In C++, `enum class` provides a strongly typed enumeration that prevents implicit conversions and name clashes, whereas the traditional `enum` allows for implicit conversions and can lead to naming conflicts.
enum Color { Red, Green, Blue }; // Traditional enum
enum class ColorClass { Red, Green, Blue }; // Strongly typed enum class
Understanding Enums in C++
What is an Enum?
An enum (short for enumerator) in C++ is a user-defined type that consists of a set of named integral constants. Enums help create a meaningful representation of a group of related values, enhancing code readability. The basic syntax for defining an enum looks like this:
enum Color {
Red,
Green,
Blue
};
In this example, we define an enum called `Color` that consists of three distinct values: `Red`, `Green`, and `Blue`. Each enum constant is associated with an integral value, with the first value defaulting to zero, and the subsequent values automatically incrementing by 1.
Advantages of Using Enums
Using enums offers numerous advantages:
-
Clarity and Readability: Enums provide intuitive names for values, making the code more readable compared to using mere integer literals.
-
Type Safety: Enums restrict the values that the variable can take, enforcing constraints at compile time and helping prevent errors.
-
Related Constants Maintenance: Enums make it easier to manage and update a related group of constants in one central place.
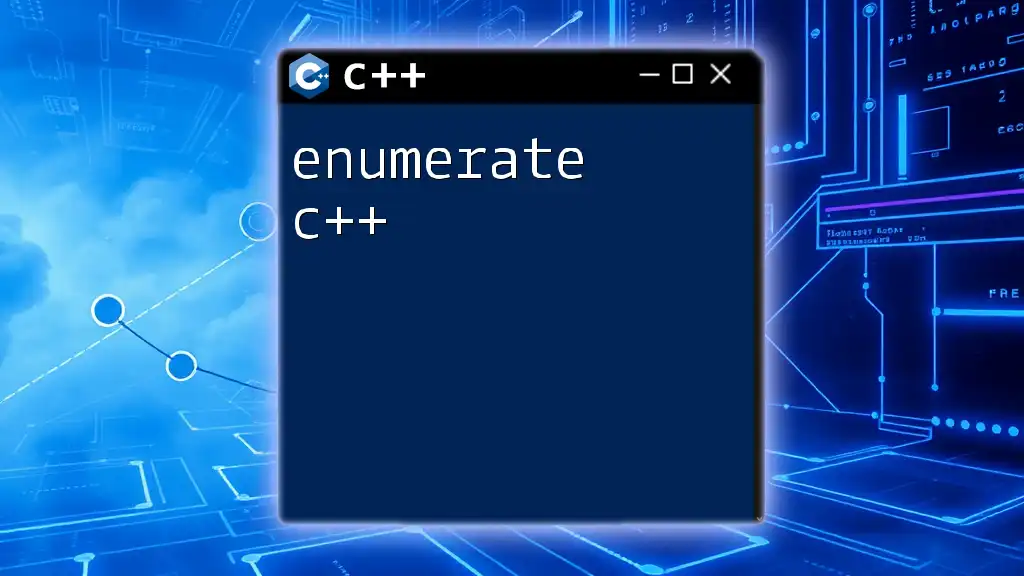
Enum vs Enum Class in C++
Key Differences
The differences between `enum` and `enum class` can significantly impact how you structure your code in C++.
Scoped vs Unscoped Enums
An important distinction is between scoped enums (declared as `enum class`) and unscoped enums (declared as `enum`).
In unscoped enums, the enumerators are injected into the enclosing scope, which can lead to name collisions. In contrast, scoped enums have their members encapsulated within the enum's scope. This means that you need to specify the enum's name when accessing its enumerators, as shown in the following examples:
For unscoped enums:
enum Color {
Red,
Green,
Blue
};
// Usage
Color myColor = Green; // No qualification needed
For scoped enums:
enum class Color {
Red,
Green,
Blue
};
// Usage
Color myColor = Color::Green; // Qualification required
Implicit Conversions
Another stark difference is the provision of implicit conversions. In unscoped enums, implicit conversions from the enum type to its underlying integral type are allowed.
enum Color {
Red,
Green,
Blue
};
Color myColor = Green;
int intColor = myColor; // Implicit conversion to int
However, in scoped enums, such implicit conversions do not occur:
enum class Color {
Red,
Green,
Blue
};
Color myColor = Color::Green;
// int intColor = myColor; // Error: cannot convert to int
Trying to perform an implicit conversion with `enum class` will result in a compile-time error, enforcing type safety.
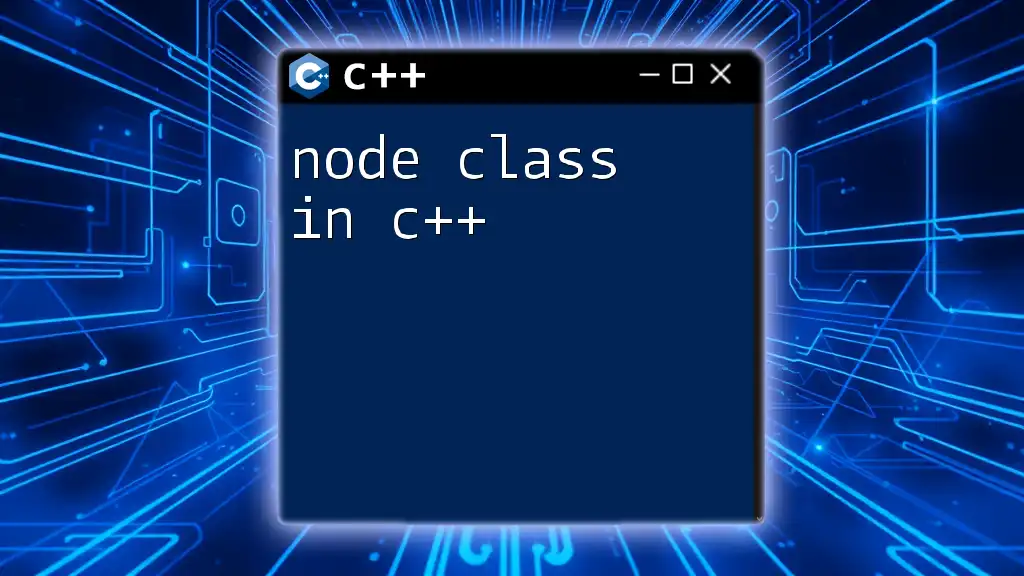
Benefits of Using Enum Class
Stronger Type Safety
One of the main advantages of using `enum class` is the stronger type safety it provides. Since scoped enums do not allow automatic conversions to their integral types, they reduce the risk of accidental misuse and increase code reliability. For instance, if you define multiple enums that can have overlapping enumerator names, `enum class` will keep them distinct within their scopes, minimizing the potential for bugs.
Collision Avoidance
With unscoped enums, you may run into naming conflicts if different enums contain the same enumerator names. Consider this example:
enum Color {
Red,
Blue
};
enum Shape {
Red, // This will cause a conflict
Circle
};
The above code snippet will present conflicts due to both `Color` and `Shape` containing a `Red` enumerator. To avoid this, using scoped enums eliminates the collision issue:
enum class Color {
Red,
Blue
};
enum class Shape {
Red, // No conflict, retains its own scope
Circle
};
This feature makes `enum class` a preferred choice in larger projects where code is often dispersed across multiple files and modules.
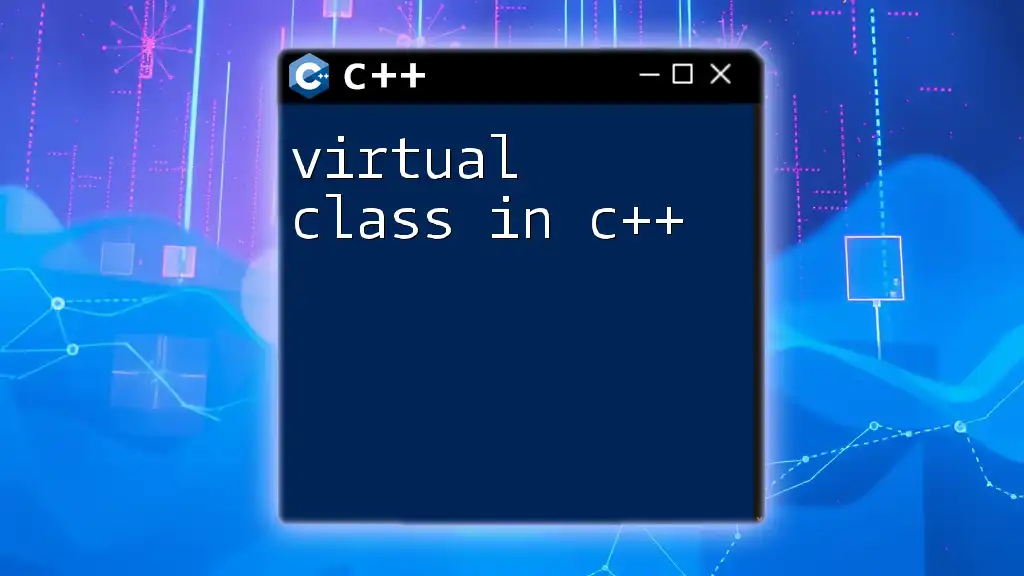
When to Use Enum vs Enum Class
Use Cases for Enum
Unscoped enums can be beneficial for simple cases, such as when you need related constants without much concern for name collisions, or in scenarios where you require implicit conversions, for instance in legacy codebases.
Use Cases for Enum Class
On the other hand, `enum class` is the ideal choice when you prioritize type safety and need to prevent naming conflicts, especially in sizable codebases or when you’re working in a modular programming environment. If you want to ensure that your enumerators are used consistently and avoid potential bugs that arise from naming conflicts, `enum class` is the way to go.
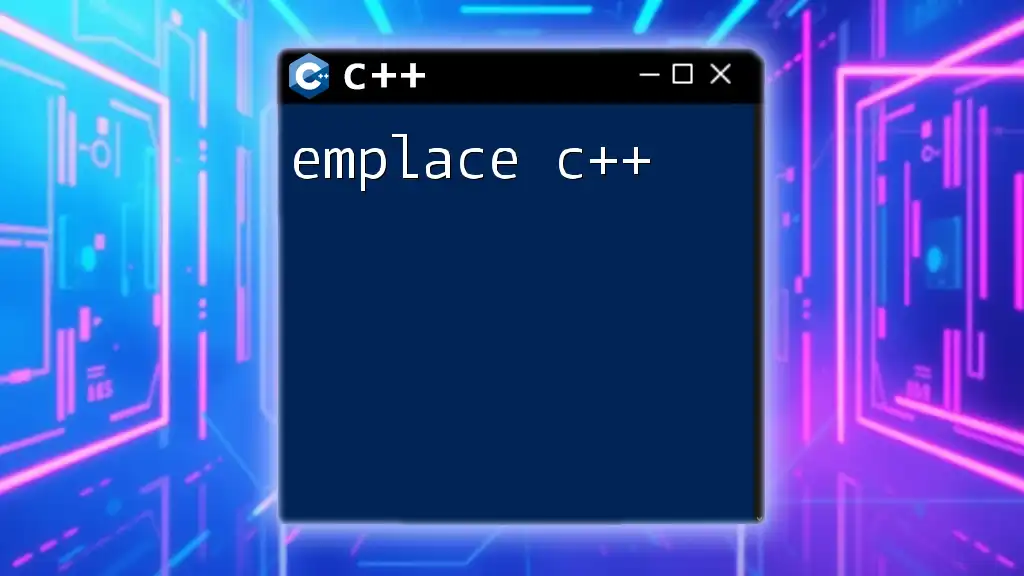
C++ Best Practices for Enums
Naming Conventions
When working with enums, it is crucial to adhere to some naming conventions for clarity. Generally, enum names should be in PascalCase, while enumerator names can be in PascalCase or UpperSnakeCase. For instance:
enum class Direction {
Up,
Down,
Left,
Right
};
Combining Enums with Other Types
Enums can be seamlessly used with control structures, like `switch` statements. This leads to cleaner and more understandable code structures. Here is an example:
Color myColor = Color::Red;
switch (myColor) {
case Color::Red:
// Handle the case for Red
break;
case Color::Green:
// Handle the case for Green
break;
case Color::Blue:
// Handle the case for Blue
break;
default:
// Handle unexpected values
break;
}
Furthermore, enums can be included within classes and structs, promoting organized code and encapsulation.
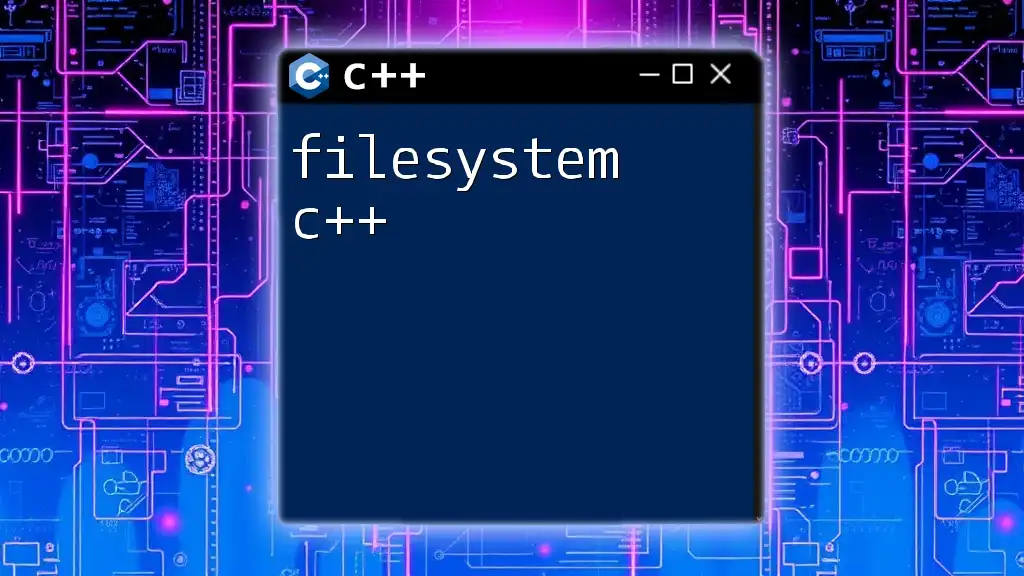
Conclusion
In summary, understanding the differences between `enum` and `enum class` in C++ is crucial for writing efficient and error-free code. The enum class vs enum c++ debate boils down to type safety versus ease of use. Depending on the requirements of your specific context, choosing wisely between these two options can significantly enhance your coding practices. By recognizing when to use each type, you can ensure that your enum usage is both effective and reliable, making your C++ programming much more robust.