In C++, you can generate a random floating-point number between two values using the `<random>` library along with a uniform distribution.
Here's a code snippet demonstrating how to do this:
#include <iostream>
#include <random>
float randomFloat(float min, float max) {
static std::random_device rd; // Obtain a random number from hardware
static std::mt19937 eng(rd()); // Seed the generator
std::uniform_real_distribution<float> dist(min, max); // Define the range
return dist(eng); // Generate and return random number
}
int main() {
std::cout << randomFloat(1.0f, 10.0f) << std::endl; // Example usage
return 0;
}
Understanding Random Number Generation in C++
What is Random Number Generation?
Random number generation (RNG) is the process of creating a sequence of numbers that cannot be reasonably predicted better than by random chance. It plays a vital role in various applications such as cryptography, simulations, statistical sampling, and gaming. Understanding RNG is crucial for developers as it helps in creating behavior that mimics randomness, which is essential in many programming scenarios.
The Need for Floating Point Randomization
While it's common to generate random integers, the ability to produce random floating-point numbers is equally important, particularly in domains where precision and a continuum of values are required. Some examples of where random floating-point numbers become crucial include:
- Graphics and Simulations: Often used in rendering algorithms and physics simulations to create natural-looking movements and behaviors.
- Statistical Analysis and Modeling: Random floats are fundamental in creating realistic models and performing analyses that require sampling from a continuous range.
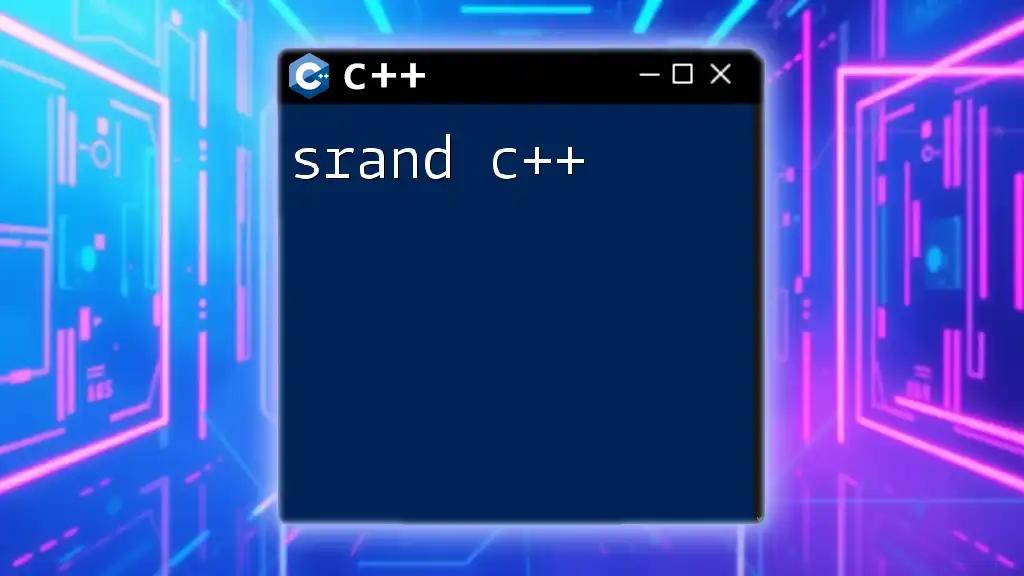
C++ Libraries for Random Number Generation
The `<random>` Header
The introduction of the `<random>` library in C++11 revolutionized how developers generate random numbers. Unlike previous approaches that relied on `rand()`, which had several limitations regarding randomness and distributions, the `<random>` library provides better algorithms and distributions.
Some benefits of using `<random>` include:
- Higher quality randomness: It offers sophisticated random number engines that provide improved distribution quality.
- Flexible distributions: It supports various distributions, allowing fine control over the types of random numbers generated.
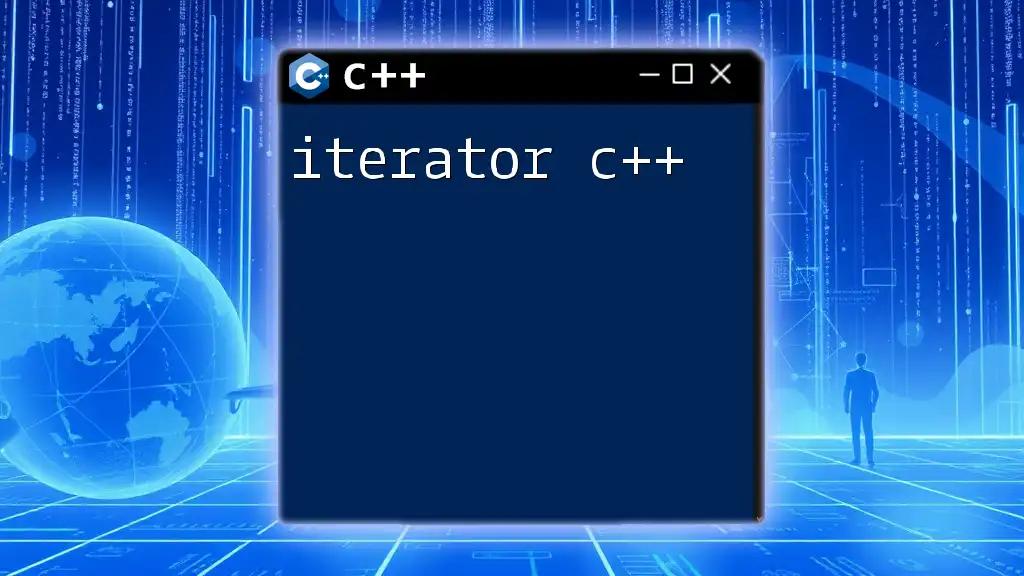
Generating Random Floats in C++
Basic Structure for Generating Random Numbers
To generate random floats in C++, you need to understand three main components: the random engine, the distribution, and the defined range. The process generally follows these steps:
- Instantiate a random engine (e.g., `std::default_random_engine`).
- Define a distribution, such as `std::uniform_real_distribution`, which determines the type of random values (uniform across a specified range).
- Use the engine and distribution together to produce random values.
Using `std::default_random_engine`
The `std::default_random_engine` is a commonly used random engine in C++. It provides a way to generate random numbers where the underlying algorithm is implementation defined, typically offering a good balance of speed and randomness. Here's a basic code demonstration:
#include <random>
#include <iostream>
int main() {
std::default_random_engine generator; // Random engine
// Generate a random float between 0 and 1
std::uniform_real_distribution<float> distribution(0.0, 1.0);
float random_float = distribution(generator);
std::cout << "Random float: " << random_float << std::endl;
return 0;
}
This snippet creates a random float between 0 and 1. The `std::uniform_real_distribution` ensures that the generated float is uniformly distributed within the specified range.
Customizing the Range of Random Floats
To generate random floating-point numbers within a specific range, you can simply adjust the parameters of `uniform_real_distribution`. Here’s an example that generates random floats between 1.5 and 5.5:
#include <random>
#include <iostream>
int main() {
std::default_random_engine generator;
std::uniform_real_distribution<float> distribution(1.5, 5.5);
float random_float_range = distribution(generator);
std::cout << "Random float in range [1.5, 5.5]: " << random_float_range << std::endl;
return 0;
}
In this example, you see the flexibility offered by distributions to specify exactly how random numbers should be generated.

Advanced Techniques for Random Float Generation
Using Other Distributions
Apart from uniform distribution, the `<random>` library provides other types of distributions such as:
- Normal Distribution (`std::normal_distribution`): Generates random numbers following a bell curve (Gaussian distribution).
- Exponential Distribution (`std::exponential_distribution`): Useful for generating random numbers that follow an exponential decay pattern, commonly used in queueing theory.
Here’s an example of generating random floats using a normal distribution:
#include <random>
#include <iostream>
int main() {
std::default_random_engine generator;
std::normal_distribution<float> distribution(0.0, 1.0); // Mean 0, StdDev 1
for(int i = 0; i < 10; i++) {
float random_float_normal = distribution(generator);
std::cout << "Random float from normal distribution: " << random_float_normal << std::endl;
}
return 0;
}
Creating Your Own Random Number Generator
If you need a simple implementation for random float generation without relying on the standard library, you can create a custom function. Here's a possible implementation:
#include <iostream>
#include <cstdlib>
#include <ctime>
float custom_random_float(float min, float max) {
return min + static_cast<float>(rand()) / (static_cast<float>(RAND_MAX / (max - min)));
}
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed the random number generator
float random_float_custom = custom_random_float(10.0, 20.0);
std::cout << "Custom Random Float: " << random_float_custom << std::endl;
return 0;
}
In this code, we implement a function `custom_random_float` that generates a random float between specified minimum and maximum values. Notice how we seed the RNG with `srand()` to ensure that we get different results each time the program is run.
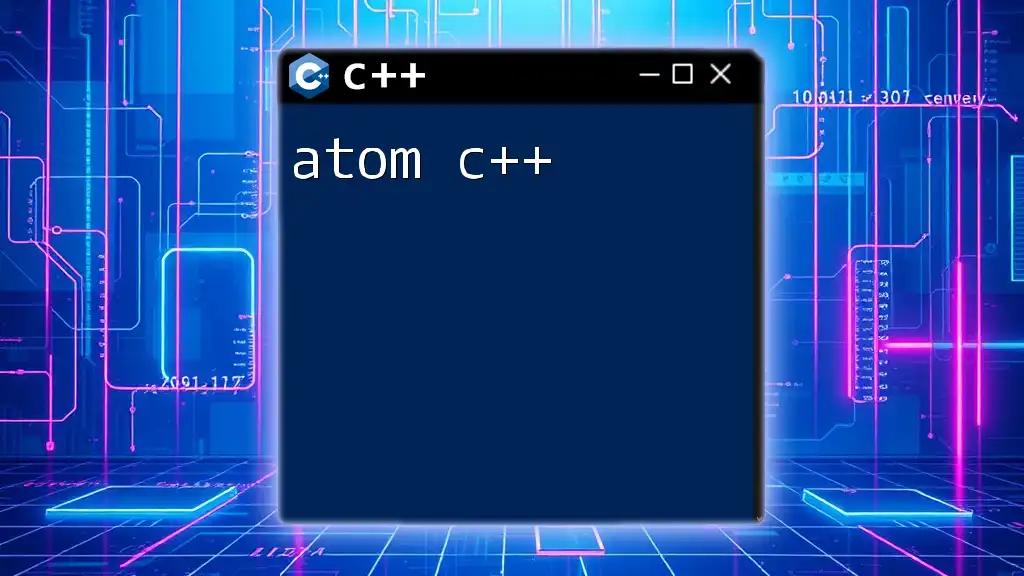
Common Pitfalls and Best Practices
Avoiding Common Mistakes
When working with random number generation in C++, there are some common pitfalls that developers should be aware of:
- Using `rand()`: While `rand()` might seem convenient, it often leads to poor randomness and should generally be avoided in favor of the `<random>` library.
- Failing to Seed the Generator: Always seed your random number generator with a unique value (like the current time) to prevent getting the same sequence of random numbers with each execution.
Best Practices for Random Float Generation
To maximize the effectiveness of your random float generation, consider following these best practices:
- Seed your random number generator appropriately before generating random numbers to ensure better randomness.
- Select the right distribution based on your application's needs. Different distributions can lead to vastly different results, impacting the application significantly.
- Test and Analyze Generated Values, especially in critical applications, to evaluate the distribution and characteristics of the generated random floats, ensuring they meet your expectations and requirements.
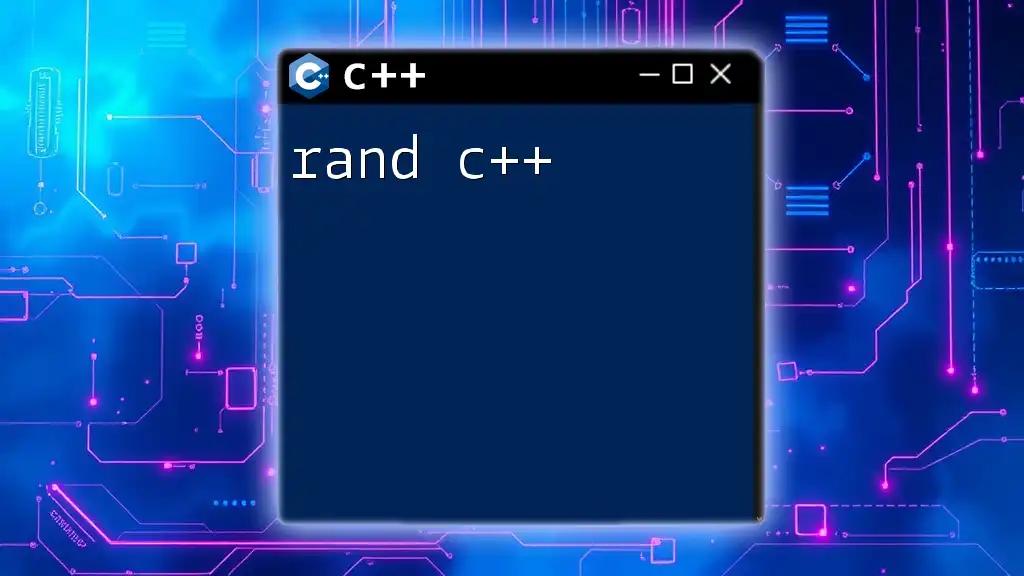
Conclusion
In this guide, we explored the generation of random floats in C++ using the `<random>` library, detailing various techniques, functions, and best practices. The ability to generate random floating-point numbers is a powerful tool for developers, enhancing the realism and unpredictability in numerous applications.
Encouragement is given for readers to practice generating random floats, experiment with different distributions, and explore their applications further. The skill in random float generation is invaluable, paving the way for sophisticated simulation, statistical modeling, and more.
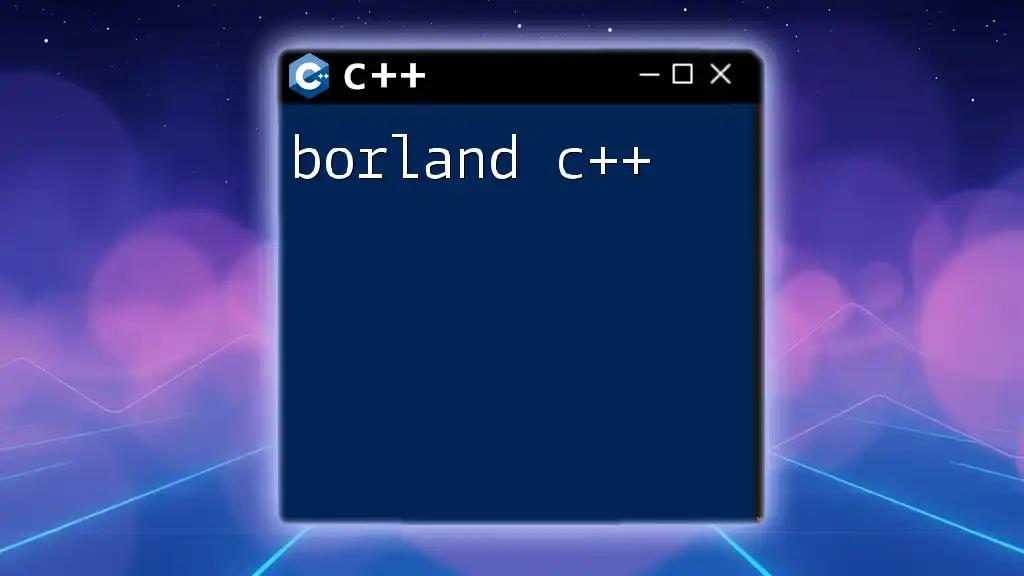
Additional Resources
To deepen your understanding of random number generation in C++, consider exploring the following resources:
- Books on C++ programming focusing on advanced topics, particularly those that cover the standard library comprehensively.
- Online Tutorials and Documentation for the `<random>` library to harness its full potential.
- Peer-reviewed papers and materials related to RNG algorithms to understand different methodologies and implementations.
Feedback and Questions
Comments and questions regarding float random generation in C++ are highly encouraged. Readers are invited to bring up challenges faced or suggest topics for future articles related to random number generation or other C++ programming techniques!