"Good C++" refers to writing code that is efficient, maintainable, and adheres to best practices in programming, such as proper memory management and clear syntax.
Here's a simple example of a well-structured C++ function that demonstrates good practices:
#include <iostream>
void printHello() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
printHello();
return 0;
}
Defining Good C++
To understand the essence of good C++, it’s essential to recognize that it encompasses more than just the syntax and structure of the language. Good C++ programming promotes not only efficient code execution but also maintainability and readability, which foster collaboration and future-proofing your projects. Code that's easy to read and understand becomes a priceless asset when you, or others, revisit it later.

Principles of Good C++ Programming
Code Readability
Readability is a cornerstone of good programming practice. Well-structured, comprehensible code makes it easier for programmers to debug and collaborate. Here are key aspects to consider:
-
Clear Naming Conventions: Use descriptive names for variables, functions, and classes. For instance, use `calculateArea` instead of a vague name like `ca`.
-
Documentation and Comments: Maintain inline comments to explain complex logic and add a detailed description at the beginning of each function. This aids anyone who reads your code, including your future self.
Maintainability
Maintainability refers to how easily a programmer can modify code in the future. This quality is vital as projects evolve over time. Here are strategies to achieve maintainability:
-
Refactoring: Regularly review and improve your code without changing its external behavior. This keeps the codebase healthy and easier to adapt with new changes.
-
Modular Programming: Break your code into smaller, reusable components. This reduces redundancy and makes it easy to update one part without affecting others.
Performance Optimization
While readability is significant, performance cannot be overlooked. Good C++ programmers often strike a balance between these two. Considerations include:
-
Understanding Time and Space Complexity: Assess whether your solution is efficient. For example, a linear time complexity \(O(n)\) is preferable over quadratic \(O(n^2)\) for large datasets.
-
When to Prioritize Performance: Identify performance bottlenecks and optimize these areas while keeping the rest of the code readable.
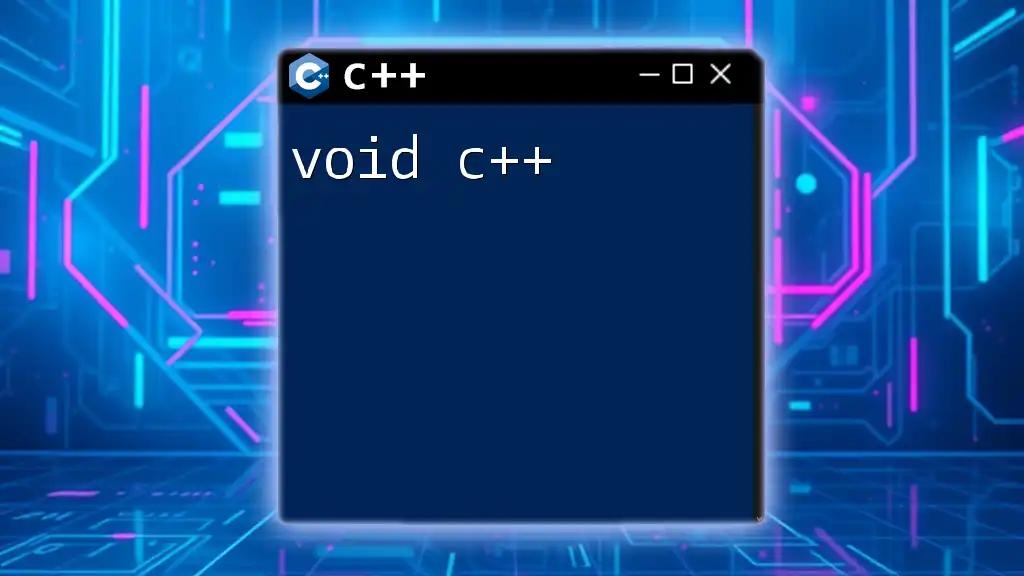
Best Practices for Writing Good C++
Consistent Style Guidelines
Adhering to a consistent style guide is crucial for maintaining a coherent codebase.
-
Adopting Established Style Guides: Guides like the Google C++ Style Guide provide standards for code formatting, function parameters, and even comment structures.
-
Code Formatting Tools: Leverage tools such as ClangFormat to automatically format your code. This saves time and eliminates potential disputes over style choices.
Smart Use of Data Structures
Choosing an appropriate data structure significantly impacts the efficiency of your program.
-
Comparison Among Data Structures: Carefully choose between vectors, lists, and maps based on the needs of your application. For example, vectors are great for dynamic arrays, while maps excel in key-value pair retrieval.
-
Performance Implications: Understand the performance trade-offs that come with each structure, and use them accordingly.
Effective Error Handling
Good C++ code incorporates effective error handling strategies to create robust applications.
-
Try-Catch Blocks: Using try-catch mechanisms ensures that exceptions are managed gracefully without crashing the application. For example:
try { int result = divide(x, y); } catch (std::exception& e) { std::cerr << "Error: " << e.what(); }
-
Assert Statements: Utilize `assert` statements during development to catch potential issues early. For instance:
assert(x > 0 && "x must be positive");
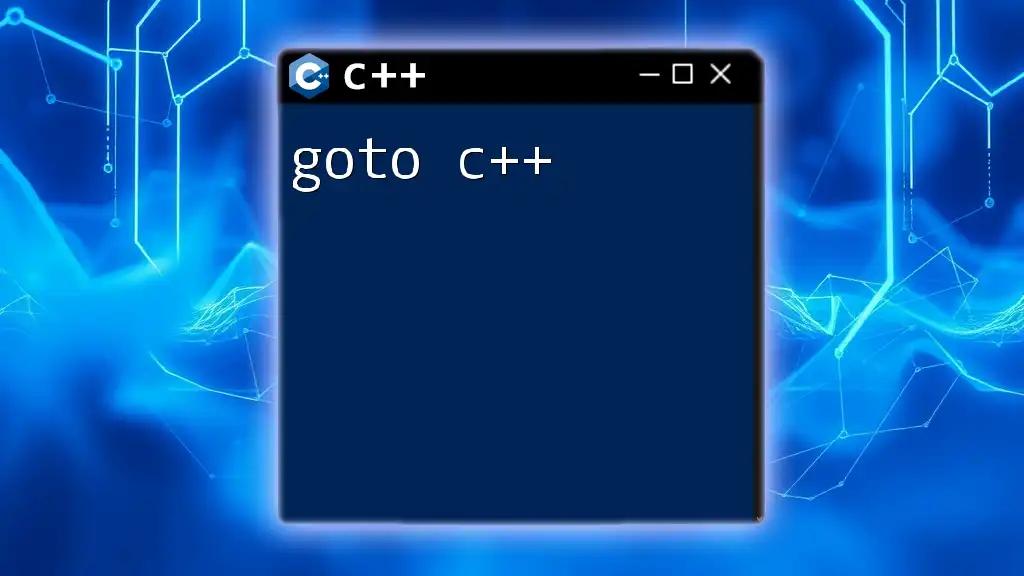
How to Write Specifically “Good” Functions
Function Design
Creating purpose-driven functions is paramount.
-
Single Responsibility Principle: Each function should serve a distinct purpose. This simplifies understanding and testing.
-
Structured Functions: For example, the design of a well-structured function should be clear:
int factorial(int n) { if (n <= 1) return 1; return n * factorial(n - 1); }
In contrast, functions that do too much lead to confusion and bugs.
Parameter Passing
A strong grasp of parameter passing is also vital for good C++:
- Passing by Value, Reference, and Pointer:
- Value: Creates a copy, using more memory—ideal for small, simple types.
- Reference: Efficient, allows modifications—use when passing large objects.
- Pointer: Grants flexibility with dynamic memory, but requires careful memory management to avoid leaks.
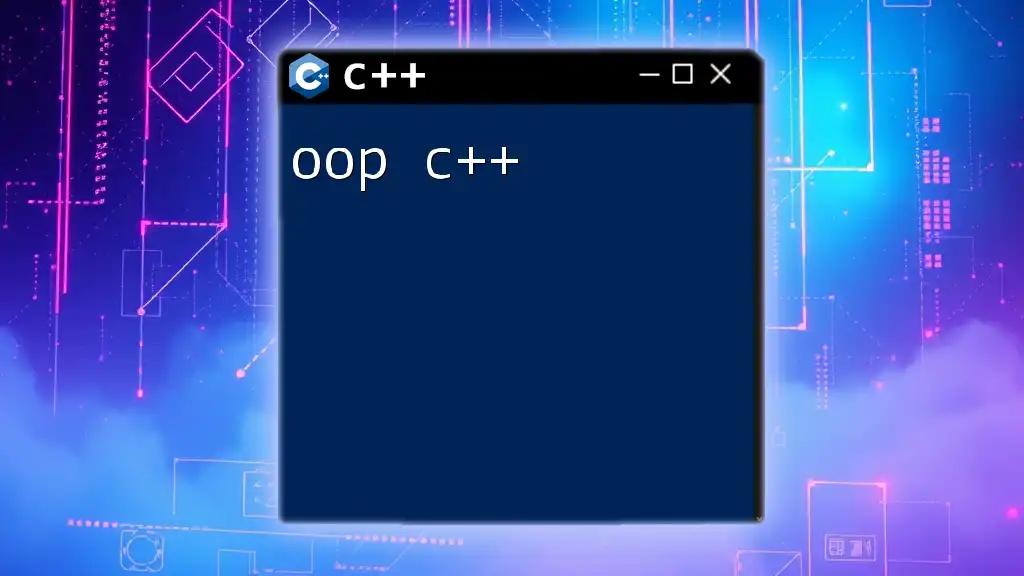
Leveraging C++ Features for Good Code
Utilizing Standard Template Library (STL)
The Standard Template Library (STL) is a treasure trove for good C++.
-
Advantages of STL: By using STL’s containers like `vector`, `list`, and `map`, alongside algorithms, you can simplify your code and improve efficiency.
-
Example of Using `std::vector`: When dealing with dynamic arrays:
#include <vector> std::vector<int> numbers = {1, 2, 3, 4, 5};
Smart Pointers
With C++, memory management becomes a lot easier by using smart pointers.
-
Unique_ptr and Shared_ptr: Understand how these can help manage memory and prevent leaks. For example:
#include <memory> std::unique_ptr<int> ptr(new int(10));
This encapsulates memory management, making it safer and more efficient.
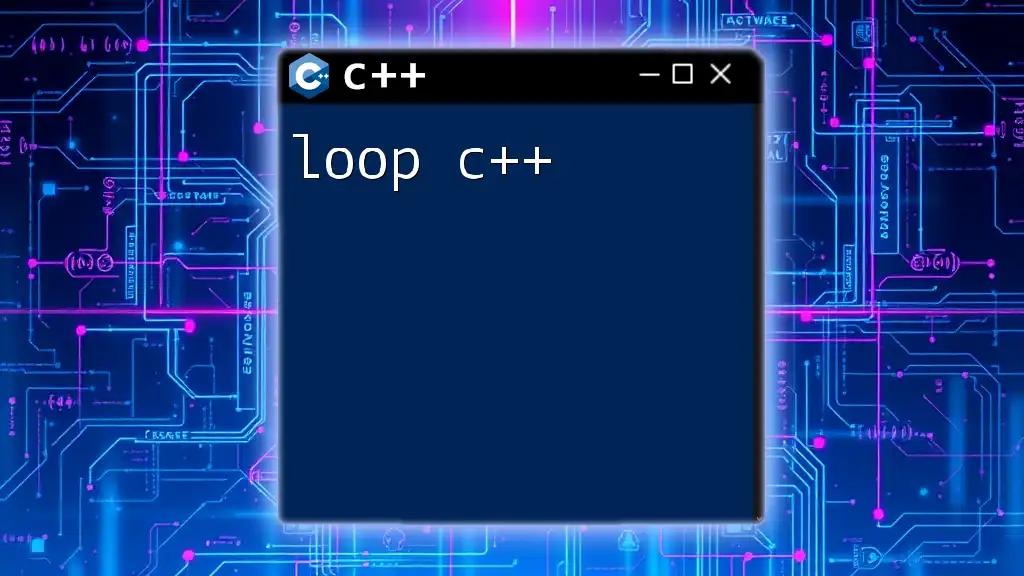
Common Pitfalls to Avoid in C++
Recognizing Bad Practices
Avoiding bad practices is crucial in maintaining high standards of C++ programming.
-
Global Variables: Global state can lead to code that is hard to understand and maintain. Strive for encapsulation and avoid unnecessary global access.
-
Overusing Macros: While macros can simplify code, they can also obscure logic and create hard-to-debug situations. Prefer inline functions or constants instead.
Debugging and Testing
Writing good tests is a hallmark of quality programming.
- Unit Testing and Test-Driven Development (TDD): Implement unit tests throughout development to ensure code works as intended. For example, you can use the Google Test framework to create test cases that verify the correctness of your functions.

Recap of Good C++ Practices
To encapsulate, "good C++" means writing code that is not only efficient but also readable and maintainable. By adhering to the principles outlined above, you position yourself for long-term success in programming endeavors. Continuous learning and community involvement are key components as you further your mastery of C++.
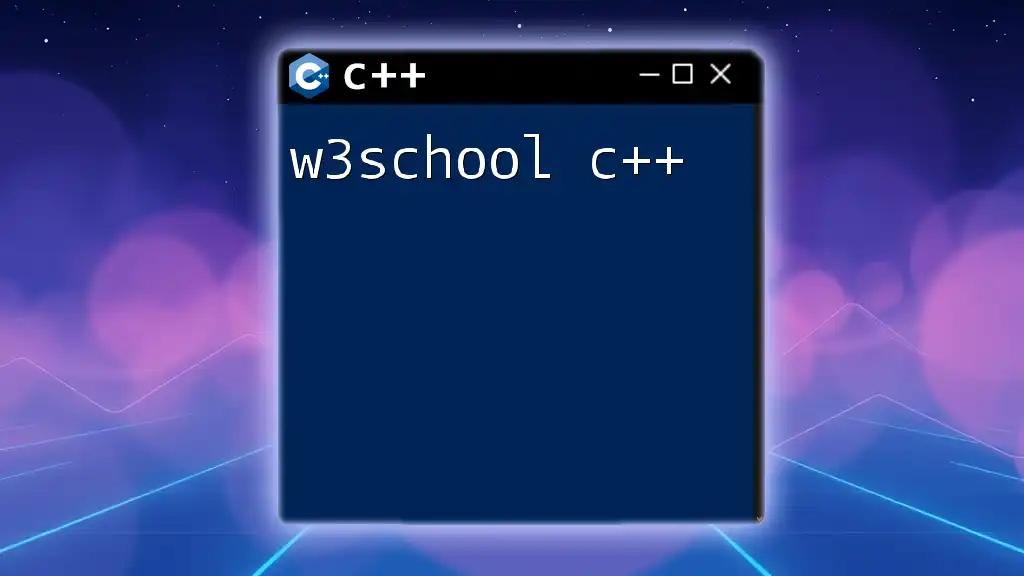
Where to Learn More
The journey to mastering good C++ continues beyond this article. Explore online courses, community forums, and literature that emphasize practical C++ programming to support your growth in the field.