Object-Oriented Programming (OOP) in C++ is a programming paradigm that uses objects and classes to organize code, promote code reuse, and enable encapsulation, inheritance, and polymorphism.
Here's a simple example of OOP in C++:
class Animal {
public:
void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
class Dog : public Animal {
public:
void speak() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Animal myAnimal;
myAnimal.speak(); // Output: Animal speaks
Dog myDog;
myDog.speak(); // Output: Woof!
return 0;
}
Core Concepts of Object-Oriented Programming
Classes and Objects
Understanding Classes
A class in C++ serves as a blueprint for creating objects. It is a user-defined data type that contains variables (attributes) and functions (methods) to perform operations on those attributes. By encapsulating data and functions into a single unit, classes help in organizing code logically.
Here’s a simple example of a class in C++:
class Car {
public:
string brand;
int year;
void displayInfo() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
What are Objects?
An object is an instance of a class. While a class defines a type of object, an object represents a specific instance of that type with actual values. You can create multiple objects from the same class, each with its own properties.
For example, to create and use an object from the `Car` class:
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2021;
myCar.displayInfo();
Encapsulation
Defining Encapsulation
Encapsulation is the practice of restricting access to certain details of an object, such as data members and methods, to protect the integrity of the object's state. In C++, this is typically achieved using access specifiers: `public`, `private`, and `protected`.
Benefits of Encapsulation
Encapsulation enhances security, reduces complexity by hiding unnecessary details from the user, and promotes data integrity by preventing unintended interactions.
Here’s an example demonstrating encapsulation:
class BankAccount {
private:
double balance;
public:
BankAccount() : balance(0) {}
void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
double getBalance() {
return balance;
}
};
Inheritance
Understanding Inheritance
Inheritance is a mechanism wherein a new class (derived class) inherits properties and methods from an existing class (base class). This allows for code reusability and the creation of a hierarchical relationship between classes.
Types of Inheritance
- Single Inheritance: A single derived class inherits from one base class.
- Multiple Inheritance: A derived class inherits from multiple base classes.
- Multilevel Inheritance: A chain of classes where each derived class inherits from a base class.
Example of single inheritance:
class Vehicle {
public:
void honk() {
cout << "Honk!" << endl;
}
};
class Car : public Vehicle {
public:
void display() {
cout << "I am a car!" << endl;
}
};
Car myCar;
myCar.honk(); // Inherited method
myCar.display();
Polymorphism
What is Polymorphism in C++?
Polymorphism allows functions or methods to perform differently based on the object that it is acting upon. There are two types of polymorphism: compile-time (method overloading) and runtime (method overriding).
Method Overloading vs. Method Overriding
- Method Overloading: Multiple methods in the same class have the same name but different parameters.
- Method Overriding: A derived class provides a specific implementation of a method that is already defined in its base class.
Example of method overloading:
class Math {
public:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
};
Example of method overriding:
class Animal {
public:
virtual void sound() {
cout << "Animal sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Woof!" << endl;
}
};
Dog myDog;
myDog.sound(); // Outputs: Woof!
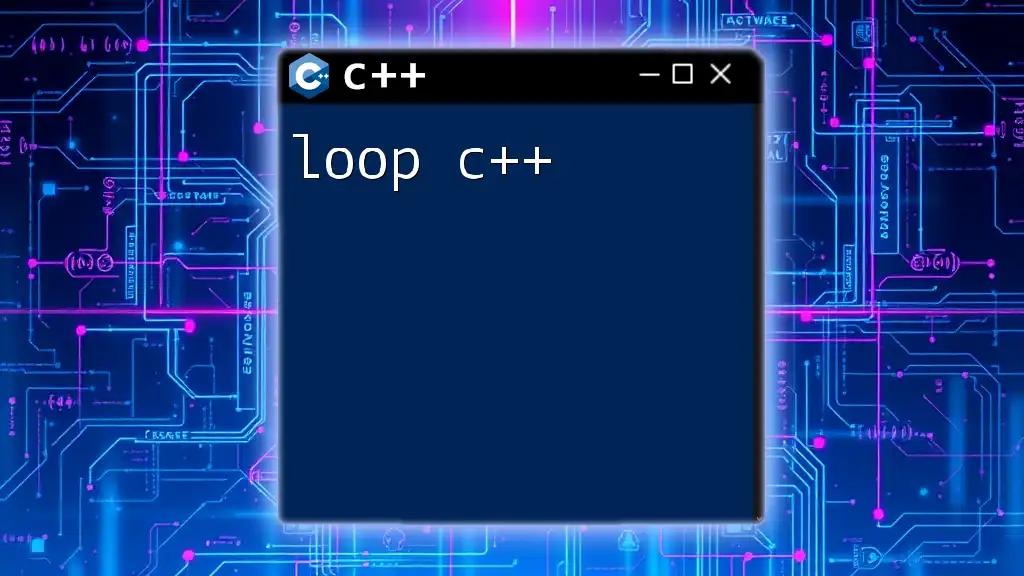
Object-Oriented Programming Principles
SOLID Principles
Breaking Down SOLID
The SOLID principles are a set of design principles that help developers create more understandable, flexible, and maintainable software. These principles include:
- Single Responsibility Principle: A class should have only one reason to change.
- Open-Closed Principle: Classes should be open for extension but closed for modification.
- Liskov Substitution Principle: Objects of a superclass should be replaceable with objects of a subclass without affecting the program.
- Interface Segregation Principle: No client should be forced to depend on methods it does not use.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules. Both should depend on abstractions.
The Four Pillars of OOP
Encapsulation, Abstraction, Inheritance, Polymorphism
These four pillars form the foundation of OOP:
-
Encapsulation ensures that the internal representation of an object is hidden from the outside.
-
Abstraction reduces complexity by providing a simplified interface to the user while hiding unnecessary details.
-
Inheritance promotes code reusability and establishes a relationship between classes.
-
Polymorphism allows for flexibility and the ability to redefine methods as needed.
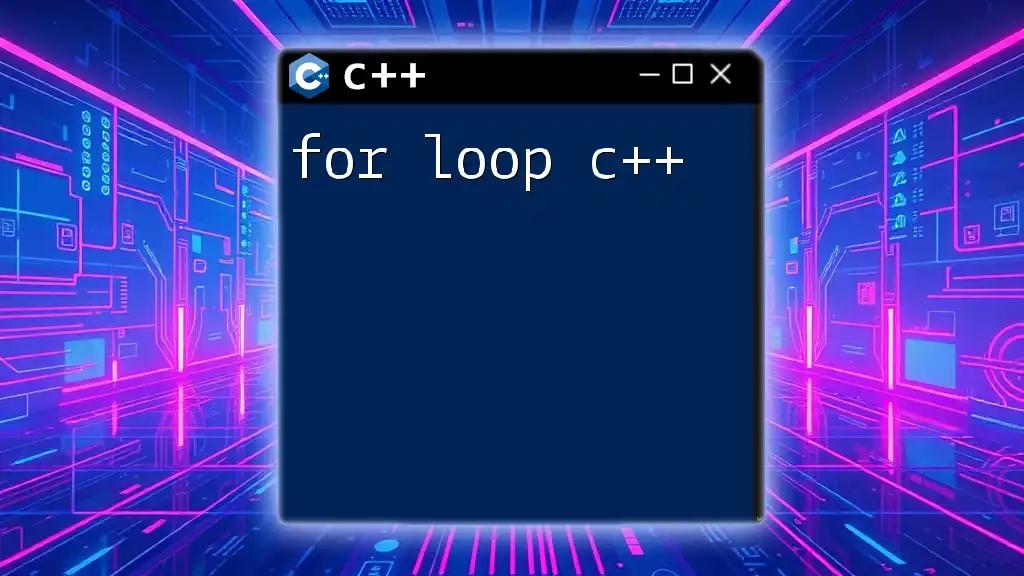
Advanced OOP Concepts in C++
Abstract Classes and Interfaces
Understanding Abstract Classes
An abstract class is a class that cannot be instantiated on its own and typically contains at least one pure virtual function. This is used as a base class for other classes and allows for defining a common interface.
Example of an abstract class:
class AbstractShape {
public:
virtual void draw() = 0; // Pure virtual function
};
class Circle : public AbstractShape {
public:
void draw() override {
cout << "Drawing Circle" << endl;
}
};
Creating Interfaces in C++
In C++, interfaces can be effectively represented by creating classes with only pure virtual functions, thus allowing multiple classes to implement the same set of methods.
Composition vs. Inheritance
When to Use Composition
While both composition and inheritance help in code reuse, there are scenarios where one is preferred over the other. Composition involves creating complex types by combining objects of other types. It is favored when you require "has-a" relationships rather than "is-a" relationships.
Example of composition:
class Engine {
public:
void start() {
cout << "Engine starting" << endl;
}
};
class Car {
private:
Engine engine; // Car "has-a" engine
public:
void startCar() {
engine.start();
}
};
Design Patterns in C++
Overview of Common Design Patterns
Design patterns are proven solutions to common software design problems. Some of the most widely used design patterns in C++ include:
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: A method for creating objects without specifying the exact class of object that will be created.
- Observer: A pattern where an object, known as the subject, maintains a list of dependents (observers) that need to be notified of state changes.
Code Snippets: Implementing a Design Pattern
For example, here’s a simplified implementation of the Singleton pattern:
class Singleton {
private:
static Singleton* instance;
// Private constructor to prevent instantiation
Singleton() {}
public:
static Singleton* getInstance() {
if (instance == nullptr) {
instance = new Singleton();
}
return instance;
}
};
// Initialize static variable
Singleton* Singleton::instance = nullptr;
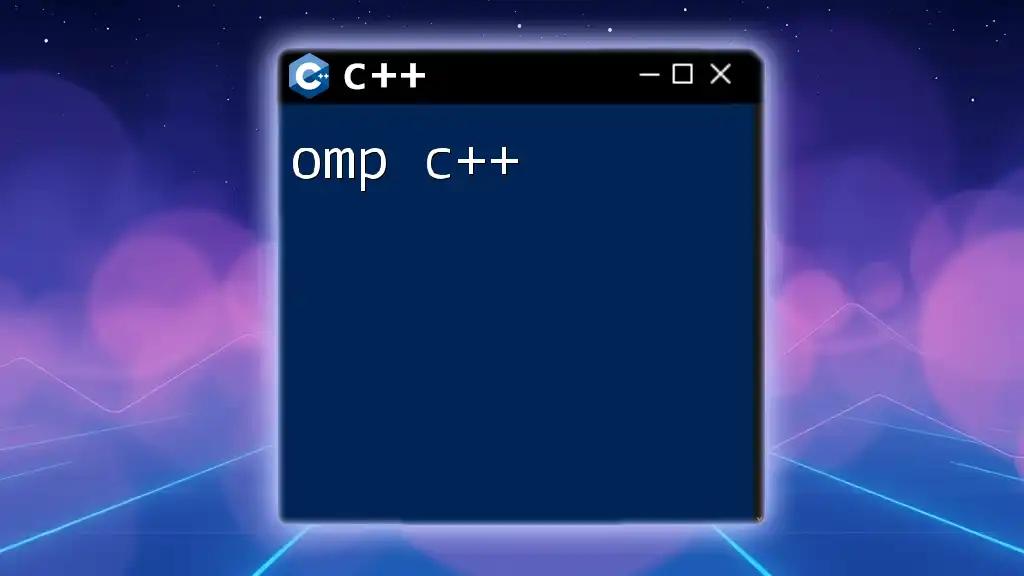
Tips and Best Practices for OOP in C++
Common Pitfalls to Avoid
- Overusing Inheritance: Stick to composition where appropriate to reduce the complexity of the code.
- Ignoring Access Specifiers: Always use access specifiers to enforce encapsulation effectively.
- Creating Large Classes: Smaller, single-responsibility classes are easier to manage and test.
Best Practices for Writing Clean OOP Code
- Use meaningful names for classes, methods, and variables for better readability.
- Document your code using comments and documentation tools to ensure clarity.
- Follow consistent naming conventions and formatting for maintaining code structure.
- Aim for high cohesion within classes and low coupling between objects to enhance reusability and maintainability.
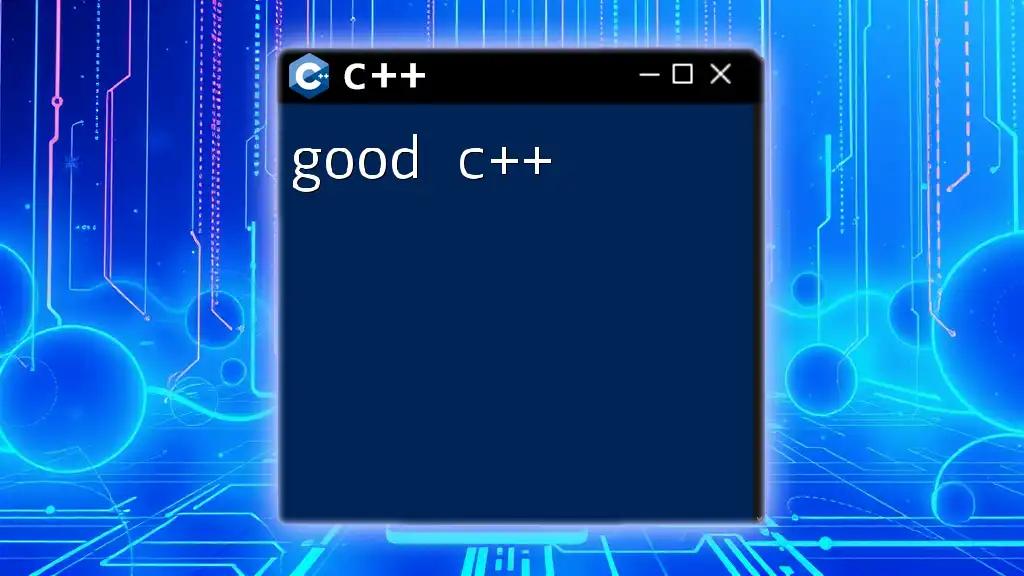
Conclusion
Recapping the importance of OOP in C++, it serves as a fundamental approach to organizing code and fostering better software design. Mastering these concepts will not only enhance the quality of your programming but also prepare you for advanced software development challenges.
Additional Resources
For further learning, consider exploring recommended books and tutorials that focus on OOP in C++. Participating in forums and communities will connect you with other developers, offering insights that enhance your programming journey in C++.