In C++, a variable variable allows you to dynamically refer to another variable by using a string representation of its name, which can be useful in certain advanced scenarios, though it's important to note that C++ does not support variable variables in the same way that some other languages like PHP do.
Here's a simple example of using maps to achieve similar functionality:
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
std::unordered_map<std::string, int> variables;
variables["myVar"] = 42; // Define a variable with the name "myVar"
std::string varName = "myVar"; // Variable variable name
std::cout << "Value of " << varName << ": " << variables[varName] << std::endl; // Access the variable
return 0;
}
Understanding Variable Variables in C++
What is a Variable Variable?
A variable variable in C++ allows developers to reference variables dynamically. This concept is useful when we want to create a reference to another variable rather than working with a static or predefined value. By using variable variables, we can access and manipulate variables indirectly, which provides a level of flexibility in programming.
Historical Context
Variable variables are more common in languages like PHP, where referencing variables by names is a fundamental part of the syntax. In C++, however, the concept is approached differently. Instead of the straightforward variable variable syntax seen in PHP, C++ relies on pointers and references to achieve similar functionality. Understanding how variable variables evolved helps programmers appreciate the strengths and limitations of C++'s type system.
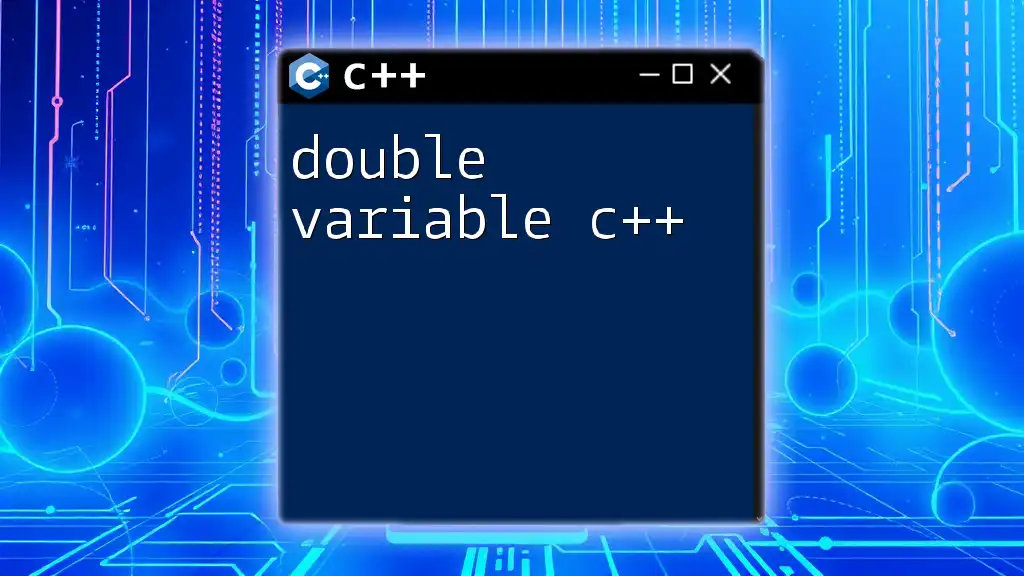
Basics of C++ Variables
Defining Variables in C++
To effectively utilize variable variables, one must first grasp the basics of defining variables in C++. In C++, variables are defined using a specific syntax, which includes the type followed by the variable name. For example:
int number;
This declares `number` as an integer variable. The essential point here is that variables in C++ must be defined before use, and they must have a specific type, which determines what kind of data they can hold.
Types of Variables in C++
Variables in C++ can be classified into different categories based on their scope and lifespan:
Local Variables
Local variables are declared within a function or block and can only be accessed within that context. Here’s a simple example:
void myFunction() {
int localVar = 5; // localVar is local to myFunction
cout << localVar;
}
In this example, `localVar` cannot be accessed outside of `myFunction`.
Global Variables
Global variables, on the other hand, are declared outside of any function and can be accessed throughout the file. Here’s an example:
int globalVar = 10; // This is a global variable
void myFunction() {
cout << globalVar; // Accessible within myFunction
}
Be cautious when using global variables, as they can lead to code that is harder to maintain.
Static Variables
Static variables maintain their state even after the function in which they were declared has finished executing. Here is an example to illustrate:
void myFunction() {
static int counter = 0; // Retains its value between calls
counter++;
cout << counter;
}
Every time `myFunction` is called, `counter` retains its value, allowing it to count the number of times the function has been executed.
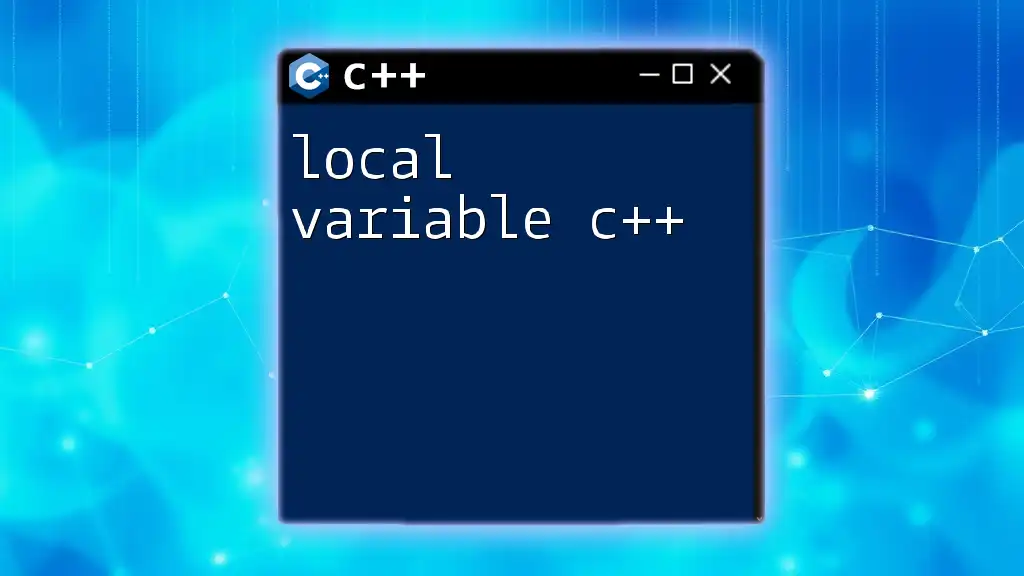
Delving Deeper into Variable Variables
Syntax and Implementation of Variable Variables
Implementing variable variables in C++ requires understanding pointers and multi-level pointers. Here’s a basic example that showcases variable variables:
int main() {
int x = 5;
int y = 10;
int *ptr = &x; // Pointer to x
int **varVar = &ptr; // Pointer to pointer
cout << **varVar; // Outputs 5
}
In this snippet, `ptr` holds the address of `x`, and `varVar` holds the address of `ptr`. When dereferencing `varVar` twice, we access the value of `x`, which is `5`. This is the essence of a variable variable: accessing a variable through multiple levels of indirection.
Practical Applications of Variable Variables
Variable variables are especially beneficial in scenarios that require dynamic data allocation, such as when working with complex data structures. For instance, in cases where the structure of data may change at runtime, using pointers and references can make your code much more flexible and efficient.
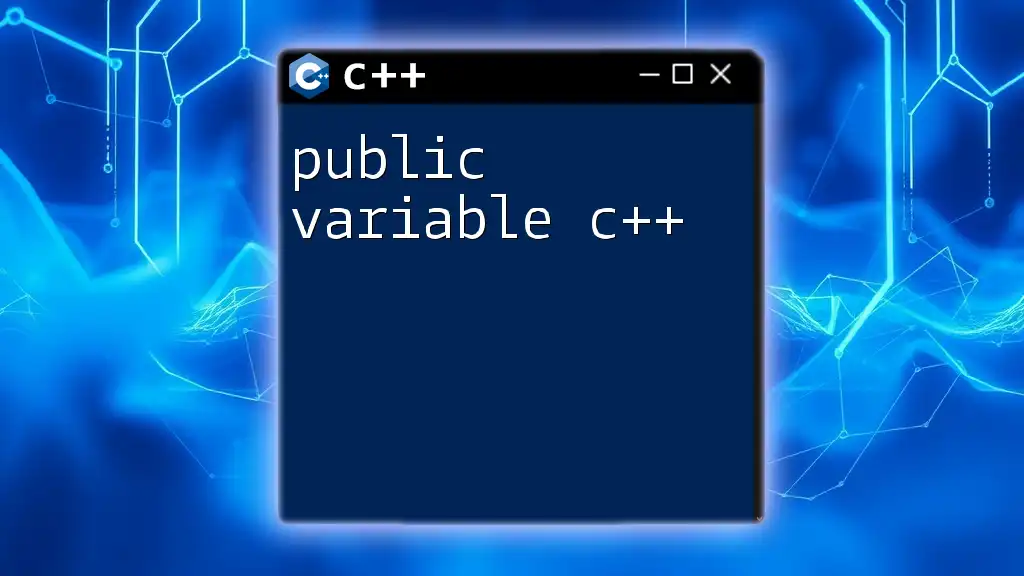
Advantages and Disadvantages of Variable Variables
Advantages
The primary advantage of using variable variables is the flexibility they offer in manipulating data. They allow for dynamic memory management, enabling developers to write functions that can handle varying amounts of data more efficiently. Additionally, they enhance functionality, facilitating complex operations that would otherwise require more cumbersome solutions.
Disadvantages
On the flip side, the use of variable variables can lead to complexity in your code, making it less readable and harder to debug. When the code involves multiple layers of pointers, tracking how different variables interact can be challenging. Furthermore, improper management of pointers can lead to serious issues, such as memory leaks or segmentation faults.
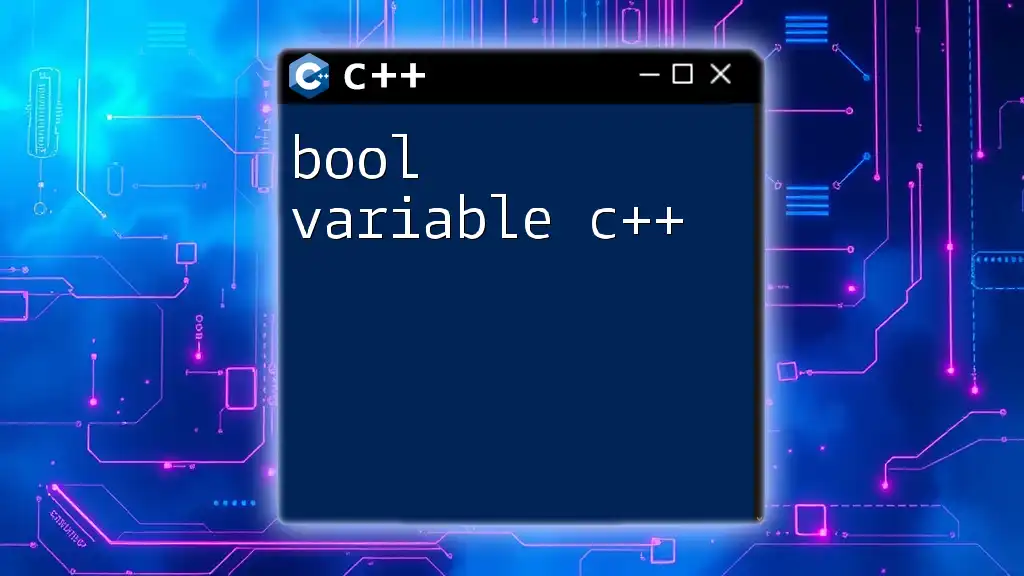
Use Cases in C++
Example 1: Function Pointer Implementation
Variable variables can also be utilized with function pointers to enhance code operation. Here’s a demonstration:
void functionOne() {
cout << "Function One Called!";
}
void functionTwo() {
cout << "Function Two Called!";
}
void (*functionPointer)() = functionOne;
functionPointer(); // Outputs: Function One Called!
In this example, `functionPointer` can dynamically reference any function signature that matches its type. If you change the pointer to refer to `functionTwo`, you'll dynamically switch the behavior of your program at runtime.
Example 2: Dynamic Configuration
Variable variables can also aid in managing dynamic configurations:
void changeSetting(int& setting) {
setting = 20; // Change setting value via reference
}
int main() {
int config = 10;
changeSetting(config);
cout << config; // Outputs: 20
}
The `changeSetting` function modifies the value of `config` directly. This illustrates how variable references allow for efficient data manipulation without the overhead of copying variables.
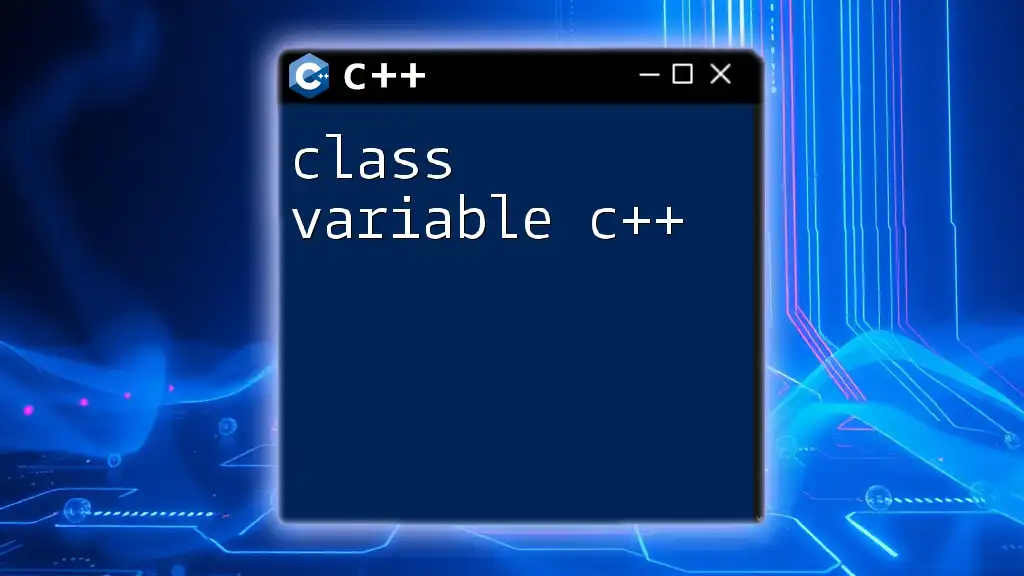
Conclusion
In summary, understanding variable variables in C++ opens the door to a range of advanced programming techniques. By harnessing pointers and references effectively, developers can create more dynamic and flexible code. However, it’s essential to balance the use of these concepts with simplicity and maintainability to avoid common pitfalls that arise from complex pointer usage.
By diving deeper into the world of C++ variables, both beginners and experienced programmers can enhance their skill set and tackle more complex programming challenges with confidence. For those eager to learn more, numerous resources—books, online courses, and communities—are available to further develop your understanding of variables in C++.