Member variables in C++ are variables that are declared within a class and represent the attributes or properties of the objects created from that class. Here's a concise example:
class Car {
public:
std::string brand; // Member variable
int year; // Member variable
};
Understanding Member Variables
What are Member Variables?
Member variables are essential components of a class in C++. They represent the attributes or properties of an object. Each class can have its own set of member variables that define its state. In essence, member variables allow you to store the unique data associated with each instance of the class, enabling the representation of real-world entities in your software.
Importance of Member Variables in C++
Member variables play a critical role in object-oriented programming (OOP) by enabling encapsulation, inheritance, and polymorphism. By grouping data and behavior together in classes, member variables help to create a structure that enhances code reusability and maintainability. Encapsulation, in particular, protects the internal state of an object and only allows interaction through well-defined interfaces.

Types of Member Variables
Instance Variables
Instance variables are unique to each object created from the class. They hold the state that is specific to that particular object.
class Car {
public:
std::string color; // Instance variable
};
In the example above, every instance of `Car` can have a different `color`, reflecting the distinct properties of each car object.
Static Variables
Unlike instance variables, static variables are shared among all instances of a class. This means that there is only one copy of a static variable, regardless of how many objects are created from the class.
class Car {
public:
static int numOfCars; // Static variable
};
// Initializing the static variable
int Car::numOfCars = 0;
Using static member variables can be beneficial when you need to maintain a count of all instances or share common data across objects.
Constant Variables
Constant variables are declared using the `const` keyword, meaning their value cannot change once set. This is useful when you want to prevent modification of important constants throughout your program.
class Circle {
public:
static const double pi; // Constant variable
};
const double Circle::pi = 3.14159; // Definition outside the class
Constant member variables ensure that values critical to the calculations or operations of the class remain unchanged, promoting reliability.

Declaring Member Variables
Syntax for Declaration
Member variables are typically declared inside the class. The syntax involves specifying the access specifier followed by the type and name of the variable.
class Person {
private:
std::string name; // Private member variable
public:
int age; // Public member variable
protected:
float height; // Protected member variable
};
Here, the `name` variable is private and cannot be accessed directly from outside the class, while `age` is public and can be viewed and modified freely.
Access Specifiers
Access specifiers determine the visibility and accessibility of member variables. The three primary access specifiers are:
- Public: Member variables declared as public can be accessed from outside the class.
- Private: Private member variables are accessible only within the class itself. This is fundamental to achieving encapsulation.
- Protected: Protected member variables can be accessed by the class itself and by derived classes.
Using these specifiers correctly is essential for maintaining the integrity and security of your data.

Initializing Member Variables
Constructor Initialization
Constructors allow you to initialize member variables when an object is created. This is effective for setting up an object’s state right from the start.
class Book {
public:
std::string title;
Book(std::string t) : title(t) {} // Constructor initialization
};
In this example, the `Book` constructor initializes the `title` of the book upon the creation of a `Book` object. This ensures that every new `Book` instance starts with a defined `title`.
Default Member Initializers
C++11 introduced default member initializers, allowing you to assign a default value to member variables directly in their declarations. This leads to cleaner and more readable code.
class Rectangle {
public:
int width = 10; // Default member initializer
int height = 5;
};
With this approach, `width` and `height` are initialized automatically if no values are provided.

Accessing Member Variables
Getter and Setter Methods
To protect the internal state of class objects, it’s essential to use getter and setter methods. These methods control access to member variables.
class Student {
private:
int grade;
public:
void setGrade(int g) { grade = g; }
int getGrade() { return grade; }
};
Using getters and setters ensures that validation can be applied when modifying member variables, contributing to better data integrity.
Direct Access
In situations where member variables are declared public, they can be accessed directly. This approach can simplify your code, although it generally undermines encapsulation.
class Animal {
public:
std::string species; // Directly accessible
};
Here, the `species` variable can be accessed and modified directly from any instance of `Animal`, which could lead to unintended consequences in a larger codebase.

Best Practices for Using Member Variables
Naming Conventions
It’s important to maintain clear and consistent naming conventions for member variables. Use descriptive names that reflect the purpose of the variable, making the code easier to read and understand.
Common styles include:
- `camelCase` (e.g., `numberOfLegs`)
- `snake_case` (e.g., `number_of_legs`)
Stick to one style throughout your codebase to improve coherence.
Minimize Public Member Variables
While declaring member variables as public can simplify access, it can also expose your data to risks. To enforce encapsulation, it's a best practice to minimize public member variables and rely on getters and setters for access. This controls how data is manipulated and avoids unintended side effects.
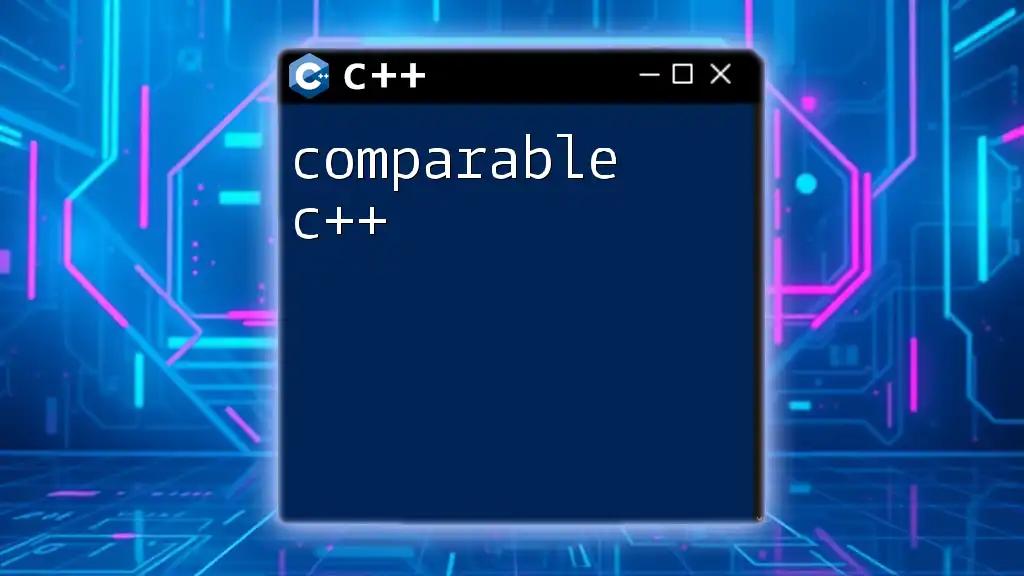
Conclusion
In summary, member variables in C++ are fundamental components of classes, representing the state associated with each instance. Understanding their types, declaration, initialization, access methods, and best practices is crucial for creating robust object-oriented applications. By leveraging member variables effectively, you can build clear, maintainable code that encapsulates data and behavior effectively.
Additional Resources
To deepen your understanding of C++ and related concepts, consider exploring further resources on OOP principles, design patterns, and best coding practices. There are numerous tutorials and documentation available to advance your programming skills.
Call to Action
Now that you have a comprehensive overview of member variables in C++, start applying these concepts by creating your own classes and experimenting with different member variable types and access methods. Happy coding!