SonarQube is a powerful tool for continuous inspection of code quality in C++ projects, helping developers identify bugs, vulnerabilities, and code smells to improve their software.
Here’s a simple C++ code snippet that you might analyze with SonarQube:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is SonarQube?
SonarQube is an open-source platform designed for continuous inspection of code quality. It provides detailed analysis of code to identify potential bugs, vulnerabilities, and code smells. When it comes to C++ development, leveraging SonarQube ensures that your projects maintain high standards, resulting in maintainable, reliable, and performant applications.

Why Use SonarQube for C++ Development?
Using SonarQube in your C++ projects comes with numerous benefits:
- Enhanced Code Quality: By utilizing automated code analysis, SonarQube helps surface issues before they escalate into critical problems.
- Early Detection of Bugs: SonarQube scores every piece of code, spotlighting parts that could harbor bugs, thus saving time in the debugging phase.
- Improved Security: With its built-in vulnerability detection, SonarQube assists in securing applications right from the start.
- Team Collaboration: The platform enhances code reviews through metrics and insights, fostering a culture of quality among team members.
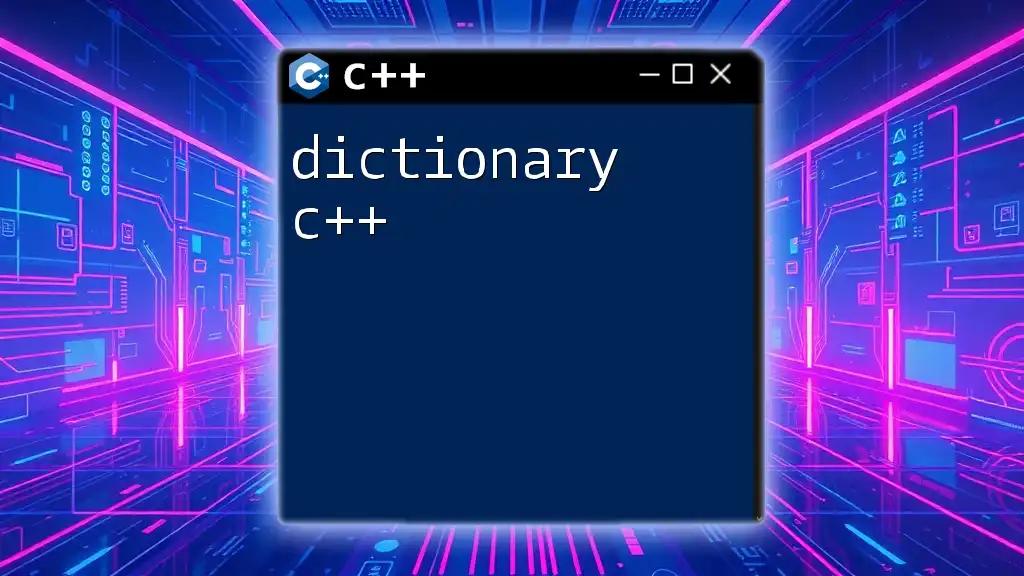
Getting Started with SonarQube
Installation and Setup
Requirements for Running SonarQube
Before you begin installing SonarQube, ensure that your system meets the necessary prerequisites, which include:
- Java Development Kit (JDK)
- Database (like PostgreSQL, MySQL, etc.)
- Sufficient memory and CPU resources
Installing SonarQube
To install SonarQube, follow these steps:
- Download the latest version of SonarQube from the [official website](https://www.sonarqube.org/downloads/).
- Extract the downloaded files to a designated folder.
- Configure the database connection by editing the `sonar.properties` file found in the configuration directory.
- Start SonarQube using the following command in your terminal from the `bin` folder:
./sonar.sh start
Setting Up a SonarQube Project
Once SonarQube is running, you can set up a new project:
- Log in to the SonarQube dashboard.
- Click on "Create Project" and fill in the required project details.
- Generate a token for authentication and link the project with your source code repository.
Integrating SonarQube with C++ Development Environments
Using SonarQube with IDEs
Integrating SonarQube with your favorite C++ Integrated Development Environment (IDE) can enhance your productivity tremendously. Most popular IDEs, such as Visual Studio and CLion, support SonarQube plugins that provide real-time code feedback. This integration allows developers to rectify issues spontaneously while writing code, rather than waiting for post-commit analysis.
Continuous Integration (CI) with SonarQube
To ensure continuous quality checks, integrating SonarQube into your CI/CD pipeline is essential. You can use tools like Jenkins, GitLab CI, or CircleCI to automate the analysis triggers. For instance, a Jenkins pipeline can be configured to execute SonarQube analysis at each build, ensuring that issues are flagged before code enters production.

Analyzing C++ Code with SonarQube
Code Quality Metrics
Key Metrics to Evaluate
SonarQube measures several critical metrics that reflect the quality of your C++ code, including:
- Bugs: Issues that can lead to incorrect behavior.
- Vulnerabilities: Potential exploits that can compromise the application.
- Code Smells: Traits indicating a deeper problem in the code that could hinder future development.
These metrics are essential for understanding your codebase's overall health and guiding your team towards improvements.
Understanding the Quality Gate
The quality gate feature in SonarQube determines the pass/fail status of your project based on the defined quality criteria. Configuring a robust quality gate specific to your C++ project helps enforce these standards consistently.
Running Code Analysis
Manual Code Analysis
To perform a manual code analysis, you will use the `sonar-scanner` command line tool. Here’s an example command you would use for analyzing your C++ project:
sonar-scanner -Dsonar.projectKey=your_project_key -Dsonar.sources=src -Dsonar.language=cpp
This command specifies your project key, where the source files are, and indicates to SonarQube that the project is a C++ application.
Automated Code Analysis
Automating code analysis is crucial for maintaining continuous quality. You can configure hooks in your version control system (VCS) to trigger SonarQube analysis automatically every time changes are pushed to the repository. This proactive approach helps catch issues early.

Utilizing SonarQube Features for C++
Code Review and Collaboration
SonarQube enhances team collaboration significantly. It provides metrics and reports that foster effective code review processes. By sharing insights and findings from SonarQube, team members can engage in informed discussions about code quality. Such collaboration makes it easier to maintain high coding standards across the board.
Custom Rules and Quality Profiles
Creating Custom Rules for C++ Analysis
SonarQube allows you to create custom rules tailored to your project's specific needs. Customizing rules can help you enforce coding standards that are unique to your development environment, ensuring that every piece of code meets team expectations.
Quality Profiles for C++ Projects
A quality profile encompasses a set of rules that define what constitutes good code within your project. Understanding how to configure and manage these profiles is essential for focusing on the issues that matter most to your C++ development process.
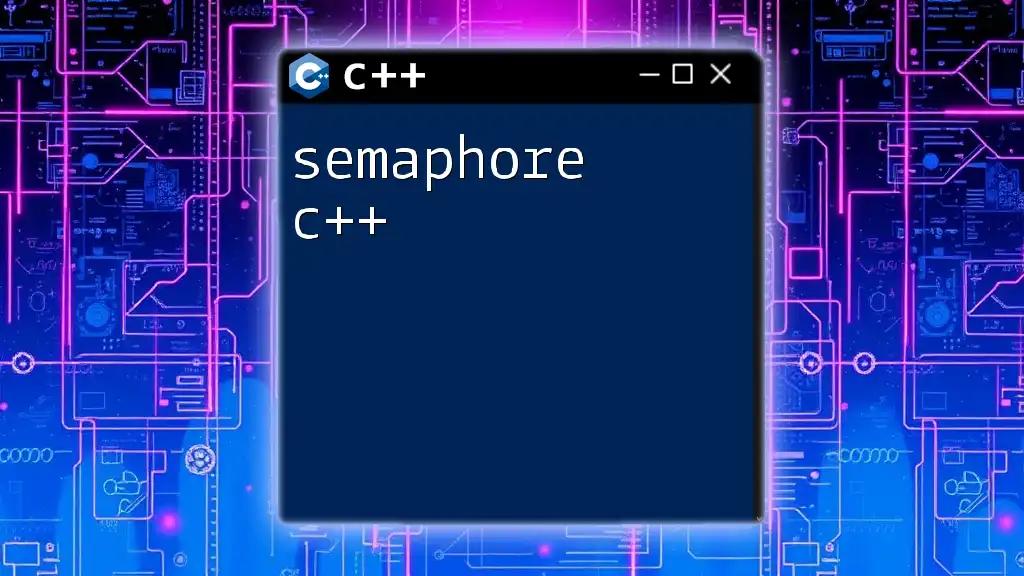
Common Challenges and Solutions
Addressing Analysis False Positives
It's common to encounter false positives when running analyses on C++ code. Understanding the reasons behind these occurrences—such as project-specific patterns or complex code structures—can help. Implement best practices for minimizing false positives, like refining custom rules and maintaining up-to-date coding standards.
Performance Optimization
When dealing with large C++ codebases, performance can become an issue. To optimize analysis time:
- Limit the scope of analysis by specifying directories or file types.
- Use incremental analysis to re-analyze only the modified parts of the codebase.
- Profile your SonarQube instance to detect performance bottlenecks and optimize configurations accordingly.
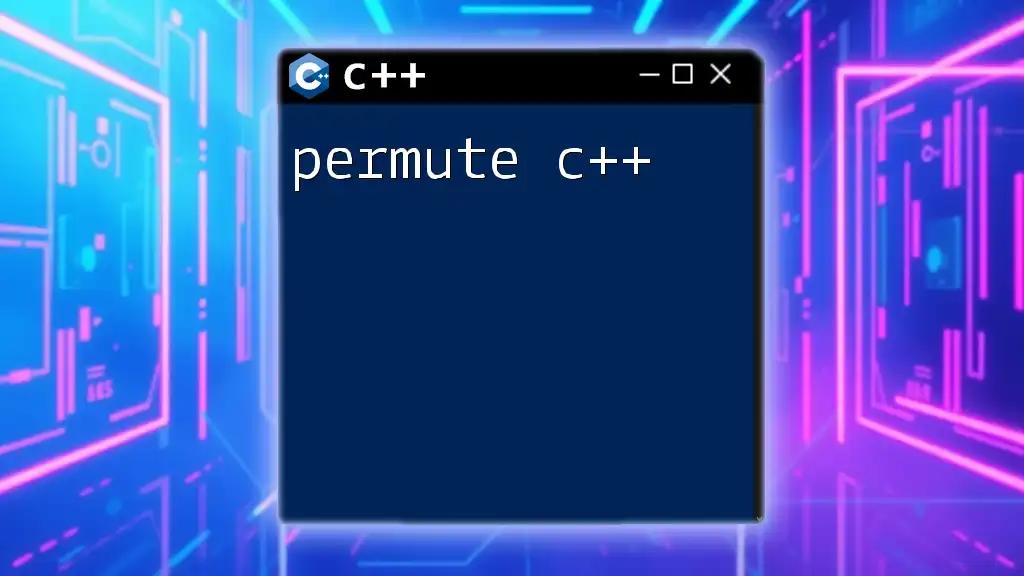
Conclusion
Incorporating SonarQube into your C++ development workflow is a strategic move to enhance code quality, foster collaboration, and ensure earlier detection of issues. By following the outlined steps, you can set up SonarQube efficiently and leverage its robust features to improve your projects substantially.
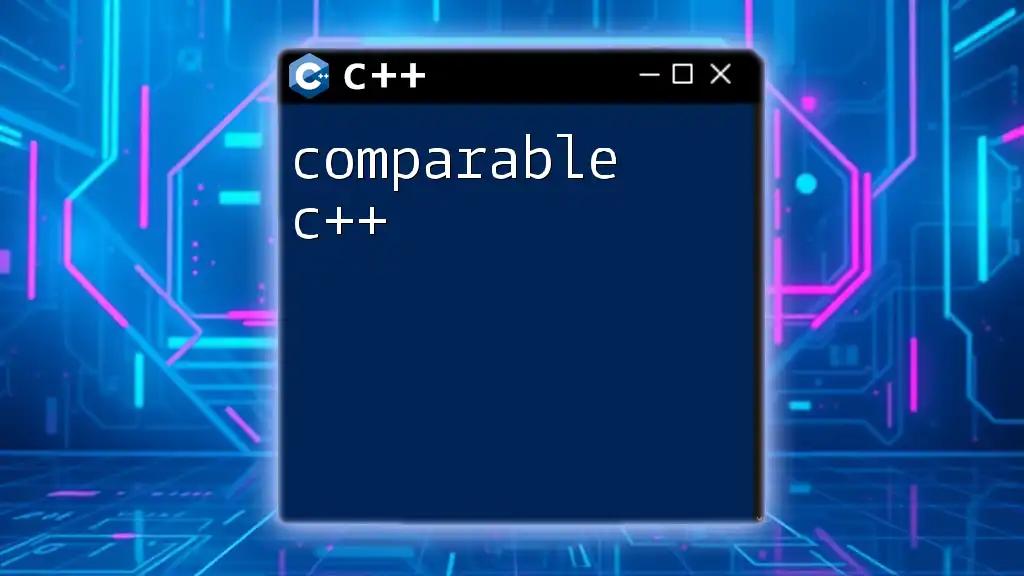
Additional Resources
To delve deeper into utilizing SonarQube with C++, consider exploring the SonarQube Documentation, participating in community forums, and looking into recommended reading materials focused on C++ and software quality assurance. These resources will help expand your understanding and elevate your code quality further.