"whisper-cpp is a C++ library designed for efficient audio transcription using state-of-the-art models, allowing developers to convert speech into text quickly and easily."
Here’s a simple example of using the whisper-cpp library in your code:
#include <whisper/whisper.h>
int main() {
Whisper whisper("path_to_model");
std::string transcription = whisper.transcribe("path_to_audio_file.wav");
std::cout << "Transcription: " << transcription << std::endl;
return 0;
}
Getting Started with Whisper-cpp
Installation
To enjoy the benefits of whisper-cpp, you'll first need to install the library on your machine. The following steps guide you through the installation process on different platforms.
MacOS: To install whisper-cpp via Homebrew, run the following command in your terminal:
brew install whisper-cpp
Windows: Using vcpkg for Windows can streamline the installation:
git clone https://github.com/microsoft/vcpkg.git
cd vcpkg
.\bootstrap-vcpkg.bat
.\vcpkg integrate install
vcpkg install whisper-cpp
Linux: For Linux users, you can install whisper-cpp using the package manager. For example, on Ubuntu, you can use:
sudo apt-get install whisper-cpp
Basic Configuration
After installation, configuring your development environment ensures smooth usage of whisper-cpp.
-
Setting Up Your Development Environment: You can utilize various IDEs like Visual Studio, Code::Blocks, or even text editors like VSCode. It's crucial to ensure whisper-cpp is recognized.
-
Configuring IDEs: Most IDEs allow you to add include paths where libraries are stored. Make sure to add the path to your whisper-cpp installation. An example configuration for Visual Studio can be done through:
- Right-clicking your project → Properties → C/C++ → General → Additional Include Directories
-
Verifying Installation: Once configured, create a simple program to test if whisper-cpp is functioning correctly. An example could look like:
#include <whisper-cpp.h>
int main() {
whisper::init(); // Initialize the library
whisper::print("Whisper-cpp is successfully installed!");
return 0;
}
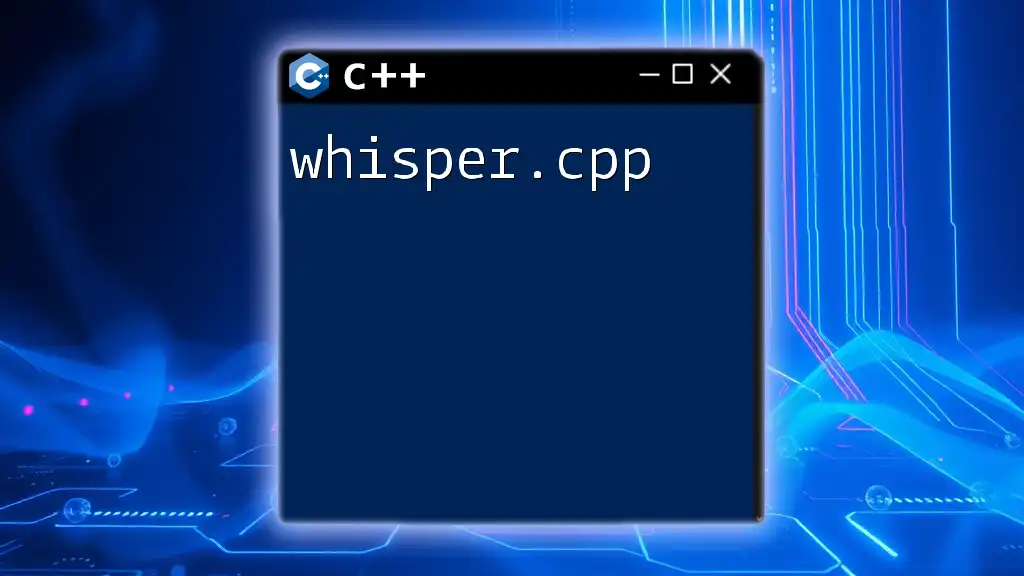
Core Features of Whisper-cpp
Ease of Use
The primary feature of whisper-cpp is its commitment to simplicity. It effectively reduces the complexity of standard C++ commands. For instance, where you might typically see:
std::cout << "Hello, World!" << std::endl;
In whisper-cpp, it can be simplified to:
whisper::print("Hello, World!");
This shift not only makes code snippets shorter but also more readable, especially for newcomers.
Key Commands and Functions
Whisper-cpp encompasses a variety of commands that streamline common programming tasks:
- Inputs and Outputs:
- Using `whisper::print()` for output, you can also fetch input using `whisper::read()`, which is remarkably intuitive:
std::string userInput = whisper::read("Enter your name: ");
whisper::print("Hello, " + userInput + "!");
- Data Manipulation:
- With whisper-cpp, manipulating data becomes easier. You can use simplified data structures that facilitate operations:
auto numbers = whisper::list{1, 2, 3, 4, 5};
whisper::filter(numbers, [](int n) { return n > 3; });
- Control Structures:
- Simplified control structures make it easier to write loops and conditions. For example, instead of verbose syntax, a simple way to use a loop is:
whisper::for_each(numbers, [](int n) {
whisper::print(n);
});
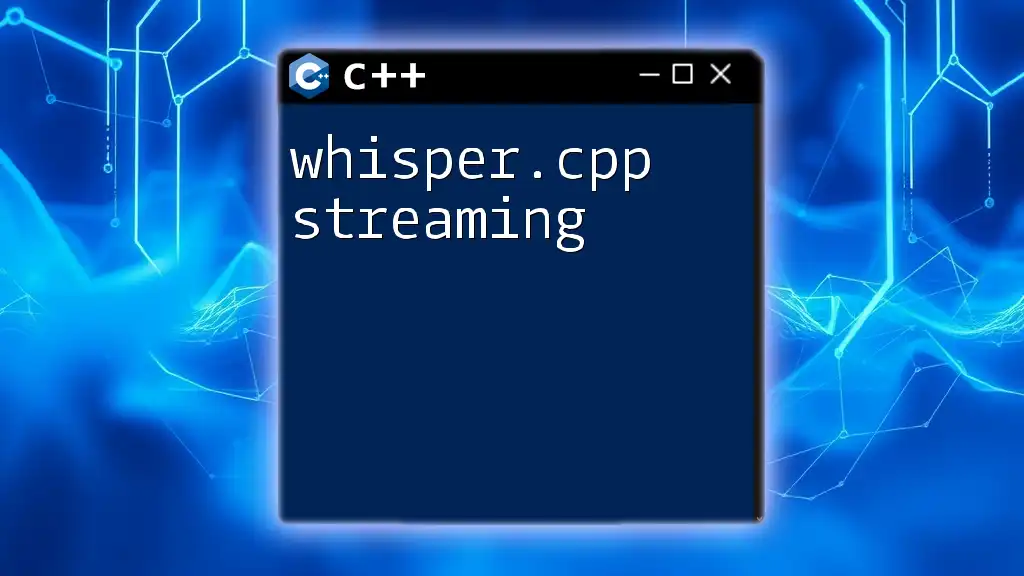
Advanced Features
Extending Whisper-cpp
If you want to elevate your coding experience further, consider creating your own commands or functions. Custom functions can enhance the pre-defined capabilities of whisper-cpp. An example of a user-defined command might be:
void myCommand() {
whisper::print("This is my custom command!");
}
By encapsulating functionality within custom commands, you create reusable components that can streamline your workflow.
Optimizing Performance with Whisper-cpp
Efficiency is vital in any programming task. When using whisper-cpp, consider the following optimization practices:
-
Efficient Coding Practices:
- Utilize built-in functions rather than reinventing the wheel. Built-in methods are usually optimized for performance.
-
Profiling and Optimizing Code:
- Always analyze your code’s performance. Whisper-cpp may offer built-in profiling tools to help identify bottlenecks.
-
Performance Comparison:
When optimizing, it’s beneficial to compare your basic implementation against an optimized one to visualize performance gains.
// Basic implementation
for(int i = 0; i < 1000; i++) {
whisper::print(i);
}
// Optimized implementation
whisper::for_each(whisper::range(0, 1000), [](int n) {
whisper::print(n);
});
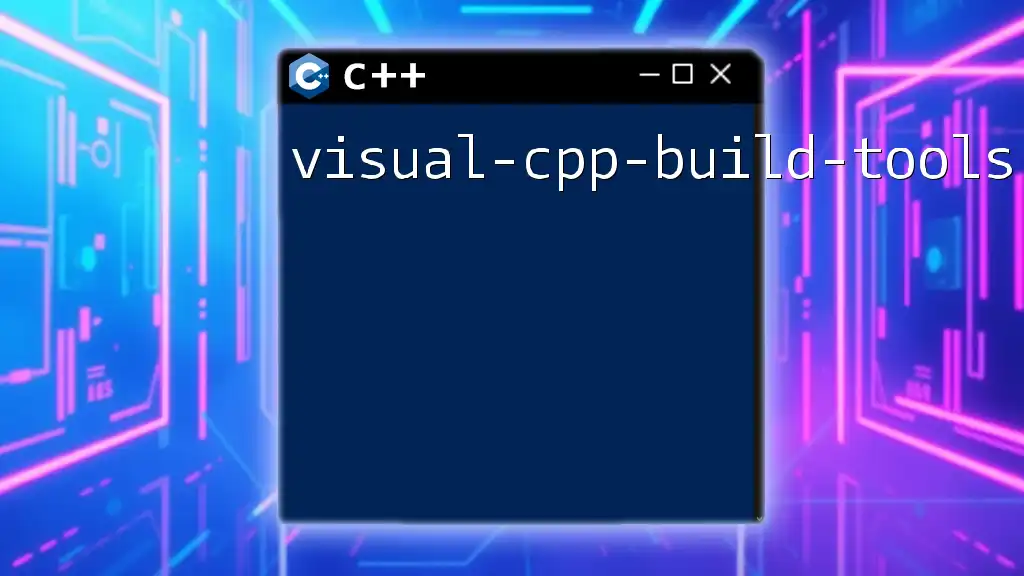
Real-world Applications
Use Cases of Whisper-cpp
Whisper-cpp shines across various domains, including:
- Game Development: It provides convenient commands for rapid prototyping.
- Data Processing: The simplified syntax allows for easier data manipulation and analysis.
- Robotics: Its easy command structure facilitates direct control over hardware components.
Case Study: A Mini Project Using Whisper-cpp
Let’s build a simple application to demonstrate whisper-cpp in action—an interactive number guessing game.
Project Overview: The game will prompt users to guess a number, providing feedback on whether the guess is too high or too low.
Implementation Steps:
- Setup:
#include <whisper-cpp.h>
int main() {
whisper::print("Welcome to the Number Guessing Game!");
int secretNumber = whisper::random(1, 100); // Random number between 1 and 100
int userGuess = 0;
while (userGuess != secretNumber) {
userGuess = whisper::read("Enter your guess: ");
if (userGuess < secretNumber) {
whisper::print("Too low! Try again.");
} else if (userGuess > secretNumber) {
whisper::print("Too high! Try again.");
} else {
whisper::print("Congratulations! You guessed it!");
}
}
return 0;
}
- Explanation:
- The program initializes by announcing the game and generating a random secret number.
- It prompts the user for guesses, providing feedback until the user correctly identifies the number.
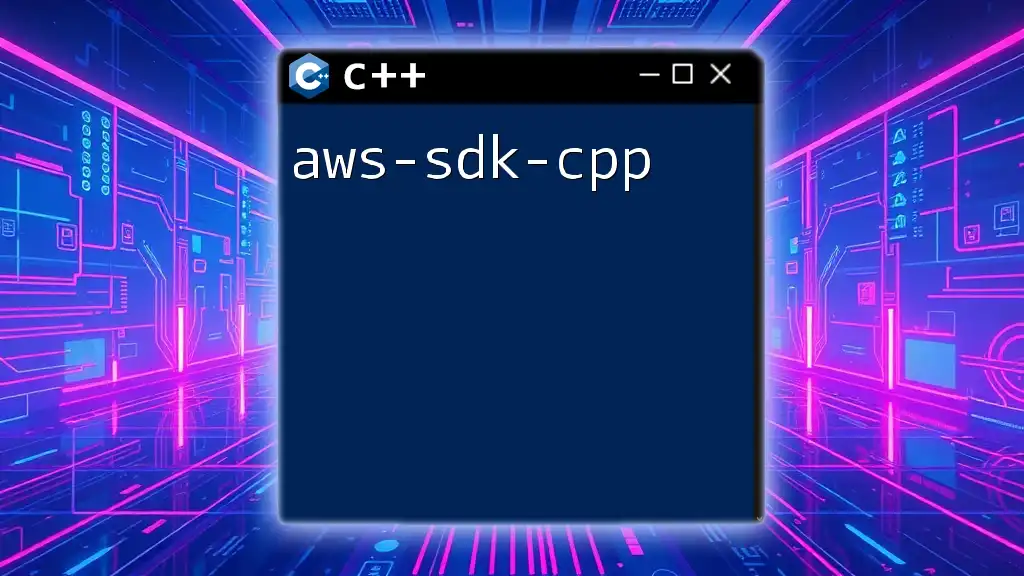
Troubleshooting Common Issues
Common Errors and Solutions
Even with whisper-cpp, you might run into issues, such as:
- Compilation Errors: Ensure you have configured your IDE properly to point to the whisper-cpp library.
- Runtime Issues: Verify variable types and library methods; using the wrong type can lead to unexpected behavior.
FAQs about Whisper-cpp
-
Is Whisper-cpp suitable for beginners? Absolutely! Its concise syntax is designed to help newcomers quickly grasp core concepts in C++.
-
Can I integrate Whisper-cpp with other libraries? Yes, whisper-cpp can coexist with standard C++ libraries and most third-party libraries.
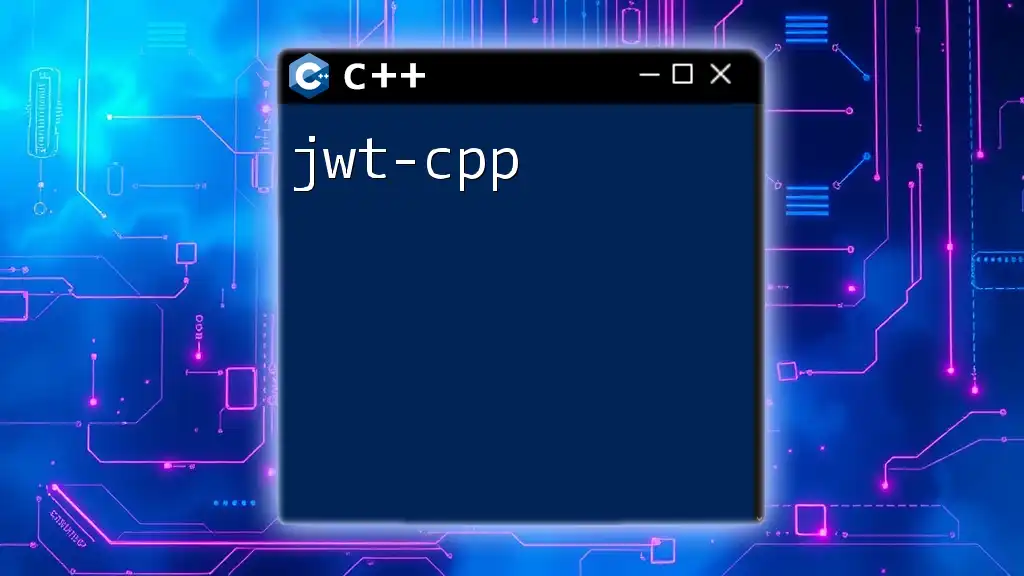
Conclusion
Summarizing the incredible benefits and features of whisper-cpp, this library simplifies C++ programming, enhances readability, and fosters an enjoyable coding experience. By incorporating whisper-cpp into your projects, you can streamline your workflow and focus more on building innovative solutions rather than wrestling with verbose syntax.
Further Learning Resources
To deepen your understanding of whisper-cpp and related topics, consider exploring these resources:
- Books on modern C++ programming
- Online courses specialized in C++
- Official whisper-cpp documentation for the latest features
Call to Action
We invite you to implement whisper-cpp into your projects and share your experiences. Whether you're teaching others or expanding your own skills, exploring this tool is an investment in your coding journey. Happy coding!
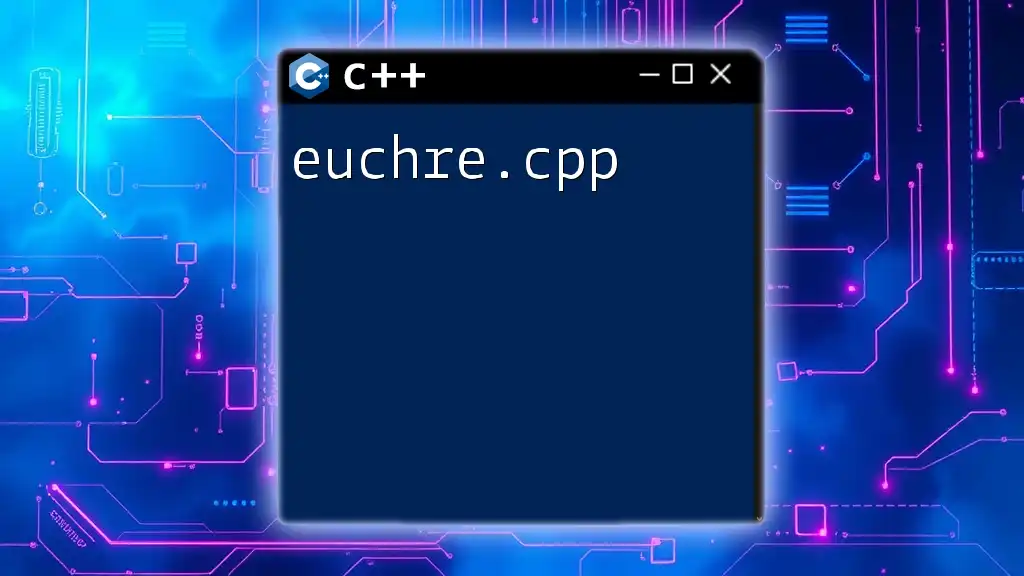
Appendix
Glossary of Key Terms
- IDE: Integrated Development Environment, a software application that provides comprehensive facilities to programmers for software development.
- Function Call: A programming construct that executes a defined procedure or function in the code.
References
For additional information and resources on C++ and whisper-cpp, consult:
- Official documentation and developer blogs
- C++ programming communities and forums