++ Compiler
At the heart of Microsoft’s C++ offering is the Microsoft Visual C++ (MSVC) compiler. This compiler is tailored for Windows applications and is designed to optimize performance while maintaining compatibility across different C++ standards. The key features of MSVC include:
- Optimization levels: MSVC enables developers to choose optimization settings that help create fast and efficient applications.
- Compatibility with C++ standards: It supports the latest features from various C++ standards, allowing developers to use the modern constructs that enhance code readability and maintainability.
To illustrate how the MSVC compiler works, consider writing a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, Microsoft C++!" << std::endl;
return 0;
}
In this example, the `#include <iostream>` statement brings in the standard input-output library, enabling the use of `std::cout` for sending output to the console. Compiling this program using MSVC is straightforward and allows you to see immediate results.
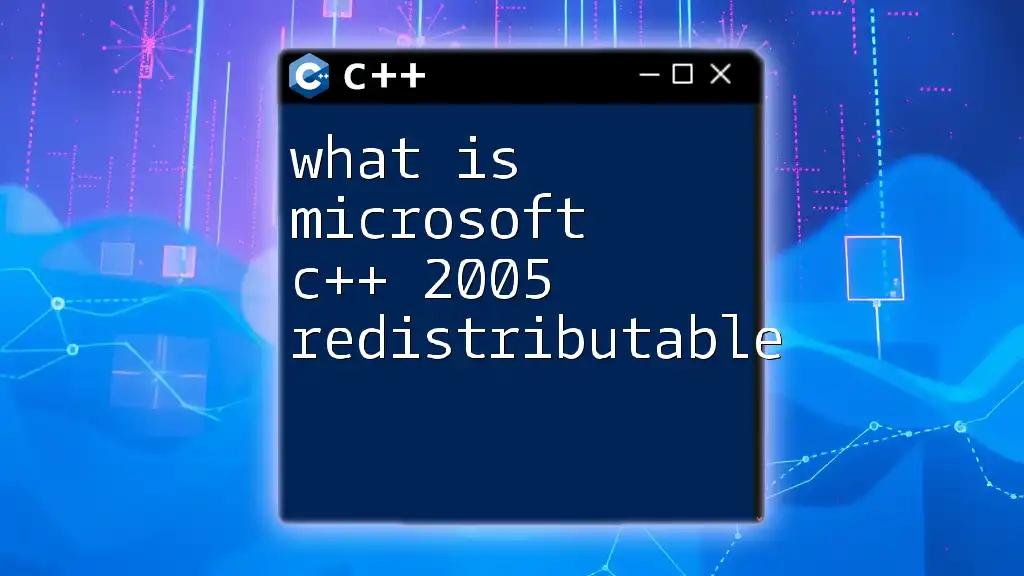
Key Features of Microsoft C++
Integrated Development Environment (IDE)
Visual Studio is more than just a compiler; it is an integrated development environment that offers a multitude of tools to streamline the development process. Some notable features include:
- Intellisense: This has become a game-changer for many developers, as it provides real-time code suggestions and autocompletion, allowing programmers to write code more efficiently.
- Debugger: The debugging tools in Visual Studio are comprehensive, enabling developers to diagnose issues effectively by setting breakpoints, stepping through code, and monitoring variable values.
- Project Management: Visual Studio simplifies the management of multiple files and dependencies, helping developers stay organized, especially in larger projects.
Libraries and Frameworks
Microsoft C++ provides access to a plethora of libraries and frameworks that empower developers to build powerful applications efficiently.
-
Standard Template Library (STL): The STL is a vital part of C++ development, including widely-used data structures like vectors and lists, as well as algorithms for sorting and searching. Its flexibility and efficiency have made it a preferred choice for many C++ developers.
-
Microsoft Foundation Classes (MFC): MFC is particularly valuable for developers looking to create Windows GUI applications. It encapsulates Windows API calls into C++ classes, simplifying the process of building user interfaces.
-
Windows API: Microsoft C++ gives direct access to the Windows Application Programming Interface, allowing developers to interact with various system-level resources and create native Windows applications.
Compatibility and Portability
Microsoft C++ also focuses on compatibility and portability. It enables developers to create applications that can run across various Windows versions and architectures, including x86 and x64. Moreover, through careful management of dependencies and using portable libraries, developers can strive for cross-platform functionality, paving the way for applications that reach a broader audience.
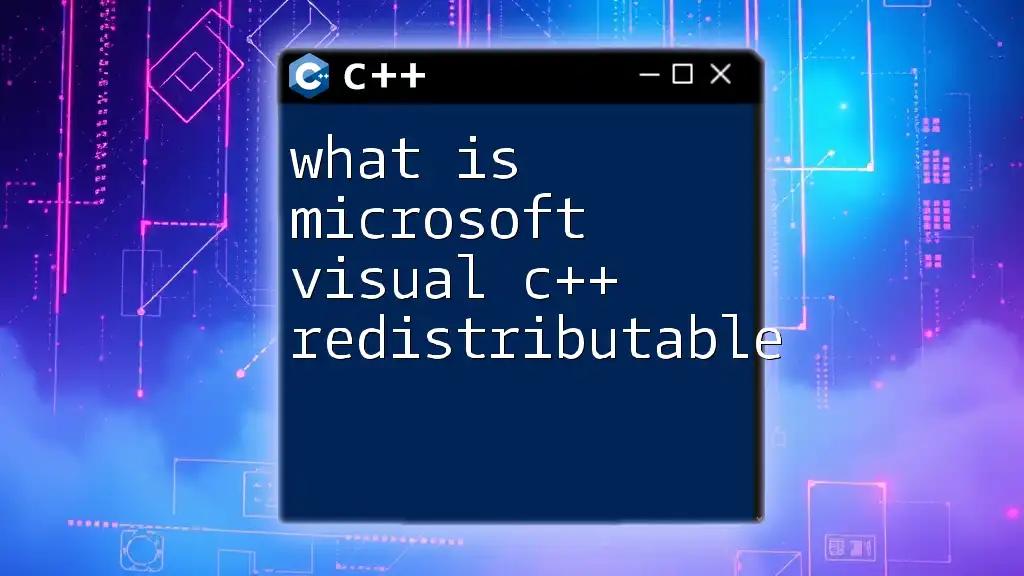
Getting Started with Microsoft C++
Setting Up Your Environment
To begin your journey with Microsoft C++, the first step is to set up your development environment. Here’s how to get started:
-
Downloading Visual Studio: Navigate to the official Microsoft website and download the latest version of Visual Studio. Choose the community edition if you’re just starting; it’s free for individual developers.
-
Creating Your First Project: Once installed, launch Visual Studio, select “Create a new project,” and choose the C++ project template that suits your needs, like a console application.
Writing and Running Your First C++ Program
When you create your project, you’ll encounter a convenient code structure. A simple C++ program in Visual Studio typically includes a `main` function, which serves as the entry point for execution. Here’s another example to emphasize the structure:
#include <iostream>
int main() {
std::cout << "Welcome to Microsoft C++ Programming!" << std::endl;
return 0;
}
Once your program is written, you can compile and run it within Visual Studio, allowing you to see your output in real-time through the integrated terminal.

Best Practices for Learning C++
Effective Learning Strategies
To become proficient in Microsoft C++, consider implementing the following strategies:
-
Practice Regularly: Consistent practice is key to mastering any programming language. Work on small projects, solve coding challenges, and experiment with new features.
-
Utilize Resources: A wealth of information is available through books, official documentation, and online courses. Explore platforms like Coursera, Udacity, or YouTube tutorials that focus on C++ and Microsoft technologies.
-
Participate in Communities: Engage in forums like Stack Overflow, GitHub, or C++ user groups to connect with other developers. Sharing your knowledge and seeking help can greatly enhance your learning experience.
Common Pitfalls
While learning, be aware of common pitfalls that can hinder your progress:
-
Memory Management: C++ gives you fine control over memory, but it also places the onus of memory management on you, the developer. Be cautious with pointers and dynamic memory allocation to avoid memory leaks and segmentation faults.
-
Error Handling: Emphasizing proper exception handling strategies will help your applications run more smoothly and provide users with better feedback in the case of errors.

Conclusion
In summary, Microsoft C++ is a robust platform for developing Windows applications, combining the power of the C++ language with the comprehensive capabilities of Visual Studio. With its extensive features, libraries, and community support, learning Microsoft C++ can open doors to a wealth of opportunities in software development. Whether you are a beginner or an experienced programmer, the journey through Microsoft C++ can enrich your skill set and expand your programming horizons.

Additional Resources
To further your knowledge of Microsoft C++, consider the following resources:
-
Official Documentation: The Microsoft Official Documentation is an invaluable resource for understanding the intricacies of Visual C++ and C++ as a whole.
-
Online Courses: Platforms like Coursera and Udacity offer structured learning paths that cover both basic and advanced C++ concepts.
-
Books: Classic texts and contemporary books on C++ programming can deepen your understanding and provide advanced techniques for software design.
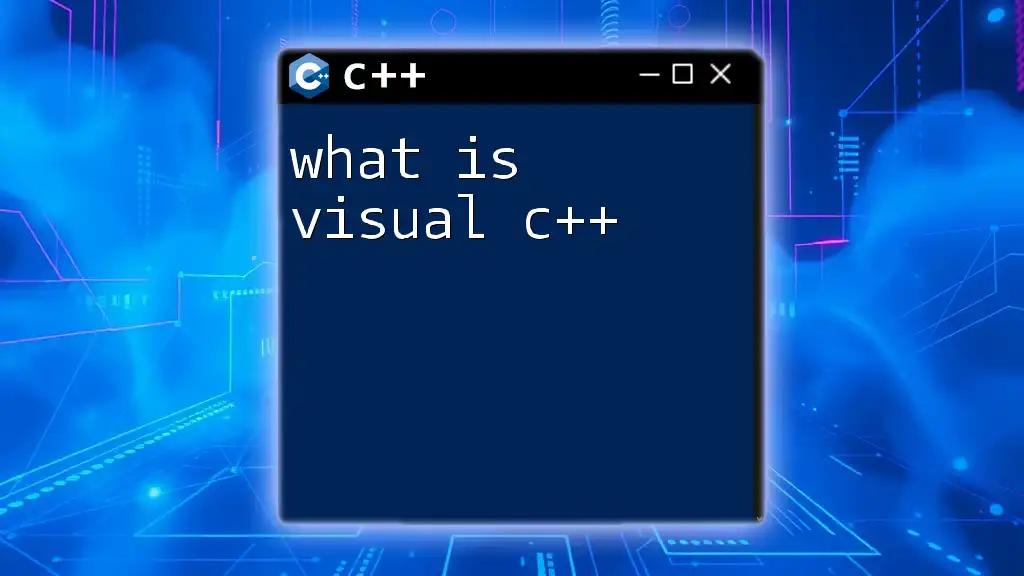
FAQs
-
What distinguishes Microsoft C++ from other C++ compilers?
Microsoft C++ offers unique features through MSVC and Visual Studio, such as advanced debugging tools and a friendly IDE environment that enhances productivity. -
Is Microsoft C++ free to use?
Yes, the Community edition of Visual Studio, which supports Microsoft C++, is free for individual developers, open-source projects, and small teams. -
What types of applications can be built with Microsoft C++?
Developers can create a wide range of applications, including desktop applications, games, system utilities, and high-performance applications that require efficient resource management.