In C++, the `::` operator is known as the scope resolution operator, and it is used to define the context in which a name (such as a variable, function, or class) is defined, helping to differentiate between entities with the same name in different scopes.
Here's a code snippet demonstrating its use:
#include <iostream>
namespace MyNamespace {
int value = 42;
}
int main() {
std::cout << "Value from MyNamespace: " << MyNamespace::value << std::endl;
return 0;
}
Understanding Scope Resolution
What Is Scope Resolution?
Scope resolution is a crucial concept in programming that defines where variables and functions are accessible within the code. Understanding where particular identifiers are located and their visibility can help in organizing code and preventing naming conflicts. This is where the scope resolution operator, denoted by `::`, comes into play in C++.
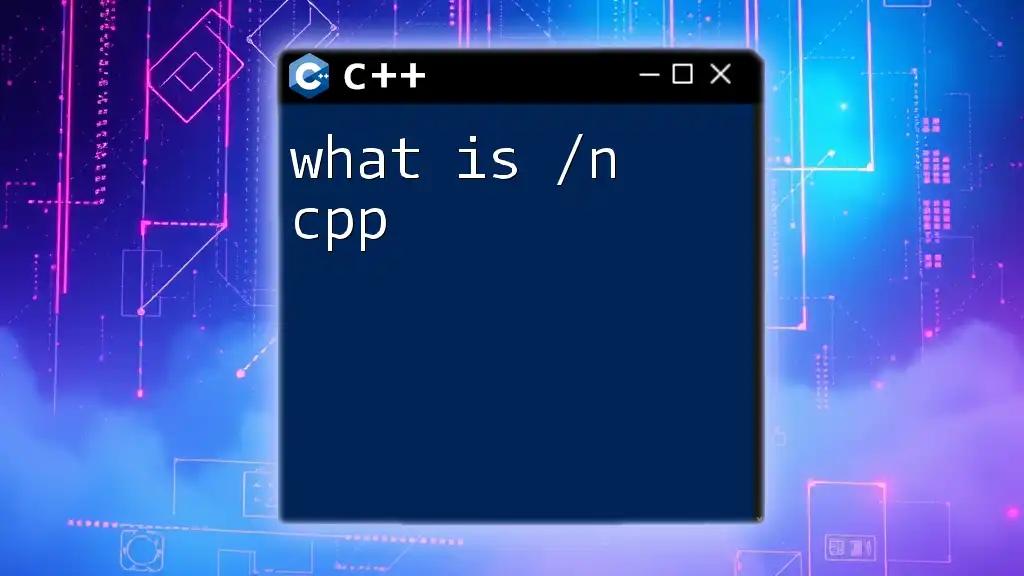
The Scope Resolution Operator (::)
What Does the Scope Resolution Operator Do?
The scope resolution operator `::` is used to specify the context in which a variable, function, or class is defined or to differentiate between entities that share the same name within different scopes. By using this operator, you can explicitly indicate whether you are referring to a global variable, a static class member, or a function defined within a specific namespace.
Why Use the Scope Resolution Operator?
The `::` operator serves multiple purposes, primarily focusing on resolving naming conflicts. Here are some scenarios where this operator is beneficial:
- It allows access to global variables from within functions where a local variable with the same name exists.
- You can access static members of classes to prevent ambiguity.
- It enables differentiated access to functions and variables defined in separate namespaces, helping to maintain organized code structures.
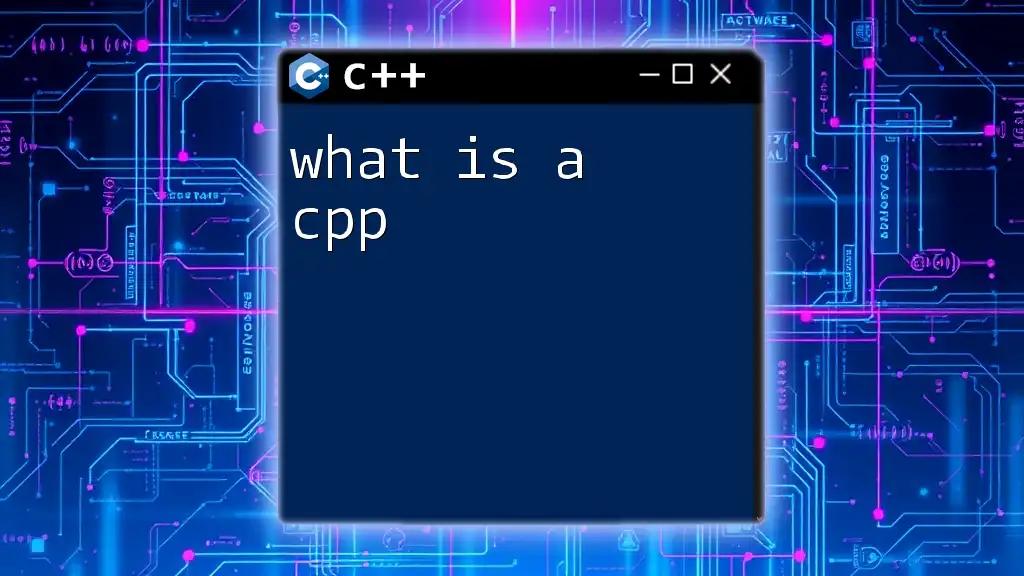
Usage of the Scope Resolution Operator
Accessing Global Variables
One of the primary uses of the `::` operator is to access global variables that might be shadowed by local variables. For instance:
int x = 10; // Global variable
void function() {
int x = 20; // Local variable
std::cout << "Local x: " << x << std::endl; // Prints 20
std::cout << "Global x: " << ::x << std::endl; // Prints 10
}
In the example above, within the `function`, attempting to use `x` directly would refer to the local variable. By using `::x`, we access the global variable instead, demonstrating how `::` resolves the scope issue.
Accessing Class Members
The scope resolution operator is also essential in accessing class members, especially static members. Static members belong to the class itself rather than any instance, and they can be accessed via:
class MyClass {
public:
static int value;
};
int MyClass::value = 5; // Definition of the static member
void display() {
std::cout << "Value: " << MyClass::value << std::endl; // Access static member
}
In this example, `MyClass::value` clearly indicates that we are referring to the static member of the class `MyClass`, avoiding any ambiguity.
Namespace Resolution
Namespaces are a powerful feature in C++ that allow you to group related code and avoid name clashes. The `::` operator serves to access entities defined in specific namespaces:
namespace MyNamespace {
void display() {
std::cout << "Hello from MyNamespace!" << std::endl;
}
}
void callNamespaceFunction() {
MyNamespace::display(); // Calls function from MyNamespace
}
In the code above, `MyNamespace::display()` explicitly refers to the `display` function defined within the `MyNamespace`, showcasing the utility of the `::` operator in namespace management.
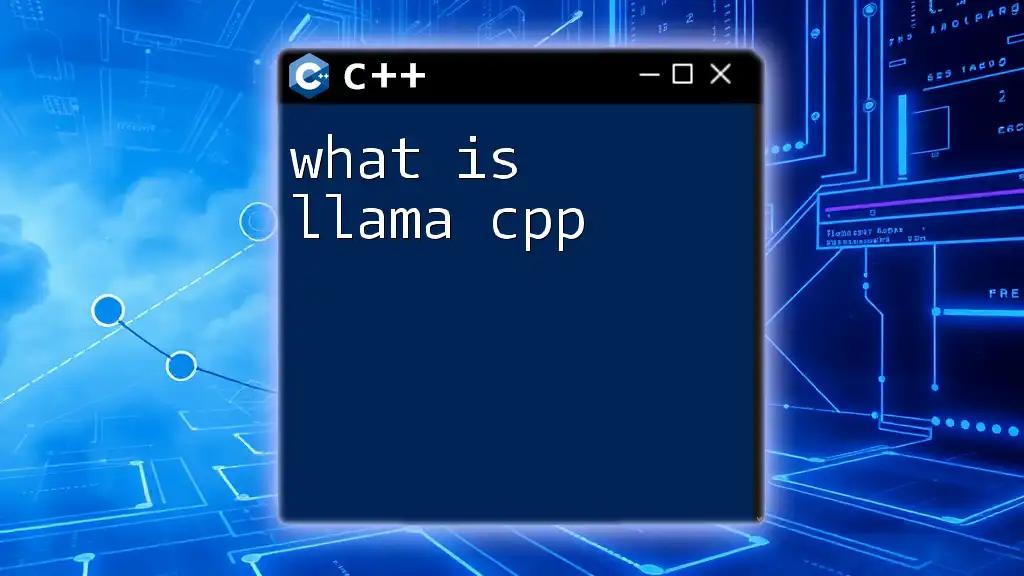
Advanced Use Cases of Scope Resolution Operator
Combining with Inheritance
When dealing with inheritance, the `::` operator can be used to call methods of base classes, which might be overshadowed by derived class implementations:
class Base {
public:
static void show() {
std::cout << "Base class" << std::endl;
}
};
class Derived : public Base {
public:
static void show() {
std::cout << "Derived class" << std::endl;
}
void display() {
Base::show(); // Call Base class method
Derived::show(); // Call Derived class method
}
};
In this example, using `Base::show()` allows us to explicitly call the method from the `Base` class, demonstrating the effectiveness of `::` in managing method visibility in inheritance hierarchies.
Scope and Templates
The `::` operator also plays a role in template programming. Accessing types or static members via templates can be crucial in generic programming:
template <typename T>
class Container {
public:
static const int size = 10;
};
void printSize() {
std::cout << "Size: " << Container<int>::size << std::endl; // Access static member from template
}
In this case, `Container<int>::size` illustrates how the scope resolution operator is used to access a specific static member from a template instantiation, helping to reinforce type safety in generic programming.
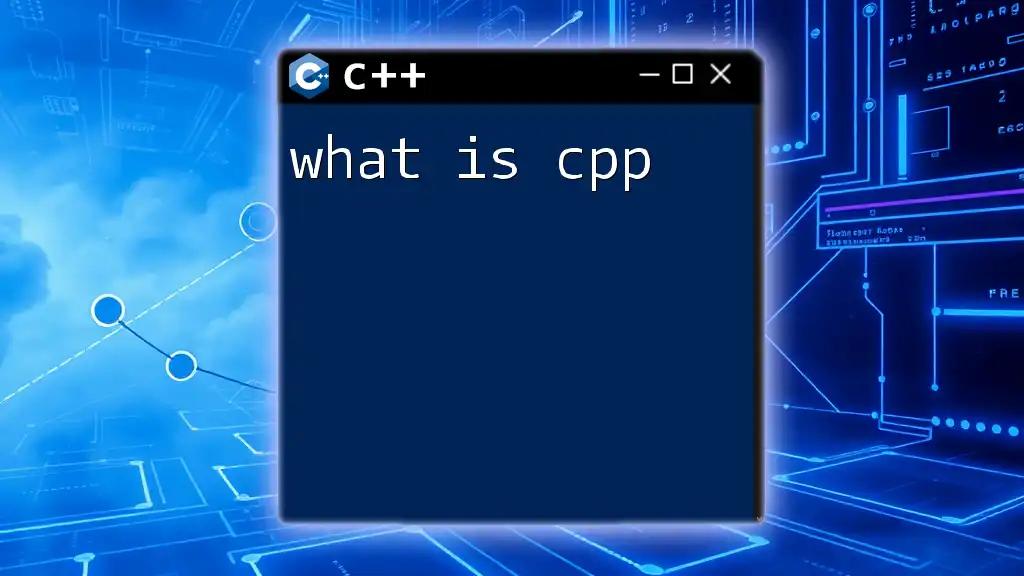
Common Mistakes When Using the "::" Operator
Confusing Scope with Different Contexts
One common issue is the confusion between local and global scopes. Failing to use the `::` operator can lead to unexpected results, especially if local variables are overshadowing global variables. Always be cautious and verify which context you are working within to prevent unintended access to variables.
Overusing the Scope Resolution Operator
While the `::` operator is a fantastic tool for resolving conflicts, it’s essential not to overuse it. Complicated chains of `::` can make code harder to read and maintain. Best practices suggest using it only when necessary to clarify context, ensuring that your code remains clean and understandable.
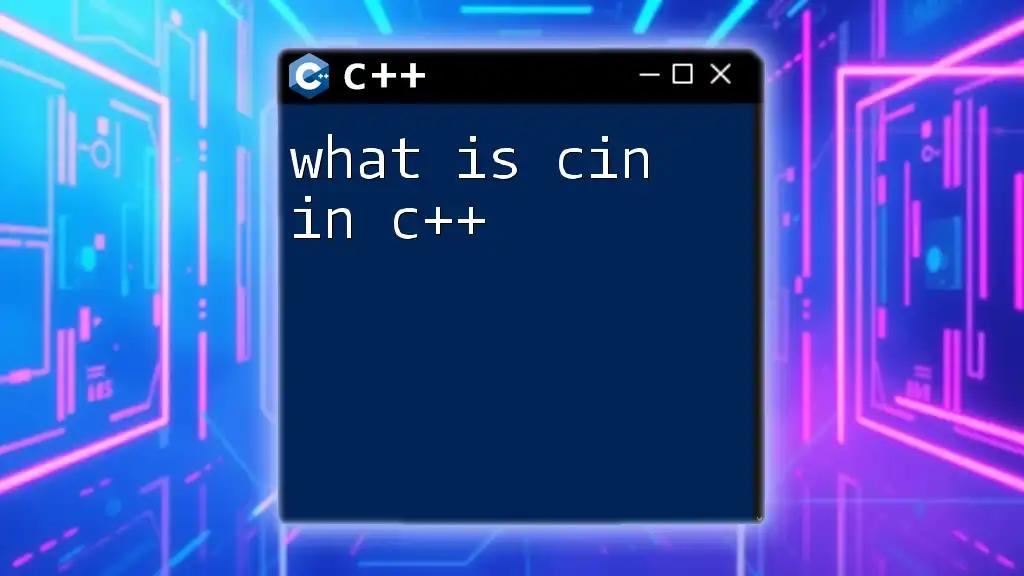
Conclusion
Understanding what is `::` in C++ is pivotal for mastering scope resolution and creating robust, conflict-free code. By using the scope resolution operator wisely, you can avoid naming conflicts and enhance the clarity of your programs. Practice these concepts in your C++ projects to become more adept and confident in your programming skills.
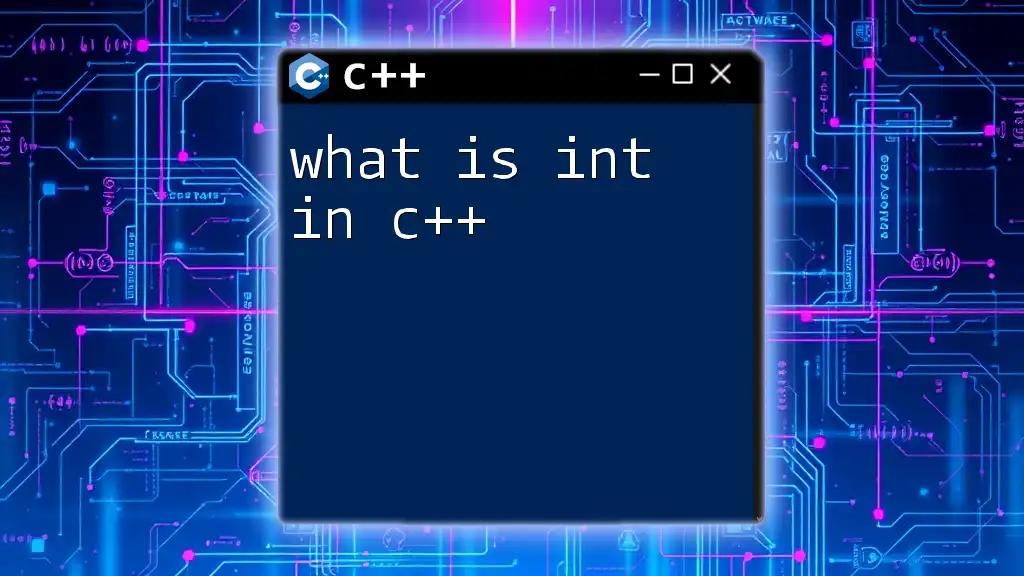
Call to Action
Have you encountered challenges with the scope resolution operator in your C++ journey? Share your experiences or questions in the comments below—let's learn from each other's challenges and successes!
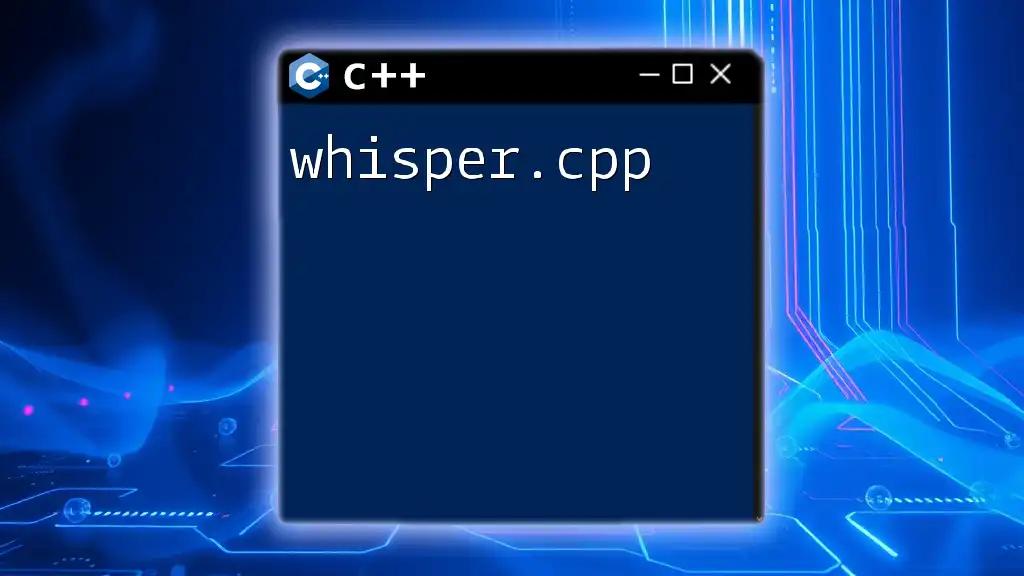
Additional Resources
For further exploration of the scope resolution operator and advanced C++ concepts, check the following resources:
- C++ documentation on scope resolution
- Comprehensive guides on namespaces and classes
- Online forums and communities for C++ programmers