An `enum class` in C++ is a strongly typed enumeration that helps encapsulate its enumerators in a namespace, preventing name conflicts and enhancing type safety.
enum class Color { Red, Green, Blue };
Color myColor = Color::Red;
What is an Enum in C++?
An enum (short for enumeration) in C++ is a user-defined data type consisting of a set of named integral constants. It allows you to define a variable that can hold a limited set of values and enhances the readability and maintainability of your code. Traditional enums expose their enumerators globally and can lead to naming conflicts.
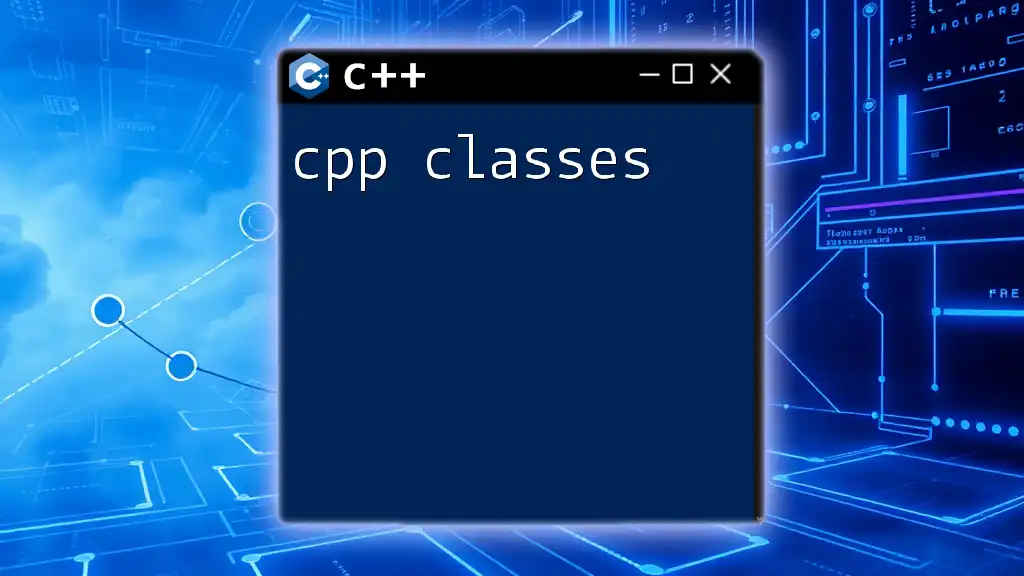
Why Use Enum Class in C++?
The cpp enum class was introduced in C++11 to address the limitations of traditional enums. The primary benefits of using an enum class include:
-
Type Safety: Enum classes do not implicitly convert to integers, which prevents unintended usage and improves code safety.
-
Scoped Enumeration: Unlike traditional enums, the enumerators declared in an enum class are scoped within the enumeration type. This means that you cannot access the enumerators without prefixing them with the enum class name, thereby avoiding naming conflicts.
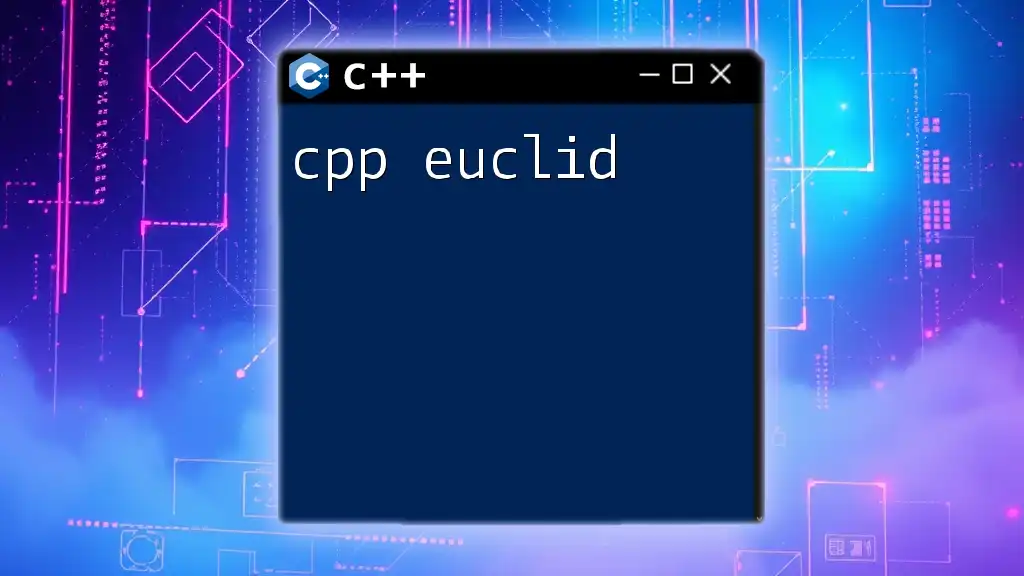
Basics of Enum Class
Syntax of an Enum Class
The syntax for defining an enum class is straightforward. Here is how you can define an enum class in C++:
enum class Color { Red, Green, Blue };
How to Use Enum Class Values
Once you've defined an enum class, you can create a variable of that enum type and assign it values. To access the values, you must use the dot operator, which is where the scoping aspect mentioned earlier comes into play:
Color myColor = Color::Red;
In this example, `myColor` is assigned the value `Color::Red`. Attempting to use `Red` without the `Color::` qualifier would result in a compilation error.
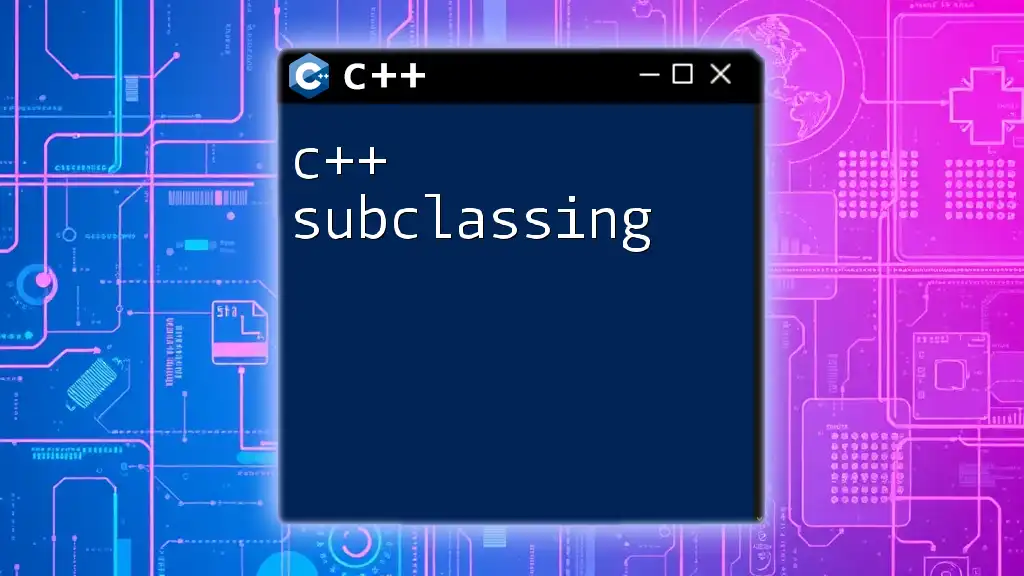
Differences Between Traditional Enum and Enum Class
Type Safety
One of the standout features of the cpp enum class is type safety. With traditional enums, an enumerator can be implicitly converted to an integer:
enum Color { Red, Green, Blue };
int num = Green; // This is valid with traditional enums
However, with an enum class, there’s no such implicit conversion, enhancing type safety:
enum class Color { Red, Green, Blue };
// int num = Color::Green; // This will cause a compilation error
Scoping
Traditional enums provide their enumerators in the global scope, which can lead to naming conflicts. For example:
enum Color { Red, Green, Blue };
enum TrafficLight { Red, Green, Yellow }; // This results in a naming conflict
In contrast, enum classes solve this issue by keeping the enumerators scoped:
enum class Color { Red, Green, Blue };
enum class TrafficLight { Red, Green, Yellow }; // No conflicts here
Now, you can access them using the appropriate qualifiers, such as `Color::Red` and `TrafficLight::Red`.
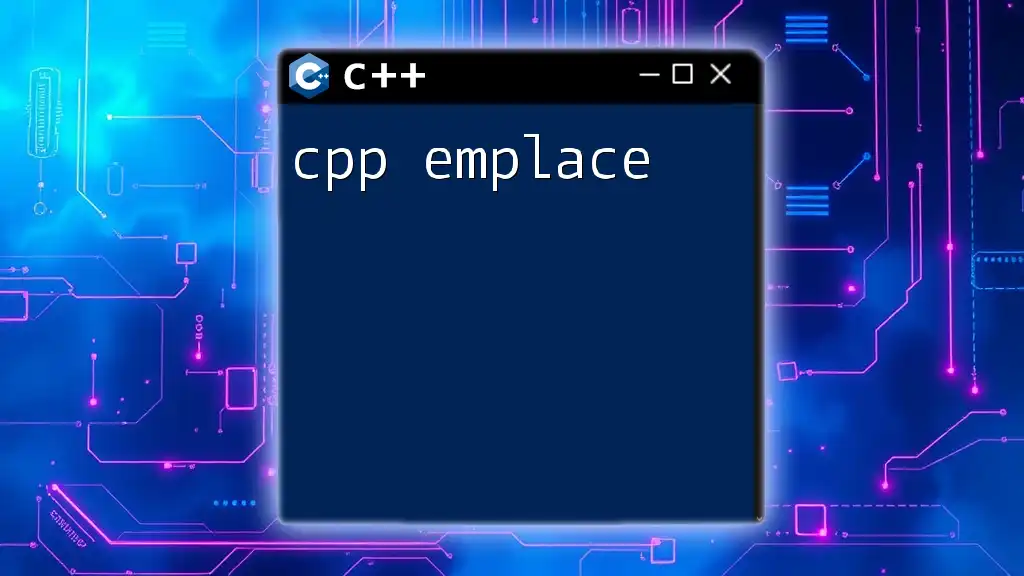
Advanced Features of C++ Enum Class
Specifying the Underlying Type
One advantage of enum classes is the ability to specify an underlying type. This allows you to customize the storage size of your enumerators.
enum class Direction : char { North, South, East, West };
By using `char` as the underlying type, this enum class will occupy less memory, which can be quite beneficial in memory-constrained environments.
Strongly Typed Enumerations
Enum classes provide strongly typed enumerations, which enhances code readability and maintainability. Unlike traditional enums, where you might inadvertently mix types, enum classes ensure that operations remain type-safe.
enum class Status { Success, Failure };
Status code = Status::Success;
// Status code2 = Status::Success; // Valid
// int s = code; // Invalid, won't compile
These features ensure that you aren’t mistakenly mixing enum values with other data types.
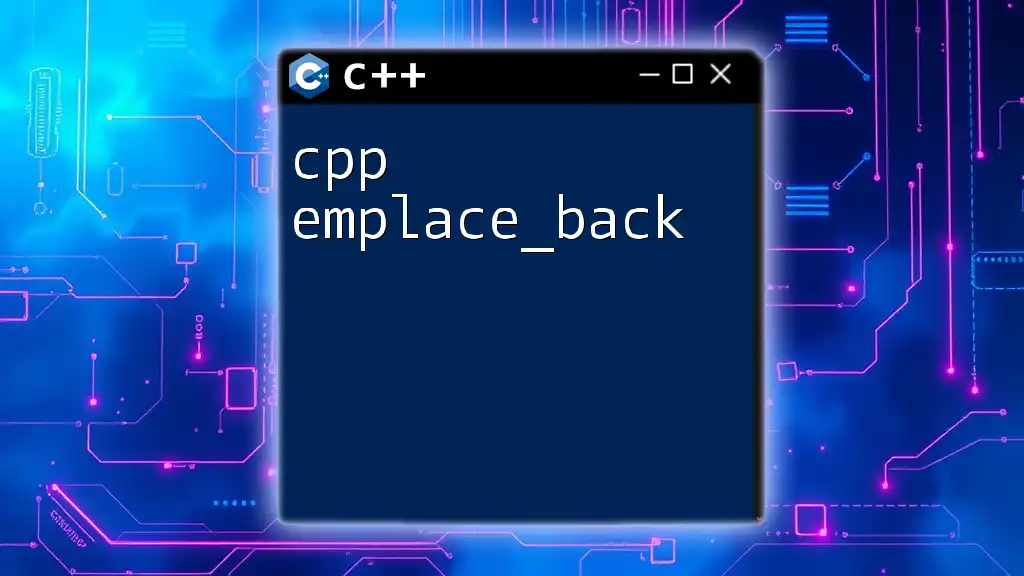
Practical Applications of Enum Class in C++
Incorporating Enum Class in Switch Cases
Using an enum class in switch statements is straightforward and enhances clarity. Below is a simple example:
switch (myColor) {
case Color::Red:
// handle red
break;
case Color::Green:
// handle green
break;
case Color::Blue:
// handle blue
break;
}
This use of enums makes it easy to understand what cases you’re handling, especially in large switch statements.
Using Enum Class with Classes and Structs
Enum classes can also be beneficial when used as members of classes or structs, adding an extra layer of organization to your data structures.
class Vehicle {
public:
enum class Type { Car, Truck, Motorcycle };
Type vehicleType;
};
In this example, the `Type` enum class is a member of the `Vehicle` class, ensuring that any vehicle can only take on specified types.
Thread Safety and Enum Class
In multi-threaded environments, you often need to ensure that your variables are thread-safe. Using enum classes adds a layer of safety since their type guarantees that only valid enumerators can be used, reducing the chances of accidental data corruption across threads.
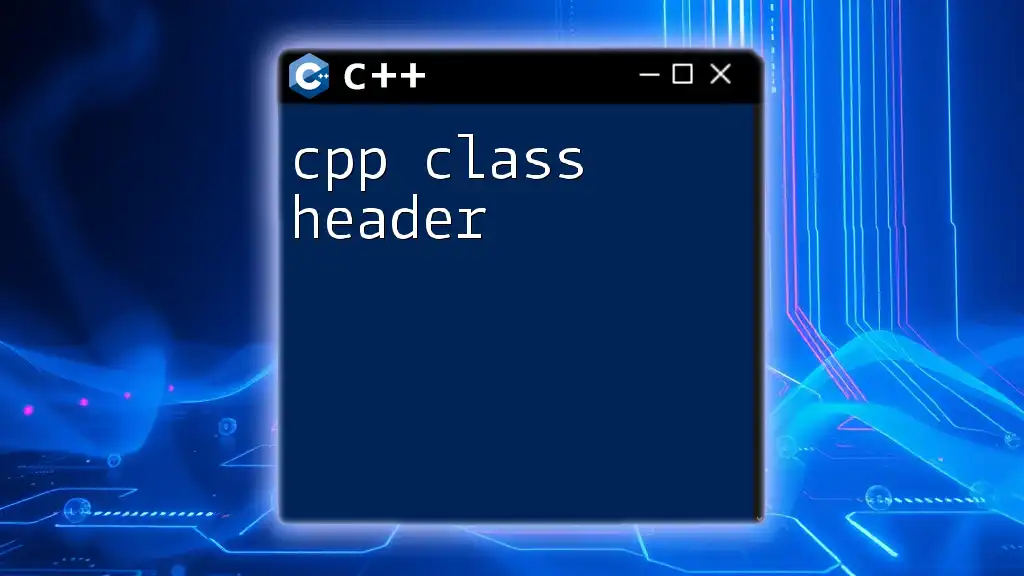
Best Practices
When to Use Enum Class Over Traditional Enum
When designing your classes and structures, consider using an enum class instead of a traditional enum when:
- You need type safety to avoid unintended conversions.
- You want to prevent naming conflicts for better code organization.
- You are working with a large codebase where clarity and maintainability are paramount.
Naming Conventions for Enum Classes
Adhere to clear and consistent naming conventions for your enum classes and their enumerators. Typically, name the enum class with a noun (e.g., `Color`, `Direction`) and use PascalCase for enumerator names (e.g., `Red`, `North`).
Common Pitfalls
Be mindful of:
- Ambiguities: Avoid combining enums and traditional enums in ways that could lead to confusion.
- Initialization: Ensure the enum class variables are always properly initialized before usage to optimize program flow and avoid undefined behavior.
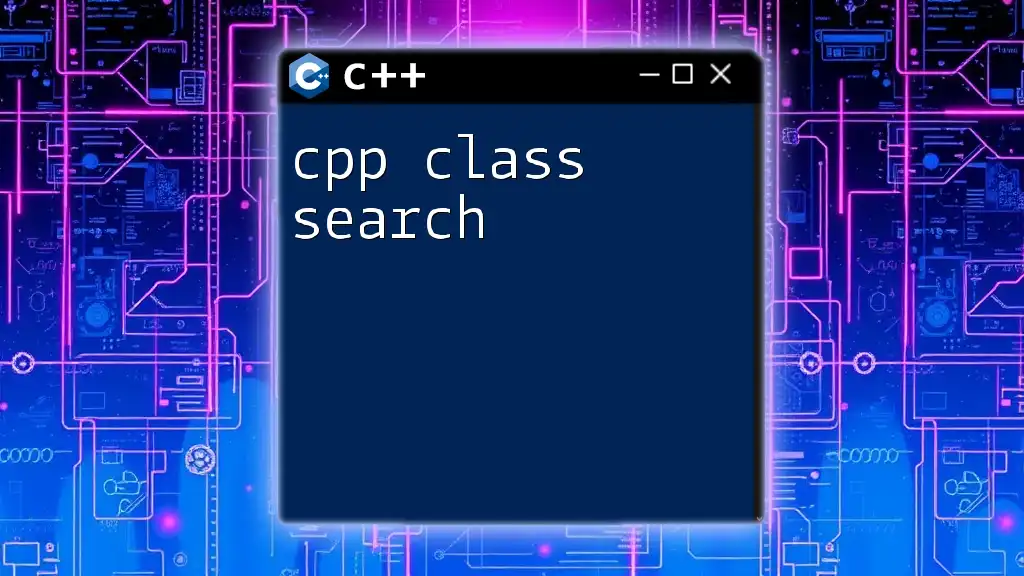
Conclusion
C++ enum classes, encapsulated in the term cpp enum class, provide modern developers with robust tools for defining enumerated types that enhance both type safety and code clarity. By choosing enum classes over traditional enums, you can write cleaner, safer, and more maintainable code in your C++ projects. Practicing their implementation will solidify your understanding and capability in using this powerful feature effectively.