In C++, `this` is a special pointer that refers to the instance of the class that is currently being manipulated or accessed within a member function.
Here's a code snippet demonstrating the use of `this`:
#include <iostream>
class MyClass {
public:
int value;
MyClass(int val) : value(val) {}
void display() {
std::cout << "Value: " << this->value << std::endl; // Using 'this' to refer to the member variable
}
};
int main() {
MyClass obj(10);
obj.display(); // Output: Value: 10
return 0;
}
Understanding 'this' in C++
In C++, the keyword `this` is a special pointer available inside non-static member functions of a class. It serves several crucial roles in maintaining clarity and integrity of code. Let's dive into the details of this vital component of C++.
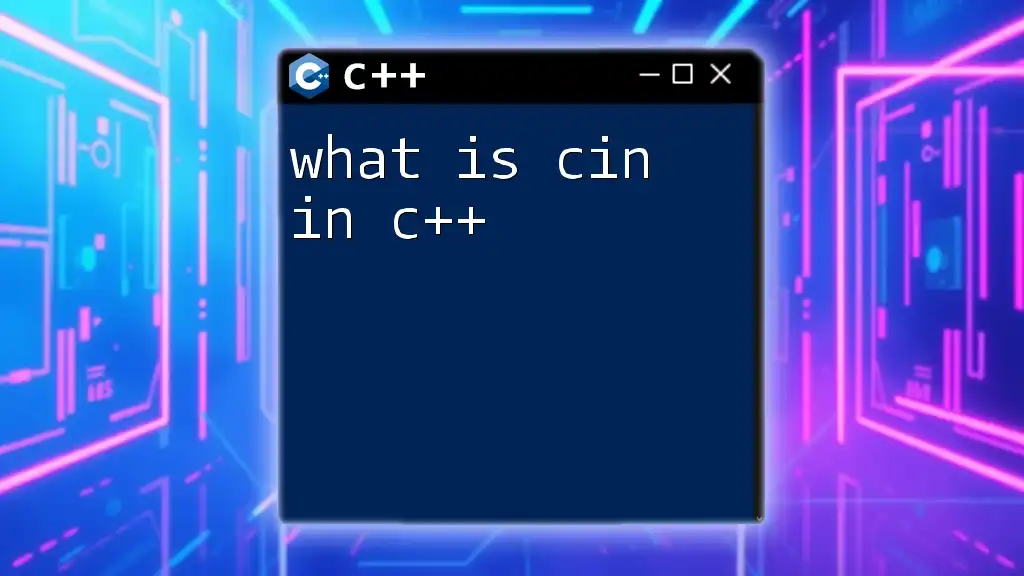
The Context of 'this'
Using 'this' in Member Functions
When a member function is invoked on an object of a class, `this` points to the object that called the function. This means that when you write code within that function, you can access the object's members using `this`, which helps eliminate ambiguity.
For example, consider the following class definition:
class MyClass {
public:
void display() {
std::cout << "Object address: " << this << std::endl;
}
};
In this example, when the `display` function is called, `this` provides the address of the instance invoking the method, allowing you to refer to the specific object directly.
The 'this' Pointer as a Way to Access Calling Object
Often, parameter names may clash with member variable names. In such cases, `this` assists in resolving these conflicts. By using `this`, you can clearly indicate that you are referencing the member variable rather than the parameter.
Here is an illustrative code snippet:
class MyClass {
int value;
public:
MyClass(int value) {
this->value = value; // differentiating member variable from parameter
}
};
In this constructor, using `this->value` makes it clear that we are assigning the parameter `value` to the member variable `value`.
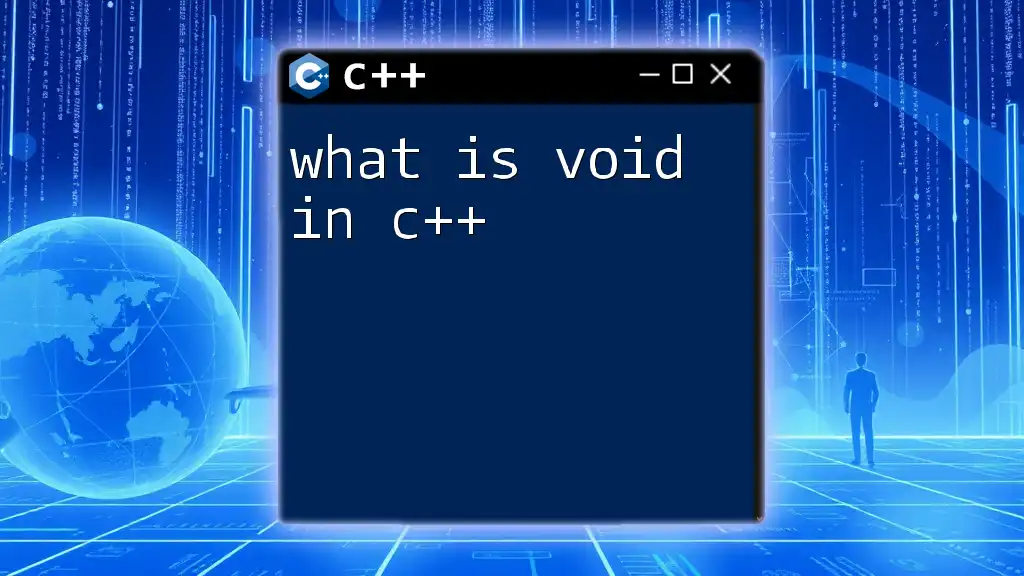
Implicit Type of 'this'
The 'this' Pointer Type
It is also crucial to understand the type of `this`. Inside a member function, `this` is implicitly converted to a pointer type of the class being defined. For instance, in `MyClass`, `this` is equivalent to `MyClass*`. Understanding this type can prevent confusion, especially when dealing with overloaded functions or templates.
Types of Functions: Const and Non-Const
The interpretation of `this` varies in const and non-const member functions.
In a const member function, `this` points to a const object. Hence, you cannot modify the object's members directly:
class MyClass {
public:
void show() const {
std::cout << "Address is: " << this << std::endl; // 'this' is of type const MyClass*
}
};
This distinction is significant for maintaining const-correctness in your codebase, which ultimately enhances safety and predictability.
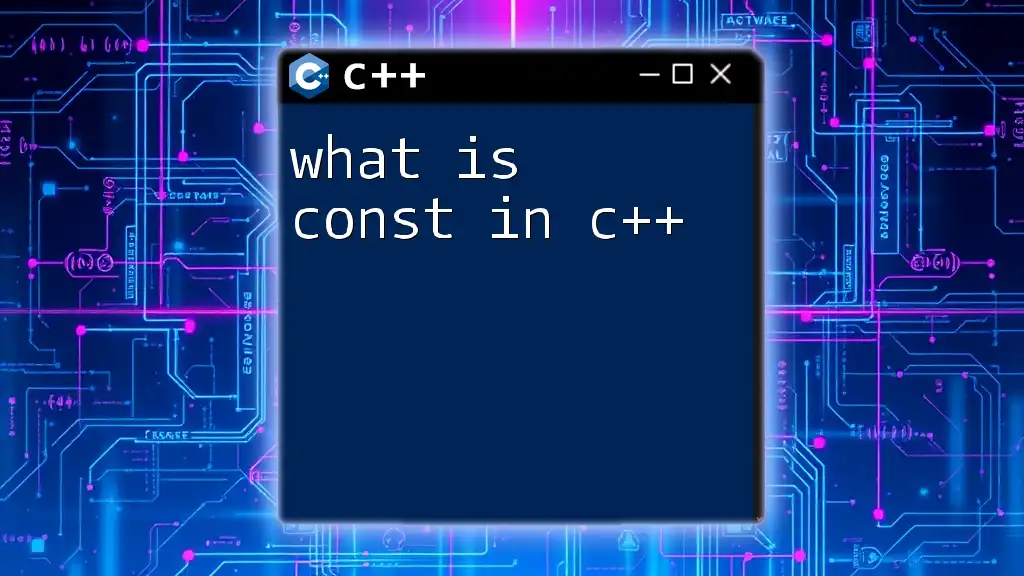
Special Cases Using 'this'
Returning *this from Member Functions
Chaining multiple method calls can enhance code readability and fluidity. When you return `this` from a member function, you enable such chaining. The following example illustrates how to use `this` to facilitate method chaining:
class MyClass {
int value;
public:
MyClass* setValue(int value) {
this->value = value; // returning 'this' for chaining
return this;
}
};
In this example, after setting the value, the function returns a pointer to the same object, allowing for calls like `obj.setValue(5)->setValue(10);`.
'this' in Operator Overloading
When overloading operators, `this` becomes essential for defining operator behavior. This is particularly true for assignment operators where managing self-assignment is crucial to prevent unintended results.
Consider the following code snippet:
class MyClass {
int value;
public:
MyClass& operator=(const MyClass& other) {
if (this != &other) { // checking for self-assignment
this->value = other.value;
}
return *this; // returning *this for chaining
}
};
Here, `this` is used to check if the object is being assigned to itself, protecting the integrity of your class.
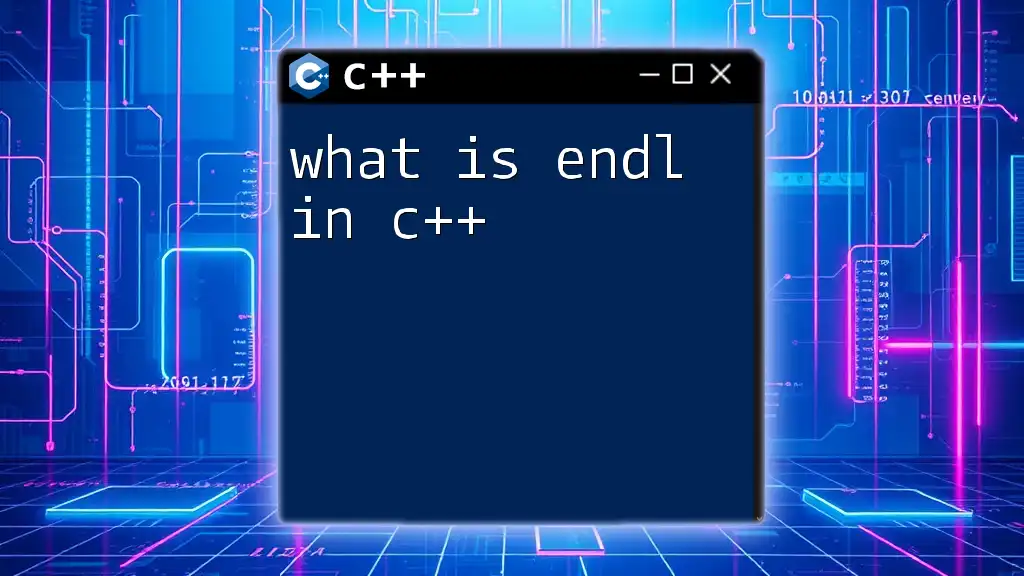
Common Mistakes Involving 'this'
Though `this` appears straightforward, improper usage can lead to subtle bugs. A common mistake occurs in operator overloads or assignment functions where the programmer forgets to check for self-assignment. This can lead to unexpected behavior, especially if your class manages resources like dynamic memory. Implementing `this` checks is fundamental to avoiding such errors.
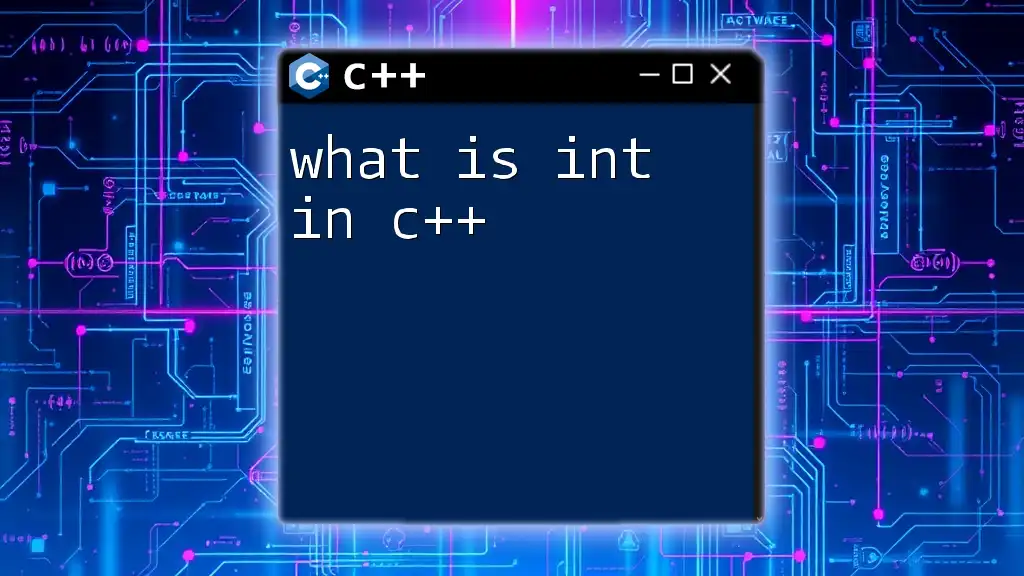
Conclusion
Understanding `this` in C++ is integral to mastering object-oriented programming. By clarifying context and enabling method chains, `this` significantly enhances the functionality and readability of your code. The concepts explored above establish a robust foundation for effectively using `this` in your projects.
Be sure to experiment with various examples and utilize resources available to deepen your understanding of this crucial part of C++. Embracing the full scope of `this` will undoubtedly benefit your programming endeavors.