C++ loops are control structures that allow you to execute a block of code repeatedly based on a specified condition or for a defined number of iterations.
Here's an example of a `for` loop in C++:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 5; i++) {
cout << "Iteration: " << i << endl;
}
return 0;
}
Understanding C++ Loops
What Are C++ Loops?
C++ loops are control structures that allow you to execute a block of code repeatedly based on a specified condition. They are essential for automating repetitive tasks and help in managing the flow of execution within a program. By mastering loops in C++, you can write more efficient and effective code, reducing redundancy and improving readability.
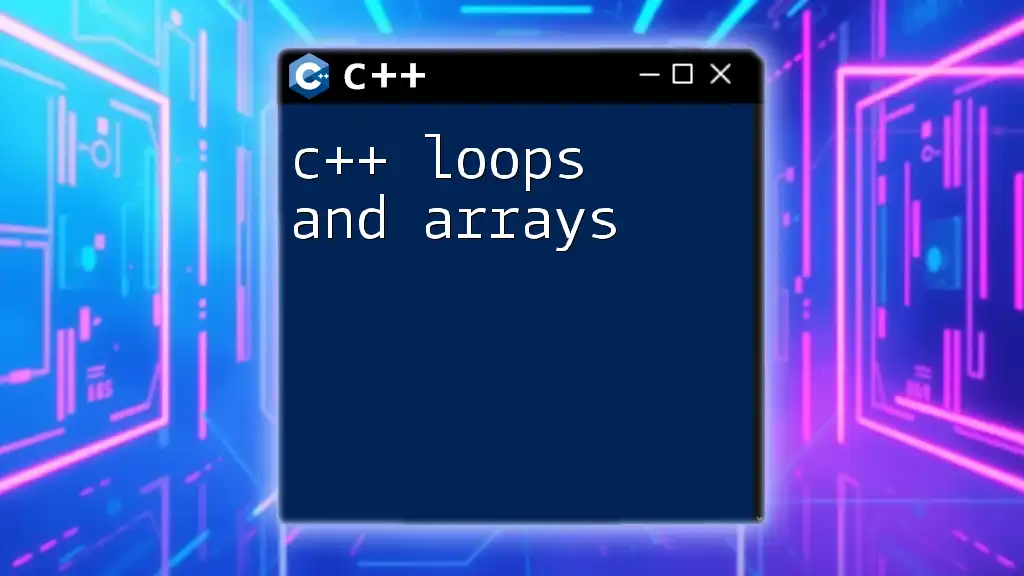
Types of Loops in C++
Introduction to Loop Types
C++ provides several types of loops, each designed to handle different scenarios:
- For Loop: Best when the number of iterations is known beforehand.
- While Loop: Suitable for situations where the number of iterations is not predetermined but depends on a condition.
- Do-While Loop: Similar to the while loop but guarantees at least one execution of the loop body.
The For Loop
Structure and Syntax
The for loop is primarily used for counting and iterating over a range. Its basic syntax consists of three components: initialization, condition, and iteration.
Example of a For Loop
for(int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
In this example, the loop initializes `i` to zero. It continues to execute as long as `i` is less than 5, and after each iteration, `i` is incremented by one. This structure makes it clear and concise for tasks that require counting.
The While Loop
Structure and Syntax
The while loop works based on a condition and continues executing as long as that condition remains true. Unlike the for loop, the initialization and iteration happen outside the loop statement.
Example of a While Loop
int i = 0;
while(i < 5) {
std::cout << "Iteration: " << i << std::endl;
i++;
}
Here, we manually initiate `i` and increase its value within the loop. The loop will execute until `i` reaches 5. This is particularly useful when the number of iterations is not known in advance.
The Do-While Loop
Structure and Syntax
A do-while loop is similar to a while loop, but it guarantees that the loop body will execute at least once, regardless of the condition. The condition is checked after the loop body is executed.
Example of a Do-While Loop
int i = 0;
do {
std::cout << "Iteration: " << i << std::endl;
i++;
} while(i < 5);
In this scenario, the block executes first, displaying the current value of `i`, and checks the condition afterwards. This can be particularly useful in situations where you need at least one execution.
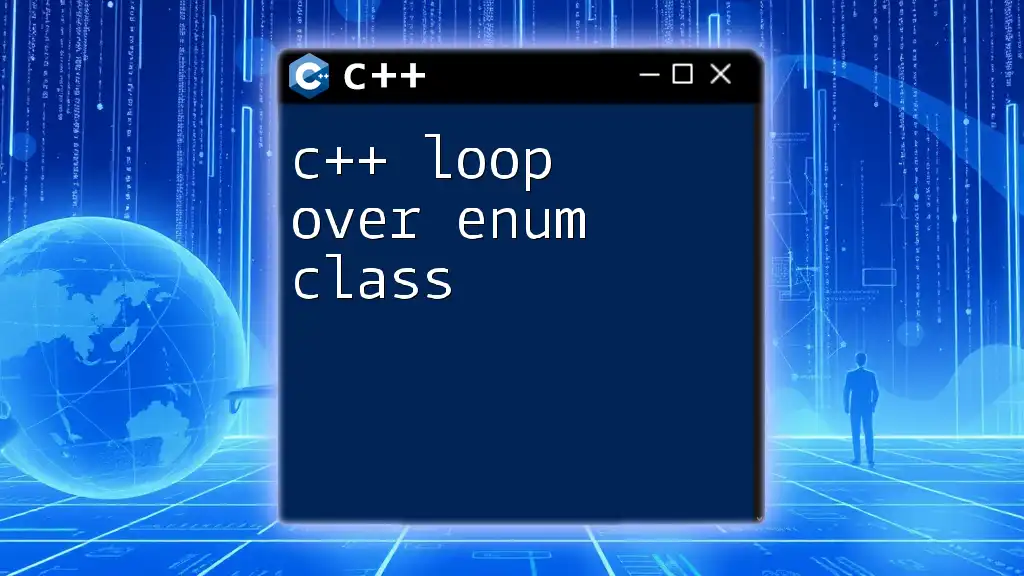
Choosing the Right Loop
When to Use Which Loop
Selecting the appropriate loop type is crucial for writing efficient code. Use a for loop when you know the exact number of iterations ahead of time. Opt for a while loop if you need to repeat an action until a certain condition is met. A do-while loop is perfect in cases where you want to ensure the code block executes at least once, regardless of the condition.
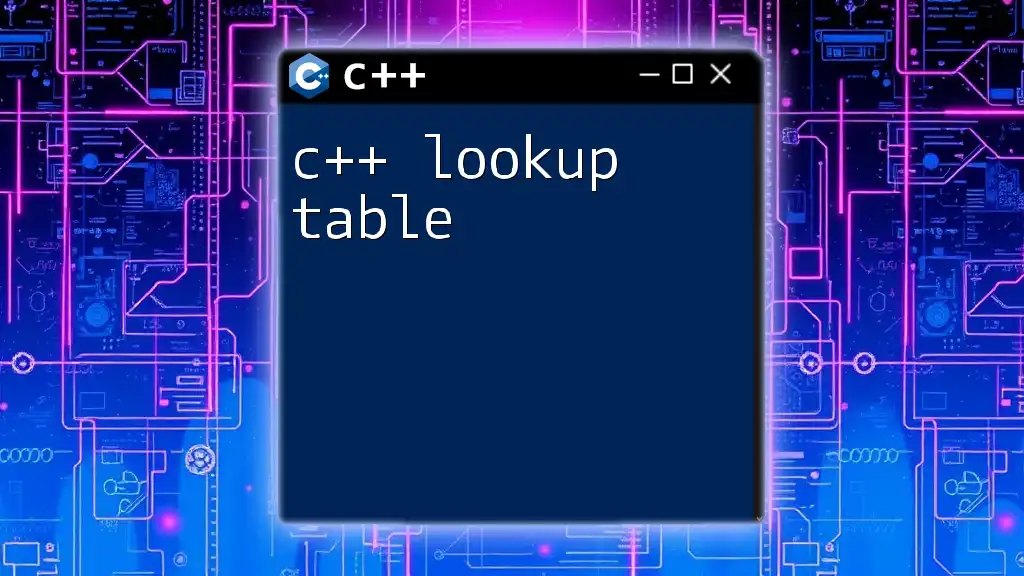
Nested Loops in C++
What Are Nested Loops?
Nested loops are loops within loops. They are particularly useful for multi-dimensional data structures like arrays or when you need to perform repeated actions for each iteration of another loop. The complexity of nested loops grows with each additional layer, so they should be used judiciously.
Example of Nested Loops
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 3; j++) {
std::cout << "(" << i << ", " << j << ")" << std::endl;
}
}
In this example, for each iteration of the outer loop (controlled by `i`), the inner loop runs completely. This results in outputting pairs of coordinates, demonstrating how nested loops can manage complex iteration scenarios effectively.
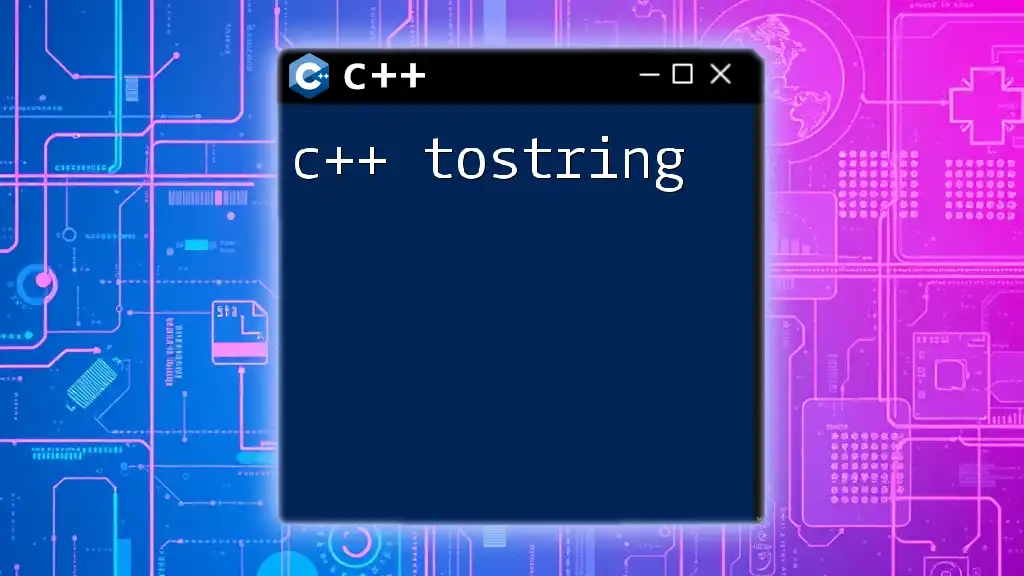
Loop Control Statements
Breaking Out of Loops
The Break Statement
The `break` statement allows you to exit a loop prematurely when a specific condition is met. This can help control the flow of your program more effectively.
Example of a Break Statement
for(int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
std::cout << "Iteration: " << i << std::endl;
}
In this example, as soon as `i` reaches 5, the loop halts, and no further iterations occur. Understanding how to use `break` can enhance the versatility of your loops.
Skipping Iterations
The Continue Statement
The `continue` statement skips the current iteration and proceeds to the next iteration of the loop. This can be helpful in scenarios where certain conditions do not require further processing.
Example with Continue
for(int i = 0; i < 10; i++) {
if (i % 2 == 0) {
continue;
}
std::cout << "Odd number: " << i << std::endl;
}
In this case, even numbers are skipped, and only odd numbers are printed. This shows how you can selectively control which iterations to execute.
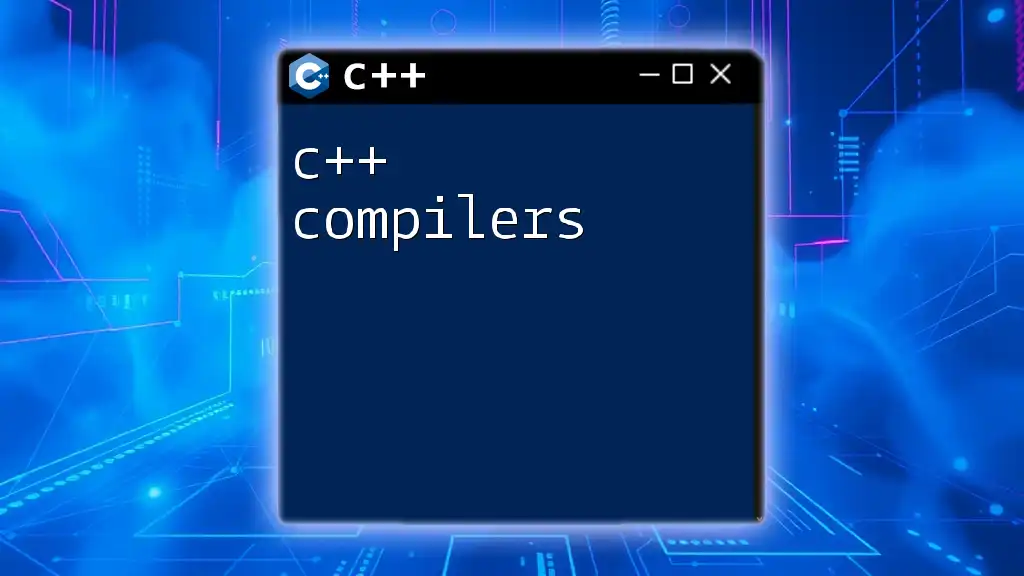
Best Practices for Using Loops in C++
Write Clear and Maintainable Code
Clarity in code is paramount. Use meaningful variable names, and structure your loops to be easily understood. Avoid overly complicated conditions that can confuse other developers (or your future self) when revisiting the code.
Efficiency Matters
When writing loops, consider the efficiency of your code. Nested loops, in particular, can lead to increased time complexity. Aim to minimize the number of iterations and optimize the conditions to ensure the program runs efficiently.
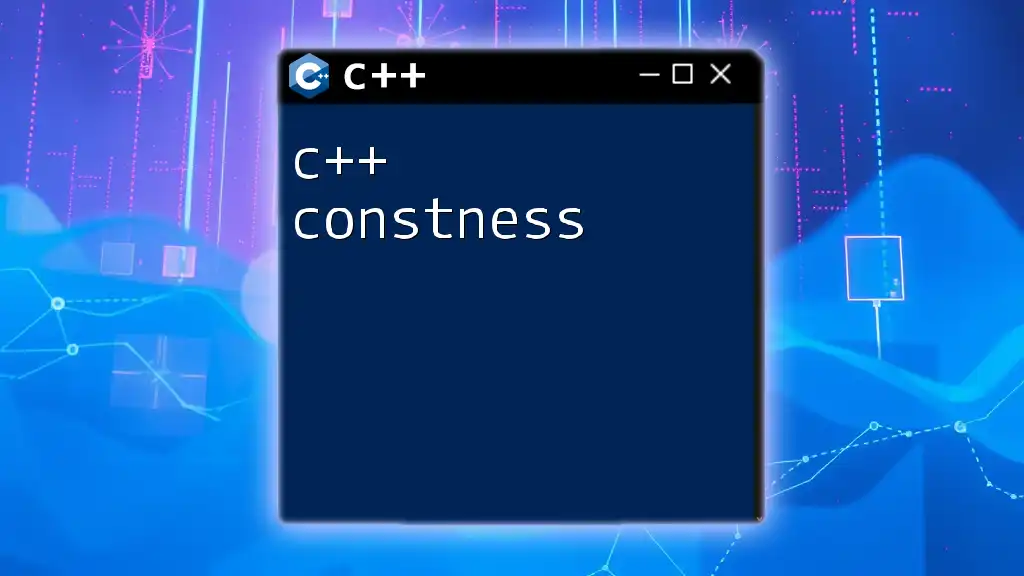
Common Mistakes to Avoid
Infinite Loops
Creating infinite loops is a common pitfall, often stemming from neglecting to update the loop variable or incorrect condition checking. To prevent this, always ensure that the condition will eventually evaluate to false.
Misusing Loop Control Statements
Misusing `break` and `continue` can lead to unexpected behavior. It is essential to be clear on when to break out of a loop or skip an iteration—to maintain control over your program flow and ensure logic is preserved.
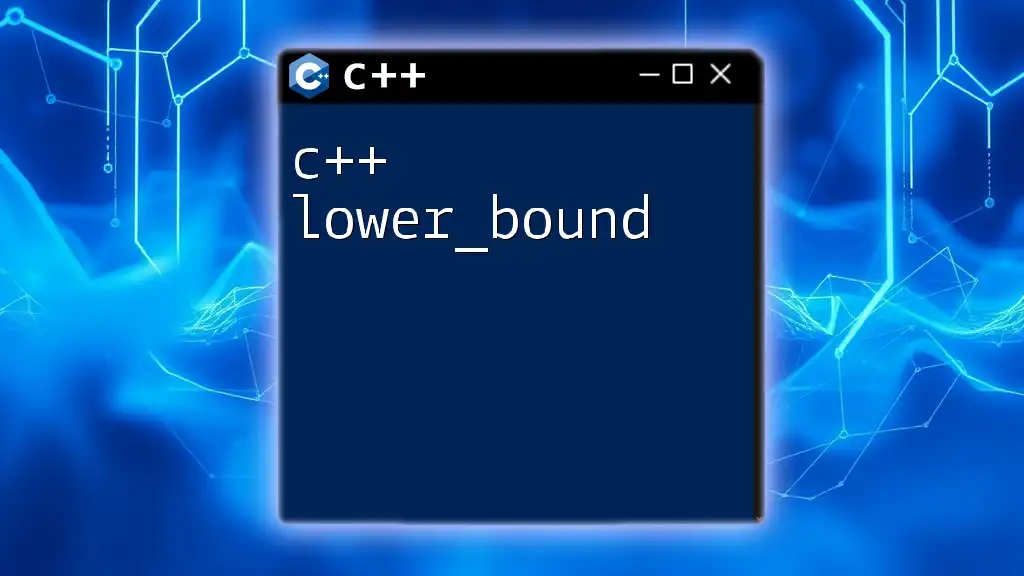
Conclusion
In summary, C++ loops are powerful structures that enhance code efficiency and reduce redundancy. By mastering the different types of loops—like for loops, while loops, and do-while loops—you can execute repetitive tasks effectively. Additionally, with a firm understanding of control statements like `break` and `continue`, you can manage loop executions with precision. As you continue to practice and apply these concepts, your proficiency in C++ will grow, enabling you to tackle more complex programming challenges with ease.