`static_assert` in C++ is a compile-time assertion mechanism that checks a condition during compilation and triggers a compilation error if the condition is false.
Here's a code snippet demonstrating `static_assert`:
#include <type_traits>
static_assert(std::is_integral<int>::value, "int must be an integral type!");
int main() {
return 0;
}
What is `static_assert`?
`static_assert` is a compile-time assertion mechanism in C++ that allows programmers to enforce certain conditions at compile time rather than at runtime. This means that if the expression in `static_assert` evaluates to false, the compiler will generate an error, preventing the code from compiling. This robust feature is particularly useful for catching errors early in the development process, ensuring that the code adheres to essential requirements.
Comparing `static_assert` to Traditional Assertions
Traditional assertions, such as those provided by the `<cassert>` library, evaluate conditions during runtime. This can lead to the potential for errors to manifest when the application is executed. In contrast, `static_assert` allows developers to catch these issues at compile time, leading to safer and more reliable code.
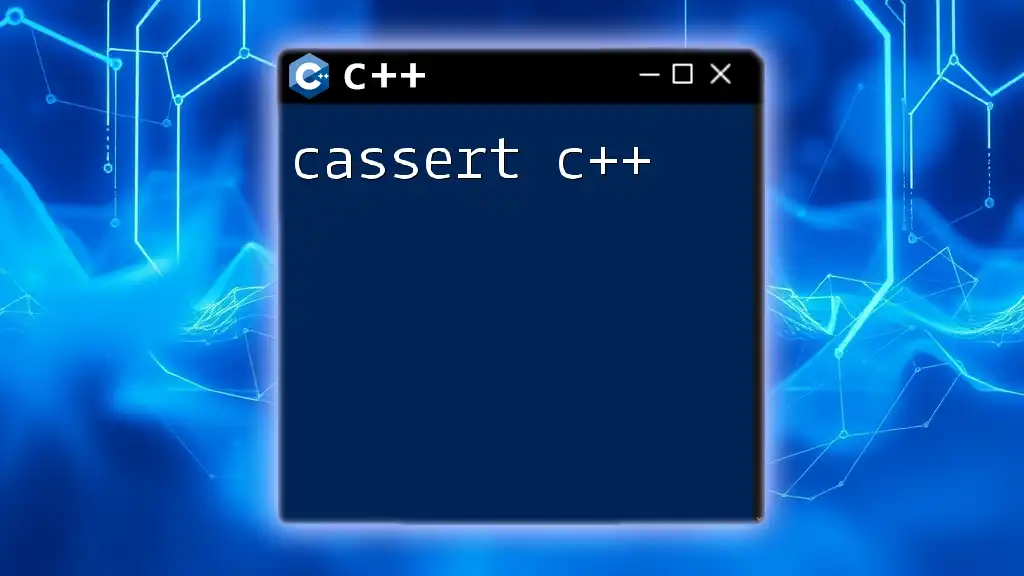
Benefits of Using `static_assert`
Employing `static_assert` comes with several advantages:
-
Early Error Detection: By checking conditions at compile time, developers can identify mistakes before the code is ever executed, which saves invaluable debugging time later on.
-
Improved Code Readability: When `static_assert` is utilized, it clearly communicates constraints and intended usage directly in the code, making it easier for others to understand the expected conditions.
-
Enhanced Safety in Template Programming: In template code, different types can be passed in, and `static_assert` can guarantee that these types adhere to specified criteria, preventing errors that could arise from inappropriate type usage.
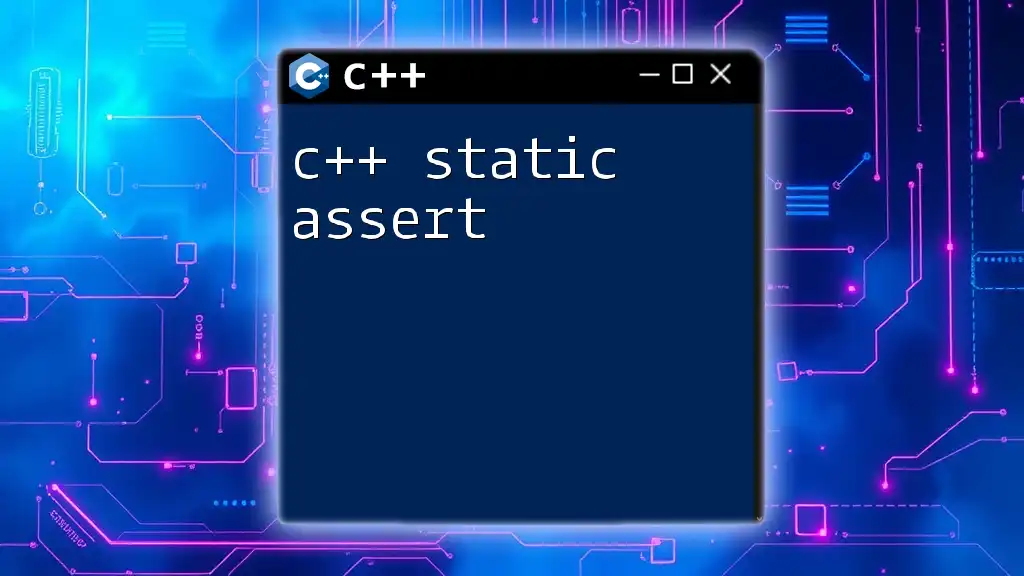
Syntax and Structure of `static_assert`
Basic Syntax
The syntax for `static_assert` is straightforward:
static_assert(expression, message);
Here, expression is a compile-time constant that is evaluated, and message is a string that gets displayed if the assertion fails.
Expression and Message Components
Expression
The expression can be any constant expression. When this expression evaluates to `false`, a compile-time error is triggered. For instance, checking sizes or properties of types can be an effective use:
static_assert(sizeof(int) == 4, "Size of int must be 4");
Message
The message parameter serves as feedback to the developer, explaining the assertion's failure. This message should be descriptive enough to ease debugging efforts. For example, the message can clearly indicate what expectation was not met:
static_assert(sizeof(void*) == 8, "Pointer size must be 8 bytes on 64-bit systems");
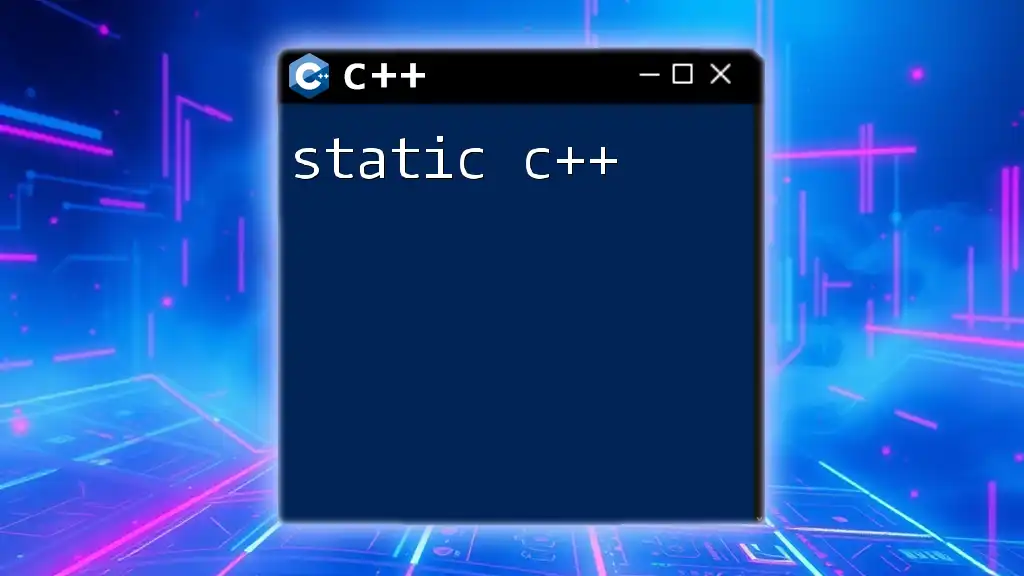
Practical Use Cases for `static_assert`
Type Traits and Type Checks
Using `static_assert` is commonplace in type checks. For example, it can be employed to ensure that a specified type is integral:
static_assert(std::is_integral<T>::value, "T must be an integral type");
Template Programming
In template code, `static_assert` can restrict the types that can be passed:
template <typename T>
void process(T value) {
static_assert(std::is_arithmetic<T>::value, "T must be an arithmetic type");
// Processing code goes here
}
This guarantees that only valid types are accepted during template instantiation.
Function Parameter Validation
Compile-time checks on function template arguments can also be done using `static_assert`. For example:
template <typename T>
void checkValue(T value) {
static_assert(std::is_floating_point<T>::value, "T must be a floating-point type");
// Function logic here
}
Here, if a user attempts to call `checkValue` with a non-floating point type, the compilation will fail immediately.
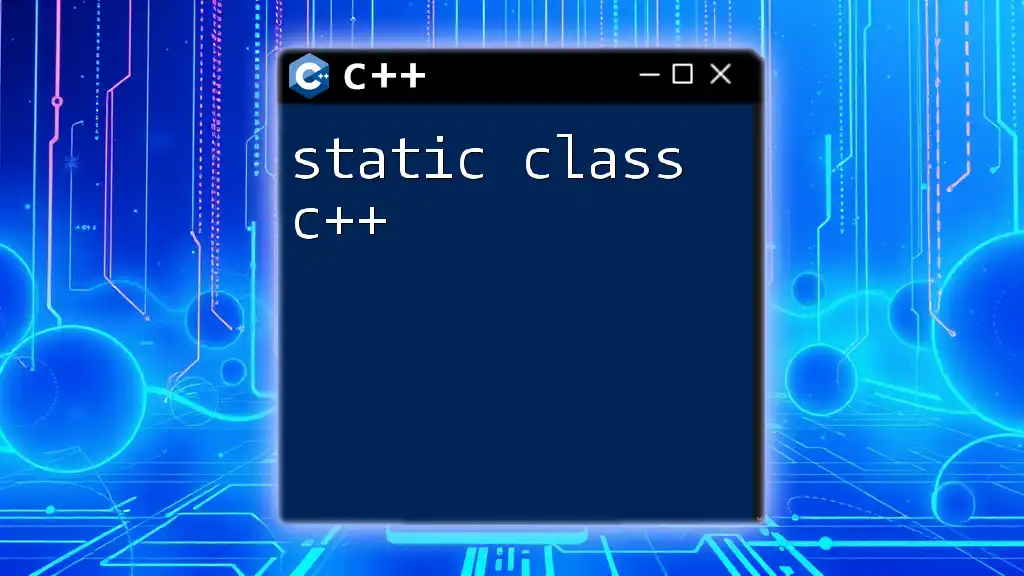
Advanced Usage of `static_assert`
Combining Multiple Assertions
In scenarios where complex conditions are necessary, several `static_assert` statements can be employed:
static_assert(sizeof(int) == 4, "Size of int must be 4 bytes");
static_assert(std::is_integral<int>::value, "int must be an integral type");
This permits multiple conditions to be asserted, allowing for rigorous validation.
Conditional Compilation with `static_assert`
For platform-specific code, `static_assert` can be useful:
#if defined(__x86_64__)
static_assert(sizeof(void*) == 8, "64-bit architecture requires 8 byte pointers");
#else
static_assert(sizeof(void*) == 4, "32-bit architecture requires 4 byte pointers");
#endif
Such assertions can direct the compiler to accommodate specific architectures, enhancing portability.
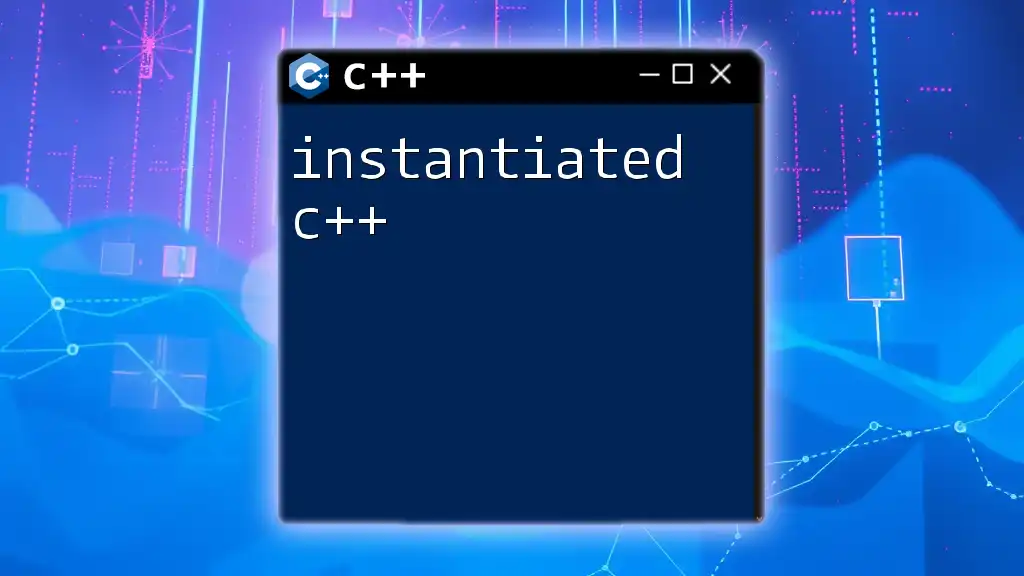
Common Pitfalls and Best Practices
Understanding scope and context is crucial. `static_assert` can only be placed in locations where it is valid in the syntax of C++. For instance, trying to use `static_assert` within a function or outside a class context will result in errors.
Writing Clear Error Messages
The clarity of the error message is key. Take time to craft error messages that explain the exact nature of the failure. For instance, instead of vague messages like "assertion failed", consider clearer variations like "template argument must be a pointer type".
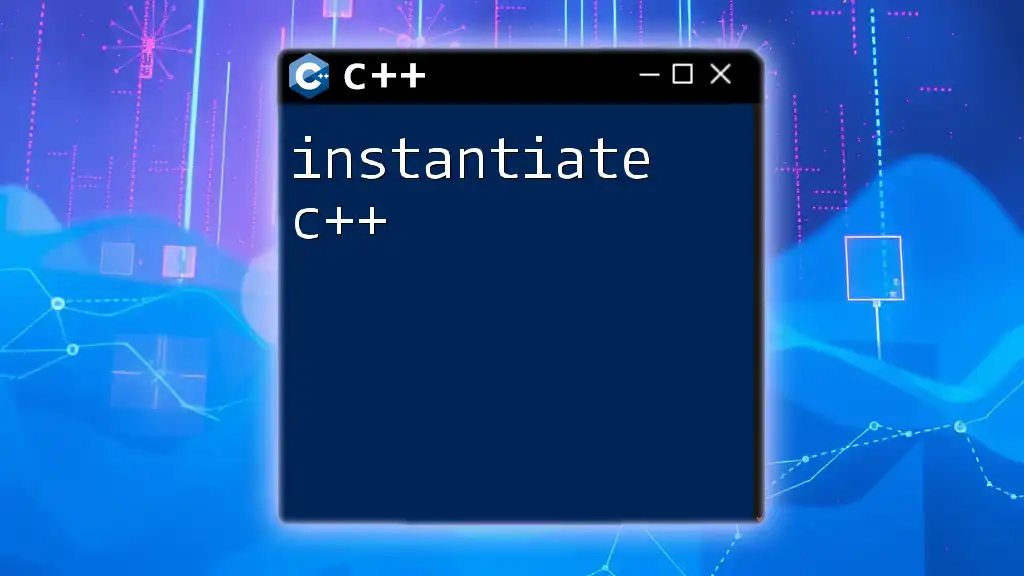
Real-World Examples
Example 1: Basic Usage
Using `static_assert` to verify size:
static_assert(sizeof(int) == 4, "Unexpected size of int");
This assertion checks that the size of the `int` data type is exactly 4 bytes and helps prevent platform-specific assumptions from causing errors.
Example 2: Template Example
In this example, `static_assert` validates type parameters in a function template:
template <typename T>
void process(T value) {
static_assert(std::is_integral<T>::value, "T must be an integral type");
// Processing code
}
This ensures that the `process` function can only accept integral types during instantiation, enhancing type safety.
Example 3: Advanced Template Constraints
Here’s another useful template example that ensures a variable is an arithmetic type:
template <typename T>
void checkValue(T value) {
static_assert(std::is_arithmetic<T>::value, "T must be an arithmetic type");
}
This allows for straightforward functions that operate correctly, irrespective of the specific arithmetic type provided.
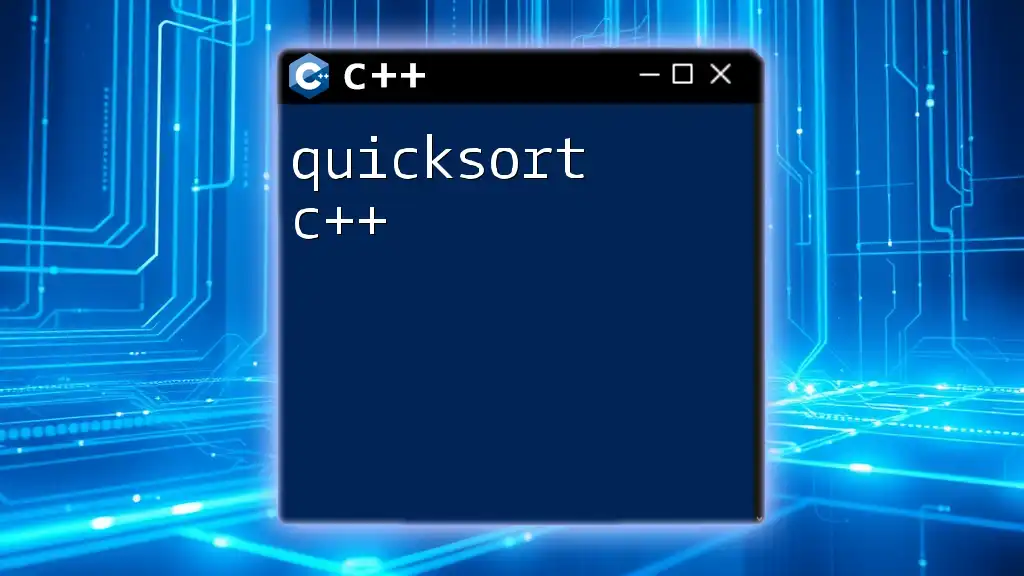
Conclusion
`static_assert c++` is a powerful feature that allows developers to enforce compile-time constraints effectively. These assertions promote error detection, improved code clarity, and safer template programming practices. By understanding the nuances of its usage, programmers can significantly enhance the reliability of their C++ applications.
Additional Resources
For further information, developers are encouraged to explore the official C++ documentation for `static_assert`, and delve into related topics concerning compile-time programming and type traits to deepen their knowledge.