In C++, a class variable, also known as a data member, is a variable that is declared within a class and is used to store the state of an object.
class MyClass {
public:
int myVariable; // Class variable
};
What is a Class Variable in C++?
A class variable in C++ is a variable that belongs to the class itself rather than to any specific instance of the class. This means that all instances of the class share the same class variable, rather than each instance having its own copy.
Difference Between Class Variables and Instance Variables
The main distinction between class variables and instance variables lies in their scope and usage:
-
Class Variables: These are declared with the `static` keyword and are shared among all instances of a class. Changes made to a class variable are reflected across all objects.
-
Instance Variables: These are specific to each instance of the class. Each object has its own separate copy of instance variables.
For example:
class MyClass {
public:
static int classVar; // Class variable
int instanceVar; // Instance variable
};
In this example, `classVar` is a class variable shared by all instances of `MyClass`, while `instanceVar` is unique to each instance of `MyClass`.

Declaring Class Variables in C++
Syntax for Class Variable Declaration
Declaring a class variable requires specifying the `static` keyword within the class definition. The syntax is straightforward:
class MyClass {
public:
static int classVariable; // Class variable declaration
};
Access Modifiers and Class Variables
Class variables can have access modifiers just like regular variables. Here’s a brief overview of how the access specifiers affect class variables:
- Public: Accessible from any part of the code.
- Private: Only accessible within the class itself.
- Protected: Accessible in the class itself and by derived classes.
Declaring a class variable as private is a common practice to restrict direct access and enforce encapsulation.
Example: Declaring Class Variables
Consider the following example that defines a class variable:
class Counter {
private:
static int count; // private class variable
public:
static void increment() {
count++;
}
static int getCount() {
return count;
}
};
In this `Counter` class, `count` is a private class variable only accessible through the public static methods `increment()` and `getCount()`.

Initializing Class Variables
Static Keyword in Initialization
Class variables must be initialized outside the class definition since space for static variables is allocated only once. This is achieved using the `static` keyword.
Example of Initialization
Here’s how you initialize a static class variable:
int Counter::count = 0; // Initialization outside the class
This line defines and initializes the class variable `count`, setting it to zero at the start of the program.
Best Practices for Initialization
- Always initialize class variables outside the class declaration to avoid linker errors.
- Set default values where applicable to ensure the integrity of the variable before any operations are performed.

Accessing Class Variables
How to Access Class Variables Using Class Name
Accessing class variables is done using the class name followed by the scope resolution operator `::`. This emphasizes that the variable belongs to the class itself.
Example of accessing a class variable:
Counter::increment(); // Increment the counter
int totalCount = Counter::getCount(); // Get the total count
Accessing Class Variables from Objects
Technically, you can access class variables through an instance of the class, but it is not recommended. Doing so can lead to confusion, as it may imply that each instance has its own copy.
Example demonstrating instance access:
Counter obj;
obj.increment(); // Possible but not recommended
Utilizing class names adds clarity and emphasizes the shared nature of class variables.
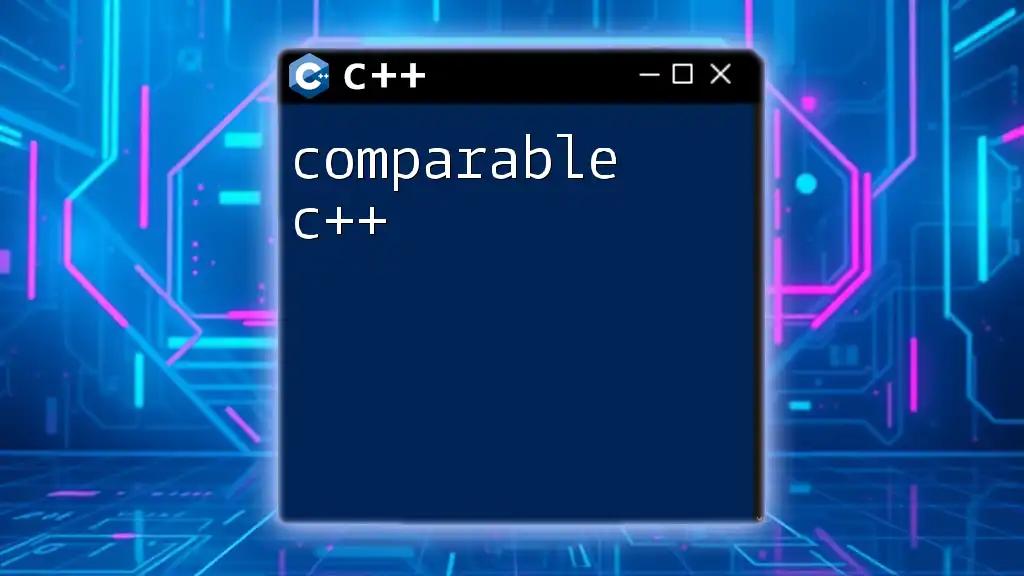
Use Cases and Benefits of Class Variables
When to Use Class Variables
Class variables are beneficial when you need to maintain a common state that is shared across all instances of a class. Use class variables to keep track of:
- Counts: E.g., tracking how many objects of a class have been created.
- Flags: E.g., a single configuration option that affects all instances.
Examples of Real-world Applications
One common application of class variables is in the implementation of the Singleton design pattern. This pattern restricts the instantiation of a class to one object and provides a global access point to it, often using a static class variable.

Common Pitfalls and Best Practices
Common Mistakes When Using Class Variables
- Misunderstanding scope and lifetime: Remember that class variables persist for the duration of the program, which may lead to unexpected behavior if not handled properly.
- Using class variables without encapsulation can lead to data integrity issues; always use access modifiers to protect data.
Best Practices for Using Class Variables in C++
- Use `static` class variables judiciously and ensure proper thread safety when accessing them in multi-threaded environments.
- Keep class variables minimal and meaningful; excessive use can make the code less maintainable.

Conclusion
Class variables are powerful tools in C++ that enhance the capabilities of object-oriented programming by allowing shared data across instances of a class. Understanding how to declare, initialize, access, and effectively utilize class variables can significantly improve your proficiency with C++. Experiment with class variables in your code to grasp their utility and best practices fully.

Additional Resources
For further exploration, consider reading about C++ classes, object-oriented programming principles, and advanced features of C++. Online coding platforms and interactive C++ tutorials can also provide invaluable hands-on practice.

FAQs
What is the difference between a class variable and a static variable?
Class variables are a specific type of static variable tied to a class, while static variables can also exist at broader scopes such as within functions, retaining their values between function calls.
Can class variables be private or protected?
Yes, class variables can be declared private or protected, restricting access according to the defined access control.
How can I reset a class variable's value?
You can reset a class variable by directly assigning a new value to it, but ensure you manage how this impacts all instances of the class sharing that variable.