To convert a string to lowercase in C++, you can use the `tolower` function along with a loop to iterate through each character of the string.
#include <iostream>
#include <string>
#include <cctype>
std::string toLower(const std::string &input) {
std::string output = input;
for (char &c : output) {
c = std::tolower(c);
}
return output;
}
int main() {
std::string str = "Hello World!";
std::cout << toLower(str) << std::endl; // Outputs: hello world!
return 0;
}
Understanding Character Case in C++
What is Case Sensitivity?
In programming, case sensitivity refers to the distinction between uppercase and lowercase letters when performing comparisons or processing strings. In C++, this means that the variable names `Variable`, `variable`, and `VARIABLE` can refer to three entirely different entities. Understanding case sensitivity is crucial when dealing with user input, string comparisons, and database queries since strings are almost always treated as dogmatically as their casing dictates.
Common Use Cases for Lowercase Transformation
Lowercasing strings has a variety of practical applications:
-
User Input: When accepting input from users, it is often prudent to convert all input to lowercase to ensure consistency, especially when comparing against other strings.
-
Data Normalization: When handling datasets, converting identifiers to a common case helps to eliminate discrepancies that arise from different casing in string data.
-
Search Operations: Lowercase conversion allows for more effective search operations, as it removes the complexity of managing case variants.
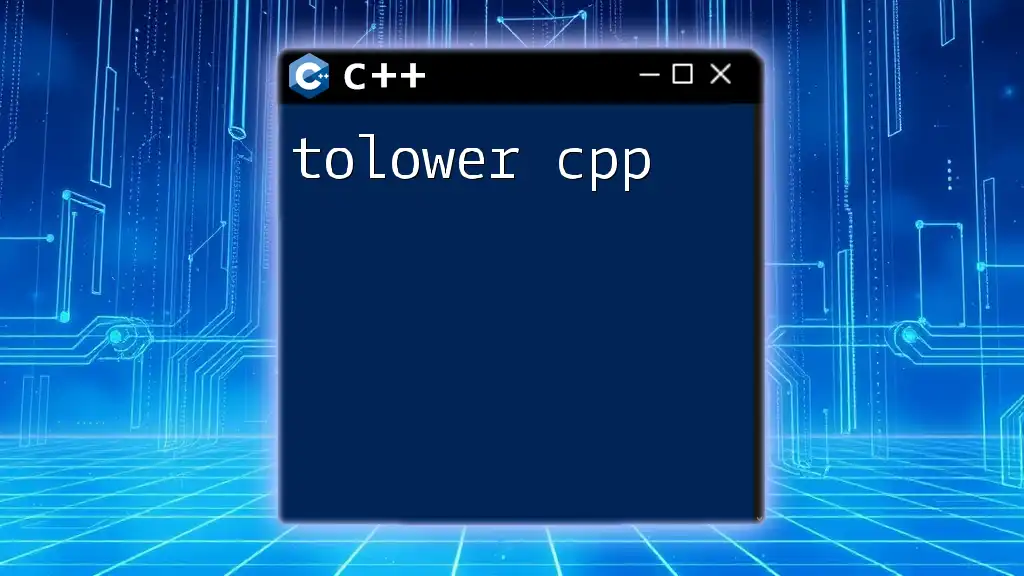
The C++ `tolower` Function
What is `tolower`?
The `tolower` function is a part of the C++ standard library and is used to convert a given character to lowercase. It is declared in the `<cctype>` header file, making it readily accessible for string manipulation tasks involving case changes.
Syntax of `tolower`
The basic syntax of `tolower` is:
int tolower(int ch);
How `tolower` Works
When you pass a character to the `tolower` function, it checks whether that character is an uppercase letter. If it is, it returns its lowercase equivalent. If the character is not an uppercase letter, it remains unchanged. This mechanism allows developers to safely process strings without modifying their non-alphabetic characters.
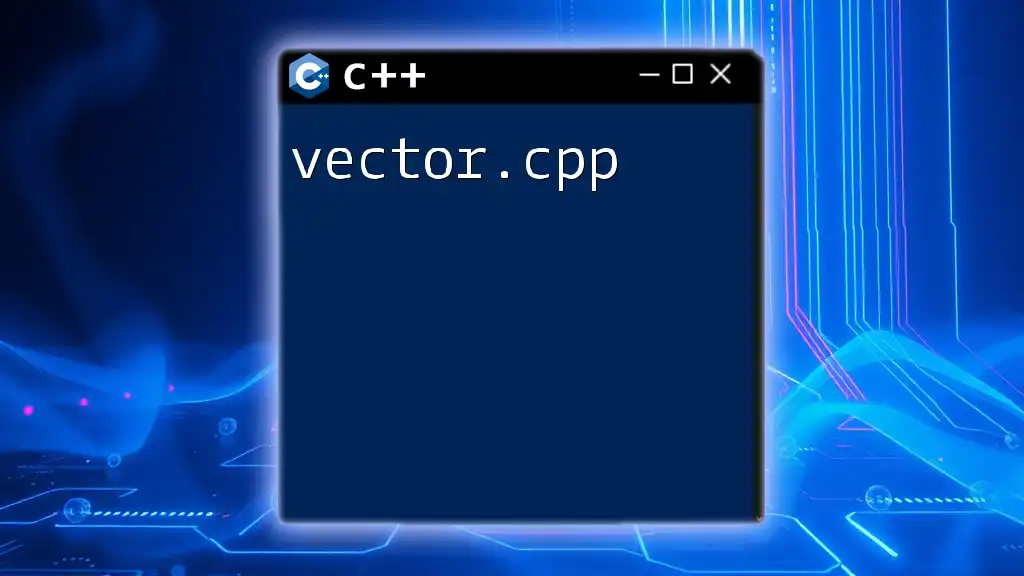
Using the `tolower` Function with Characters
Converting Individual Characters
To demonstrate how to use `tolower`, consider the following code snippet:
#include <cctype>
#include <iostream>
int main() {
char ch = 'A';
char lowerCh = tolower(ch);
std::cout << lowerCh; // Output: a
return 0;
}
In this example, the character `'A'` is passed into the `tolower` function. The function converts it to its lowercase counterpart, resulting in the output of `a`. Characters that are already lowercase or those that are not alphabetic remain unaffected, thus ensuring the integrity of the data:
#include <cctype>
#include <iostream>
int main() {
char ch1 = 'A'; // Uppercase
char ch2 = 'b'; // Lowercase
char ch3 = '1'; // Non-alphabetic character
std::cout << tolower(ch1) << " " << tolower(ch2) << " " << tolower(ch3);
// Output: a b 1
return 0;
}
Handling Different Characters
It's essential to understand that not all characters will behave the same with `tolower`. Non-alphabetic characters (like numbers or punctuation) are returned unchanged by the function, allowing for flexible and error-free data manipulation.
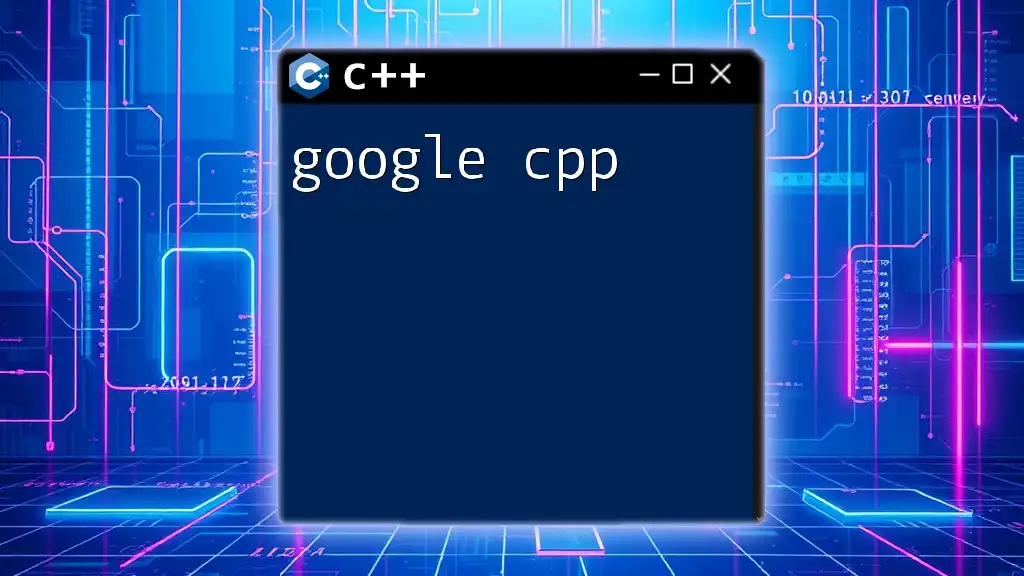
Converting Strings to Lowercase in C++
Using a Loop for String Conversion
When it comes to converting entire strings to lowercase, the most straightforward approach is to iterate through each character in the string and convert them individually. Below is a code snippet demonstrating this method:
#include <cctype>
#include <iostream>
#include <string>
void toLowercase(std::string &str) {
for (auto &ch : str) {
ch = tolower(ch);
}
}
int main() {
std::string text = "Hello, World!";
toLowercase(text);
std::cout << text; // Output: hello, world!
return 0;
}
In this example, the function `toLowercase` takes a reference to a string as a parameter. By using a range-based for loop, each character of the string is transformed to lowercase efficiently. This method is easy to understand and implement, allowing for any string to be converted effortlessly.
Using Standard Library Functions
For a more compact approach, C++ offers the `std::transform` algorithm, which can simplify the code further. Here's how you can leverage it:
#include <algorithm>
#include <cctype>
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::transform(text.begin(), text.end(), text.begin(), tolower);
std::cout << text; // Output: hello, world!
return 0;
}
In this implementation, `std::transform` iterates over each character from the beginning to the end of the string and applies the `tolower` function to convert it to lowercase directly in the string. This keeps the code precise and clean.
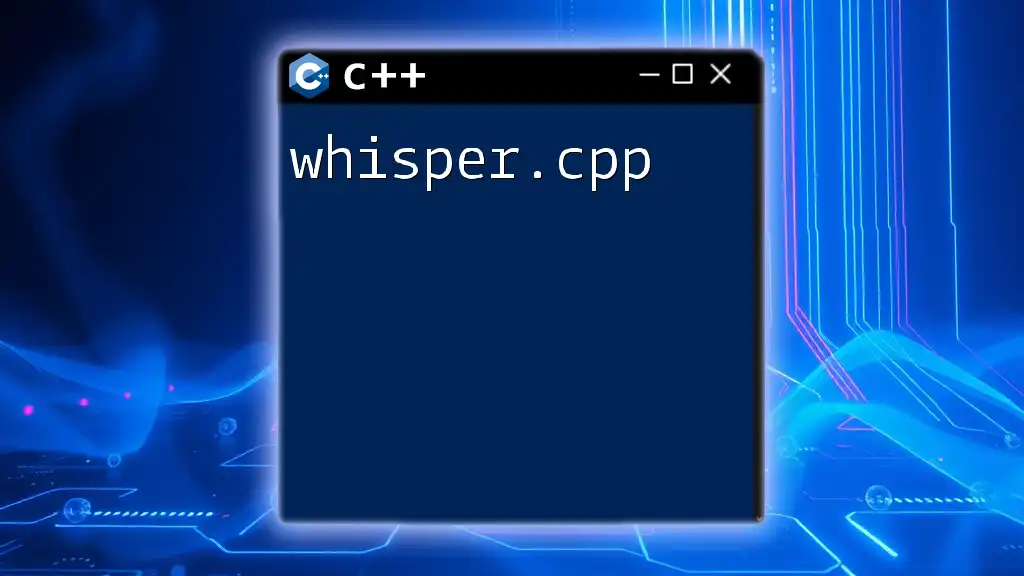
Optimization Techniques for Lowercasing Strings
Performance Considerations
When converting large strings or numerous strings in a performance-critical application, optimize your approach by minimizing unnecessary calls and reallocating memory. Both looping and using `std::transform` are efficient, but understanding the context and limits of data can greatly enhance performance.
Using Locale Awareness
Notably, string transformation can behave differently based on locales (regional settings and character bases). The `tolower` function by itself does not account for locale settings. To achieve locale-aware conversions, consider utilizing the `std::locale` functionalities available in C++. This helps ensure your application handles multilingual strings correctly and can adapt to the character expectations of different languages and regions.
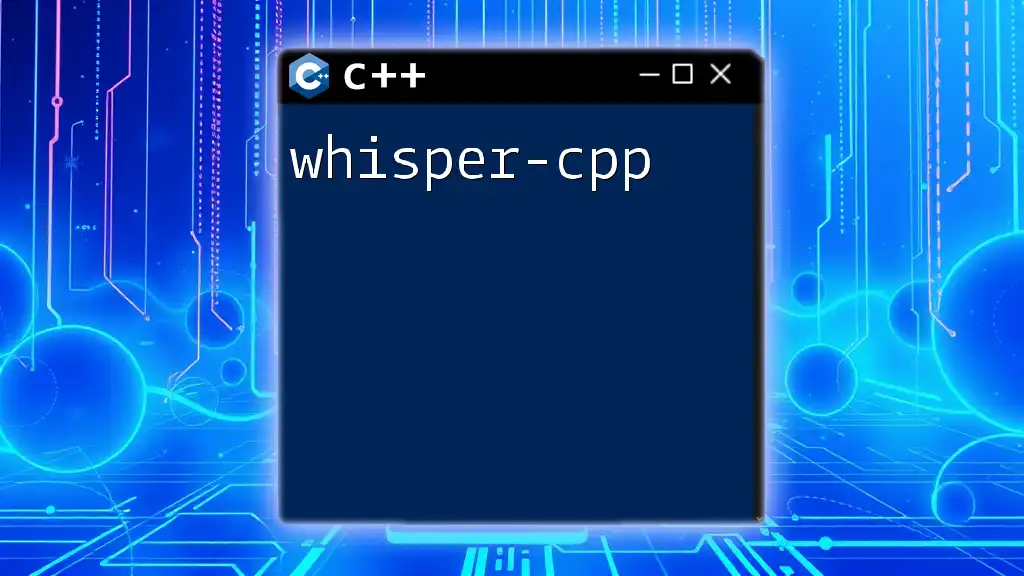
Conclusion
In C++, transforming strings to lowercase using the `tolower` function is an essential and straightforward operation that enhances data handling. It not only assures consistency in string operations but also supports effective user input management and search capabilities. Exploring these techniques will empower you to utilize C++ string manipulation to its fullest extent. As always, practice makes perfect; consider trying these methods with your own examples to solidify your understanding of how to effectively lower cpp.
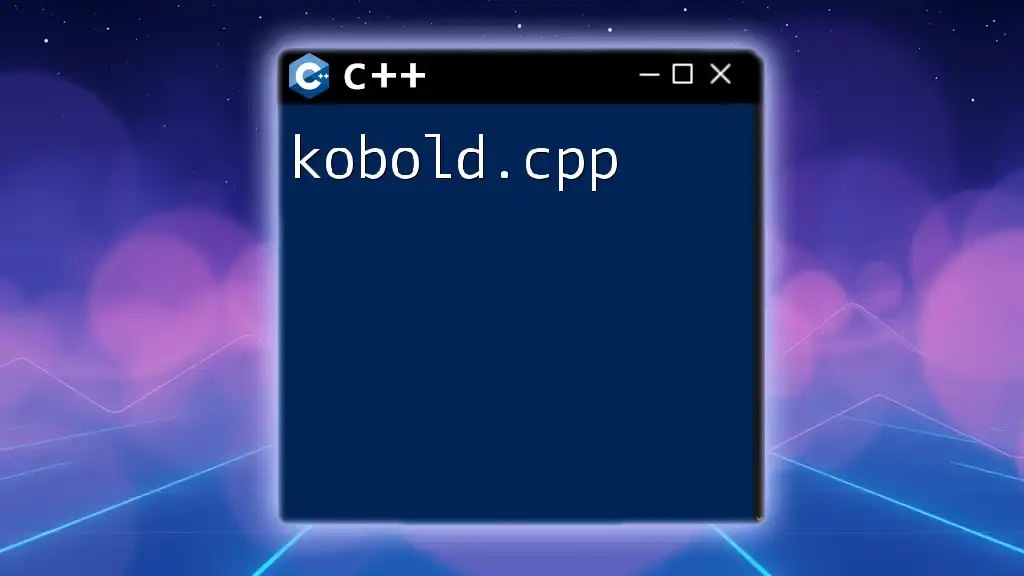
Additional Resources
Documentation Links
- Explore the official C++ documentation for `<cctype>` and its various functions.
Suggested Further Reading
- Books: Consider checking out well-regarded C++ programming books or online tutorials for deeper language insights.
With these foundational skills and your growing knowledge, you're well on your way to mastering string manipulation with C++.