The `tolower` function in C++ is used to convert a character to its lowercase equivalent, enhancing case-insensitive operations in string handling.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <cctype> // for tolower function
int main() {
char ch = 'A';
char lowerCh = tolower(ch);
std::cout << "Lowercase of " << ch << " is " << lowerCh << std::endl;
return 0;
}
Understanding `tolower` in C++
What is `tolower`?
`tolower` is a standard library function provided in C++ that is part of the `<cctype>` header. Its primary purpose is to convert a given character to its lowercase equivalent. This function plays a crucial role in text manipulation, allowing programmers to ensure consistency in string comparisons and formatting.
How `tolower` works
The `tolower` function works by taking ASCII values of characters into account. In the ASCII table, uppercase characters have different values compared to their lowercase counterparts.
The function signature is simple:
int tolower(int ch);
Here, `ch` is the character (or its ASCII value) that you want to convert. The function will return the lowercase version of that character if it is uppercase, or it will return the input unchanged if it is already lowercase, or if it is a non-alphabetic character.
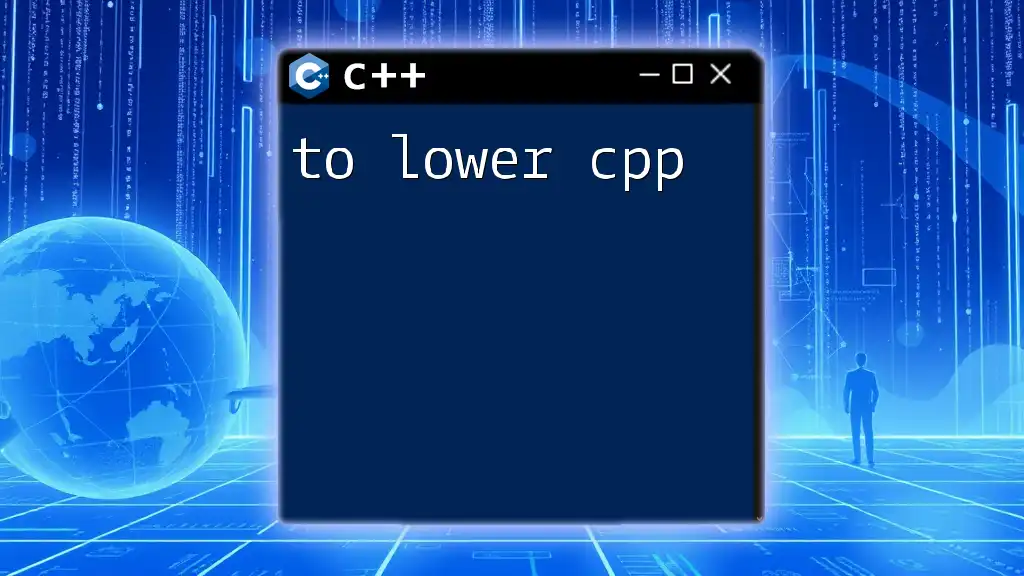
The `tolower` Function in Action
Basic Syntax
The basic syntax of `tolower` is straightforward:
int tolower(int ch);
The parameter represents a single character which can either be an integer representing an ASCII value or a character. The function returns an integer which corresponds to the lowercase version of the input character.
Importing the Required Headers
To use `tolower`, you need to include the `<cctype>` header at the beginning of your program. This is essential as it provides the prototype for the `tolower` function.
Here's how to import it:
#include <cctype>
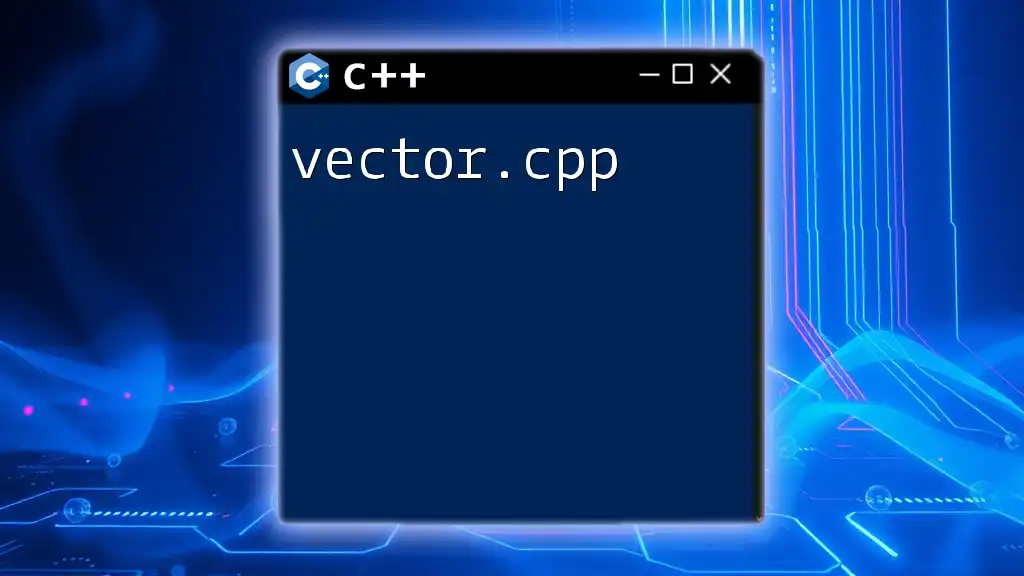
Practical Examples of Using `tolower`
Example 1: Converting a Single Character to Lowercase
To demonstrate how `tolower` works, let's start with a simple example that converts a single uppercase character to lowercase.
#include <iostream>
#include <cctype>
int main() {
char letter = 'A';
char lower = tolower(letter);
std::cout << "Lowercase of " << letter << " is " << lower << std::endl;
return 0;
}
In the above example, the character `'A'` is converted to lowercase, resulting in the output:
Lowercase of A is a
This illustrates how `tolower` effectively changes the case of a character.
Example 2: Converting a String to Lowercase
Converting an entire string to lowercase involves looping through each character and applying the `tolower` function.
#include <iostream>
#include <cctype>
#include <string>
std::string to_lowercase(const std::string &input) {
std::string output = input;
for(auto &c : output) {
c = tolower(c);
}
return output;
}
int main() {
std::string str = "Hello, World!";
std::string lowerStr = to_lowercase(str);
std::cout << "Lowercase string: " << lowerStr << std::endl;
return 0;
}
In this code, the function `to_lowercase` takes a string as input and converts each character to its lowercase version. The `for` loop traverses each character of the string, applying `tolower`. The output will be:
Lowercase string: hello, world!
This showcases how `tolower` can be utilized for more than just single characters, facilitating easier string manipulation.
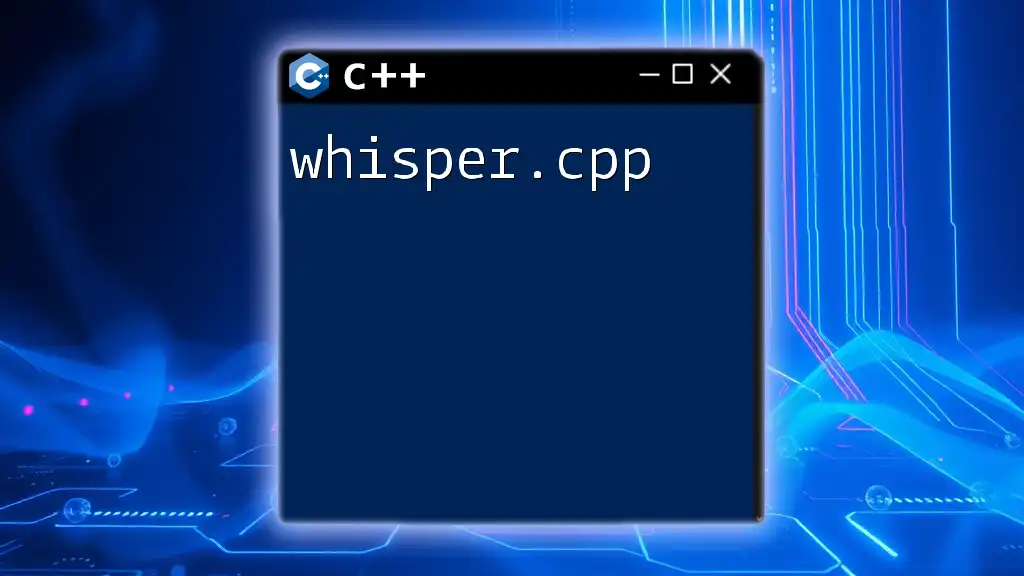
Advanced Usage of `tolower`
Handling Special Characters and Digits
It’s important to note how `tolower` behaves with non-alphabetic characters. For instance, applying `tolower` to a special character or digit results in the character being returned unchanged.
#include <iostream>
#include <cctype>
int main() {
char specialChar = '#';
char digit = '5';
std::cout << "Lowercase of " << specialChar << " is " << tolower(specialChar) << std::endl;
std::cout << "Lowercase of " << digit << " is " << tolower(digit) << std::endl;
return 0;
}
The output for this example will be:
Lowercase of # is #
Lowercase of 5 is 5
This emphasizes that `tolower` is designed specifically for alphabetic characters and will leave non-alphabetic characters unaffected.
Performance Considerations
When using `tolower` on larger datasets or strings, performance can become a concern. While the function is efficient for small scale use, if you're implementing it on large strings multiple times, consider whether it’s being called excessively.
Best practices include minimizing the number of calls to `tolower` through optimized string handling techniques and batch processing where applicable.
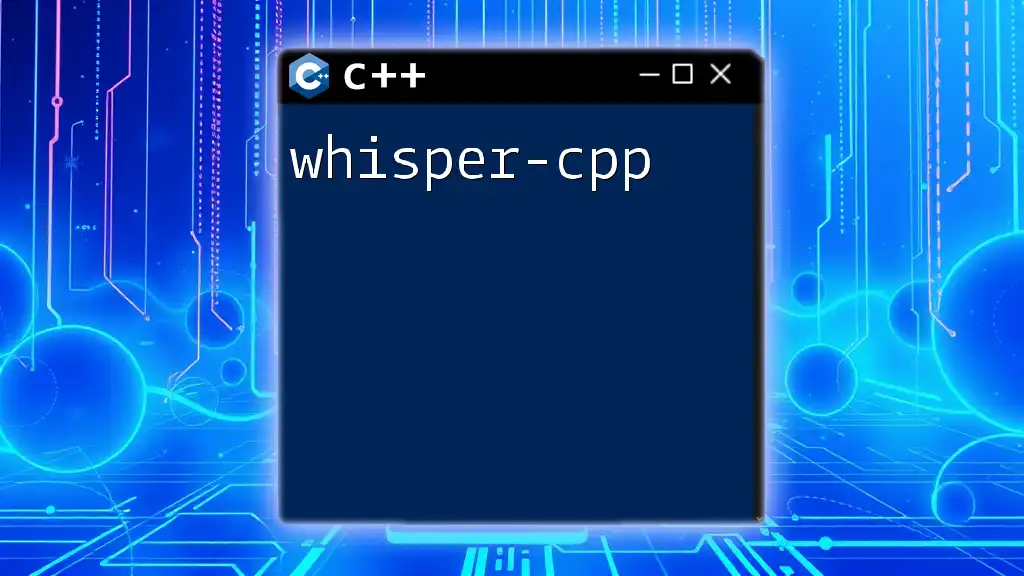
Alternatives to `tolower`
Other Libraries for String Manipulation
While `tolower` serves its purpose, other libraries also provide capabilities for string manipulation. Starting from C++11, the `<algorithm>` header provides the `std::transform` function, which can be used for case conversion as well.
Here is how you would implement it:
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string str = "C++ Programming!";
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
std::cout << str << std::endl;
return 0;
}
Using `std::transform`, we can easily convert an entire string to lowercase in a single line of code. This method can improve readability and may offer better performance when working with larger strings due to the internal optimizations of the Standard Library.
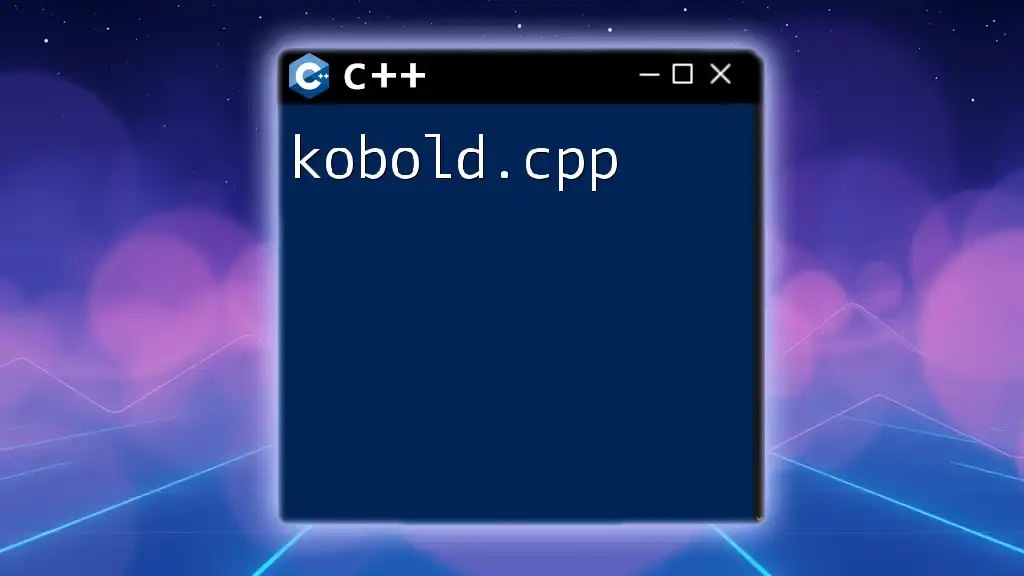
Conclusion
Recap of Key Points
In summary, the `tolower` function in C++ is crucial for converting characters to lowercase, enabling consistent text handling. Its simplicity and utility make it a go-to tool for many string manipulation tasks. Remember that it exclusively operates on alphabetic characters.
Recommendations for Further Learning
To delve deeper into text manipulation in C++, consider exploring:
- Official C++ documentation for `<cctype>`.
- Tutorials on string handling and algorithm libraries such as `<algorithm>`.
Call to Action
Practice with different examples using the `tolower` function and explore more commands to enhance your programming skills! Don’t hesitate to experiment with various data types and strings to see how you can utilize case conversion in your projects.