In C++, the `float` data type is used to declare variables that can hold single-precision floating-point numbers, providing a way to represent decimal values.
float pi = 3.14f; // Declares a float variable named 'pi' and initializes it with the value 3.14
What is a Float in C++?
In C++, a float is a data type that is used to represent numbers with a fractional component. It can store decimal values and is particularly useful for calculations requiring precision, such as scientific computations or any form of graphics and game programming. It operates on a 32-bit architecture, which allows it to represent a wide range of numbers, albeit with some limitations in precision when compared to types like `double` or `long double`.
Differences Between Float, Double, and Long Double
- A float takes up 4 bytes of memory, typically providing 6-7 decimal digits of precision.
- A double occupies 8 bytes and offers about 15-16 decimal digits of accuracy.
- A long double can vary based on the compiler and platform, but it generally provides even greater precision than a double, often 10 bytes or more.
This variation provides developers with flexibility depending on their specific needs for performance and precision.
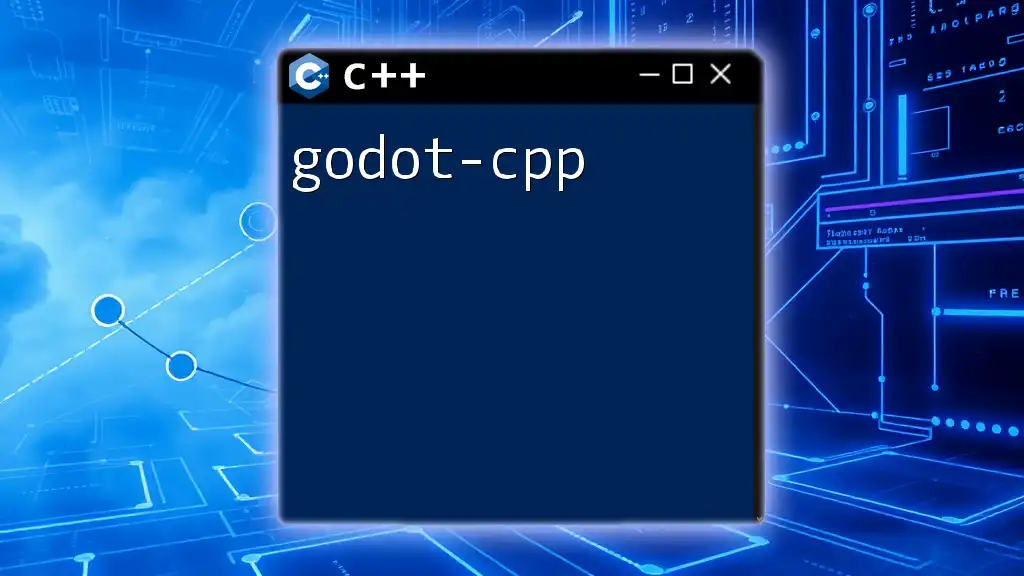
Why Use Floats in C++?
Floats are invaluable in programming for a myriad of reasons:
- Efficiency: When memory resources are limited, using `float` can significantly reduce memory consumption compared to using `double`.
- Performance: For many applications, the performance impact between `float` and `double` is minor; however, in specific contexts like gaming and real-time processing, `float` types can provide significantly faster calculations due to reduced processing time.
- Real-world Applications: Floats are heavily utilized in simulations, graphics (like OpenGL), and wherever decimal arithmetic is required.
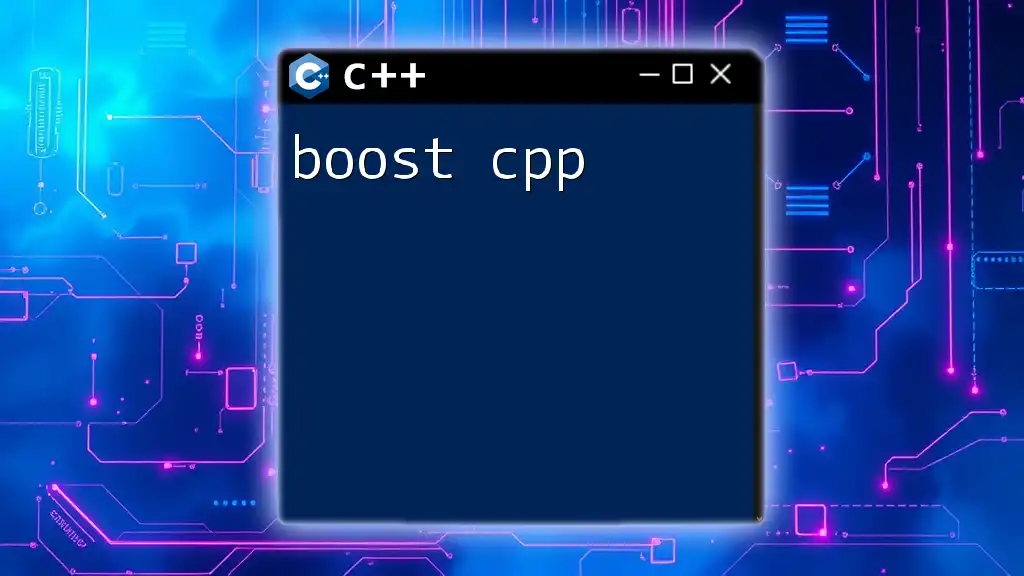
Declaring Float Variables in C++
Syntax for Declaring a Float
Declaring a float variable in C++ is straightforward. The basic syntax looks like this:
float myNumber;
In this example, `myNumber` can store a floating-point value.
Initializing Float Variables
There are several ways to initialize a float variable:
float myNumber = 5.5;
float myOtherNumber(10.0);
Both lines of code correctly initialize float variables. It’s also essential to note that if not initialized, a float variable will contain undefined data, which can lead to unpredictable behavior in your application.
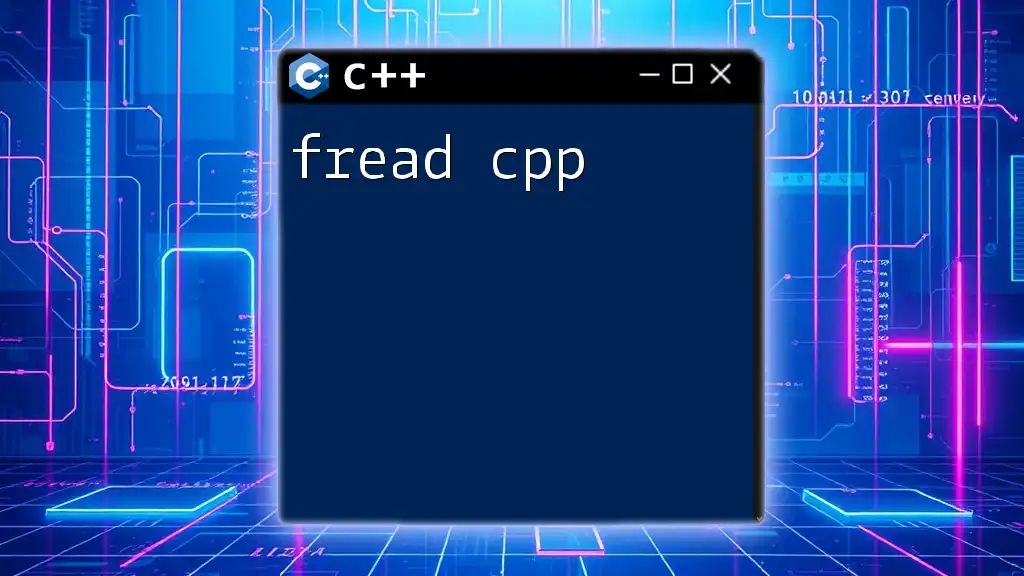
Operations with Float in C++
Basic Arithmetic Operations
Floats can be manipulated in various ways through arithmetic operations. Using a few examples:
float a = 5.5, b = 2.2;
float sum = a + b; // 7.7
float difference = a - b; // 3.3
float product = a * b; // 12.1
float quotient = a / b; // 2.5
These calculations demonstrate how floats can be combined mathematically just like integers. Each operation yields a result that maintains float precision.
Increment and Decrement Operators
Just like integers, floats can also utilize increment (`++`) and decrement (`--`) operators. Here's how they work:
float num = 5.5;
num++; // num is now 6.5
num--; // num is now 5.5
This flexibility makes float variables not just easy to handle, but also intuitive for performing common mathematical operations.
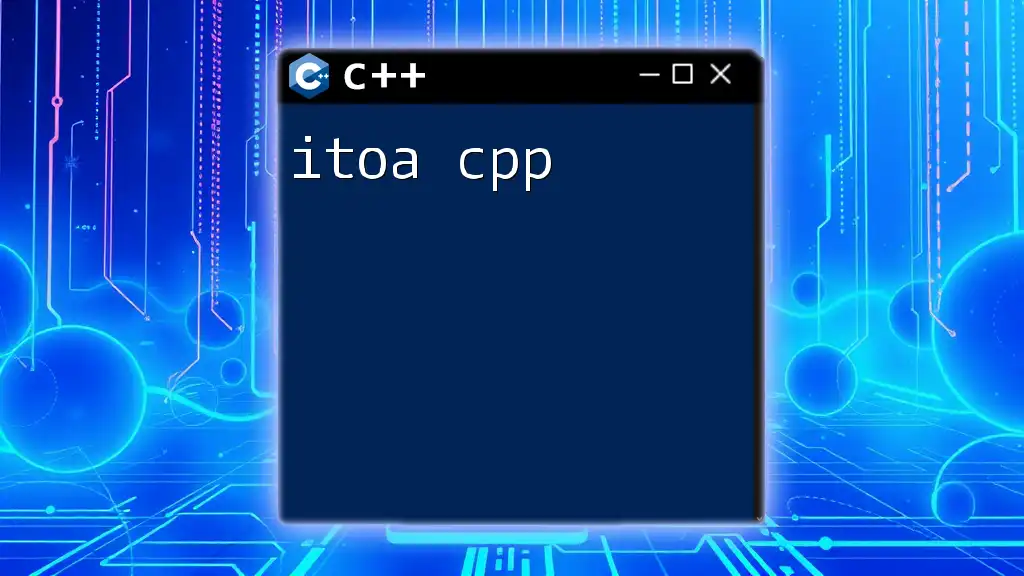
Floating-Point Precision
Understanding Floating-Point Precision
One of the key challenges with floating-point numbers is precision. Because floats represent numbers in binary, certain decimal values cannot be accurately represented. For example, the value `0.1` cannot be precisely expressed as a float.
Code Example for Precision Control
To manage precision in output, the `<iomanip>` library can be used alongside `std::setprecision()`. Here's how:
#include <iostream>
#include <iomanip>
int main() {
float pi = 3.14159;
std::cout << std::setprecision(3) << pi << std::endl; // Outputs: 3.14
return 0;
}
In this code, setting the precision ensures that the output is rounded, maintaining clarity without losing necessary detail.
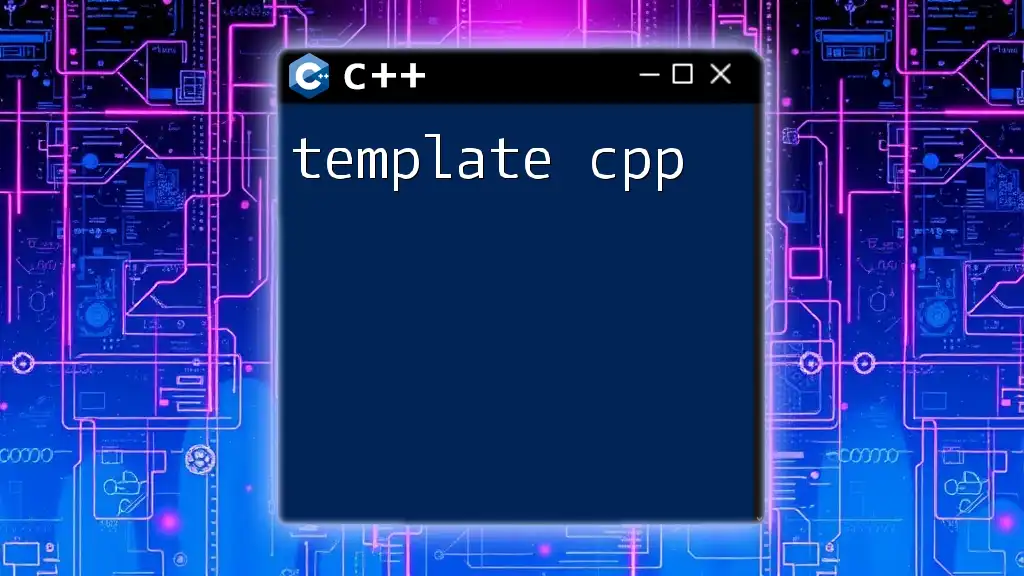
Common Functions for Floats in C++
Mathematical Functions
When working with floats, the C++ Standard Library provides a plethora of mathematical functions. Some common functions include:
- `sqrt()` for calculating the square root.
- `pow()` for raising a number to a certain power.
- `fabs()` to get the absolute value.
Here’s a code snippet illustrating `sqrt()` in action:
#include <cmath>
float result = sqrt(16.0f); // 4.0
Each of these functions allows for advanced numerical computations, making floats incredibly powerful in practical applications.
Casting with Floats
In scenarios where type conversion is necessary, casting between types can be employed. Static casting in particular allows for explicit conversion:
int a = 5;
float b = static_cast<float>(a); // 5.0
This way, values can be converted safely and predictably (e.g., from an integer to a float).
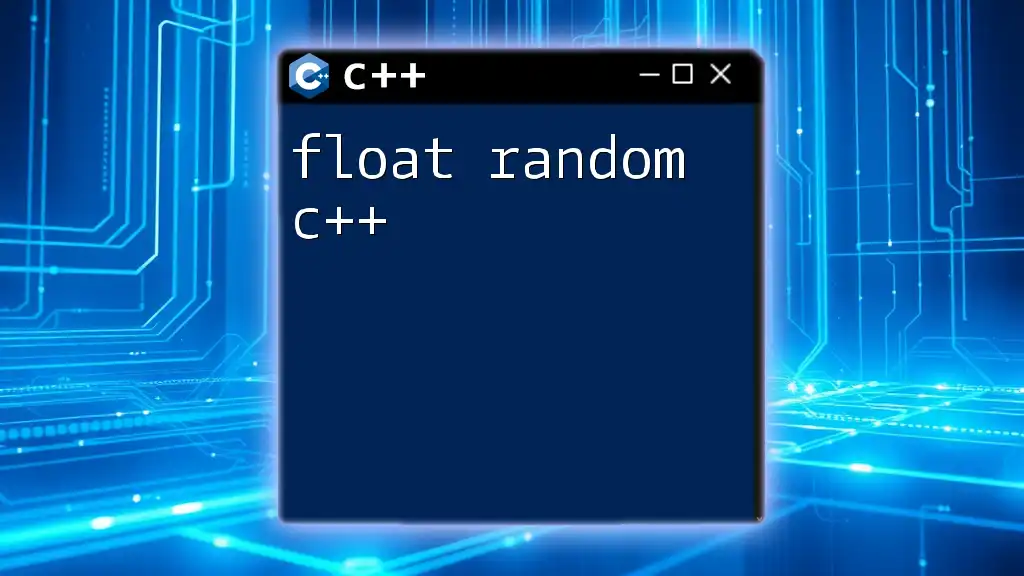
Best Practices for Using Floats in C++
Choosing Between Float and Double
A common question is when to use `float` versus `double`. Generally, floats should be employed for:
- Applications requiring less precision, such as graphics.
- Performance-critical contexts, where the slight increase in speed with float is a significant advantage.
Double should be preferred when:
- High precision is required, such as in financial applications.
- The risk of floating-point precision errors could lead to significant issues.
Avoiding Common Pitfalls
With floating-point math, precision loss is a common issue. Developers must be wary of results that seem mathematically incorrect, caused by the way floats represent numbers. Strategies to mitigate precision issues include:
- Using higher precision types (e.g., double) where necessary.
- Regularly checking calculations against expected results.
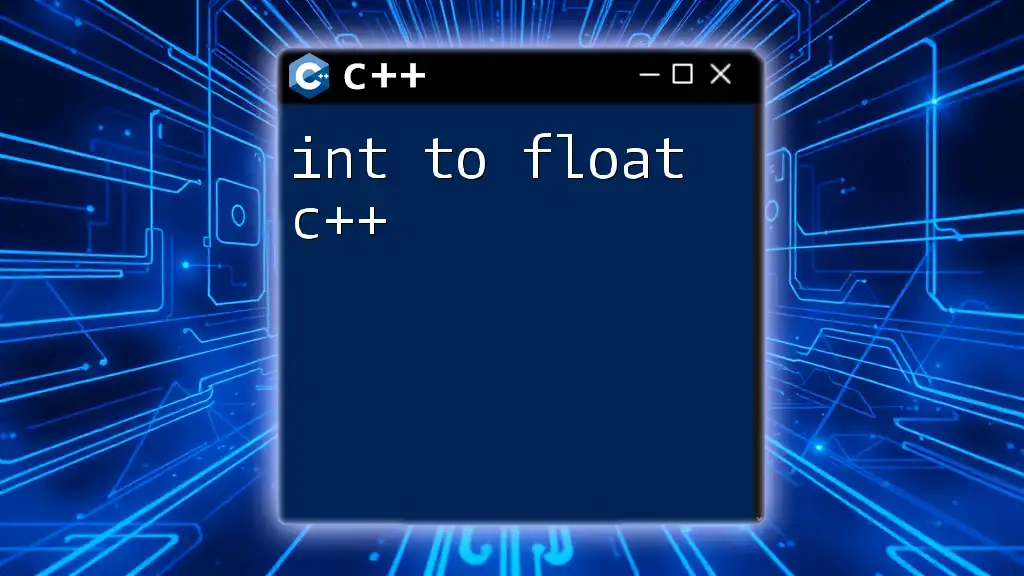
Example Projects Involving Floats in C++
Simple Calculator
Creating a simple calculator is an excellent way to showcase basic float operations. The following is a starting point for a console-based calculator:
#include <iostream>
using namespace std;
int main() {
float num1, num2, result;
char operation;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter second number: ";
cin >> num2;
cout << "Enter operation (+, -, *, /): ";
cin >> operation;
switch (operation) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0) {
result = num1 / num2;
} else {
cout << "Error: Division by zero!" << endl;
return 1;
}
break;
default:
cout << "Invalid operation!" << endl;
return 1;
}
cout << "Result: " << result << endl;
return 0;
}
This simple program illustrates how to handle floats in input/output and arithmetic operations.
Simulating Physics Calculations
Another compelling project could involve physics calculations, such as calculating projectile motion, where gravity effects and initial velocities can be modeled using floats for precision.
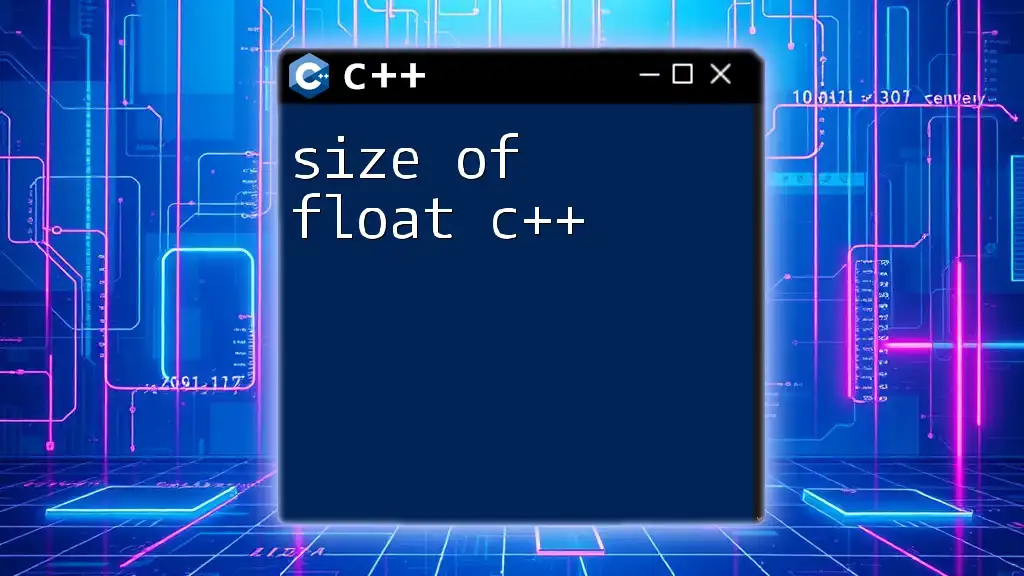
Recap of Float in C++
Floats in C++ provide a robust and efficient way to handle fractional numbers while playing a critical role in numerous programming applications. From basic arithmetic to more complex mathematical functions, grasping how to effectively utilize floats will enhance your coding proficiency.
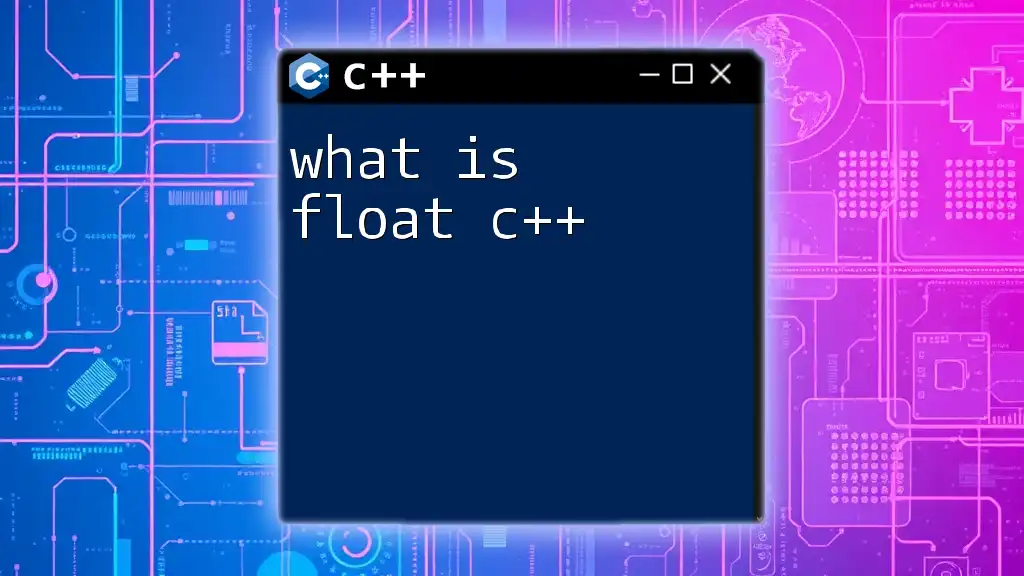
Encouraging Further Learning
To deepen understanding, consider exploring topics such as numerical methods, advanced data types, and comparative studies between different data representation techniques. Engaging with these areas can provide newfound insights and improve overall programming skills.
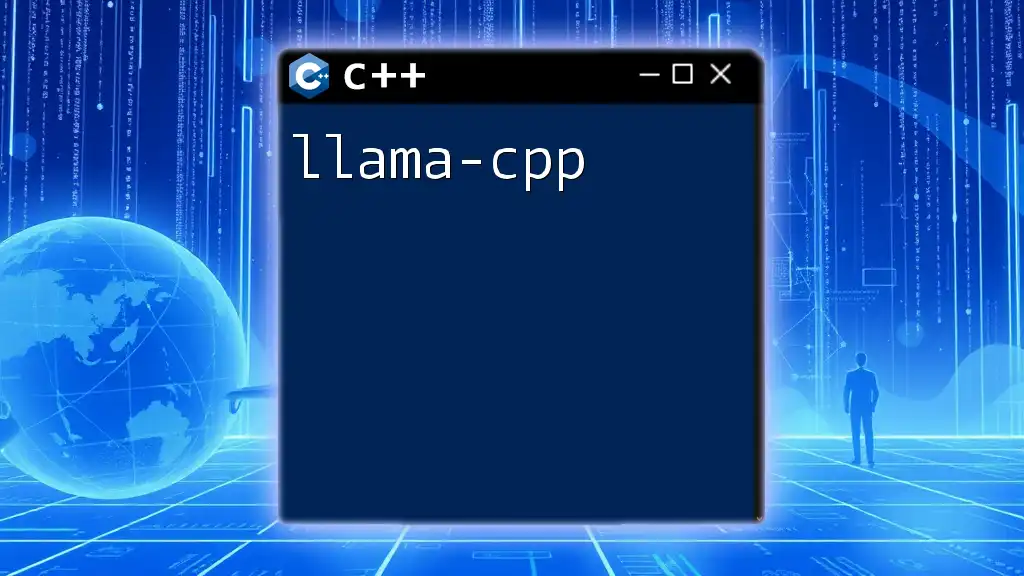
Further Reading and Links
For additional resources, consider looking into:
- The official C++ documentation for insights on mathematical functions and type conversions.
- Online forums and communities like Stack Overflow for coding help and best practices.
- Programming courses focusing on C++ that can provide hands-on experience with floats and other data types.