In C++, you can iterate over an `enum class` using a combination of a helper function and a loop, as standard `enum class` does not provide direct iteration capabilities. Here's a simple example:
#include <iostream>
enum class Color { Red, Green, Blue, Count };
template<typename T>
void loopEnum() {
for (int i = 0; i < static_cast<int>(T::Count); ++i) {
T color = static_cast<T>(i);
std::cout << static_cast<int>(color) << std::endl;
}
}
int main() {
loopEnum<Color>();
return 0;
}
Understanding Enum Classes in C++
What is an Enum Class?
An enum class in C++ is a scoped enumeration, which means that the enumerators (the named constants) are contained within the scope of the class name, preventing name collisions. This is different from traditional `enum`, where enumerators are placed in the surrounding scope. Using an enum class provides stronger type safety and better organization of related constants.
Benefits of using enum classes include:
- Type safety: Since enumerators are scoped, you can't accidentally use an enumerator of one enum class as if it were an enumerator of another.
- Clearer code: It’s clear which enum class each enumerator belongs to, improving readability and maintainability.
Defining an Enum Class
Defining an enum class is straightforward. The syntax involves the keyword `enum class`, followed by the type name and then the enumerators, enclosed in braces.
Here’s an example of a simple enum class defining colors:
enum class Color { Red, Green, Blue };
This defines an enum class named `Color` with three enumerators: `Red`, `Green`, and `Blue`.

The Need to Loop Over Enum Classes
Why Loop over Enum Classes?
Iterating over enum classes allows developers to manage and utilize enumerated values efficiently. This is especially beneficial in scenarios where enumerators represent distinct states or options, such as configuration settings, game states, or decisions in algorithms.
Real-world use cases include:
- Managing application settings (like user preferences).
- Controlling game states (for example, menu, playing, paused).
- Processing commands in a state machine.
Benefits of iteration include:
- Manageability: You can process all defined values systematically.
- Readability: Code becomes easier to follow, as it clearly outlines the logic associated with possible states or options.
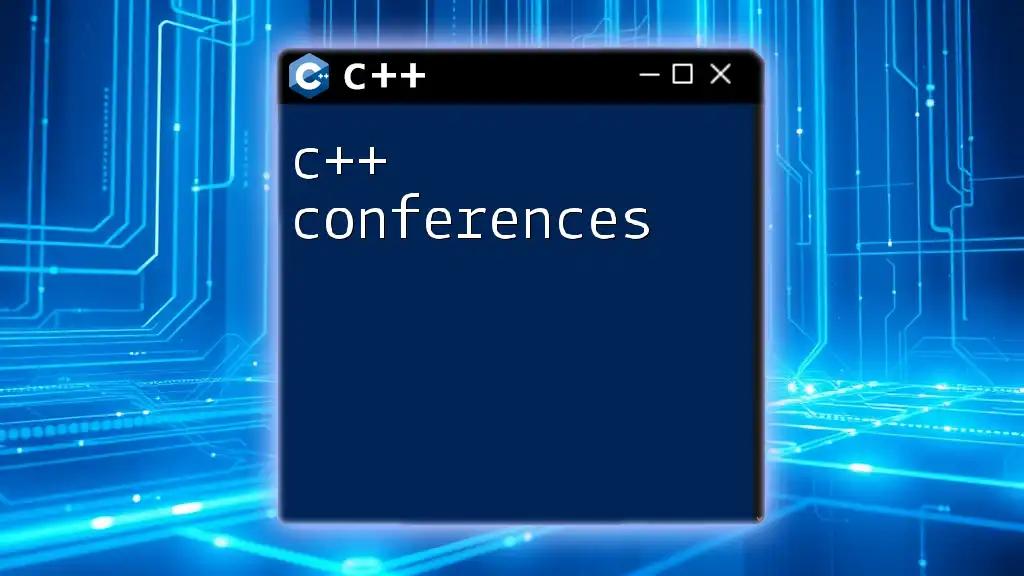
Iterating Over Enum Classes in C++
The Challenge of Iterating Over Enum Classes
Unlike ordinary arrays or containers, looping directly over enum class values in C++ is not possible using traditional for-loops due to their scoped nature and the absence of direct access to enumerator ranges. The enumerator values could be represented as integers, but direct iteration using a for loop needs an alternative approach to access all enumerators.
Method 1: Using a Custom Function
Create a Helper Function
One efficient way to iterate over enum classes is to create a helper function that returns all the enum values in a suitable container, such as a vector.
Here’s a practical example:
#include <iostream>
#include <vector>
enum class Color { Red, Green, Blue };
const std::vector<Color> colors = { Color::Red, Color::Green, Color::Blue };
void printColors() {
for (const auto& color : colors) {
std::cout << static_cast<int>(color) << std::endl; // Outputs the underlying integer
}
}
In the example above, we define a vector `colors` that contains all the enumerator values from the `Color` enum class. The `printColors` function iterates over each element in the vector, converting the `Color` type to its underlying integer representation for output.
Calling the Helper Function
You can easily use the `printColors` function within your main application, calling it whenever you need to display or process color values.
Method 2: Using a Range-Based For Loop
Explanation of Range-Based For Loop
C++11 introduced range-based for loops, which simplify iterating over collections. This approach is both intuitive and reduces the likelihood of errors related to index handling.
Implementing Range-Based For Loop
To iterate through the `colors` vector, you can use a range-based for loop effectively. Here’s how it looks in practice:
#include <iostream>
#include <vector>
enum class Color { Red, Green, Blue };
std::vector<Color> getColorValues() {
return { Color::Red, Color::Green, Color::Blue };
}
void iterateColors() {
for (const auto& color : getColorValues()) {
std::cout << static_cast<int>(color) << std::endl; // Outputs the underlying integer
}
}
In the above code, `iterateColors` uses a range-based loop to iterate over the values returned by `getColorValues`, displaying each one in its integer form.
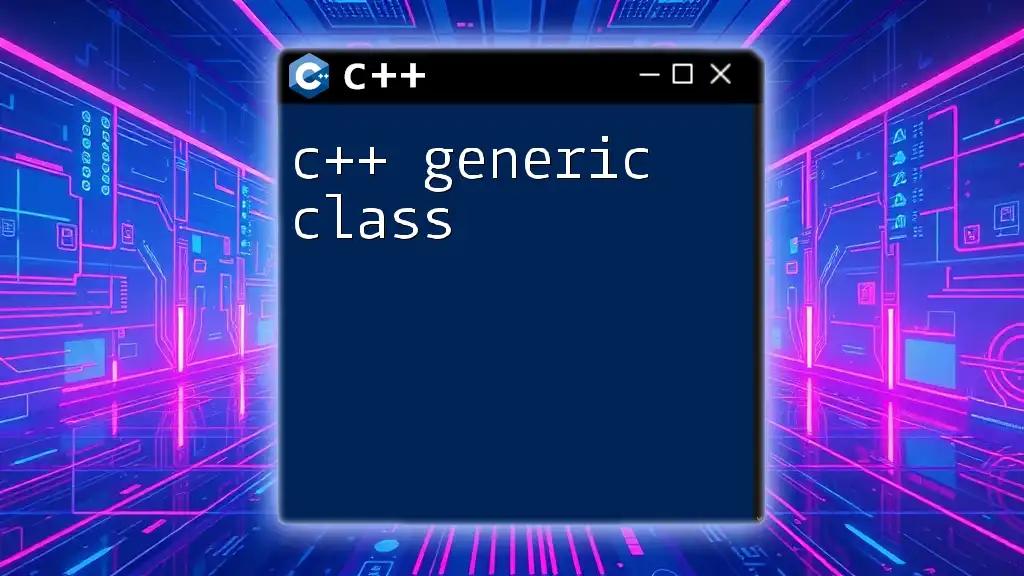
Method 3: Using Standard Library Functions
Using std::array for Iteration
The C++ Standard Library provides utility types that facilitate working with fixed-size collections, such as `std::array`. This allows for cleaner declaration and utilization of enum class values.
Here’s how you can iterate using `std::array`:
#include <array>
#include <iostream>
enum class Color { Red, Green, Blue };
std::array<Color, 3> colorArray = { Color::Red, Color::Green, Color::Blue };
void iterateWithArray() {
for (const auto& color : colorArray) {
std::cout << static_cast<int>(color) << std::endl; // Outputs the underlying integer
}
}
In this example, `colorArray` is a fixed-sized array containing all the colors. The `iterateWithArray` function demonstrates how easy and efficient it is to loop through the values.

Practical Use Cases
Implementing Loop Over Enum Class for a Game
Let’s consider a scenario in game development where you manage game states. An enum class can represent these states effectively:
enum class GameState { Menu, Playing, Paused };
std::vector<GameState> gameStates = { GameState::Menu, GameState::Playing, GameState::Paused };
void iterateGameState() {
for (const auto& state : gameStates) {
std::cout << static_cast<int>(state) << std::endl; // Outputs the integer representing the state
}
}
In this example, the `iterateGameState` function displays the numeric representation of each game state, making it clear which state is currently active or being processed.
Leveraging Enum Classes in Configuration Settings
In configuration management, an enum class can encapsulate various settings options efficiently.
For instance, consider this example of defining user settings:
enum class UserSetting { Audio, Video, Controls };
void showSettings() {
std::array<UserSetting, 3> settings = { UserSetting::Audio, UserSetting::Video, UserSetting::Controls };
for (const auto& setting : settings) {
std::cout << static_cast<int>(setting) << std::endl; // Outputs each setting's integer representation
}
}
This approach allows you to maintain a structured representation of different user settings while enabling easy iteration.

Conclusion
Iterating over enum classes in C++ may pose challenges initially, but with the right methods—such as using helper functions, range-based loops, or standard library containers—developers can effectively manage and utilize enum values. Understanding these techniques is crucial in applying enum classes in various programming scenarios, improving both code quality and efficiency.

Call to Action
Try implementing these techniques in your programming projects! Whether you’re managing a game, setting options, or just experimenting with C++, practicing with enum classes and their iterations will enhance your coding skills. Feel free to share your experiences or ask questions in the comments section!