Notepad++ is a powerful text editor that is often used for writing and editing C++ code due to its syntax highlighting, code folding, and customizable features which enhance productivity.
Here's a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What Is Notepad++?
Notepad++ is a widely-used text editor, praised for its versatility and speed. Unlike basic text editors, it supports multiple programming languages, including C++. It provides features essential for coding, such as syntax highlighting, code folding, and multi-document support. These functionalities make it easier to write and manage code, enhancing the overall programming experience for both beginners and seasoned developers.
Why Choose Notepad++ for C++ Development?
When selecting a development environment, consider these compelling reasons to use Notepad++ for your C++ projects:
- Lightweight and Fast Performance: Notepad++ is an efficient program that opens quickly, making it ideal for quick code edits.
- Customization Options and Extensibility: Users can tailor the editor to their preferences by modifying themes and installing plugins.
- Free and Open-Source Nature: The software is completely free, making it accessible for anyone interested in learning C++ without financial barriers.
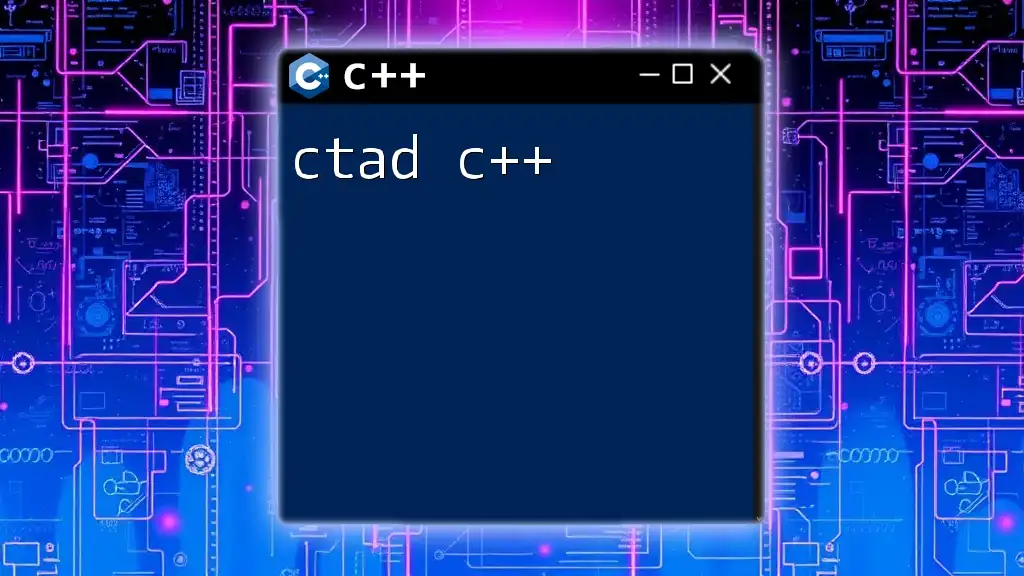
Installation Guide
Getting started with Notepad++ is straightforward. Begin by downloading the installer from the [official Notepad++ website](https://notepad-plus-plus.org/downloads/). Once the installer is downloaded:
- Run the Installer: Follow the prompts to complete the installation process.
- System Requirements: Ensure your system meets the requirements, which are minimal for Notepad++ to function properly. Most modern systems will be compatible.
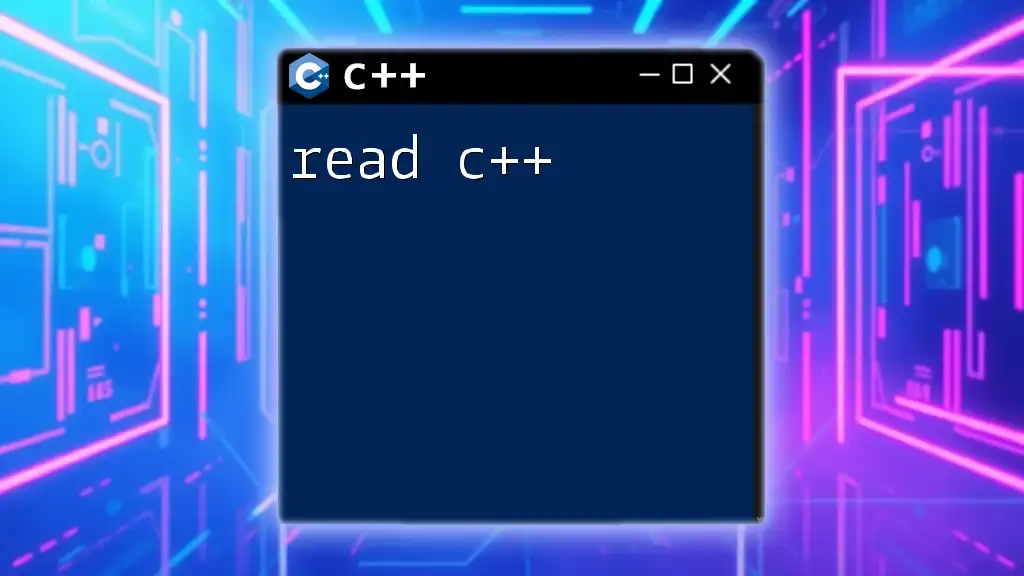
Setting Up the Environment for C++
To use Notepad++ efficiently for C++ development, you will need to install a C++ compiler. The GNU Compiler Collection (g++) is a popular choice. Here’s how to set it up:
- Install g++: You can download it through package management systems like MinGW for Windows or directly install it using package managers on Linux and macOS.
- Setting Environment Variables: Ensure that your system recognizes the `g++` command. Add the path to your compiler's bin directory to your system's environment variables.
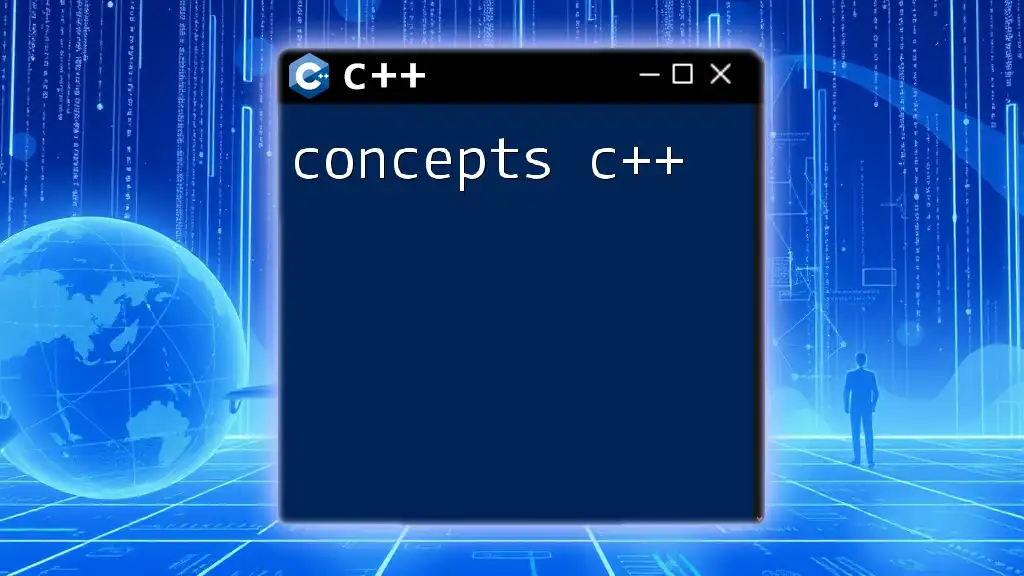
Understanding the User Interface
The user interface of Notepad++ is designed to maximize usability:
- Menus: Access various functions like File, Edit, Search, etc.
- Toolbar: Quickly access common tasks such as opening or saving files.
- Status Bar: Displays important information about the current document, such as the line number and column.
Customizing the Interface
To tailor the editor to your preferences, Notepad++ offers extensive customization options. You can adjust the following:
- Themes and Fonts: Change the appearance to prevent eye strain and enhance readability.
- Line Numbers and Syntax Highlighting: Enable these features in the "View" menu for a more structured code-writing experience. This makes it easier to identify code errors.
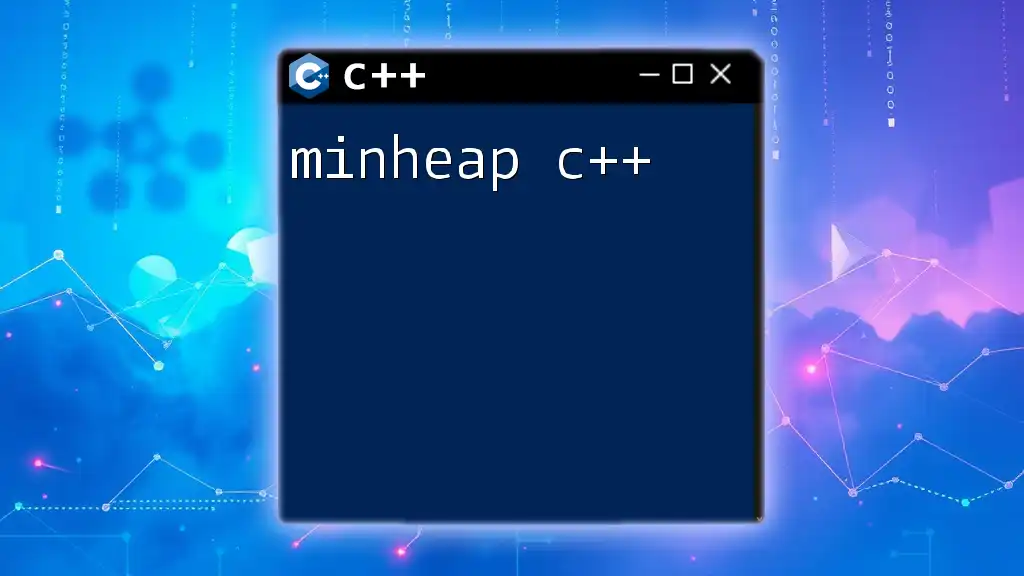
Writing Your First C++ Program
Creating your first C++ program in Notepad++ is simple. To begin:
Creating a New C++ File
Open Notepad++, then go to File > New. You now have a blank canvas to write your code.
Writing a Basic C++ Program
Here’s an example of a simple program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code includes the iostream library, which is necessary for input and output functionalities. The `main` function marks the starting point of the program, whereas `std::cout` is used to display text in the console. Understanding this structure is foundational for any C++ developer.
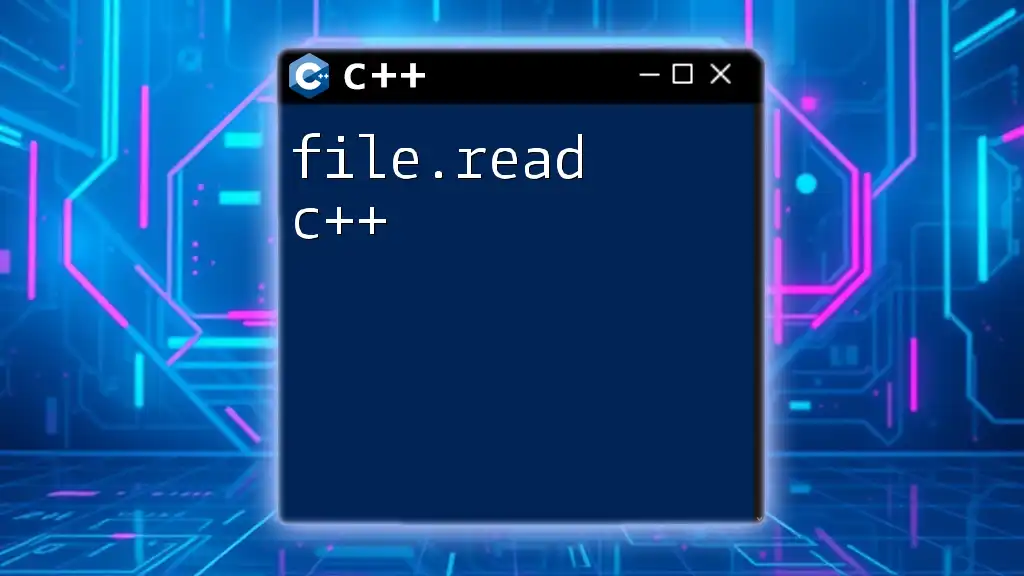
Compiling and Running C++ Code in Notepad++
To compile and run your C++ code, you might want to use an external compiler, like g++. Here’s how you can do it:
Using the Built-in Compiler
Set up Notepad++ to integrate a build system. To configure it, follow these steps in Notepad++:
- Go to Run > Run...
- Enter the following command (update the file name accordingly):
g++ "$(NAME_PART)" -o "$(NAME_PART)"; "$(NAME_PART)"
- Save this command to a shortcut for quicker access.
Using External Compiler (g++)
To compile your C++ program externally, you can follow this step-by-step compilation process:
- Save your C++ file as `hello.cpp`.
- Open the Command Prompt (Windows) or Terminal (Linux/Mac).
- Navigate to the directory where your file is saved.
- Use the following command to compile and run:
g++ hello.cpp -o hello ./hello
In this command, `g++ hello.cpp -o hello` compiles the code and produces an executable named `hello`. The `./hello` command then runs the program. Each step in this process is crucial for successfully executing your code.
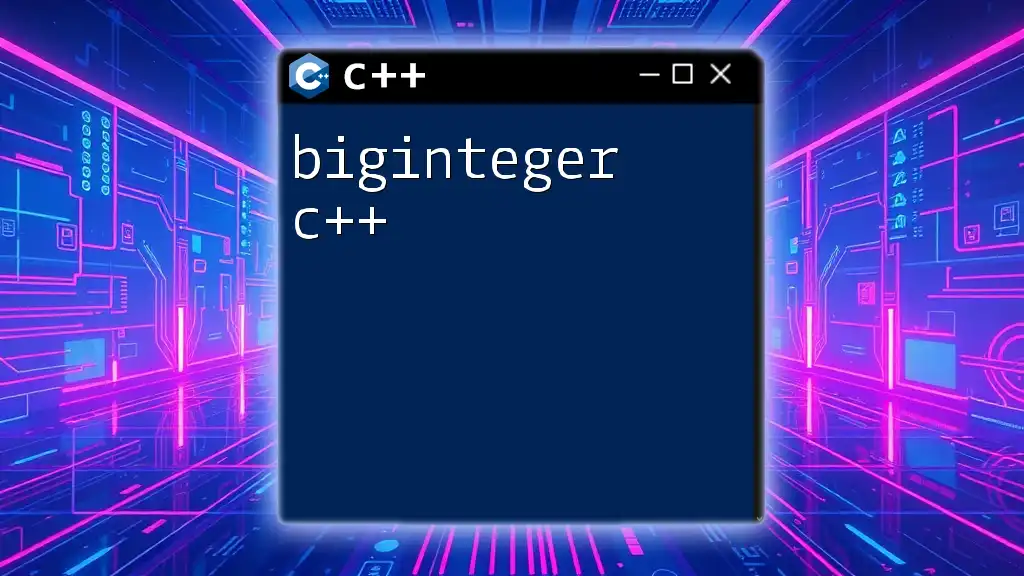
Advanced Features of Notepad++
Notepad++ comes with various advanced features that can enhance your C++ development experience.
Plugins and Add-ons
The editor supports multiple plugins that extend its functionality. Some notable plugins for C++ development include:
- NppExec: This plugin allows you to execute scripts and commands inside Notepad++, enabling advanced functionalities.
- JSON Viewer: Great for handling JSON data structures if your project involves APIs.
How to Install and Configure Plugins
To install plugins, go to Plugins > Plugins Admin. You can search for plugins, check them, and install them with ease.
Macro Recording for Repetitive Tasks
Notepad++ allows you to record macros, which can automate repetitive tasks. For instance, if you often perform a specific formatting task, you can record a macro to execute that task in a few clicks.
- Navigate to Macro > Start Recording.
- Perform the actions you wish to automate.
- Stop recording by going back to Macro > Stop Recording.
- Save the macro for future use.
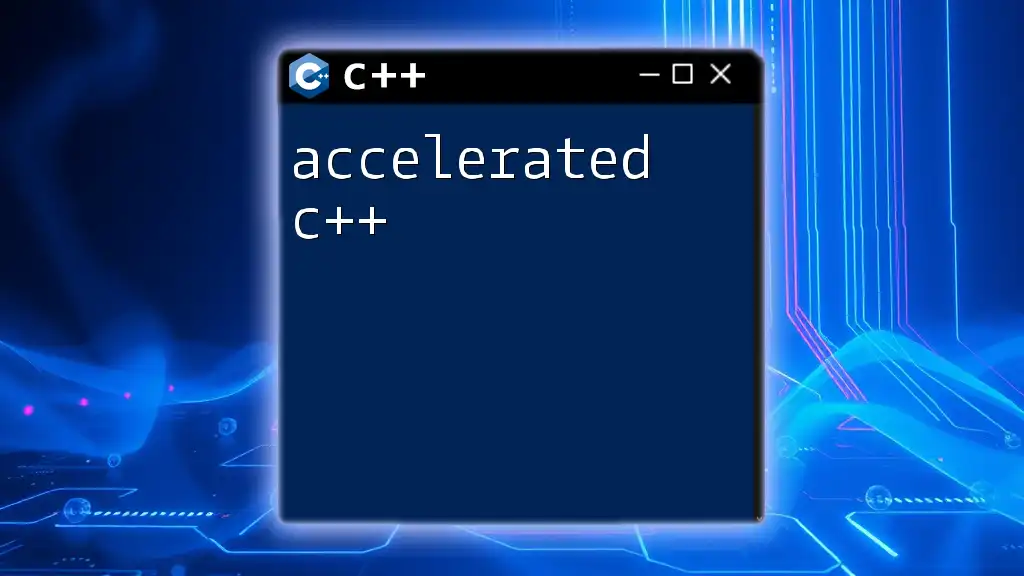
Debugging C++ Code in Notepad++
Understanding how to debug your code is essential for any developer.
Basic Debugging Techniques
When you encounter errors, check for common mistakes such as:
- Syntax errors: Look for missing semicolons or braces.
- Logic errors: Ensure your logic aligns with your intended outcome.
- Compilation warnings: Always address these, as they can lead to errors during execution.
Using an External Debugger with Notepad++
Integrating an external debugger, such as GDB, can provide you with powerful debugging capabilities:
- Set up GDB on your system according to your operating system’s guidelines.
- Configure Notepad++ to link to GDB. You can use a plugin like NppExec to facilitate this integration.
- Follow a specific workflow to compile and debug your code effectively.
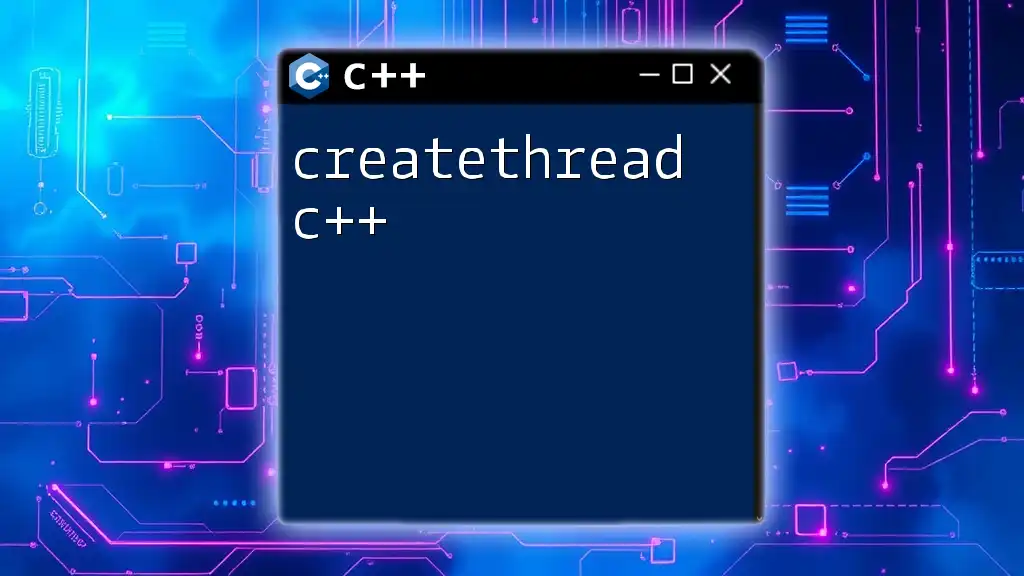
Customizing Notepad++ for C++ Development
Tailoring Notepad++ for C++ development can significantly enhance your coding efficiency.
Creating Custom Syntax Highlighting Rules
You can create and implement custom syntax highlighting rules by customizing the language definitions. This allows you to differentiate your code components better and increase readability. Access these settings in Language > Define your language.
Snippets and Shortcuts
Creating code snippets for frequently used code helps streamline your workflow. To make a snippet:
- Select the code you often use.
- Save it as a snippet in your personal library.
- Assign a keyboard shortcut for quick access.
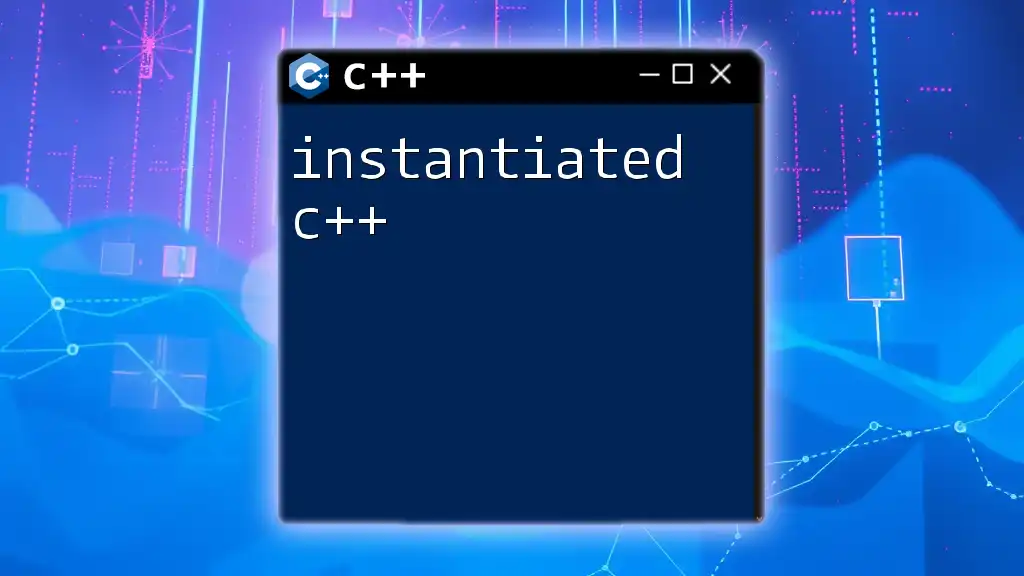
Conclusion
Using Notepad++ for C++ development offers numerous advantages. Its lightweight nature, customization options, and support for various programming features make it an excellent choice for both beginners and experts. Engaging in practice with the setup, writing code, and utilizing advanced features will strengthen your coding skills, preparing you for more complex projects.
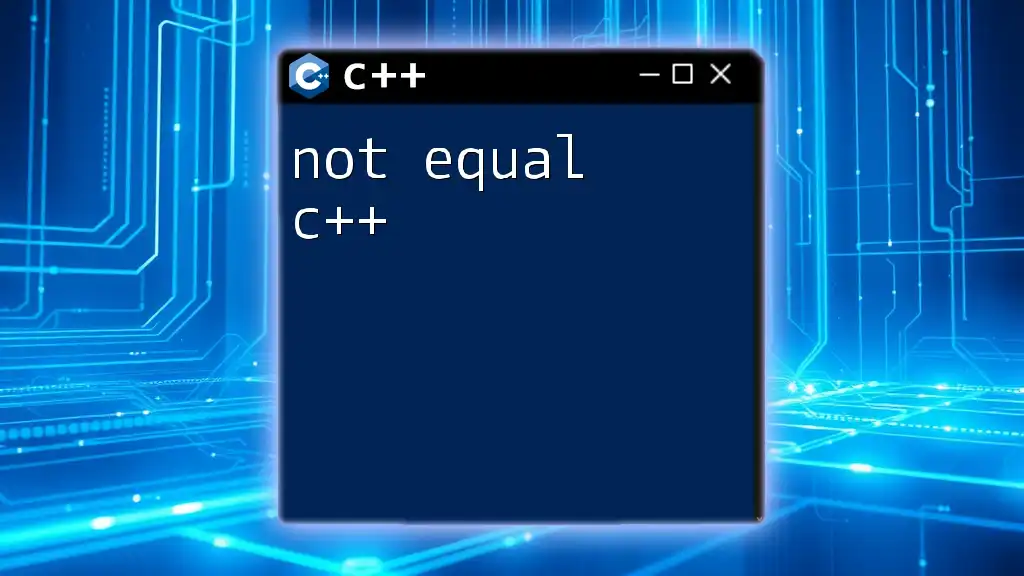
Additional Resources
For more advanced techniques, you might explore online tutorials, official documentation, and community forums. These platforms provide invaluable support and further enhance your learning journey.
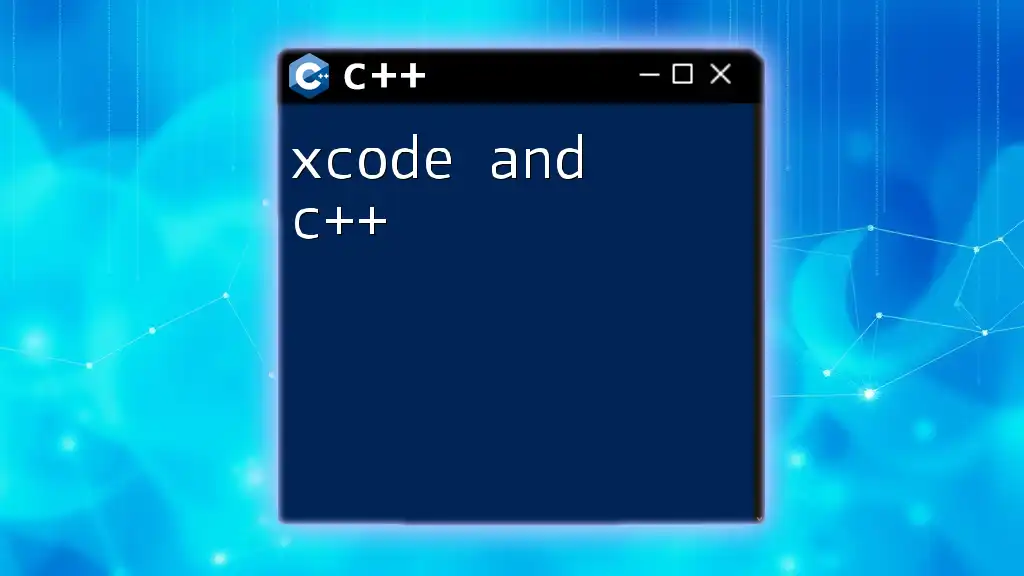
Community and Support
If you run into issues or have questions, consider engaging with the Notepad++ community on forums or GitHub. You can find assistance, share knowledge, and connect with other eager learners and professionals.