A `bool` variable in C++ is used to store one of two values: `true` or `false`, allowing for simple logical conditions in programming. Here's a quick example:
bool isRaining = false; // Declaring a boolean variable
if (isRaining) {
std::cout << "Don't forget your umbrella!" << std::endl;
} else {
std::cout << "Enjoy the sunshine!" << std::endl;
}
Understanding Bool in C++
In C++, a `bool` variable is a fundamental data type that represents two possible values: true and false. This variable type is essential for logic and decision-making in programming. Understanding how to effectively use `bool` is key to mastering C++.
Definition of `bool`
The `bool` keyword defines a Boolean variable in C++. Under the hood, Boolean values are often represented as integers: `false` is typically `0` and `true` is represented by any non-zero value. This allows for easy manipulation of Boolean values within numerical contexts.
Memory Size of a Bool in C++
A `bool` variable generally occupies 1 byte of memory in C++, although its true storage requirement can vary based on system architecture. This small memory footprint provides efficiency when handling numerous Boolean variables.
Comparison Between Boolean and Other Data Types
While `bool` is primarily for logical true/false values, it's important to distinguish it from other data types:
- `int`: Represent numeric values that can perform arithmetic operations.
- `char`: Used for single characters, such as 'a', 'b', or '1'.
- `float`/`double`: Used to represent decimal numbers.
In contrast, `bool` is mainly for logic and conditions, making it indispensable for programming constructs like if statements and loops.
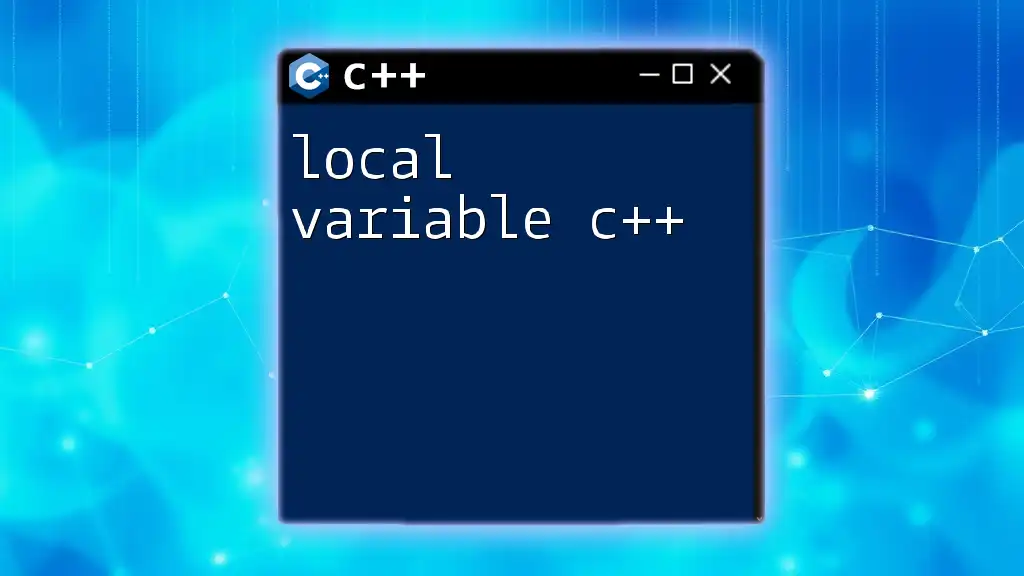
Declaring and Initializing Bool Variables in C++
Declaring a `bool` variable in C++ is straightforward:
How to Declare a Bool Variable
The syntax for declaring a Boolean variable is simple and clear:
bool variableName;
Example of Bool Variable Declaration
Here's a quick example of how to declare and initialize a `bool` variable:
bool isRaining = false;
In this case, the variable `isRaining` is set to false, indicating the lack of rain.
Initializing Bool Variables
You can initialize `bool` variables either directly or through expressions. Here's how:
bool hasAccess = true;
In this code snippet, `hasAccess` is set to true, meaning the user has access to a certain resource or area.
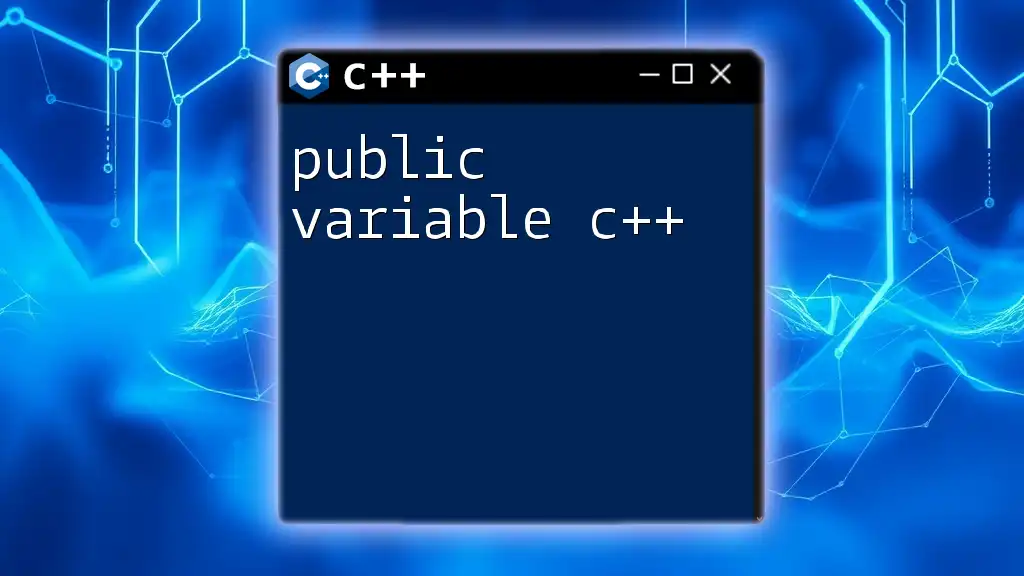
Using Boolean Expressions in C++
Boolean expressions evaluate conditions that yield a `true` or `false` outcome. Mastering Boolean logic is vital for effective coding in C++.
Definition of Boolean Expressions
A Boolean expression often involves using conditional comparisons, such as `==`, `!=`, `<`, `>`, `<=`, and `>=`.
Logical Operators with Booleans
Several logical operators allow you to combine Boolean expressions:
- AND (`&&`): Both conditions must be true.
- OR (`||`): At least one condition must be true.
- NOT (`!`): Reverses the outcome of a Boolean expression.
Examples of Boolean Expressions
Utilizing Boolean variables within if statements is commonplace. Here’s an example of a simple condition check:
if (isRaining) {
// Take an umbrella
}
If it is raining, the code inside the block executes, prompting the user to take an umbrella.
Combining Conditions
You can also combine conditions using logical operators. This enables more complex decision-making processes:
if (hasAccess && isRaining) {
// Allow entry
}
In this example, entry is allowed if the user has access and it is raining, showcasing how Boolean logic can create layered conditions.
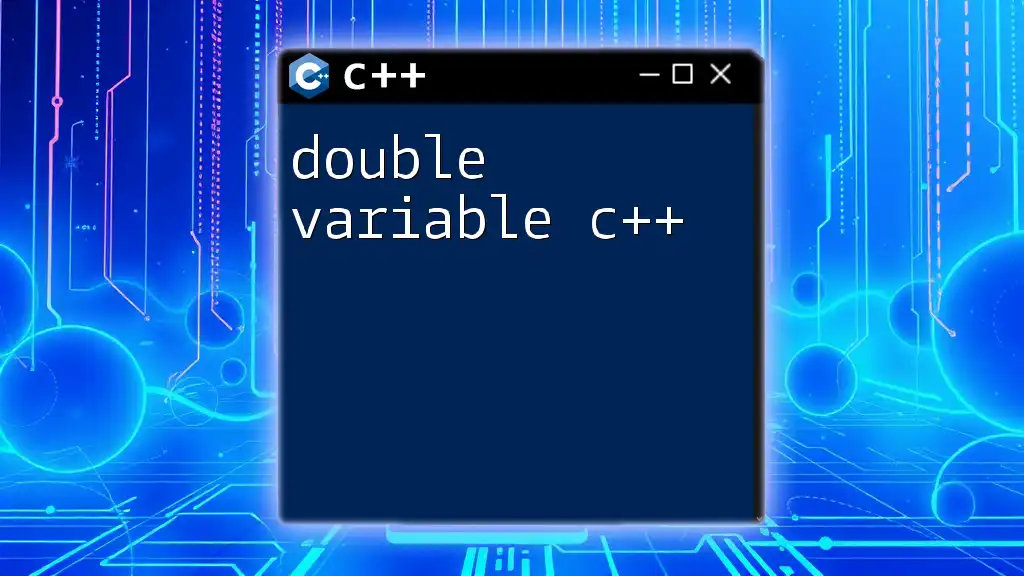
The Role of Boolean Variables in Control Flow
Boolean variables are instrumental in controlling the flow of programs through decision-making structures.
If Statements Using Boolean Variables
The `if` statement is one of the primary structures that utilize Boolean variables. For example:
if (isRaining) {
cout << "It's raining!";
}
This code checks the `isRaining` variable and, if true, outputs a warning.
Loop Control Using Boolean Flags
In addition to condition checks, Boolean variables can manage loop execution. Consider the following example:
bool keepRunning = true;
while (keepRunning) {
// Do something
keepRunning = false; // condition to end loop
}
Here, `keepRunning` acts as a flag controlling the loop's execution, demonstrating how Booleans can dictate the flow of operations in a program.
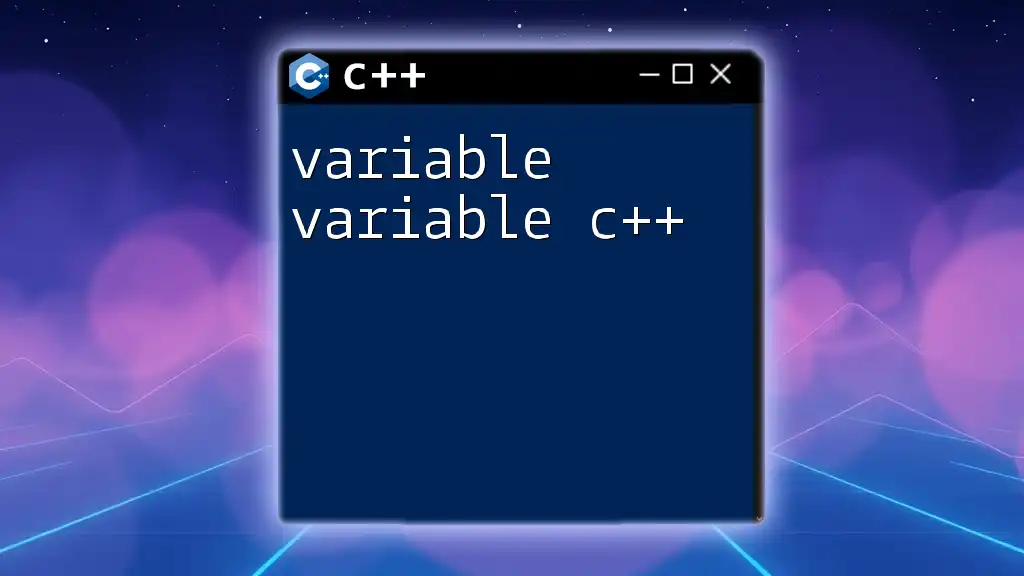
Boolean Functions in C++
Creating functions that return Boolean values is another powerful aspect of using `bool` in C++.
Creating Functions with Boolean Return Types
Functions can be designed to perform checks and return a Boolean result.
Example of a Simple Boolean Function
Here’s an example of a function that checks if a number is even:
bool isEven(int number) {
return number % 2 == 0;
}
In this function, if the number is even, it returns true; otherwise, it returns false.
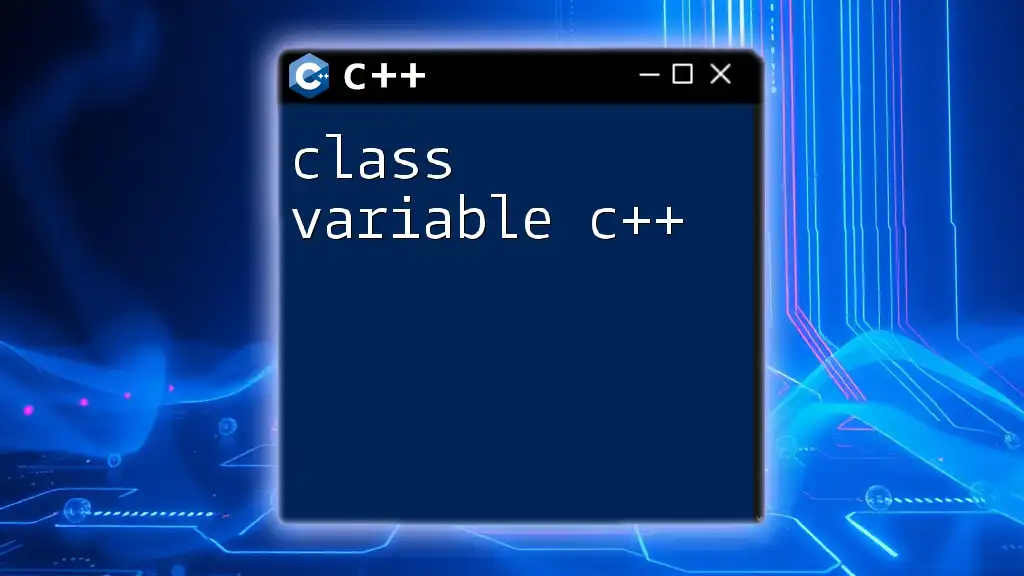
Common Use Cases for Bool Variables in C++
Booleans find application across various programming contexts. Some common use cases include:
- Validation and User Input Conditions: Check whether user inputs meet required criteria.
- State Management in Applications: Track the status of objects or processes.
- Managing Game States: Control options like Game Over or level progression.
By utilizing Boolean variables effectively, developers can streamline logic throughout their applications.
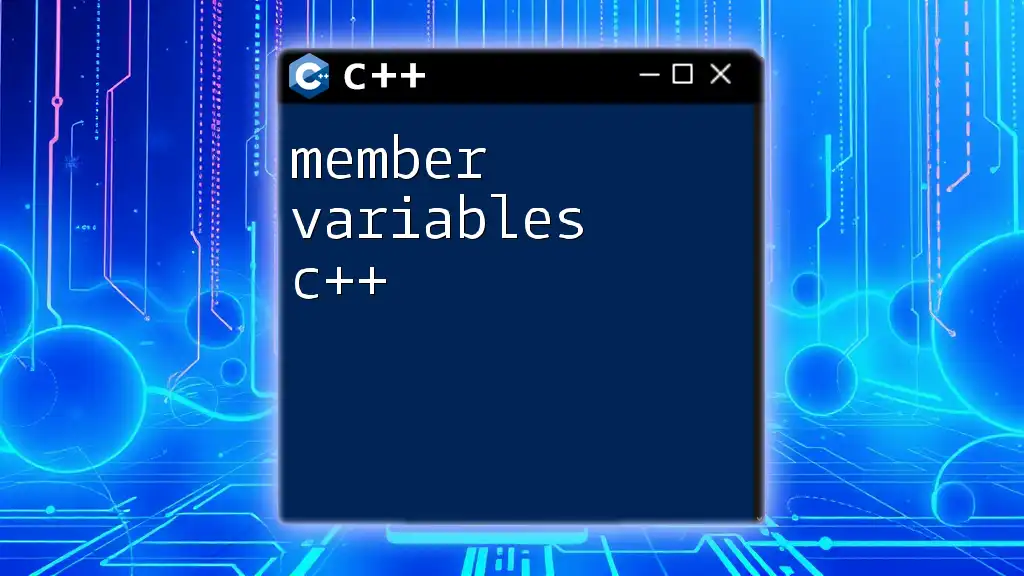
Debugging Common Issues with Bool Variables
While working with `bool` variables, certain pitfalls can occur:
Common Mistakes with Typing and Initialization
Errors often arise from incorrect initialization. Always ensure that Boolean variables are explicitly set to true or false rather than relying on default values.
Logical Errors in Boolean Logic
A frequent mistake in Boolean logic is misunderstanding operator precedence, which can yield unintended results. Carefully structure complex logic to avoid such pitfalls.
Debugging Tips
Utilize debugging tools to evaluate the state of your Boolean variables at runtime. This can help identify where logical errors may have crept in.
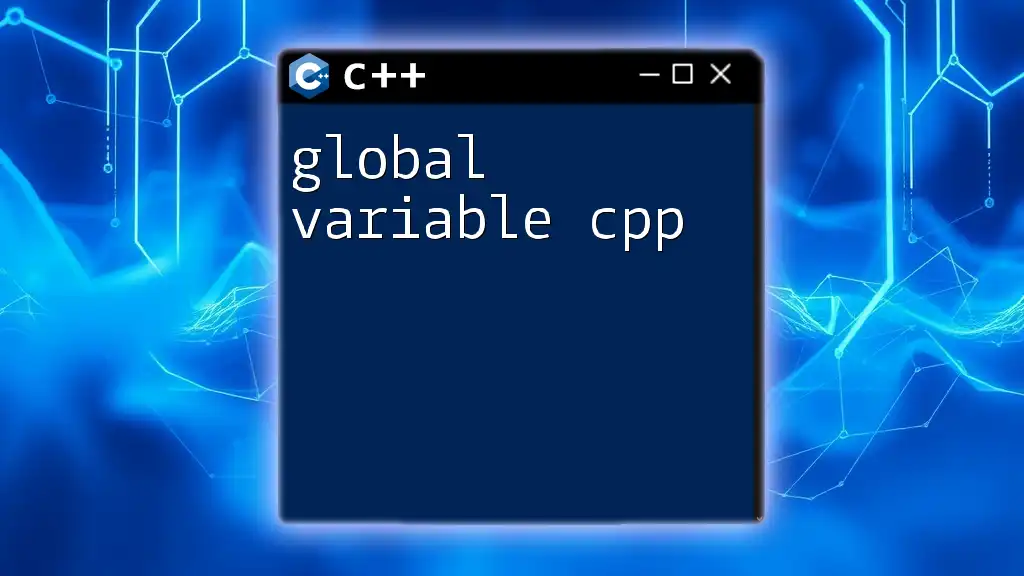
Best Practices for Using Bool Variables in C++
To maximize the clarity and effectiveness of your code, follow these best practices:
Naming Conventions for Booleans
Use clear and descriptive names for Boolean variables, such as `isAuthenticated` or `isAvailable`. This increases code readability.
Clear and Concise Conditions
Keep your conditions straightforward. Rather than using negation (`!`), aim for expressions that are easy to understand at a glance.
Avoiding Negation in Booleans for Clarity
Negations can introduce confusion. Selecting positive framing for `if` statements contributes to clearer logic, which is easier to follow and maintain.
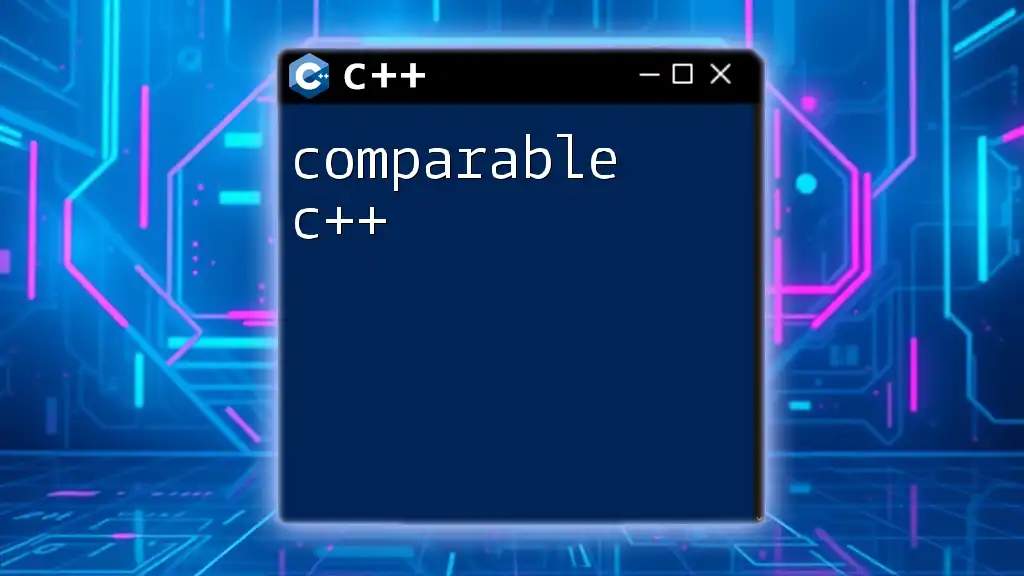
Conclusion
Understanding and utilizing bool variables in C++ is vital for effective programming. With a solid grasp of Boolean logic, conditional statements, and function returns, you'll enhance your ability to create robust applications. Practice implementing these concepts in your projects to become proficient in C++ programming.
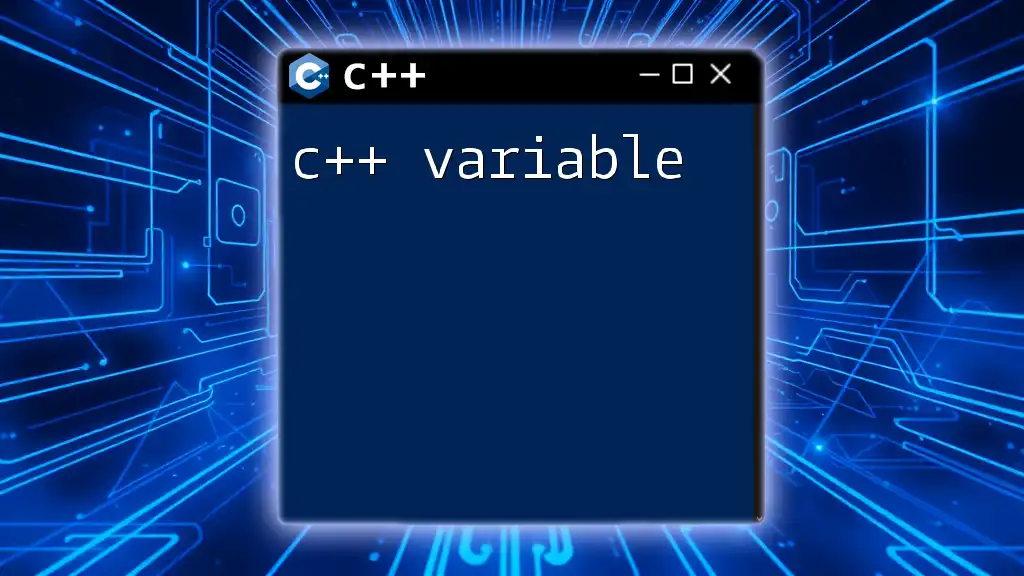
Additional Resources
For those looking to expand their knowledge, consider checking out recommended books or online courses dedicated to C++. There’s a wealth of information that can aid in mastering C++ and Boolean logic.
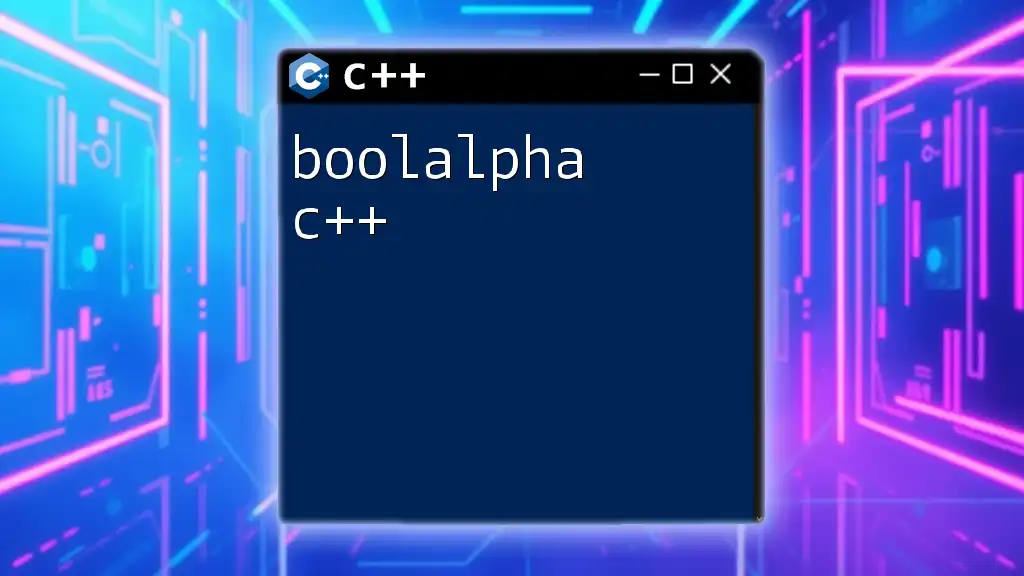
FAQs
-
What is the difference between `bool` and `boolean` in C++?
While `boolean` is often used in other programming languages, C++ specifically uses the keyword `bool` for the Boolean data type. -
How do you convert an integer to a Boolean in C++?
You can convert an integer to a Boolean by directly assigning it to a `bool` variable. Non-zero integers evaluate as true, while `0` evaluates as false. -
What are the limitations of Boolean variables in C++?
The primary limitation is their capacity to only represent two states: true or false. For more complex states, consider using enums or other data types.