In C++, the right shift operator (`>>`) shifts the bits of a number to the right, effectively dividing the number by 2 for each shift position.
Here's a code snippet demonstrating the right shift operation:
#include <iostream>
int main() {
int number = 16; // 0001 0000 in binary
int shifted = number >> 2; // Right shift by 2 positions
std::cout << "Result: " << shifted << std::endl; // Outputs: 4
return 0;
}
Understanding the C++ Shift Operators
What Are Shift Operators?
Shift operators in C++ allow programmers to manipulate the bits of an operand directly. They are primarily used for bitwise operations, which are crucial in systems programming, performance optimization, and hardware control. In C++, there are two types of shift operators:
- Left Shift (`<<`): Shifts bits to the left, filling vacated bits with zeros. This operator effectively multiplies the operand by powers of two.
- Right Shift (`>>`): Shifts bits to the right. This is what we will focus on in this article.
The Right Shift Operator in C++
The right shift operator (`>>`) works by shifting each bit of the binary representation of its operand to the right by a specified number of positions. The syntax is straightforward:
result = value >> n;
In this syntax:
- `value` is the integer whose bits will be shifted.
- `n` specifies the number of positions to shift the bits to the right.
- `result` stores the outcome of the operation.
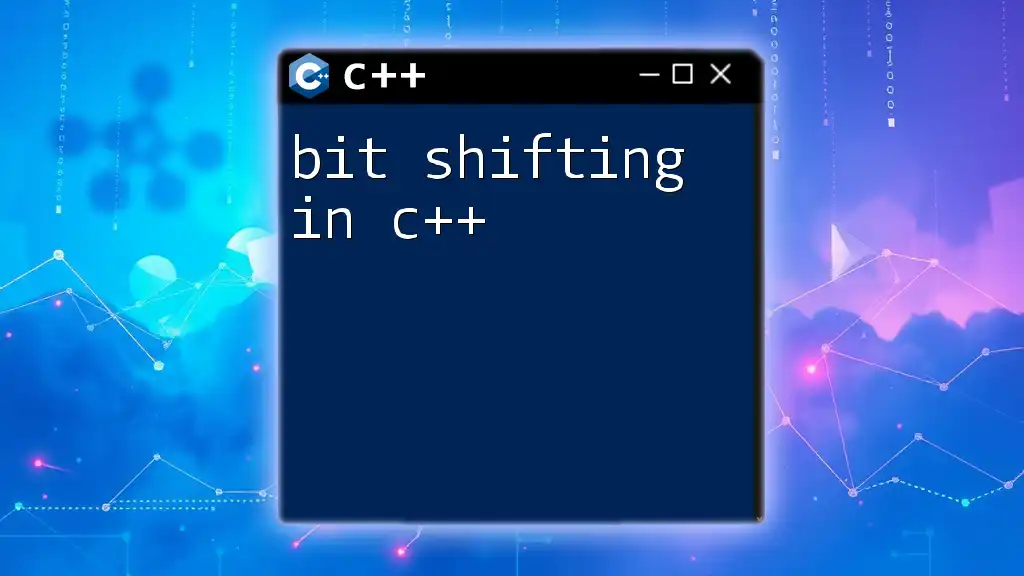
Types of Right Shift Operations
Arithmetic Right Shift
When performing an arithmetic right shift, the sign bit is preserved. This means that if the leftmost bit (sign bit) is 1 (indicating a negative number in two's complement representation), it will fill the vacated bits on the left with 1s. Conversely, if the leftmost bit is 0, zeros will fill the vacated bits.
For example:
int x = -8; // In binary: 11111111 11111111 11111111 11111000
int result = x >> 1; // Result will be -4
In this case, shifting `-8` to the right by one position results in `-4`, with the sign bit maintained as 1.
Logical Right Shift
Logical right shift ignores the sign bit and simply fills vacated bits with zeros. This is applicable primarily to unsigned integers.
For example:
unsigned int y = 8; // In binary: 00000000 00000000 00000000 00001000
unsigned int result = y >> 1; // Result will be 4
Here, `8` is shifted to the right by one position, resulting in `4`, with no regard for a sign bit since it is an unsigned integer.
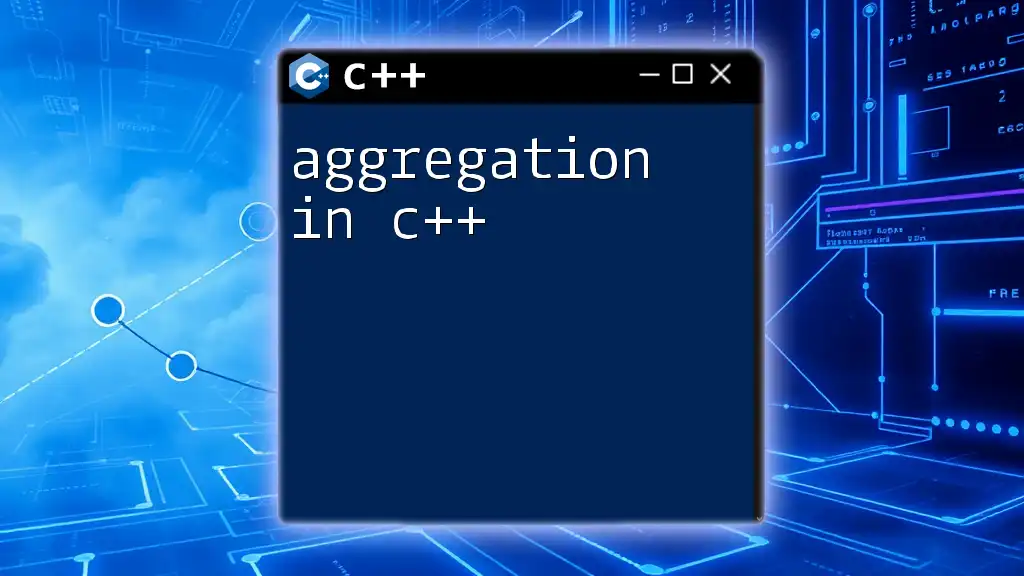
How the Right Shift Operator Works
Bit Manipulation
When you apply the right shift operator to an integer, each bit in the binary representation of that integer is shifted right by `n` positions. For example, shifting the binary number `00000010` (which is `2` in decimal) by one position results in `00000001` (which is `1` in decimal).
The following illustration provides a comprehensive overview:
- Original: `00000010` (2)
- After Right Shift (by 1): `00000001` (1)
- After Right Shift (by 2): `00000000` (0)
Impact on Signed vs. Unsigned Integers
The behavior of the right shift operator differs significantly based on whether you are working with signed or unsigned integers.
For signed integers, the arithmetic right shift preserves the sign bit:
int signed_example = -4; // Binary representation: 11111111 11111111 11111111 11111100
int result = signed_example >> 1; // Result will be -2 (sign bit preserved)
For unsigned integers, the logical right shift will always fill the leftmost bits with zeros:
unsigned int unsigned_example = 4; // Binary representation: 00000000 00000000 00000000 00000100
unsigned int result = unsigned_example >> 1; // Result will be 2
It is crucial to recognize this distinction to avoid unexpected results in your calculations.
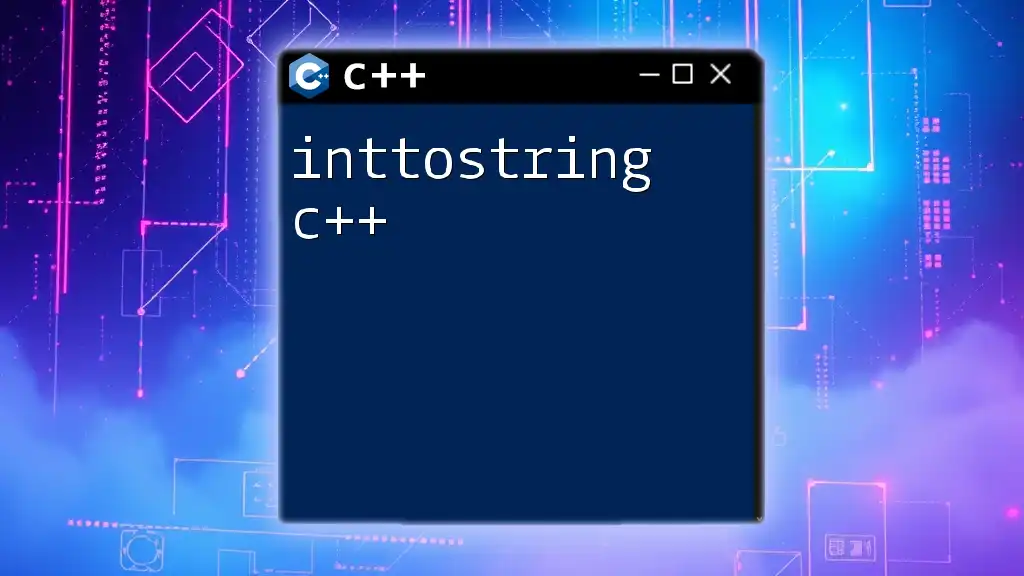
Practical Applications of Right Shift in C++
Efficient Division by Powers of Two
One of the most common uses for the right shift operator is to perform division by powers of two. Instead of using division, which is generally slower, you can effectively utilize right shifts for improved performance.
For instance:
int number = 32;
int half = number >> 1; // Equivalent to number / 2
In this example, shifting `32` right by one position yields `16`, effectively dividing it by `2`.
Masking Operations
The right shift operator can also be used in masking operations, particularly when you want to isolate specific bits. Combining the shift operator with bitwise `AND` allows for efficient data extraction.
For example:
int value = 0xFF00; // Binary representation: 11111111 00000000 00000000 00000000
int masked_result = (value & 0x00FF) >> 1; // Result is shifted to the right
In this case, the masking operation helps isolate the lower byte, and shifting it right helps compute the appropriate result.
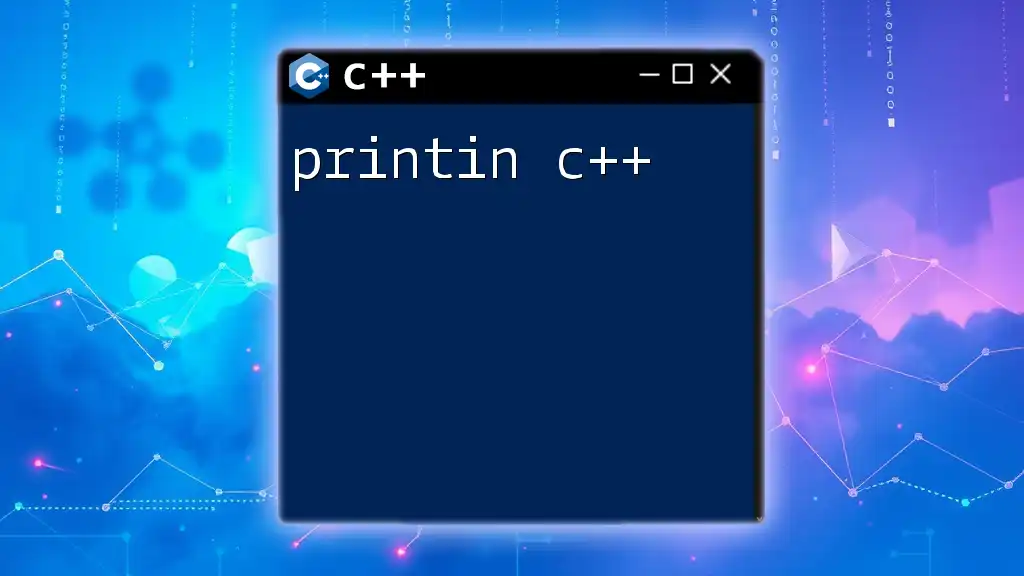
Common Mistakes Using the Right Shift Operator
Confusing Arithmetic and Logical Shifts
A prevalent mistake among programmers is confusing arithmetic with logical right shifts. This often leads to unexpected values, particularly when dealing with negative integers.
An example of this mistake is as follows:
int negative_value = -1; // Binary representation: 11111111 11111111 11111111 11111111
// Using >> will perform an arithmetic shift
int wrong_result = negative_value >> 1; // Result will still be -1 (due to sign extension)
To prevent such issues, always be mindful of whether you are working with signed or unsigned types and use the appropriate shift operator.
Overlooking Data Type Implications
Another common pitfall is neglecting the impact of data types when using the right shift operator. The size of the data type can alter the behavior of the shift due to the way bits are represented.
For example:
int small_value = 1;
char small_shift = small_value >> 1; // Result is 0 but may - risk of overflow
Always ensure that you are conscious of the data types involved to avoid potential data loss or errors.
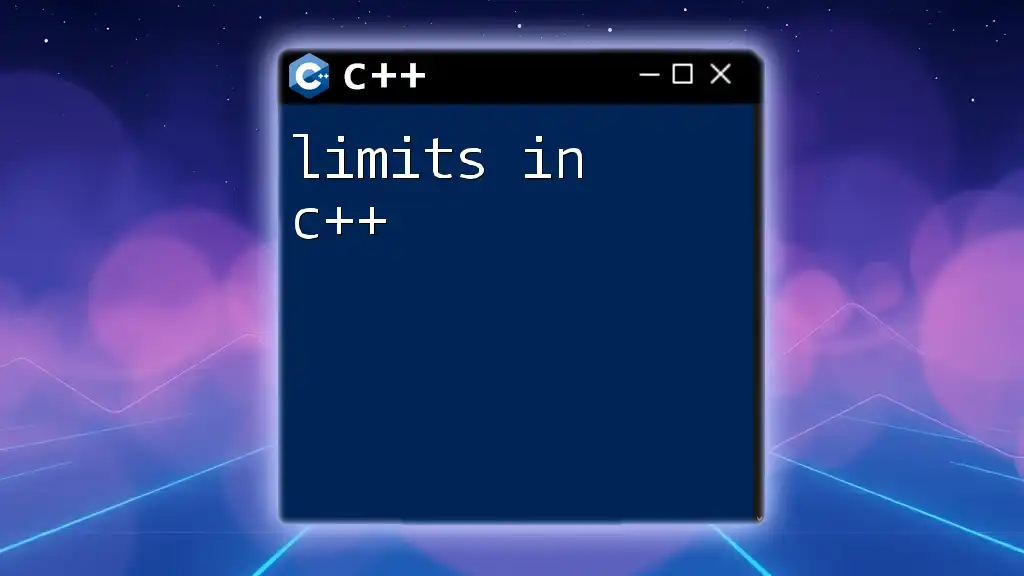
Conclusion
Understanding the right shift in C++ is pivotal for efficient programming, especially in fields that demand optimized performance. From bit manipulation to effective division and masking operations, the right shift operator offers a variety of applications that can significantly enhance your code's efficiency and clarity.
As you continue your journey with C++, practice using shift operators in diverse scenarios to strengthen your skills and avoid common pitfalls in bitwise operations. Stay curious and explore further topics related to C++ for a well-rounded understanding of this powerful language!
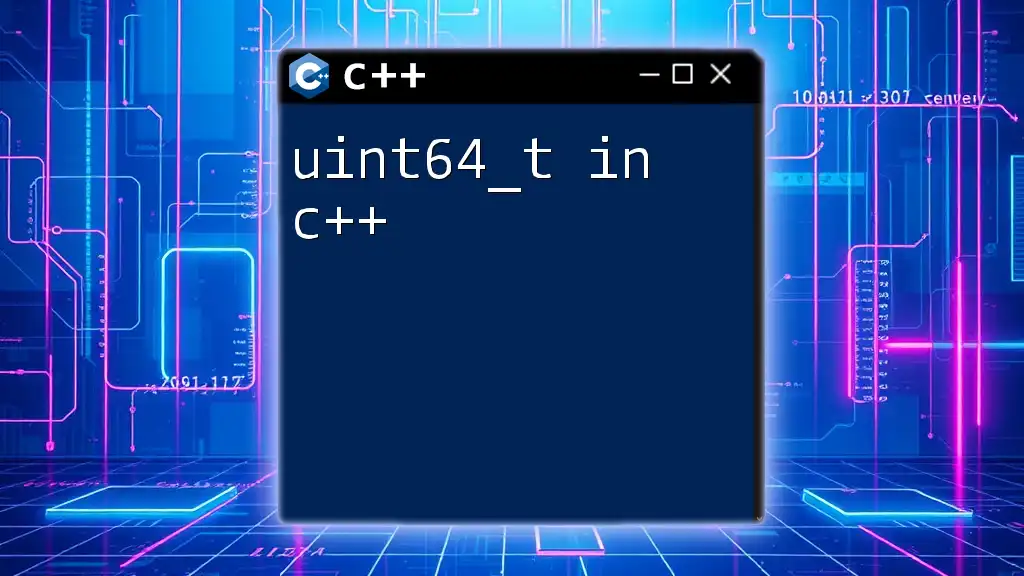
Quick Reference Section
- Arithmetic Right Shift: Preserves sign bit; fills with 1s for negatives.
- Logical Right Shift: Fills vacated bits with zeros; used for unsigned numbers.
- Power of Two Division: `x >> n` is equivalent to `x / (2^n)`.
- Masking: Combine shifting with bitwise operations for efficient data handling.
These quick references can help reinforce your understanding and serve as a guide for practical implementation.