Discover our top recommendations for good C++ books that can enhance your programming skills with concise examples, like this classic variable declaration:
int main() {
int number = 42; // Declaration of an integer variable
return 0;
}
Why Choose C++ Books?
Choosing good C++ books can significantly enhance your learning experience. Books offer distinct advantages that other resources may lack:
-
In-depth knowledge: Books are designed to delve into concepts thoroughly, providing rich background information and detailed explanations. This in contrast to other formats, which may skim over essential material.
-
Structured learning: Many C++ books follow a carefully crafted curriculum that leads readers through a logical progression of ideas, making the learning process smoother and more cohesive.
-
Reference material: Even after mastering a topic, a good book serves as a valuable long-term resource. You can always return to it for reference when tackling new projects or problems.
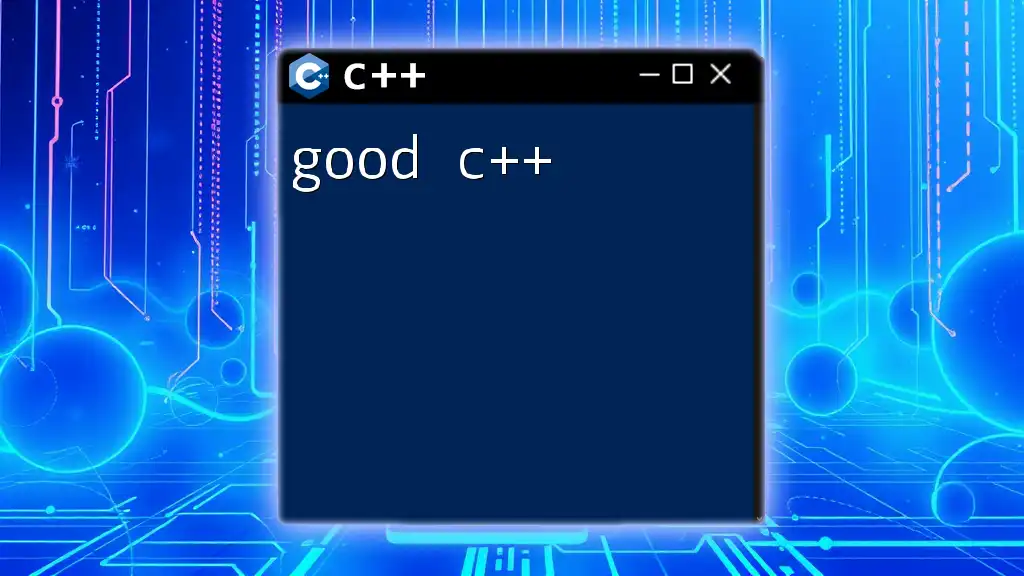
Types of C++ Books
Textbooks
Textbooks are perfect for those seeking foundational knowledge and theory, making them great resources for both beginners and experienced programmers refreshing their knowledge.
Examples:
- "C++ Primer": Renowned for its comprehensive coverage from the basics to advanced concepts.
- "Effective C++": Offers critical insights and best practices for writing high-quality C++ code.
Practical Guides
Practical guides focus on hands-on coding and real-world applications. These texts can help bridge the gap between theoretical knowledge and practical skills.
Example:
- "The C++ Programming Language" by Bjarne Stroustrup: This book is an authoritative text that introduces core concepts and advanced features of the language.
Advanced Reference Books
These books cater to experienced programmers looking to refine their skills or explore advanced topics.
Example:
- "C++ Concurrency in Action": A comprehensive guide on using C++ for multi-threading and concurrent programming, crucial for modern software development.
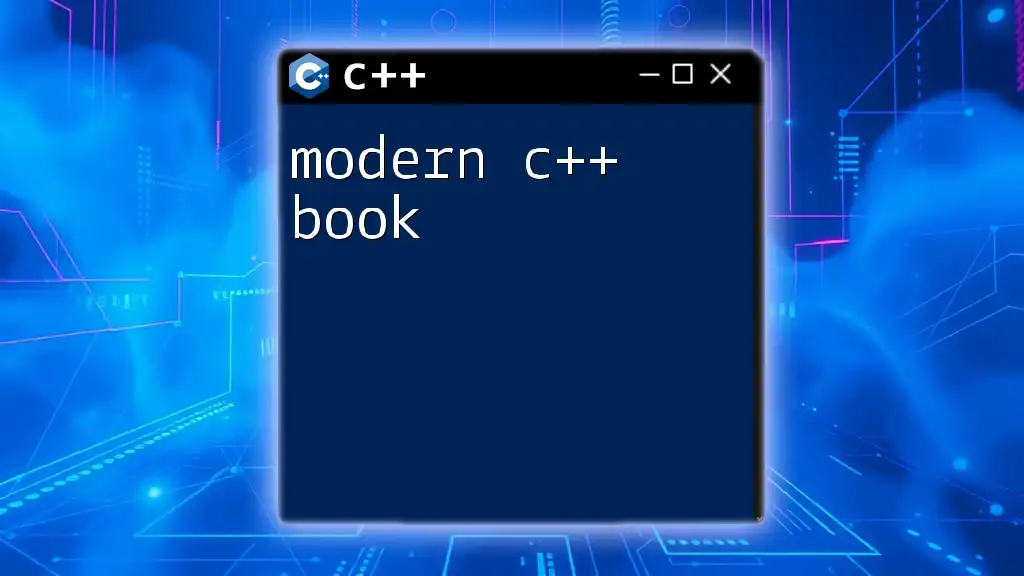
Top Recommendations for C++ Books
C++ Primer
"C++ Primer" is a highly recommended book for both beginners and those looking to solidify their knowledge.
-
Overview: The book covers a wide array of topics, from basic syntax to more complex programming paradigms.
-
Key features: It presents concepts with practical examples and exercises, ensuring a hands-on approach.
-
Code Snippet Example: A simple "Hello, World!" program:
#include <iostream> using namespace std; int main() { cout << "Hello, World!" << endl; return 0; }
Effective C++
"Effective C++" provides 55 specific ways to improve your programs and designs.
-
Overview: This book emphasizes best practices and idiomatic C++ programming.
-
Key features: Readers gain insights into common pitfalls and how to avoid them, significantly improving code quality.
-
Sample Content Discussion: One critical lesson is the "Rule of Three", advising that if a class needs a custom destructor, copy constructor, or copy assignment operator, it likely needs all three.
The C++ Programming Language
Authored by the creator of C++, Bjarne Stroustrup, this book is essential for understanding both foundational and advanced features.
-
Overview: Offering a professional perspective on language design and usage, it's suitable for anyone serious about mastering C++.
-
Key features: Comprehensive chapters on topics such as object-oriented programming, templates, and standard libraries.
-
Example of an advanced concept: Templates allow programmers to create functions and classes that operate with any data type. This promotes code reusability and type safety.
C++ Concurrency in Action
"C++ Concurrency in Action" is essential in today's multi-threaded application development landscape.
-
Overview: Covers C++11 features tailored for multi-threading.
-
Key features: The book not only explains core concepts but also presents real-world examples and best practices.
-
Example Code Snippet: A demonstration of creating a thread:
#include <iostream> #include <thread> void printHello() { std::cout << "Hello from thread!" << std::endl; } int main() { std::thread t(printHello); t.join(); // Wait for the thread to finish return 0; }
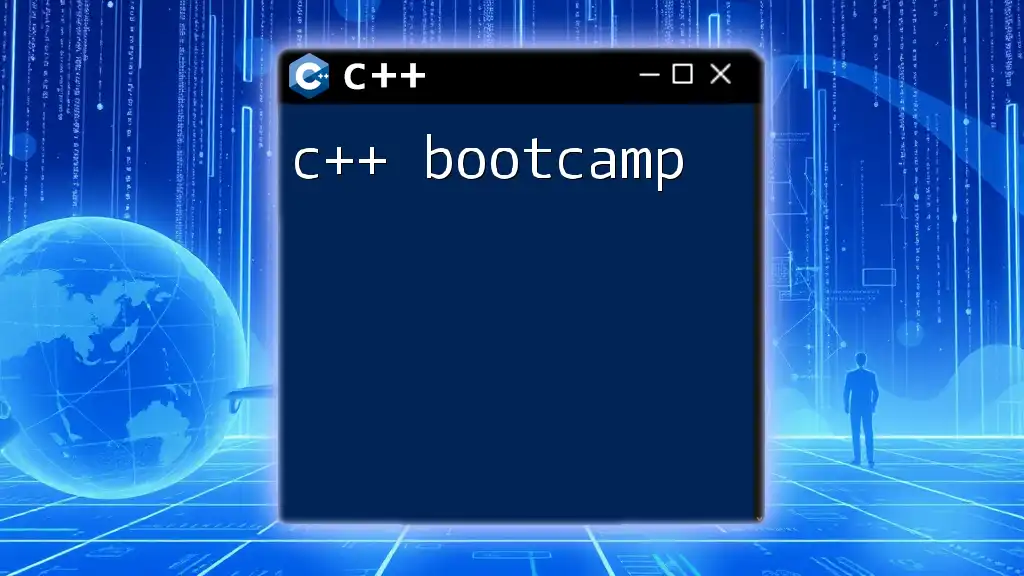
Books About C++ Programming for Beginners
Head First C++
"Head First C++" is an engaging book aimed at individuals new to programming.
-
Overview: Blending humor with pedagogy, it strives to make learning programming concepts enjoyable.
-
Approach to teaching concepts: The book relies heavily on visual learning, using illustrations and examples to reinforce complex topics.
Programming: Principles and Practice Using C++
This book serves as an introductory text for absolute beginners.
-
Overview: Covers fundamental programming concepts through C++ and promotes logical thinking and problem-solving skills.
-
Learning Methodology: Rich in hands-on projects, it encourages readers to actively apply what they learn, reinforcing knowledge through practice.
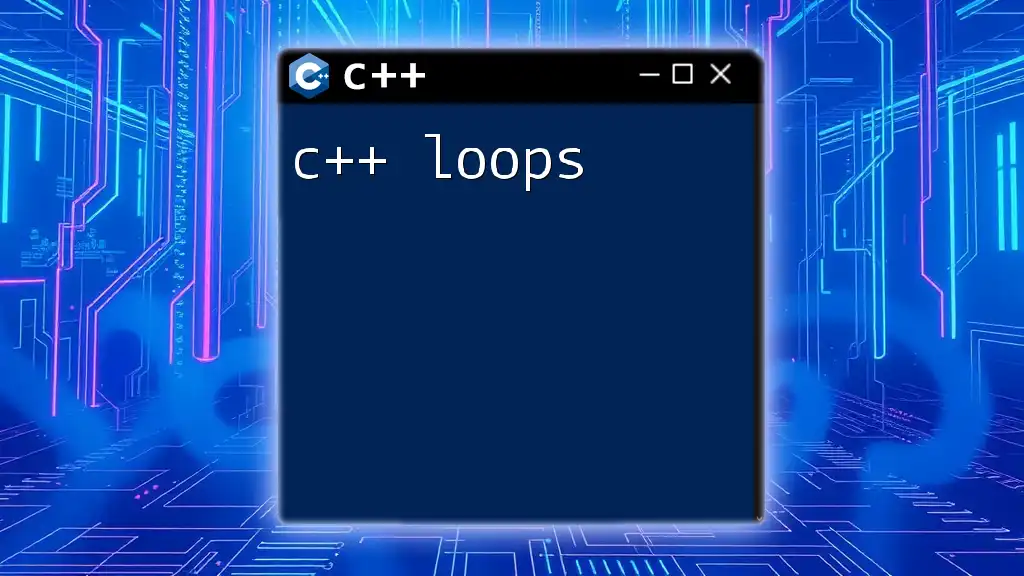
Best C++ Books for Intermediate to Advanced Programmers
C++17 in a Nutshell
"C++17 in a Nutshell" is excellent for seasoned programmers wanting to stay current.
-
Overview: This book discusses the latest features and updates of the language introduced in C++17.
-
Provides Comprehensive Reference: Each chapter covers the implications of changes and the best strategies for implementing them in your codebase.
Design Patterns in C++
This book dives into common design patterns applicable when programming in C++.
-
Overview: Each pattern's context, applicability, and solutions are discussed thoroughly.
-
Importance: Familiarity with design patterns helps in structuring code efficiently and promotes robust software design.
-
Example of a pattern: The Singleton Pattern: This pattern ensures that a class has only one instance and provides a global point of access to it.
class Singleton {
public:
static Singleton& getInstance() {
static Singleton instance; // Guaranteed to be destroyed
return instance; // Instantiated on first use
}
private:
Singleton() {} // Constructor is private
Singleton(Singleton const&) = delete; // Prevents copying
void operator=(Singleton const&) = delete; // Prevents assignment
};
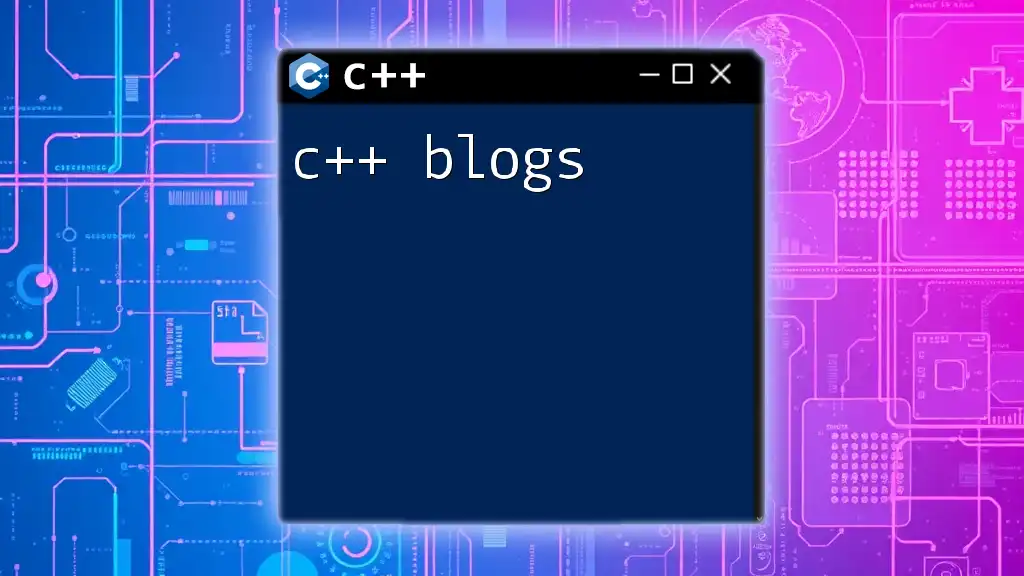
How to Choose the Right C++ Book for You
When selecting the good C++ books that best suit your learning journey, consider the following factors:
Consider Your Current Skill Level
Identify whether you're a beginner, intermediate, or advanced programmer. This will guide you in selecting books that match your expertise.
Define Your Learning Goals
Determine what you aim to achieve with your programming skills, whether it's software development, game programming, or systems programming. Tailor your book selection accordingly.
Look for Practical Exercises
Hands-on learning is crucial in programming. Choose books that offer practical exercises to apply concepts effectively. Being able to test your knowledge with real code will deepen your understanding of C++.
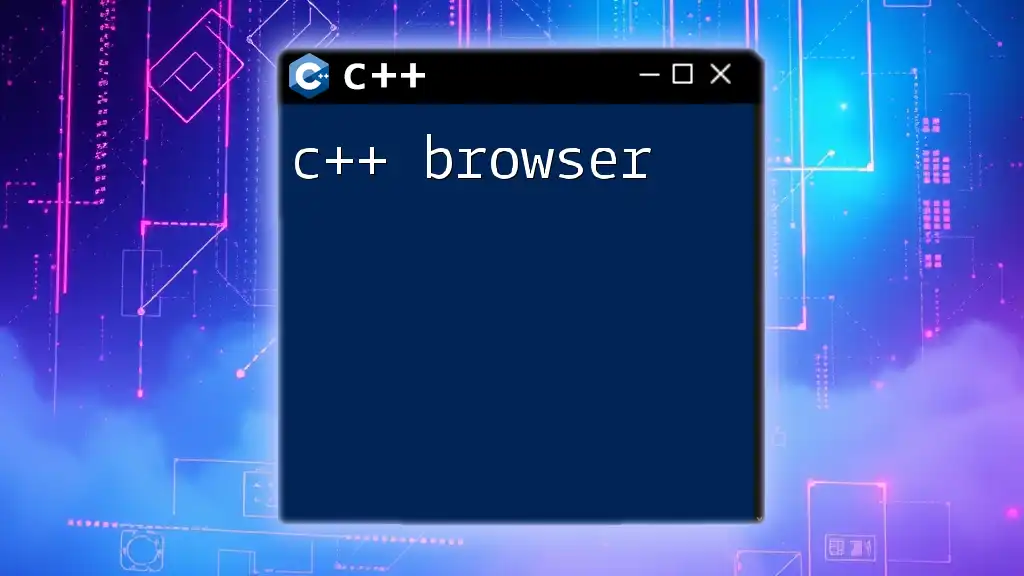
Conclusion
In conclusion, the right choice of good C++ books can shape your programming journey immensely. Not only do they provide foundational knowledge and coding techniques, but they also act as long-term references. Delve into the recommended titles, explore their content, and leverage them to enhance your skills. As you begin your learning path, consider sharing your thoughts and your favorite C++ books with others!
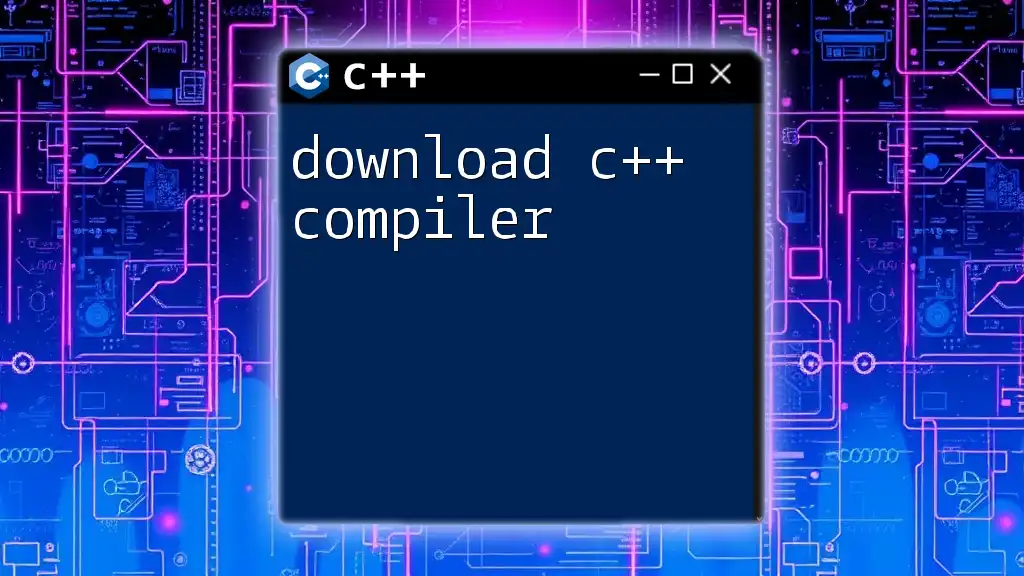
Additional Resources
To bolster your learning experience further, consider exploring online forums, tutorials, and programming communities. Engaging with others can provide additional perspectives and resources that complement your C++ literature.
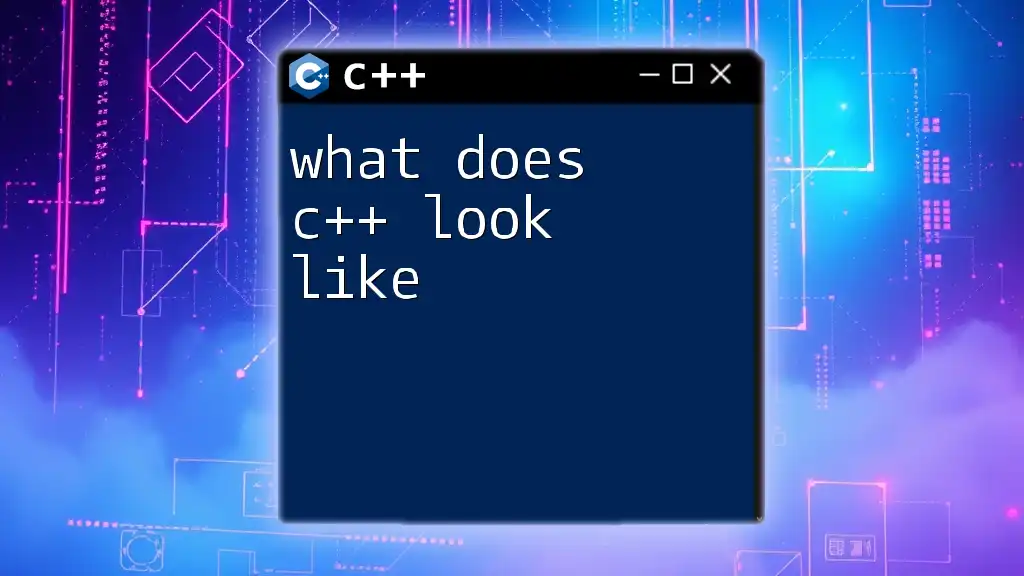
Frequently Asked Questions
What is C++ primarily used for?
C++ is a versatile programming language widely used for system/software development, game development, and applications requiring high-performance computations.
How can I benefit from reading several C++ books?
Reading various books allows you to gather different viewpoints, techniques, and coding styles, enriching your understanding of the language.
Are there any free resources comparable to these recommended books?
Yes, many websites, online courses, and programming blogs provide excellent free resources that complement the textbooks and can enhance your C++ knowledge.