In C++, `std::variant` is a type-safe union that can hold one of several predefined types, allowing for flexible data structures without the risk of invalid type access.
Here’s a simple example demonstrating its usage:
#include <iostream>
#include <variant>
#include <string>
int main() {
std::variant<int, float, std::string> myVariant;
myVariant = "Hello, Variant!";
std::cout << std::get<std::string>(myVariant) << std::endl; // Outputs: Hello, Variant!
return 0;
}
Understanding Variants
What is a Variant?
A variant in C++ is a type-safe union that can hold one of several specified types at any given time. This feature is encapsulated by the `std::variant` class template introduced in C++17. Unlike traditional unions, which could lead to undefined behavior if one accesses a member that is not active, `std::variant` provides a stricter guarantee of safety through type checking.
A critical advantage of variants is their type safety. While C-style unions can lead to bugs due to erroneous type access, variants prevent such errors by disallowing invalid access and ensuring that the type being accessed is the one currently held by the variant.
Key Features of Variants
When using variants in your C++ programs, you'll enjoy several key features:
- Type Safety: `std::variant` helps ensure you access the right type, minimizing bugs due to wrong type assumptions.
- Ownership Semantics: Variants maintain the ownership of the object they store, adhering to the principles of RAII (Resource Acquisition Is Initialization).
- Performance Considerations: Variants can be efficient in terms of memory usage, especially when handling a limited set of types, which allows for better optimization when compiled.
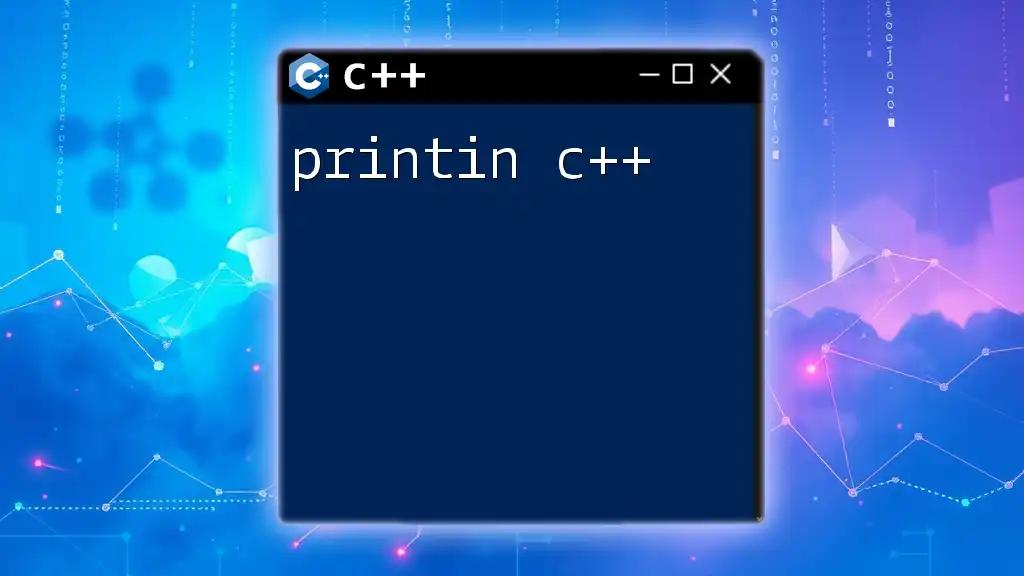
Basic Syntax and Declaration
Declaring a Variant
To declare a variant, you use the `std::variant` template, specifying the types it can hold. Here’s the basic syntax:
#include <variant>
std::variant<int, float, std::string> myVariant;
In this example, `myVariant` can hold either an integer, a float, or a string. The types specified are known as the alternatives. This allows for more complex data structures while being practically type-safe.
Initializing Variants
A variant can be initialized with one of its types as follows:
myVariant = 10; // holds int
myVariant = 15.5f; // now holds float
myVariant = "Hello, C++"; // now holds std::string
This flexibility allows developers to write more dynamic and less rigid code, making `std::variant` a powerful tool.
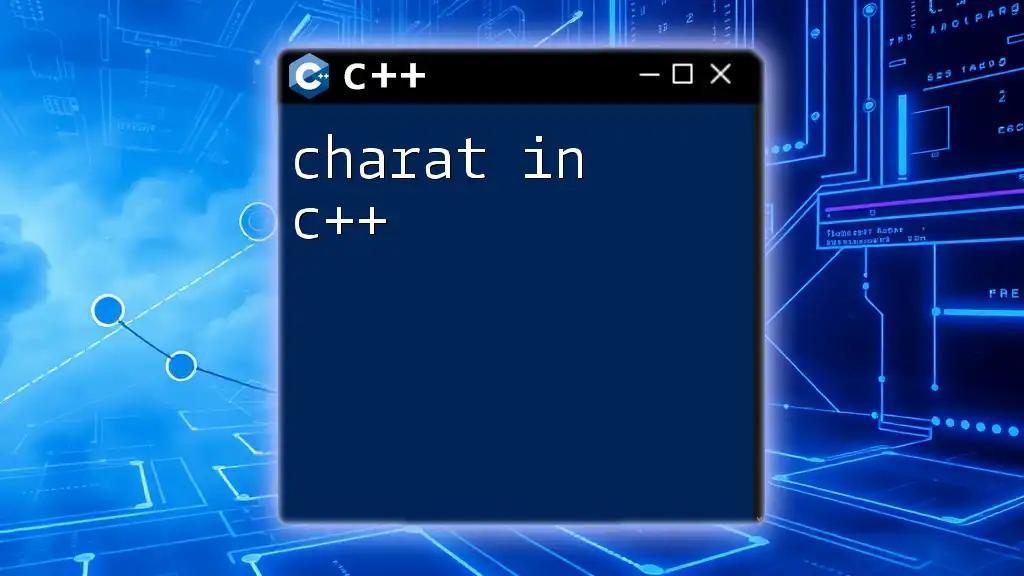
Working with Variants
Accessing Values in a Variant
To access the value stored in a variant, you typically use the `std::get` function. However, it’s crucial to ensure you’re accessing the right type; otherwise, you may end up with a `std::bad_variant_access` exception. Here’s an example:
try {
std::cout << std::get<int>(myVariant);
} catch (const std::bad_variant_access& e) {
std::cout << "Error: " << e.what();
}
If `myVariant` holds a `float` or `std::string` at the time you're trying to retrieve an `int`, this code will throw an exception. Handling these exceptions properly is a significant aspect of working with variants.
Using `std::get_if`
To safely access a value without throwing exceptions, you can use `std::get_if`. This function attempts to cast the variant to the specified type, returning a pointer if successful. Here’s how you can do this:
auto pInt = std::get_if<int>(&myVariant);
if (pInt) {
std::cout << *pInt;
} else {
std::cout << "Not an int!";
}
This approach is safer, as it allows you to check if the variant is currently of the desired type without risking an exception.

Practical Usage Scenarios
When to Use Variants
Variants shine in scenarios where you need to handle multiple types dynamically. Here are some use cases:
- Type-erasing Code: When you need to write code that can handle different types without knowing them ahead of time.
- Handling Multiple Types Gracefully: For APIs that need to return various types of responses without being tied to a specific type.
Variants in Function Parameters
You can use variants in function parameters to create more flexible APIs. For instance:
void processVariant(const std::variant<int, float>& v);
This function can accept either an integer or a float, allowing for more general processing logic without overloading functions for every type combination.

Advanced Features
Visitation with std::visit
`std::visit` allows you to define a visitor that processes the value held by the variant. This is particularly elegant for performing type-specific operations. Here's how it works:
struct Visitor {
void operator()(int i) const { std::cout << "int: " << i; }
void operator()(float f) const { std::cout << "float: " << f; }
void operator()(const std::string& s) const { std::cout << "string: " << s; }
};
std::visit(Visitor(), myVariant);
With `std::visit`, you can define behavior for each type held by the variant in a clean and expressive manner, consolidating type-specific logic in one location.
Combining Variants with Other STL Types
Variants can be particularly powerful when combined with other Standard Template Library (STL) containers like `std::vector` or `std::map`. Here’s an example of a vector that holds variants:
std::vector<std::variant<int, std::string>> myVector;
myVector.emplace_back(10);
myVector.emplace_back("Hello");
This allows for a single collection that can hold mixed types while maintaining the advantages of both vectors and variants.

Best Practices
When Not to Use Variants
Despite their advantages, variants may not always be the best fit. Avoid using them when:
- You have a large number of alternatives, as it may lead to performance overhead.
- The logic seems overly complicated compared to other object-oriented designs such as polymorphism or inheritance.
Performance Considerations
While variants are generally efficient, consider the memory overhead associated with them. When working with large variants or using them extensively in performance-sensitive applications, evaluate their impact on cache performance and overall efficiency.

Conclusion
In summary, `std::variant` introduces a powerful, type-safe way to handle multiple types in modern C++. Its flexibility and safety make it a superior choice compared to traditional unions, while features like `std::visit` enhance the expressiveness of type handling. By understanding the nuances of variants and practicing their usage, you can write more robust and maintainable C++ code, making it a cornerstone of your programming toolkit.

Further Reading
For those interested in diving deeper into the topic, consult the official C++ documentation on `std::variant`, or explore dedicated books and online resources for a more comprehensive understanding of type systems in C++. Exploring community forums and discussions can also yield practical insights and advanced use cases.